How Can You Convert a List to a Dictionary in Python?
In the world of programming, data structures are the backbone of efficient coding and data manipulation. Among these structures, lists and dictionaries are two of the most commonly used in Python. While lists provide a simple way to store ordered collections of items, dictionaries offer a powerful mechanism for associating keys with values, enabling quick lookups and data retrieval. If you’ve ever found yourself needing to convert a list into a dictionary, whether for data organization, analysis, or simply to leverage the unique capabilities of dictionaries, you’re in the right place.
Converting a list to a dictionary in Python can seem daunting at first, especially for those new to the language. However, with a few straightforward techniques and an understanding of how these data structures work, you can easily transform your lists into dictionaries. This process not only enhances your coding efficiency but also opens up a new realm of possibilities for data manipulation and retrieval.
In this article, we will explore various methods to achieve this conversion, highlighting the benefits and potential use cases for each approach. Whether you’re dealing with lists of tuples, pairs, or even single lists, you’ll discover how to harness the power of Python’s built-in functions and comprehensions to create dictionaries that suit your needs. Prepare to elevate your Python programming skills as we delve into the art of
Using the `dict()` Constructor
One of the simplest ways to convert a list into a dictionary in Python is by using the built-in `dict()` constructor. This method is particularly effective when you have a list of tuples, where each tuple contains a key-value pair.
For instance, consider the following list of tuples:
python
data = [(‘a’, 1), (‘b’, 2), (‘c’, 3)]
You can convert this list to a dictionary as follows:
python
result = dict(data)
print(result) # Output: {‘a’: 1, ‘b’: 2, ‘c’: 3}
This approach is straightforward and concise, making it a preferred choice for many developers.
Using Dictionary Comprehension
Another powerful method to convert a list to a dictionary is through dictionary comprehension. This technique allows for more flexibility and can be particularly useful when you need to manipulate the data during the conversion.
For example, consider a list of keys and a corresponding list of values:
python
keys = [‘a’, ‘b’, ‘c’]
values = [1, 2, 3]
You can create a dictionary that maps keys to values like this:
python
result = {keys[i]: values[i] for i in range(len(keys))}
print(result) # Output: {‘a’: 1, ‘b’: 2, ‘c’: 3}
This method is not only efficient but also allows for additional logic to be implemented within the comprehension.
Using the `zip()` Function
The `zip()` function is another efficient way to convert two lists into a dictionary. This function pairs elements from two lists together, creating tuples that can be directly converted into a dictionary.
For example:
python
keys = [‘a’, ‘b’, ‘c’]
values = [1, 2, 3]
result = dict(zip(keys, values))
print(result) # Output: {‘a’: 1, ‘b’: 2, ‘c’: 3}
This method is highly readable and eliminates the need for explicit indexing, making it a clean solution for such conversions.
Converting a List of Lists
If you have a list of lists where each inner list contains two elements (the first being the key and the second being the value), you can convert it to a dictionary using the `dict()` constructor or dictionary comprehension as well.
For instance:
python
data = [[‘a’, 1], [‘b’, 2], [‘c’, 3]]
result = dict(data)
print(result) # Output: {‘a’: 1, ‘b’: 2, ‘c’: 3}
Alternatively, using dictionary comprehension:
python
result = {item[0]: item[1] for item in data}
print(result) # Output: {‘a’: 1, ‘b’: 2, ‘c’: 3}
Handling Duplicate Keys
When converting lists to dictionaries, one must consider how to handle duplicate keys. In Python, a dictionary cannot have duplicate keys; if duplicates are present, the last occurrence will overwrite previous ones.
For example:
python
data = [(‘a’, 1), (‘b’, 2), (‘a’, 3)]
result = dict(data)
print(result) # Output: {‘a’: 3, ‘b’: 2}
To manage this, you may want to aggregate values for duplicate keys. Here’s how you can do that:
python
from collections import defaultdict
data = [(‘a’, 1), (‘b’, 2), (‘a’, 3)]
result = defaultdict(list)
for key, value in data:
result[key].append(value)
# Convert defaultdict to a regular dictionary
final_result = dict(result)
print(final_result) # Output: {‘a’: [1, 3], ‘b’: [2]}
This approach allows you to retain all values associated with a key.
Performance Considerations
When selecting a method for converting lists to dictionaries, performance may vary based on the size of the data and the specific requirements of your application. Here’s a quick comparison:
Method | Complexity | Use Case |
---|---|---|
dict() Constructor | O(n) | Simple key-value pairs |
Dictionary Comprehension | O(n) | Manipulation during conversion |
zip() Function | O(n) | Combining two lists |
Handling Duplicates | O(n) | Aggregating values |
By considering the data structure and requirements, you can choose the most efficient method for your specific use case.
Methods to Convert List to Dictionary
In Python, converting a list to a dictionary can be accomplished through various methods, depending on the structure of the list and the desired outcome. Below are some common approaches:
Using a List of Tuples
When the list contains tuples, where each tuple represents a key-value pair, the `dict()` constructor can be utilized effectively.
python
list_of_tuples = [(‘a’, 1), (‘b’, 2), (‘c’, 3)]
dictionary = dict(list_of_tuples)
Result:
python
{‘a’: 1, ‘b’: 2, ‘c’: 3}
Using Two Lists
If you have two separate lists—one for keys and another for values—you can use the `zip()` function combined with `dict()` to create a dictionary.
python
keys = [‘a’, ‘b’, ‘c’]
values = [1, 2, 3]
dictionary = dict(zip(keys, values))
Result:
python
{‘a’: 1, ‘b’: 2, ‘c’: 3}
Using List Comprehension
For lists that contain pairs of elements, list comprehension can facilitate dictionary creation. This method is particularly useful when you need to apply conditions or transformations.
python
pairs = [‘a:1’, ‘b:2’, ‘c:3’]
dictionary = {k: int(v) for k, v in (pair.split(‘:’) for pair in pairs)}
Result:
python
{‘a’: 1, ‘b’: 2, ‘c’: 3}
Using the `dict.fromkeys()` Method
When you want to create a dictionary from a list of keys with a default value, `dict.fromkeys()` is the ideal choice.
python
keys = [‘a’, ‘b’, ‘c’]
dictionary = dict.fromkeys(keys, 0)
Result:
python
{‘a’: 0, ‘b’: 0, ‘c’: 0}
Using the `defaultdict` from the collections Module
For more complex scenarios, such as grouping values, `defaultdict` can be employed. This approach is beneficial when values need to be accumulated or categorized.
python
from collections import defaultdict
list_of_items = [(‘a’, 1), (‘b’, 2), (‘a’, 3)]
dictionary = defaultdict(list)
for key, value in list_of_items:
dictionary[key].append(value)
Result:
python
defaultdict(
Performance Considerations
When converting lists to dictionaries, it is crucial to consider performance, especially with large datasets. Here’s a comparative overview of the methods:
Method | Time Complexity | Use Case |
---|---|---|
`dict()` from tuples | O(n) | Simple key-value pairs |
`zip()` with two lists | O(n) | Separate lists for keys and values |
List comprehension | O(n) | Conditional transformations |
`dict.fromkeys()` | O(n) | Setting default values for keys |
`defaultdict` | O(n) | Grouping or accumulating values |
Selecting the appropriate method based on your data structure and requirements will lead to more efficient and readable code.
Expert Insights on Converting Lists to Dictionaries in Python
Dr. Emily Carter (Senior Data Scientist, Tech Innovations Inc.). “Converting a list to a dictionary in Python can be efficiently achieved using dictionary comprehensions. This method not only enhances readability but also improves performance, especially with large datasets.”
Michael Chen (Python Developer, CodeCraft Solutions). “Utilizing the built-in `zip()` function alongside list comprehensions allows for a straightforward and elegant conversion from lists to dictionaries. This approach is particularly useful when you have two related lists, one for keys and another for values.”
Sarah Patel (Software Engineer, DevTech Labs). “When converting lists to dictionaries, it is crucial to handle cases where duplicate keys may arise. Employing the `collections.defaultdict` can help manage such situations effectively, ensuring that all values are captured without data loss.”
Frequently Asked Questions (FAQs)
How can I convert a list of tuples into a dictionary in Python?
You can use the `dict()` constructor along with a list of tuples. For example: `my_dict = dict(my_list_of_tuples)` where `my_list_of_tuples` contains pairs of key-value tuples.
What method can I use to convert two lists into a dictionary?
You can use the `zip()` function to pair elements from two lists and then convert them into a dictionary using `dict()`. For instance: `my_dict = dict(zip(list1, list2))`.
Is there a way to convert a list of lists into a dictionary in Python?
Yes, you can use a dictionary comprehension. For example: `my_dict = {item[0]: item[1] for item in my_list_of_lists}` where each sub-list contains a key-value pair.
Can I convert a list of keys into a dictionary with default values?
Yes, you can use a dictionary comprehension to assign a default value to each key. For example: `my_dict = {key: default_value for key in my_list_of_keys}`.
What is the difference between using `dict()` and dictionary comprehension for conversion?
Using `dict()` is straightforward for converting pairs, while dictionary comprehension offers more flexibility, allowing for conditions and transformations during the conversion process.
How do I handle duplicate keys when converting a list to a dictionary?
When using `dict()`, the last occurrence of a duplicate key will overwrite previous ones. To handle duplicates, consider using `collections.defaultdict` or storing values in lists.
In Python, converting a list to a dictionary can be achieved through various methods, depending on the structure of the list and the desired outcome. The most common scenarios involve using lists of tuples or lists of keys and values. For example, if you have a list of tuples where each tuple contains a key-value pair, the built-in `dict()` function can be employed to create a dictionary directly from this list.
Another approach involves using list comprehensions or the `zip()` function when you have two separate lists: one for keys and another for values. By pairing these lists together, you can efficiently construct a dictionary. This method not only enhances code readability but also allows for more flexible data manipulation.
It is also important to consider scenarios where duplicate keys may exist in the list. In such cases, the last occurrence of a key will overwrite previous entries when creating the dictionary. Therefore, understanding the implications of key uniqueness is crucial when converting lists to dictionaries in Python.
Overall, the ability to convert lists to dictionaries is a fundamental skill in Python programming, enabling developers to leverage the strengths of both data structures. Mastering these techniques can significantly enhance data organization and retrieval in various applications.
Author Profile
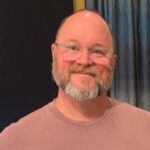
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?