How Can You Create a List in TypeScript: A Step-by-Step Guide?
Creating a list in TypeScript is an essential skill for any developer looking to harness the power of this statically typed superset of JavaScript. As applications grow in complexity, the need for structured data management becomes increasingly critical. TypeScript offers robust features that not only enhance code readability and maintainability but also provide type safety, which helps prevent common errors during development. Whether you’re building a simple application or a large-scale enterprise solution, understanding how to effectively create and manipulate lists in TypeScript will empower you to write more efficient and reliable code.
At its core, a list in TypeScript is typically represented using arrays, which can hold multiple values of a specific type. This allows developers to leverage the full potential of TypeScript’s type system, ensuring that the data being processed adheres to the expected structure. By utilizing interfaces and generics, you can create lists that are not only type-safe but also flexible enough to accommodate various data forms. This combination of features makes TypeScript an ideal choice for managing collections of data in a way that is both intuitive and powerful.
As we delve deeper into the topic, we will explore various methods for creating lists, manipulating their contents, and leveraging TypeScript’s rich type system to ensure that your code remains robust and error-free. From basic
Defining a List in TypeScript
To create a list in TypeScript, you can utilize arrays, which are a fundamental data structure. Arrays can hold multiple values of the same type, allowing for easy manipulation and iteration through their elements.
You can define an array by specifying its type followed by square brackets. For example:
“`typescript
let numbers: number[] = [1, 2, 3, 4, 5];
“`
This declaration creates an array of numbers. Similarly, you can create arrays for strings or any other data type:
“`typescript
let fruits: string[] = [‘apple’, ‘banana’, ‘cherry’];
“`
Using Tuples for Lists with Fixed Sizes
If you need a list with a fixed number of elements, you can use tuples. Tuples allow you to define an array with different types and fixed lengths. For instance:
“`typescript
let person: [string, number] = [‘Alice’, 30];
“`
This tuple contains a string and a number. You can access tuple elements similarly to arrays, using their index:
“`typescript
console.log(person[0]); // Alice
“`
Iterating Over Lists
Iterating over lists in TypeScript can be done using various methods, with the most common being the `for` loop, `forEach`, and the `map` function. Here is how each method works:
- For Loop:
“`typescript
for (let i = 0; i < numbers.length; i++) {
console.log(numbers[i]);
}
```
- **forEach Method**:
```typescript
fruits.forEach(fruit => {
console.log(fruit);
});
“`
– **map Method**:
The `map` function creates a new array by applying a function to each element.
“`typescript
let upperFruits = fruits.map(fruit => fruit.toUpperCase());
“`
Array Methods in TypeScript
TypeScript supports a variety of array methods for manipulating lists, making it more versatile. Here are some commonly used methods:
Method | Description | Example |
---|---|---|
push | Adds one or more elements to the end of the array | `numbers.push(6);` |
pop | Removes the last element from the array | `let last = numbers.pop();` |
shift | Removes the first element from the array | `let first = fruits.shift();` |
unshift | Adds one or more elements to the beginning of the array | `fruits.unshift(‘orange’);` |
slice | Returns a shallow copy of a portion of the array | `let someFruits = fruits.slice(1, 3);` |
splice | Changes the contents of an array by removing or replacing elements | `fruits.splice(1, 1, ‘kiwi’);` |
These methods provide powerful tools for managing lists effectively in TypeScript.
Type Safety with Lists
One of the significant advantages of TypeScript is its type safety, which prevents runtime errors due to type mismatches. When you define an array, TypeScript checks that all elements conform to the specified type. For example:
“`typescript
let mixedArray: (string | number)[] = [‘hello’, 42];
“`
In this case, `mixedArray` can hold both strings and numbers, providing flexibility while maintaining type safety. Attempting to add an element of an incompatible type will result in a compile-time error, which helps catch issues early in the development process.
Creating a List in TypeScript
To create a list in TypeScript, you can utilize the built-in array type. TypeScript enhances JavaScript arrays by allowing you to define the type of elements contained within them. This provides better type safety and helps catch errors at compile time.
Defining an Array
You can define an array in TypeScript in several ways. Here are the most common methods:
- Using the Array Generic Type:
“`typescript
let numbers: Array
“`
- Using the Type Annotation with Square Brackets:
“`typescript
let fruits: string[] = [‘apple’, ‘banana’, ‘cherry’];
“`
Both methods achieve the same result, and the choice depends on your preference for readability and consistency.
Initializing an Array
Arrays can be initialized with values at the time of declaration or left empty for later use.
- With Values:
“`typescript
let colors: string[] = [‘red’, ‘green’, ‘blue’];
“`
- Empty Initialization:
“`typescript
let emptyArray: number[] = [];
“`
You can then add elements to the empty array using methods like `.push()`.
Accessing Array Elements
Access elements in an array using the index, starting from zero. For example, to access the first element:
“`typescript
let firstColor: string = colors[0]; // ‘red’
“`
TypeScript will enforce the type of the accessed element.
Iterating Over an Array
You can iterate through arrays using various methods, such as `for` loops, `forEach`, or the `map` function:
- Using a for loop:
“`typescript
for (let i = 0; i < colors.length; i++) {
console.log(colors[i]);
}
```
- **Using forEach**:
```typescript
colors.forEach(color => {
console.log(color);
});
“`
– **Using map**:
“`typescript
const upperColors = colors.map(color => color.toUpperCase());
“`
Multidimensional Arrays
TypeScript supports multidimensional arrays, which can be declared as follows:
“`typescript
let matrix: number[][] = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
];
“`
Accessing elements in a multidimensional array requires two indices:
“`typescript
let element: number = matrix[1][2]; // 6
“`
Array Methods
TypeScript arrays come with various built-in methods that enhance their functionality. Below is a table of commonly used array methods:
Method | Description |
---|---|
`push()` | Adds one or more elements to the end |
`pop()` | Removes the last element |
`shift()` | Removes the first element |
`unshift()` | Adds one or more elements to the start |
`slice()` | Returns a shallow copy of a portion |
`splice()` | Adds or removes elements from an array |
`sort()` | Sorts the elements of an array |
Using these methods, you can manipulate arrays efficiently while leveraging TypeScript’s type system for safety and clarity.
Expert Insights on Creating Lists in TypeScript
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Creating a list in TypeScript can be efficiently achieved using arrays. TypeScript’s strong typing system allows developers to define the type of elements in the array, enhancing code reliability and reducing runtime errors.”
Michael Chen (Lead Developer, CodeCraft Solutions). “When working with lists in TypeScript, leveraging the built-in array methods such as `map`, `filter`, and `reduce` can significantly streamline data manipulation. These methods not only improve code readability but also align with functional programming principles.”
Sarah Thompson (Technical Writer, Dev Insights Magazine). “TypeScript offers a powerful feature known as tuples, which allows for lists with fixed sizes and specific types. This can be particularly useful when you need to create a list where each element has a different type, ensuring better data integrity.”
Frequently Asked Questions (FAQs)
How do I create a simple list in TypeScript?
To create a simple list in TypeScript, you can use an array. For example: `let myList: number[] = [1, 2, 3, 4];` This defines an array of numbers.
Can I create a list of objects in TypeScript?
Yes, you can create a list of objects by defining an interface or type for the object structure. For instance:
“`typescript
interface Person {
name: string;
age: number;
}
let people: Person[] = [{ name: ‘Alice’, age: 30 }, { name: ‘Bob’, age: 25 }];
“`
What is the difference between a tuple and an array in TypeScript?
A tuple is a fixed-size array where each element can have a different type, while an array can contain multiple elements of the same type. For example:
“`typescript
let tuple: [string, number] = [‘Alice’, 30];
let array: number[] = [1, 2, 3];
“`
How can I initialize an empty list in TypeScript?
You can initialize an empty list by declaring an array without any elements. For example: `let emptyList: string[] = [];` This creates an empty array that can hold strings.
Is it possible to create a list with mixed types in TypeScript?
Yes, you can create a list with mixed types using a union type. For example: `let mixedList: (string | number)[] = [‘Alice’, 30, ‘Bob’];` This allows the array to contain both strings and numbers.
How do I add items to a list in TypeScript?
You can add items to a list using the `push` method. For example:
“`typescript
let myList: number[] = [];
myList.push(1);
myList.push(2);
“`
This appends the specified items to the end of the array.
Creating a list in TypeScript is a straightforward process that leverages the language’s strong typing system to enhance code reliability and maintainability. TypeScript allows developers to define arrays with specific data types, ensuring that only the intended types of elements can be added to the list. This feature significantly reduces runtime errors and improves code clarity, making it easier to understand the data structures being used.
To create a list in TypeScript, one can utilize the array syntax along with type annotations. For instance, declaring a list of numbers can be done using `let numbers: number[] = [];`, while a list of strings can be defined as `let names: string[] = [];`. Additionally, TypeScript supports tuples and generics, providing further flexibility in defining lists that may contain mixed types or require more complex structures.
Key takeaways from this discussion include the importance of type safety in TypeScript, which helps prevent common programming errors. Furthermore, understanding how to effectively use arrays and other collection types in TypeScript can greatly enhance a developer’s ability to write robust and efficient code. Overall, mastering list creation and manipulation in TypeScript is an essential skill for any developer looking to leverage the full capabilities of the language.
Author Profile
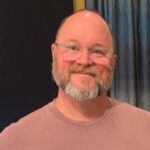
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?