How Can You Easily Convert a String to a Date in Java?
In the world of programming, handling dates and times is a fundamental skill that every developer must master. Java, a widely-used programming language, offers robust tools and libraries for managing date and time data. However, one common challenge that many programmers encounter is converting strings into date objects. This seemingly simple task can often lead to confusion, especially when dealing with various date formats and localization issues. In this article, we will explore the intricacies of converting strings to dates in Java, equipping you with the knowledge to navigate this essential aspect of date manipulation confidently.
Understanding how to convert a string to a date in Java is crucial for applications that rely on user input or external data sources. The process typically involves utilizing Java’s built-in classes, such as `SimpleDateFormat` and the more modern `DateTimeFormatter`, which provide flexible ways to parse and format date strings. Each approach has its nuances, and knowing when to use each can significantly enhance your application’s performance and reliability.
Moreover, as Java has evolved, so too have its date and time APIs. The of the `java.time` package in Java 8 brought a new level of sophistication to date handling, making it easier to work with time zones and immutable date objects. This article will guide you through the various
Understanding Java Date and Time API
Java’s date and time handling has evolved significantly with the of the Java 8 Date and Time API. The new API is built around the `java.time` package, which provides a more comprehensive and flexible approach to date and time manipulation compared to the older `java.util.Date` and `java.util.Calendar` classes. The primary components of the new API include:
- `LocalDate`: Represents a date without time-zone.
- `LocalTime`: Represents a time without date.
- `LocalDateTime`: Combines date and time without time-zone.
- `ZonedDateTime`: Represents date and time with time-zone.
This enhancement allows developers to work with dates and times in a more intuitive way, minimizing errors related to time zones and daylight saving time.
Converting String to Date Using Java 8
To convert a `String` to a `Date` object in Java 8 and later, the recommended approach is to use the `LocalDate`, `LocalTime`, or `LocalDateTime` classes along with the `DateTimeFormatter` class. The conversion process typically involves defining a format that matches the input string.
Here is an example of how to convert a `String` to a `LocalDate`:
“`java
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
public class StringToDateExample {
public static void main(String[] args) {
String dateString = “2023-10-15”;
DateTimeFormatter formatter = DateTimeFormatter.ofPattern(“yyyy-MM-dd”);
LocalDate date = LocalDate.parse(dateString, formatter);
System.out.println(“Converted LocalDate: ” + date);
}
}
“`
Common Date Formats
When converting strings to dates, it is essential to ensure that the format matches the input string. Below is a list of common date formats and their corresponding patterns:
Format Description | Pattern |
---|---|
ISO Local Date | yyyy-MM-dd |
ISO Local Date Time | yyyy-MM-dd’T’HH:mm:ss |
Custom Date Format | dd/MM/yyyy |
Month Day Year | MMMM dd, yyyy |
Handling Exceptions
When converting a string to a date, it is crucial to handle potential exceptions that may arise from incorrect formats or invalid dates. The `DateTimeParseException` can be thrown if the input string does not match the expected format.
Example of exception handling:
“`java
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
import java.time.format.DateTimeParseException;
public class SafeStringToDate {
public static void main(String[] args) {
String dateString = “2023-15-10”; // Invalid date
DateTimeFormatter formatter = DateTimeFormatter.ofPattern(“yyyy-MM-dd”);
try {
LocalDate date = LocalDate.parse(dateString, formatter);
System.out.println(“Converted LocalDate: ” + date);
} catch (DateTimeParseException e) {
System.out.println(“Invalid date format: ” + e.getMessage());
}
}
}
“`
This code snippet demonstrates how to gracefully handle parsing errors, ensuring that the program does not crash due to unhandled exceptions.
Using SimpleDateFormat
The `SimpleDateFormat` class is one of the most commonly used methods for converting strings to dates in Java. This class allows for parsing and formatting dates based on a specified pattern.
“`java
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Date;
public class DateConversion {
public static void main(String[] args) {
String dateString = “2023-10-04”;
SimpleDateFormat formatter = new SimpleDateFormat(“yyyy-MM-dd”);
try {
Date date = formatter.parse(dateString);
System.out.println(“Converted Date: ” + date);
} catch (ParseException e) {
e.printStackTrace();
}
}
}
“`
- Pattern Explanation:
- `yyyy`: Year in four digits
- `MM`: Month in two digits (01-12)
- `dd`: Day in two digits (01-31)
Using LocalDate and DateTimeFormatter
For applications that require a more modern approach, Java 8 introduced the `java.time` package, which includes the `LocalDate` class and `DateTimeFormatter` for date conversion.
“`java
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
import java.time.format.DateTimeParseException;
public class LocalDateConversion {
public static void main(String[] args) {
String dateString = “2023-10-04”;
DateTimeFormatter formatter = DateTimeFormatter.ofPattern(“yyyy-MM-dd”);
try {
LocalDate date = LocalDate.parse(dateString, formatter);
System.out.println(“Converted LocalDate: ” + date);
} catch (DateTimeParseException e) {
e.printStackTrace();
}
}
}
“`
- Benefits of Using LocalDate:
- Immutable and thread-safe
- More intuitive API
- Represents a date without time-zone
Handling Different Date Formats
When dealing with various string formats, it’s essential to adapt the parsing logic accordingly. Below are examples of different formats and how to handle them.
Format | Example | Pattern |
---|---|---|
ISO Local Date | 2023-10-04 | yyyy-MM-dd |
US Format | 10/04/2023 | MM/dd/yyyy |
Custom Format | 04-Oct-2023 | dd-MMM-yyyy |
“`java
String[] dateStrings = {“2023-10-04”, “10/04/2023”, “04-Oct-2023”};
String[] patterns = {“yyyy-MM-dd”, “MM/dd/yyyy”, “dd-MMM-yyyy”};
for (int i = 0; i < dateStrings.length; i++) { DateTimeFormatter formatter = DateTimeFormatter.ofPattern(patterns[i]); try { LocalDate date = LocalDate.parse(dateStrings[i], formatter); System.out.println("Converted Date: " + date); } catch (DateTimeParseException e) { System.out.println("Error parsing date: " + dateStrings[i]); } } ```
Timezone Considerations
When converting strings to dates, consider the time zone. Use the `ZonedDateTime` class if you need to account for time zones.
“`java
import java.time.ZonedDateTime;
import java.time.format.DateTimeFormatter;
public class ZonedDateTimeConversion {
public static void main(String[] args) {
String dateString = “2023-10-04T10:15:30+01:00[Europe/Paris]”;
DateTimeFormatter formatter = DateTimeFormatter.ISO_ZONED_DATE_TIME;
ZonedDateTime zonedDateTime = ZonedDateTime.parse(dateString, formatter);
System.out.println(“Converted ZonedDateTime: ” + zonedDateTime);
}
}
“`
- Key Points:
- Ensure the string includes time zone information.
- Use `ZonedDateTime` for time zone-aware dates.
By utilizing these various methods and classes, Java developers can effectively convert strings to date objects while accommodating different formats and time zones.
Expert Insights on Converting Strings to Dates in Java
Dr. Emily Carter (Senior Software Engineer, Tech Solutions Inc.). “When converting strings to dates in Java, it is crucial to use the `SimpleDateFormat` class, which allows for flexible date parsing. However, developers should be aware of the potential pitfalls related to date formats and locale settings to avoid unexpected results.”
Michael Thompson (Java Development Consultant, CodeCraft). “Utilizing the `LocalDate` and `DateTimeFormatter` classes from the `java.time` package is highly recommended. This approach not only simplifies the conversion process but also enhances code readability and maintainability, especially when dealing with different date formats.”
Sarah Jenkins (Lead Java Architect, Innovative Software Solutions). “It is essential to handle exceptions properly during string-to-date conversions. Implementing try-catch blocks can prevent runtime errors and ensure that your application can gracefully manage invalid date formats, which is a common issue in real-world applications.”
Frequently Asked Questions (FAQs)
How can I convert a string to a date in Java?
You can convert a string to a date in Java using the `SimpleDateFormat` class. Create an instance of `SimpleDateFormat` with the desired date format and then call the `parse` method, passing the string to be converted.
What format should the string be in for successful conversion?
The string should match the format specified in the `SimpleDateFormat` instance. For example, if you use `new SimpleDateFormat(“yyyy-MM-dd”)`, the string should be in the format “2023-10-01”.
What exceptions should I handle when converting a string to a date?
You should handle `ParseException`, which is thrown if the string cannot be parsed into a date according to the specified format. It is advisable to also handle `NullPointerException` if the input string is null.
Can I use the `java.time` package for converting strings to dates?
Yes, starting from Java 8, you can use the `java.time` package. The `LocalDate` and `LocalDateTime` classes provide the `parse` method, which can convert strings directly to date objects without needing a specific format if the string is in ISO-8601 format.
What is the difference between `SimpleDateFormat` and `DateTimeFormatter`?
`SimpleDateFormat` is part of the older `java.util` package and is not thread-safe. `DateTimeFormatter`, introduced in Java 8, is part of the `java.time` package, is immutable, and is thread-safe, making it a better choice for modern applications.
How do I handle different date formats when converting strings?
You can create multiple `SimpleDateFormat` or `DateTimeFormatter` instances for different formats and attempt to parse the string with each format until one succeeds. Alternatively, you can use a `try-catch` block to catch exceptions for each format until a successful conversion occurs.
Converting a string to a date in Java is a fundamental task that is essential for handling date and time data effectively. Java provides the `SimpleDateFormat` class in the `java.text` package, which allows developers to define a specific date format and parse strings accordingly. This approach is particularly useful when dealing with user input or data from external sources, ensuring that the string representation of a date can be accurately transformed into a `Date` object.
Another important aspect to consider is the of the `java.time` package in Java 8, which offers a more modern and flexible approach to date and time manipulation. The `DateTimeFormatter` class in this package is recommended for new applications, as it provides a more robust and thread-safe way to parse and format dates. The use of `LocalDate`, `LocalDateTime`, and `ZonedDateTime` enhances the ability to work with dates in a more intuitive manner, accommodating various time zones and date-time representations.
When converting strings to dates, it is crucial to handle exceptions that may arise due to invalid formats or parsing errors. Utilizing try-catch blocks can help manage these scenarios gracefully, ensuring that the application remains robust and user-friendly. Additionally, developers should
Author Profile
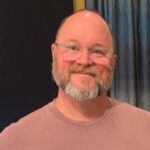
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?