How Can You Effectively Compare Two Strings in Python?
In the world of programming, strings are one of the most fundamental data types, representing sequences of characters that can be manipulated in countless ways. Whether you’re developing a simple application or working on complex algorithms, the ability to compare strings is a crucial skill that every Python programmer should master. From checking for equality to determining which string is lexicographically greater, understanding how to effectively compare strings can significantly enhance your coding efficiency and accuracy.
Python offers a variety of methods and operators that make string comparison not only straightforward but also intuitive. At its core, comparing two strings involves analyzing their content and determining their relationship to one another—whether they are identical, similar, or entirely different. This comparison can be performed using built-in functions, relational operators, and even custom logic, allowing for flexibility in how you approach string comparison tasks.
As we dive deeper into the nuances of string comparison in Python, we will explore the various techniques available, including case sensitivity, substring checks, and the importance of encoding. Whether you’re a novice programmer or an experienced developer looking to refine your skills, this guide will equip you with the knowledge you need to effectively compare strings and leverage this functionality in your projects.
String Comparison Operators
Python provides several built-in operators to compare strings. The most common comparison operators are:
– **Equality (`==`)**: Checks if two strings are exactly equal.
– **Inequality (`!=`)**: Checks if two strings are not equal.
– **Greater than (`>`)**: Determines if one string is lexicographically greater than another.
- Less than (`<`): Determines if one string is lexicographically less than another.
– **Greater than or equal to (`>=`)**: Checks if one string is greater than or equal to another.
- Less than or equal to (`<=`): Checks if one string is less than or equal to another.
When comparing strings, Python uses lexicographical order, which is similar to dictionary order. This means that strings are compared based on the Unicode values of their characters.
Using the `str` Methods for Comparison
In addition to operators, Python’s `str` class provides methods that can be used for string comparison, enhancing flexibility and control. Key methods include:
- `str.lower()`: Converts a string to lowercase, allowing for case-insensitive comparisons.
- `str.upper()`: Converts a string to uppercase for similar reasons.
- `str.strip()`: Removes leading and trailing whitespace, which can be crucial for accurate comparisons.
Here’s an example of using these methods for comparison:
“`python
str1 = ” Hello ”
str2 = “hello”
Case-insensitive comparison
if str1.strip().lower() == str2.lower():
print(“The strings are equal (case insensitive).”)
“`
Comparing Strings in Lists
When you need to compare strings within a list or between two lists of strings, Python provides robust tools. You can use loops or comprehensions to evaluate conditions across multiple strings. Consider the following example:
“`python
list1 = [“apple”, “banana”, “cherry”]
list2 = [“banana”, “cherry”, “date”]
Finding common strings
common_strings = [s for s in list1 if s in list2]
print(“Common strings:”, common_strings)
“`
This approach is efficient for checking membership and can be adapted to various conditions.
Performance Considerations
When comparing strings, especially large strings or numerous comparisons, performance can become a concern. The complexity of string comparisons is O(n), where n is the length of the strings. Here are some considerations:
- Short-circuit Evaluation: Python uses short-circuit evaluation in boolean comparisons, meaning it stops evaluating as soon as the result is determined.
- Immutable Strings: Strings in Python are immutable, which can affect how memory is handled during comparisons.
Comparison Type | Operator/Method | Description |
---|---|---|
Equality | == | Checks if two strings are identical. |
Inequality | != | Checks if two strings are not identical. |
Lexicographic Comparison | > | Compares strings based on Unicode values. |
Case-Insensitive | str.lower()/str.upper() | Compares strings without case sensitivity. |
By leveraging these operators and methods, you can effectively compare strings in various contexts, ensuring that your string handling is both efficient and accurate.
String Comparison Operators
In Python, string comparison can be performed using various operators that evaluate the relationship between two strings. The primary operators include:
– **Equality (`==`)**: Checks if two strings have the same value.
– **Inequality (`!=`)**: Checks if two strings have different values.
– **Greater than (`>`)**: Evaluates if one string is greater than another based on lexicographical order.
- Less than (`<`): Evaluates if one string is lesser than another based on lexicographical order.
– **Greater than or equal to (`>=`)**: Checks if one string is greater than or equal to another.
- Less than or equal to (`<=`): Checks if one string is less than or equal to another.
These operators are case-sensitive and will consider the ASCII values of characters when comparing.
Using the `str` Methods
Python provides several built-in string methods that assist in comparing strings effectively. Some of the commonly used methods are:
- `str.lower()`: Converts all characters in the string to lowercase, allowing for case-insensitive comparison.
- `str.upper()`: Converts all characters in the string to uppercase.
- `str.strip()`: Removes any leading and trailing whitespace, which can affect comparison.
Example usage:
“`python
string1 = “Hello”
string2 = “hello”
Case-sensitive comparison
print(string1 == string2) Output:
Case-insensitive comparison
print(string1.lower() == string2.lower()) Output: True
“`
Comparing Strings for Substring Presence
To check if one string is a substring of another, the `in` keyword is utilized. This operator returns a boolean value indicating the presence of a substring.
Example:
“`python
main_string = “Python programming”
substring = “Python”
Check for substring presence
if substring in main_string:
print(f”‘{substring}’ is found in ‘{main_string}’.”)
else:
print(f”‘{substring}’ is not found in ‘{main_string}’.”)
“`
Utilizing the `locale` Module for Locale-Aware Comparison
When comparing strings that may include locale-specific characters, the `locale` module can be employed for accurate comparisons. This is particularly useful in internationalization scenarios.
Example:
“`python
import locale
locale.setlocale(locale.LC_ALL, ‘en_US.UTF-8’)
string1 = “café”
string2 = “cafe”
Compare using locale-aware comparison
if locale.strcoll(string1, string2) == 0:
print(“Both strings are considered equal in the specified locale.”)
else:
print(“Strings are not equal in the specified locale.”)
“`
Summary of Comparison Techniques
The following table summarizes the methods and techniques discussed for comparing strings:
Technique | Description | Example Usage |
---|---|---|
Equality Operator (`==`) | Checks for equality between two strings | `string1 == string2` |
Inequality Operator (`!=`) | Checks for inequality between two strings | `string1 != string2` |
Lexicographical Comparison | Uses `<`, `>`, `<=`, `>=` for ordering | `string1 < string2` |
Case-Insensitive Comparison | Compares strings without case sensitivity | `string1.lower() == string2.lower()` |
Substring Check | Checks if a string contains another string | `substring in main_string` |
Locale-Aware Comparison | Compares strings considering locale specifics | `locale.strcoll(string1, string2)` |
This overview provides a comprehensive understanding of string comparison methodologies in Python, catering to various scenarios and requirements.
Expert Insights on Comparing Strings in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “When comparing two strings in Python, it is essential to consider the method of comparison you choose. The most straightforward approach is using the equality operator ‘==’, which checks for exact matches. However, for case-insensitive comparisons, utilizing the .lower() or .upper() methods can be beneficial to ensure consistency in your comparisons.”
Michael Chen (Data Scientist, AI Solutions Group). “In scenarios where performance is critical, especially with large datasets, I recommend using the ‘difflib’ library. This library provides tools to compare sequences, including strings, and can help identify similarities and differences efficiently, which is invaluable for data analysis tasks.”
Sarah Patel (Python Developer, CodeCraft Labs). “For more complex string comparisons, such as when dealing with variations in whitespace or punctuation, employing regular expressions through the ‘re’ module can be extremely useful. This allows for flexible matching patterns that can accommodate a wider range of string formats, enhancing the robustness of your comparison logic.”
Frequently Asked Questions (FAQs)
How can I compare two strings for equality in Python?
You can compare two strings for equality using the `==` operator. For example, `string1 == string2` will return `True` if both strings are identical and “ otherwise.
What is the difference between case-sensitive and case-insensitive string comparison?
Case-sensitive comparison considers uppercase and lowercase letters as different characters, while case-insensitive comparison treats them as equivalent. Use `string1.lower() == string2.lower()` for case-insensitive comparison.
How do I check if one string contains another string in Python?
You can use the `in` keyword to check if one string is a substring of another. For example, `substring in string` returns `True` if `substring` is found within `string`.
Can I compare strings using the `is` operator in Python?
The `is` operator checks for object identity, not value equality. It is not recommended for string comparison. Use `==` for comparing string values instead.
What methods can I use for lexicographical string comparison?
You can use relational operators like `<`, `>`, `<=`, and `>=` to perform lexicographical comparisons. For example, `string1 < string2` checks if `string1` comes before `string2` in dictionary order.
How can I compare strings while ignoring whitespace differences?
To compare strings while ignoring leading and trailing whitespace, use the `strip()` method. For example, `string1.strip() == string2.strip()` will compare the two strings after removing any extra spaces.
In Python, comparing two strings can be accomplished through various methods, each serving different needs depending on the context. The most straightforward approach is using the equality operator (`==`), which checks if two strings are identical in both value and type. For case-insensitive comparisons, the `lower()` or `upper()` methods can be employed to standardize the strings before comparison, ensuring that differences in casing do not affect the outcome.
Beyond basic equality checks, Python offers additional functionalities for more complex comparisons. The `str` class provides methods such as `startswith()` and `endswith()`, which allow for checking if one string begins or ends with another substring. Furthermore, the `in` keyword can be utilized to determine if a substring exists within a larger string, making it a powerful tool for substring searches.
For scenarios requiring lexicographical comparisons, Python’s built-in comparison operators (`<`, `>`, `<=`, `>=`) can be employed. These operators evaluate the strings based on their Unicode values, enabling sorting and ordering operations. Additionally, the `difflib` module can be leveraged for a more detailed comparison, providing insights into the differences between two strings, which can be particularly useful for applications like version control or
Author Profile
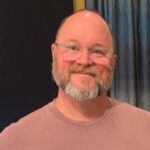
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?