How Can You Easily Create a New File in Python?
Creating and managing files is a fundamental skill for any programmer, and Python makes this task remarkably straightforward. Whether you’re developing a simple script to log data, building a complex application that requires file manipulation, or just experimenting with code, knowing how to create a new file in Python is essential. In this article, we will explore the various methods and best practices for file creation, providing you with the tools to effectively handle files in your projects.
Python offers a variety of built-in functions and libraries that streamline the file creation process. From the basic `open()` function to more advanced libraries that facilitate file handling, the language provides multiple ways to create and manage files efficiently. Understanding these methods not only enhances your programming skills but also empowers you to handle data more effectively, whether it’s text, JSON, or binary files.
As we delve deeper into this topic, we will cover the different modes of file creation, discuss how to handle exceptions, and highlight some common use cases. By the end of this article, you’ll have a solid grasp of how to create new files in Python, setting the stage for more complex file operations and data management tasks.
Using the Open Function
To create a new file in Python, the most common method is by utilizing the built-in `open()` function. This function allows you to specify the mode in which the file should be opened. To create a new file, you will typically use the ‘w’ (write) or ‘x’ (exclusive creation) modes.
Here is a quick overview of the file modes:
- ‘w’: Opens a file for writing. If the file already exists, it truncates the file to zero length. If it does not exist, it creates a new file.
- ‘x’: Opens a file for exclusive creation. If the file already exists, the operation fails with an error.
- ‘a’: Opens a file for appending. It creates a new file if it does not exist.
Example usage for creating a new file:
python
# Using ‘w’ mode
with open(‘newfile.txt’, ‘w’) as file:
file.write(“Hello, World!”)
# Using ‘x’ mode
try:
with open(‘exclusivefile.txt’, ‘x’) as file:
file.write(“This file is exclusively created.”)
except FileExistsError:
print(“File already exists.”)
Writing Data to the File
Once you have opened a file in write mode, you can write data to it using the `write()` method. This method writes a string to the file. If you want to write multiple lines, you can use `writelines()` which takes a list of strings.
Here is an example of writing multiple lines:
python
lines = [“First line.\n”, “Second line.\n”, “Third line.\n”]
with open(‘multilines.txt’, ‘w’) as file:
file.writelines(lines)
Handling File Paths
When creating files, it’s essential to understand how to manage file paths. You can create files in the current working directory or specify an absolute or relative path.
Path Type | Example |
---|---|
Absolute Path | `’/home/user/newfile.txt’` |
Relative Path | `’./newfile.txt’` |
Using an absolute path will ensure that the file is created in the specified location, while a relative path is created in relation to the current working directory.
Ensuring File Closure
Python automatically handles file closure when using the `with` statement, which is the preferred method for file handling. However, if you open a file without a `with` statement, you must ensure that you close it manually using the `close()` method.
Example of manual closure:
python
file = open(‘manualclose.txt’, ‘w’)
file.write(“This file will be closed manually.”)
file.close()
Failure to close files can lead to memory leaks or data not being written properly, especially in applications that open multiple files.
Creating and managing files in Python is straightforward when using the `open()` function. By understanding the different modes, handling paths correctly, and ensuring proper file closure, you can effectively manage file operations in your Python applications.
Using the Built-in Open Function
To create a new file in Python, the built-in `open()` function is primarily used. This function allows you to specify the file name and the mode in which you want to open the file. For creating a new file, you typically use the `’w’` (write) or `’x’` (exclusive creation) mode.
python
# Creating a new file using the write mode
file = open(‘newfile.txt’, ‘w’)
file.write(‘Hello, World!’)
file.close()
### Modes for File Creation
- Write (`’w’`): Opens a file for writing. If the file already exists, it truncates the file to zero length.
- Exclusive Creation (`’x’`): Opens a file for exclusive creation. If the file already exists, it raises a `FileExistsError`.
Using the With Statement
Utilizing the `with` statement when handling files is recommended as it ensures proper acquisition and release of resources. The file is automatically closed after exiting the block, even if an error occurs.
python
# Creating a new file using the with statement
with open(‘newfile.txt’, ‘w’) as file:
file.write(‘Hello, World!’)
This method enhances code readability and safety.
Handling Errors During File Creation
When creating files, it is essential to handle potential errors, especially when using exclusive creation mode. Employing try-except blocks can help manage these exceptions effectively.
python
try:
with open(‘newfile.txt’, ‘x’) as file:
file.write(‘Hello, World!’)
except FileExistsError:
print(“File already exists.”)
### Common Exceptions
- FileExistsError: Raised if the file already exists when using `’x’` mode.
- IOError: Raised for I/O-related issues, such as permission errors.
Creating Directories Before File Creation
If the directory where you want to create the file does not exist, you can create it using the `os` module. This is important for preventing `FileNotFoundError`.
python
import os
directory = ‘my_directory’
if not os.path.exists(directory):
os.makedirs(directory)
with open(os.path.join(directory, ‘newfile.txt’), ‘w’) as file:
file.write(‘Hello, World!’)
### Directory Creation Steps
- Check if the directory exists: Use `os.path.exists()`.
- Create the directory: Use `os.makedirs()` to create the directory recursively.
Writing Different Data Types to Files
When creating files, you can write various data types, such as strings, lists, or dictionaries. For complex data structures, consider using libraries like `json` for serialization.
#### Writing a List to a File
python
data = [‘Line 1’, ‘Line 2’, ‘Line 3’]
with open(‘newfile.txt’, ‘w’) as file:
for line in data:
file.write(line + ‘\n’)
#### Writing a Dictionary to a JSON File
python
import json
data = {‘name’: ‘Alice’, ‘age’: 30}
with open(‘data.json’, ‘w’) as file:
json.dump(data, file)
### Summary of Writing Methods
Data Type | Method |
---|---|
String | `file.write()` |
List | Loop through items |
Dictionary | `json.dump()` |
Expert Insights on Creating Files in Python
Dr. Emily Carter (Senior Software Engineer, Code Innovations). “Creating a new file in Python is straightforward and can be accomplished using the built-in `open()` function. It is essential to specify the mode, such as ‘w’ for writing, to ensure the file is created correctly.”
Michael Thompson (Python Developer Advocate, Tech Solutions Inc.). “When working with file creation in Python, it is crucial to handle exceptions properly. Utilizing a `try-except` block can prevent your application from crashing due to issues like permission errors or disk space limitations.”
Lisa Chen (Data Scientist, Analytics Hub). “For data-intensive applications, I recommend using context managers with the `with` statement when creating files. This approach ensures that files are properly closed after their use, which is vital for resource management.”
Frequently Asked Questions (FAQs)
How do I create a new file in Python?
To create a new file in Python, use the built-in `open()` function with the mode set to `’w’` or `’x’`. For example, `open(‘newfile.txt’, ‘w’)` will create a new file named `newfile.txt`. If the file already exists, using `’w’` will overwrite it, while `’x’` will raise an error.
What is the difference between ‘w’ and ‘x’ modes in file creation?
The `’w’` mode opens a file for writing and will create the file if it does not exist, but it will overwrite the file if it does. The `’x’` mode, on the other hand, is used to create a new file and will raise a `FileExistsError` if the file already exists.
Can I create a new file in a specific directory?
Yes, you can create a new file in a specific directory by providing the full path in the `open()` function. For example, `open(‘/path/to/directory/newfile.txt’, ‘w’)` will create `newfile.txt` in the specified directory.
How do I write content to a new file in Python?
To write content to a new file, first create the file using `open()` in write mode, then use the `write()` method. For example:
python
with open(‘newfile.txt’, ‘w’) as file:
file.write(‘Hello, World!’)
This code creates `newfile.txt` and writes “Hello, World!” to it.
What should I do if I want to append content to an existing file?
To append content to an existing file, use the `’a’` mode in the `open()` function. For example, `open(‘existingfile.txt’, ‘a’)` allows you to add new content without overwriting the existing data.
Is it necessary to close a file after creating it?
Yes, it is essential to close a file after creating it to ensure that all data is flushed from the buffer and resources are released. This can be done using the `close()` method or by using a `with` statement, which automatically handles closing the file.
Creating a new file in Python is a straightforward process that can be accomplished using built-in functions and methods. The most common way to create a file is by using the `open()` function with the mode set to ‘w’ (write) or ‘x’ (exclusive creation). The ‘w’ mode will create a new file or overwrite an existing one, while the ‘x’ mode will raise an error if the file already exists, ensuring that you do not accidentally overwrite important data.
Additionally, it is essential to handle file operations within a context manager using the `with` statement. This approach ensures that the file is properly closed after its suite finishes, even if an error occurs during the file operation. This practice enhances code reliability and resource management. Furthermore, developers can write data to the newly created file using methods like `write()` or `writelines()`, which allow for both single string and list inputs respectively.
In summary, the process of creating a new file in Python involves using the `open()` function with appropriate modes, leveraging context managers for better resource handling, and employing various methods to write data. Understanding these fundamental concepts not only aids in effective file management but also enhances overall programming proficiency in Python.
Author Profile
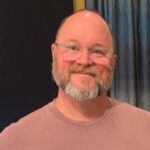
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?