How Can You Effectively Compile a Python Program for Optimal Performance?
How to Compile a Python Program: A Beginner’s Guide
In the world of programming, Python stands out as one of the most accessible and versatile languages available today. With its clean syntax and dynamic typing, it has become a favorite among beginners and seasoned developers alike. However, as you delve deeper into the realm of Python, you might find yourself wondering how to compile a Python program effectively. While Python is an interpreted language, understanding the compilation process can unlock new levels of performance and efficiency for your applications.
Compiling a Python program involves transforming your high-level code into a format that can be executed by the computer. This process not only optimizes the execution time but also allows for the creation of standalone applications that can run independently of the Python interpreter. As you explore the methods and tools available for compiling Python code, you’ll discover the benefits of creating bytecode and the various options for packaging your scripts into executable files.
Whether you’re looking to improve the performance of your Python applications or simply want to share your work with others in a more user-friendly format, mastering the compilation process is a valuable skill. In the following sections, we will delve into the intricacies of compiling Python programs, exploring the tools and techniques that can help you streamline your development workflow and enhance your programming
Understanding the Compilation Process
Compiling a Python program involves converting the human-readable Python code into bytecode, which is a low-level representation of the code that can be executed by the Python virtual machine. This process is primarily handled by the Python interpreter automatically when a script is run. However, understanding this process can be beneficial for optimizing performance and managing dependencies.
Using the Command Line to Compile
To compile a Python program from the command line, you can utilize the built-in `py_compile` module or the `compileall` module. These modules allow you to compile Python files into bytecode files with a `.pyc` extension.
Compiling a single file:
To compile a single Python file, you can use the following command:
“`bash
python -m py_compile your_script.py
“`
This command will generate a `.pyc` file located in the `__pycache__` directory.
Compiling all files in a directory:
If you want to compile all Python files in a directory, use the `compileall` module:
“`bash
python -m compileall your_directory/
“`
This will traverse the directory and compile each `.py` file, creating corresponding `.pyc` files.
Compiling with Optimizations
Python also allows for optimized compilation, which can improve the performance of the generated bytecode. To compile with optimizations, use the `-O` flag:
“`bash
python -O -m py_compile your_script.py
“`
This creates an optimized bytecode file with a `.pyo` extension (in Python 2.x) or a `.pyc` file with optimizations in Python 3.x.
Using IDEs for Compilation
Many Integrated Development Environments (IDEs) provide features for compiling Python code directly. For example:
- PyCharm: Automatically compiles Python files when run.
- VS Code: Supports extensions that enable compilation commands.
- Eclipse with PyDev: Offers a built-in option to compile Python scripts.
These environments simplify the process, allowing developers to focus on writing code rather than managing compilation manually.
Comparison of Compilation Methods
The following table summarizes the different methods of compiling Python code:
Method | Command | Output |
---|---|---|
Single file compilation | python -m py_compile your_script.py | Bytecode (.pyc) |
Directory compilation | python -m compileall your_directory/ | Bytecode for all .py files |
Optimized compilation | python -O -m py_compile your_script.py | Optimized bytecode (.pyc) |
Each method has its use cases depending on the development and production needs. Understanding these options allows developers to choose the best approach for their specific scenario.
Understanding Python Compilation
Python is an interpreted language, which means that Python code is executed line by line. However, there are scenarios where you may want to compile Python code into bytecode or an executable format for performance or distribution purposes.
Compiling to Bytecode
When a Python program is run, the Python interpreter converts the source code (.py files) into bytecode (.pyc files). This process is automatic and happens under the hood, but you can also do it manually.
To compile a Python program to bytecode, use the following command:
“`bash
python -m py_compile your_script.py
“`
This command generates a `.pyc` file located in the `__pycache__` directory. The bytecode can be executed by the Python interpreter, which can lead to slightly faster startup times.
Creating an Executable
To distribute a Python application, you might want to create a standalone executable file. This can be achieved using several tools:
- PyInstaller
- cx_Freeze
- py2exe
These tools package your Python application along with its dependencies into a single executable file.
Using PyInstaller
PyInstaller is one of the most popular tools for creating executables. Follow these steps to compile your Python program using PyInstaller:
- Install PyInstaller:
“`bash
pip install pyinstaller
“`
- Navigate to the directory containing your script.
- Use the following command to create an executable:
“`bash
pyinstaller –onefile your_script.py
“`
- The executable will be found in the `dist` directory.
Configuration Options for PyInstaller
PyInstaller provides various options to customize the build process. Here’s a summary of some useful command-line options:
Option | Description |
---|---|
`–onefile` | Create a single executable file. |
`–noconsole` | Suppress the console window for GUI applications. |
`–icon=icon.ico` | Specify an icon for the executable. |
`–add-data` | Include additional files or data in the build. |
Compiling with cx_Freeze
cx_Freeze is another tool that can be used for creating executables. Here’s how to use it:
- Install cx_Freeze:
“`bash
pip install cx_Freeze
“`
- Create a setup script (`setup.py`):
“`python
from cx_Freeze import setup, Executable
setup(
name=”YourAppName”,
version=”0.1″,
description=”Description of your application”,
executables=[Executable(“your_script.py”)]
)
“`
- Build the executable:
“`bash
python setup.py build
“`
The executable will be placed in the `build` directory.
Best Practices for Compiling Python Programs
- Always test the executable on multiple environments to ensure compatibility.
- Include all necessary dependencies and files to avoid runtime errors.
- Utilize virtual environments to manage dependencies effectively.
- Maintain clear documentation on how to run the executable.
By following these guidelines and using the appropriate tools, you can effectively compile Python programs into bytecode or standalone executables, enhancing performance and making distribution easier.
Expert Insights on Compiling Python Programs
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Compiling a Python program is often misunderstood; many believe Python is purely an interpreted language. However, tools like Cython and PyInstaller allow developers to compile Python code into executable binaries, enhancing performance and distribution.”
Michael Chen (Lead Python Developer, CodeCraft Solutions). “When compiling Python programs, it is crucial to consider the target platform. Using a cross-compilation approach can streamline the process, enabling developers to create executables for different operating systems from a single codebase.”
Sophia Patel (Technical Writer, Python Programming Journal). “Documentation is key when compiling Python programs. Clear instructions on dependencies and environment setup can significantly reduce errors during the compilation process, making it easier for both novice and experienced developers.”
Frequently Asked Questions (FAQs)
What does it mean to compile a Python program?
Compiling a Python program refers to the process of converting Python code (source code) into bytecode, which is a lower-level, platform-independent representation. This bytecode can then be executed by the Python interpreter.
How do I compile a Python program from the command line?
To compile a Python program from the command line, use the `py_compile` module. For example, run `python -m py_compile your_script.py`. This will generate a `.pyc` file in the `__pycache__` directory.
Can I compile Python code into an executable file?
Yes, you can compile Python code into an executable file using tools like PyInstaller or cx_Freeze. These tools package your Python program along with the Python interpreter, allowing it to run independently on systems without Python installed.
What are the benefits of compiling a Python program?
Compiling a Python program can improve performance by reducing load times and optimizing execution speed. It also provides a level of code obfuscation, making it harder for others to read the source code.
Is it necessary to compile Python programs for deployment?
It is not strictly necessary to compile Python programs for deployment, as Python is an interpreted language. However, compiling can enhance performance and simplify distribution, especially for larger applications.
Are there any limitations to compiling Python programs?
Yes, compiled Python programs may not support dynamic features such as runtime code generation or reflection. Additionally, compiled bytecode is still dependent on the Python interpreter, which must be present on the target system.
In summary, compiling a Python program involves transforming the source code written in Python into a format that can be executed by the computer. While Python is primarily an interpreted language, there are methods to compile Python code into bytecode or executable files. This process can enhance performance, facilitate distribution, and protect source code to some extent. Understanding the different compilation methods available, such as using tools like PyInstaller, cx_Freeze, or the built-in `py_compile` module, is essential for developers looking to optimize their applications.
Key takeaways include the importance of choosing the right compilation method based on the specific needs of the project. For instance, if the goal is to create a standalone executable, tools like PyInstaller are particularly useful. On the other hand, for simple bytecode compilation, the `py_compile` module suffices. Additionally, developers should be aware of the potential trade-offs between performance and ease of use, as well as the implications of code obfuscation when compiling Python programs.
Overall, mastering the compilation of Python programs not only enhances a developer’s skill set but also contributes to the efficiency and security of their applications. As Python continues to evolve, staying informed about the latest tools and techniques for compilation will be crucial
Author Profile
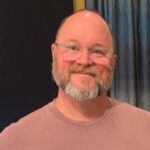
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?