How Can You Concatenate Strings and Integers in Python Effectively?
In the world of programming, the ability to manipulate data types is crucial, and Python makes this task both intuitive and powerful. One common challenge that many beginners encounter is how to effectively concatenate strings and integers. Whether you’re crafting dynamic messages, building user interfaces, or simply displaying results, knowing how to seamlessly combine these different data types can enhance your coding experience and improve the readability of your output. This article will explore the various methods and best practices for concatenating strings and integers in Python, equipping you with the skills to handle this essential task with confidence.
At its core, concatenation is the process of joining two or more pieces of data together, and in Python, this often involves strings and integers. While strings are sequences of characters, integers represent whole numbers, and the interplay between these types can lead to some confusion for those new to the language. Understanding how Python treats these data types and the nuances of converting between them is key to mastering string concatenation.
In the following sections, we will delve into the different approaches available for combining strings and integers, including type conversion techniques and built-in functions. By the end of this article, you’ll not only grasp the mechanics of concatenation but also appreciate the flexibility and simplicity that Python offers in handling diverse data types. Get ready
Understanding String and Integer Types
In Python, data types are crucial for ensuring that operations are performed correctly. Strings are sequences of characters enclosed in quotes, while integers are whole numbers without any decimal points. When you attempt to concatenate these two types, Python raises a `TypeError` because it cannot directly combine a string with an integer.
To effectively concatenate strings and integers in Python, one must convert the integer into a string type. This can be achieved using various methods, as outlined below.
Methods to Concatenate Strings and Integers
There are several methods to concatenate strings and integers in Python. Each method has its own use cases and advantages. Here are the most common methods:
- Using the `str()` function: This function converts an integer into a string.
“`python
number = 42
result = “The answer is ” + str(number)
“`
- Using f-strings (formatted string literals): Available in Python 3.6 and later, f-strings provide a concise way to include variables in strings.
“`python
number = 42
result = f”The answer is {number}”
“`
- Using the `format()` method: This method formats specified values and can be used to concatenate strings and integers.
“`python
number = 42
result = “The answer is {}”.format(number)
“`
- Using the `%` operator: This is an older method that allows for string formatting.
“`python
number = 42
result = “The answer is %d” % number
“`
Comparison of Methods
The choice of method may depend on the specific needs of your application, such as readability or compatibility with older versions of Python. Below is a comparison table of the methods discussed:
Method | Readability | Python Version | Example |
---|---|---|---|
str() | Moderate | All | “The answer is ” + str(number) |
f-strings | High | 3.6+ | f”The answer is {number}” |
format() | Moderate | 2.7, 3+ | “The answer is {}”.format(number) |
% operator | Low | All | “The answer is %d” % number |
Best Practices
When concatenating strings and integers, consider the following best practices:
- Prefer f-strings when working with Python 3.6 or newer due to their readability and efficiency.
- Use the `str()` function for straightforward conversions when backward compatibility is a concern.
- Avoid using the `%` operator for new code, as it is considered less readable compared to newer methods.
By understanding and applying these methods, you can effectively concatenate strings and integers in Python, enhancing the clarity and functionality of your code.
Concatenating Strings and Integers in Python
In Python, concatenating strings with integers requires careful handling to avoid type errors. Strings and integers are fundamentally different types, and direct concatenation will lead to a `TypeError`. Below are methods to effectively concatenate these types.
Using the `str()` Function
One of the simplest ways to concatenate strings and integers is by converting the integer to a string using the `str()` function. This allows you to combine them seamlessly.
“`python
integer_value = 42
string_value = “The answer is: ”
result = string_value + str(integer_value)
print(result) Output: The answer is: 42
“`
Formatted Strings (f-Strings)
Python 3.6 and later versions support formatted string literals, commonly referred to as f-strings. This method allows for elegant and readable formatting.
“`python
integer_value = 42
result = f”The answer is: {integer_value}”
print(result) Output: The answer is: 42
“`
Using the `format()` Method
The `format()` method provides another versatile option for concatenating strings and integers. It allows you to define placeholders in the string.
“`python
integer_value = 42
result = “The answer is: {}”.format(integer_value)
print(result) Output: The answer is: 42
“`
Using String Interpolation with `%` Operator
Older versions of Python also support string interpolation using the `%` operator. This method, while less common today, is still valid.
“`python
integer_value = 42
result = “The answer is: %d” % integer_value
print(result) Output: The answer is: 42
“`
Concatenating Multiple Strings and Integers
When concatenating multiple strings and integers, you can use any of the methods mentioned above. Here’s an example using f-strings:
“`python
name = “Alice”
age = 30
result = f”{name} is {age} years old.”
print(result) Output: Alice is 30 years old.
“`
For the `format()` method, the syntax would look like this:
“`python
name = “Alice”
age = 30
result = “{} is {} years old.”.format(name, age)
print(result) Output: Alice is 30 years old.
“`
Table of Concatenation Methods
Method | Syntax Example | Python Version |
---|---|---|
`str()` | `string + str(integer)` | All versions |
f-Strings | `f”{variable}”` | 3.6 and later |
`format()` | `”{}”.format(variable)` | 2.7 and later |
`%` Operator | `”%d” % variable` | All versions |
These methods provide you with flexibility in how you handle string and integer concatenation in Python, catering to different coding styles and version compatibilities.
Expert Insights on Concatenating Strings and Integers in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “When concatenating strings and integers in Python, it is essential to convert the integer to a string using the `str()` function. This ensures that you avoid type errors and achieve the desired output seamlessly.”
Michael Johnson (Python Developer, CodeCraft Solutions). “One effective approach to concatenate strings and integers is using f-strings, available in Python 3.6 and later. This method not only enhances readability but also improves performance by directly embedding expressions within string literals.”
Susan Lee (Data Scientist, Analytics Hub). “In data manipulation tasks, concatenating strings and integers can often lead to confusion. I recommend always being explicit about type conversions, as it helps maintain code clarity and prevents unexpected behavior in larger projects.”
Frequently Asked Questions (FAQs)
How can I concatenate a string and an integer in Python?
To concatenate a string and an integer in Python, you must first convert the integer to a string using the `str()` function. For example, `result = “The number is ” + str(5)` will yield `”The number is 5″`.
What error occurs if I try to concatenate a string and an integer directly?
If you attempt to concatenate a string and an integer directly, Python will raise a `TypeError`. This error indicates that the operation is unsupported between the two different data types.
Can I use formatted strings to concatenate strings and integers in Python?
Yes, you can use formatted strings (f-strings) to concatenate strings and integers. For example, `number = 5; result = f”The number is {number}”` will produce `”The number is 5″`.
Is there an alternative method to concatenate strings and integers besides using `str()`?
Yes, you can use the `format()` method or f-strings as alternatives. For instance, `result = “The number is {}”.format(5)` or `result = f”The number is {5}”` both achieve the same result.
What is the difference between using `+` and `join()` for concatenation?
Using `+` concatenates individual strings and requires type conversion for integers, while `join()` is typically used to concatenate a list of strings. For example, `”.join([“The number is “, str(5)])` is another way to achieve the same result.
Are there performance differences between using `+` and `join()` for string concatenation?
Yes, using `join()` is generally more efficient for concatenating multiple strings, especially in loops, as it minimizes the creation of intermediate string objects. Using `+` repeatedly can lead to higher memory usage and slower performance.
In Python, concatenating strings and integers requires an understanding of data types and the appropriate methods to combine them effectively. Since strings and integers are different types, attempting to concatenate them directly will result in a TypeError. Therefore, it is essential to convert integers to strings before concatenation. This can be achieved using the built-in `str()` function, which ensures that the integer is properly formatted as a string for seamless integration.
Another method to concatenate strings and integers is by utilizing formatted strings, such as f-strings introduced in Python 3.6. This approach allows for more readable and concise code, as it enables the embedding of expressions directly within string literals. Additionally, the `format()` method and the older `%` operator can also be employed for string formatting, providing flexibility in how strings and integers are combined.
In summary, understanding the data types and conversion methods is crucial for successfully concatenating strings and integers in Python. By employing techniques such as type conversion, formatted strings, and string formatting methods, developers can effectively manage and manipulate different data types in their applications. Mastering these techniques enhances code readability and functionality, ultimately leading to more efficient programming practices.
Author Profile
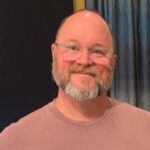
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?