How Can You Effectively Comment Out Lines in Python?
### Introduction
In the world of programming, clarity and organization are paramount, especially when working with complex codebases. One essential skill every Python developer should master is the ability to comment out lines of code effectively. Whether you’re debugging, collaborating with others, or simply trying to make your code more readable, comments serve as invaluable tools for communication and documentation. In this article, we will explore the various methods of commenting in Python, empowering you to enhance your coding practices and streamline your development process.
### Overview
Commenting in Python is straightforward yet powerful, allowing developers to leave notes, explanations, or reminders within their code. By using comments, you can temporarily disable certain lines of code without deleting them, making it easier to test and refine your programs. This practice not only aids in debugging but also helps others (or your future self) understand the thought process behind your code decisions.
Moreover, Python offers different ways to implement comments, catering to various needs and preferences. From single-line comments to multi-line annotations, mastering these techniques will significantly improve your coding efficiency and collaboration with fellow developers. As we delve deeper into the specifics, you’ll discover how to leverage comments to create cleaner, more maintainable code.
Single-line Comments
In Python, single-line comments are initiated by using the hash symbol (`#`). Any text following this symbol on the same line will be considered a comment and will not be executed by the interpreter. This is particularly useful for annotating code to explain its purpose or to temporarily disable certain lines during testing.
Example:
python
# This is a single-line comment
print(“Hello, World!”) # This prints a message
Multi-line Comments
While Python does not have a specific multi-line comment syntax like some other programming languages, you can achieve a similar effect using triple quotes (`”’` or `”””`). Although these are technically multi-line strings, they can be utilized as comments when they are not assigned to any variable.
Example:
python
“””
This is a multi-line comment.
It can span several lines.
“””
print(“Hello, World!”)
Alternatively, you can use multiple single-line comments in succession. This method explicitly indicates that each line is a comment.
Example:
python
# This is the first line of a comment
# This is the second line of a comment
print(“Hello, World!”)
Best Practices for Commenting
Effective commenting improves code readability and maintainability. Here are some best practices to consider:
- Be concise: Aim for clarity without being verbose.
- Use comments to explain why, not what: The code itself should be clear enough to explain what it does; comments should provide context and reasoning.
- Update comments: Always keep comments up-to-date with the code to avoid confusion.
- Avoid obvious comments: Comments should add value; for example, avoid stating that a line of code prints a message.
Commenting Style Guide
Below is a table summarizing various styles and their preferred usage:
Comment Type | Usage |
---|---|
Single-line comments | Use for brief explanations or notes. |
Multi-line comments (triple quotes) | Use when commenting out large blocks of code or providing detailed descriptions. |
Inline comments | Use sparingly to clarify specific lines of code. |
By adhering to these practices and styles, developers can ensure that their code remains understandable and maintainable over time.
Commenting in Python
In Python, comments are essential for documenting code, explaining complex logic, or temporarily disabling code during debugging. They can be created using two primary methods: single-line comments and multi-line comments.
Single-line Comments
Single-line comments in Python begin with the hash symbol (`#`). Everything following the `#` on that line is ignored by the interpreter. This method is straightforward and effective for short explanations or annotations.
Example:
python
# This is a single-line comment
print(“Hello, World!”) # This prints a greeting
Best Practices for Single-line Comments:
- Use them to explain complex operations.
- Keep comments concise and relevant to the surrounding code.
- Avoid stating the obvious; ensure comments add value.
Multi-line Comments
Python does not have a specific syntax for multi-line comments, but developers commonly use triple quotes (`”’` or `”””`) for this purpose. While these are technically string literals, when not assigned to a variable, they serve as comments.
Example:
python
“””
This is a multi-line comment.
It can span multiple lines and is useful for longer explanations.
“””
print(“Hello, World!”)
Considerations for Multi-line Comments:
- Use triple quotes when providing detailed explanations or documentation.
- Ensure that the content is relevant and does not clutter the code.
- Be cautious, as these can be mistakenly interpreted as docstrings if placed at the beginning of functions or classes.
Commenting Best Practices
Effective commenting enhances code maintainability and readability. Below are key best practices to follow:
- Be Clear and Concise: Avoid verbosity; make comments easy to read and understand.
- Update Comments: Regularly revise comments to reflect changes in code logic or functionality.
- Use Comments Sparingly: Rely on descriptive variable and function names as much as possible, only using comments when necessary.
- Avoid Redundancy: Do not restate what the code does; instead, explain why certain decisions were made.
Tools for Commenting
While Python’s built-in commenting features are sufficient for most scenarios, several tools can assist in managing comments effectively:
Tool | Description |
---|---|
Linters | Tools like `pylint` or `flake8` check code style and can enforce comment standards. |
IDE Features | Integrated Development Environments (IDEs) like PyCharm or VSCode offer functionalities to toggle comments quickly. |
Documentation Generators | Tools like Sphinx can convert comments and docstrings into formatted documentation. |
By applying these techniques and best practices, developers can ensure that their Python code remains clear and maintainable, facilitating better collaboration and understanding among team members.
Expert Insights on Commenting Out Lines in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Commenting out lines in Python is a fundamental practice that enhances code readability and maintainability. Utilizing the hash symbol (#) allows developers to temporarily disable code without deletion, which is particularly useful during debugging.”
Michael Chen (Lead Python Developer, CodeCraft Solutions). “For those new to Python, understanding how to comment out lines effectively is crucial. The single-line comment using # is straightforward, but remember that for multi-line comments, triple quotes can also serve this purpose, enhancing clarity when documenting larger code sections.”
Sarah Johnson (Technical Instructor, Python Academy). “Incorporating comments into your Python code is not just about disabling lines; it is about communicating intent. Properly commented code can significantly ease collaboration among team members, making it easier to understand the rationale behind certain coding choices.”
Frequently Asked Questions (FAQs)
How do I comment out a single line in Python?
You can comment out a single line in Python by placing a hash symbol (#) at the beginning of the line. This will prevent the interpreter from executing that line.
Can I comment out multiple lines in Python?
Yes, you can comment out multiple lines by placing a hash symbol (#) at the beginning of each line. Alternatively, you can use triple quotes (”’ or “””) to create a multi-line string that is not assigned to any variable, effectively ignoring those lines.
What is the purpose of commenting in Python?
Commenting in Python serves to explain code functionality, improve readability, and provide context for future reference. It helps other developers (or yourself) understand the logic behind the code.
Are comments ignored by the Python interpreter?
Yes, comments are completely ignored by the Python interpreter during execution. They have no effect on the program’s output or performance.
Can I use comments in Python to temporarily disable code?
Yes, comments are often used to temporarily disable code during debugging or development. By commenting out specific lines, you can prevent them from executing without permanently deleting them.
Is there a difference between single-line and multi-line comments in Python?
In Python, single-line comments are created with a hash symbol (#), while multi-line comments are typically created using triple quotes. However, multi-line comments are technically multi-line strings that are not assigned to a variable.
In Python, commenting out lines of code is an essential practice that enhances code readability and maintainability. Comments are used to explain the purpose of code segments, making it easier for others (or the original author) to understand the logic behind the implementation. Python provides two primary methods for commenting: single-line comments using the hash symbol (#) and multi-line comments using triple quotes (”’ or “””). Understanding how to effectively utilize these commenting techniques is crucial for writing clean and professional code.
One of the key takeaways regarding commenting in Python is that comments should be used judiciously. While they are invaluable for explaining complex logic or providing context, excessive commenting can lead to clutter and confusion. It is important to strike a balance by commenting on non-obvious code while allowing self-explanatory code to stand on its own. Additionally, comments should be kept up to date to reflect any changes made to the code, as outdated comments can mislead future readers.
Another important insight is that comments can also serve as a temporary means to disable code during testing or debugging. By commenting out specific lines, developers can isolate issues without permanently removing code. This practice allows for a more efficient debugging process, as it provides the flexibility to revert changes easily
Author Profile
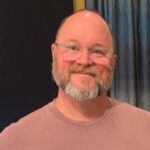
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?