How Can You Effectively Comment Out a Block of Code in Python?
In the world of programming, clarity and organization are paramount. Whether you’re a seasoned developer or just starting your coding journey, understanding how to effectively manage your code is essential. One important skill that every Python programmer should master is the ability to comment out blocks of code. This seemingly simple task can significantly enhance your workflow, making your code easier to read, debug, and maintain. In this article, we will explore the various methods of commenting out code in Python, shedding light on why this practice is crucial for both individual projects and collaborative environments.
Commenting out code serves multiple purposes, from temporarily disabling sections of code during testing to providing clear explanations of complex logic for future reference. By strategically using comments, you can create a roadmap for yourself and others who may work with your code later. This not only aids in debugging but also fosters better communication among team members, ensuring that everyone is on the same page regarding the functionality and intent behind each segment of code.
As we delve deeper into the specifics of commenting out blocks of code in Python, you’ll discover the different techniques available, their advantages, and best practices to follow. Whether you’re looking to streamline your coding process or enhance your collaborative efforts, mastering this skill will undoubtedly elevate your programming proficiency. Let’s embark on this journey
Commenting Techniques in Python
In Python, comments are essential for improving code readability and maintenance. While single-line comments can be created easily using the hash symbol (“), commenting out a block of code requires a different approach. Below are the most effective techniques to comment out multiple lines of code in Python.
Using Triple Quotes
One common method to comment out a block of code is using triple quotes (`”’` or `”””`). Although intended for multi-line strings, Python ignores these strings when they are not assigned to a variable, effectively treating them as comments.
Example:
“`python
”’
This is a block of code that will not be executed.
print(“This will not run.”)
”’
“`
When you use triple quotes, ensure that the block is not assigned to any variable. Otherwise, Python will interpret it as a string variable.
Using Multiple Single-Line Comments
Another straightforward method is to prepend each line with the “ symbol. This is less elegant but can be more readable in some cases.
Example:
“`python
print(“This line is commented out.”)
print(“This line is also commented out.”)
“`
While this method is effective, it can be tedious for long blocks of code.
Commenting Best Practices
When commenting out code, consider the following best practices to maintain clarity:
- Be Consistent: Use the same method throughout your codebase.
- Avoid Excessive Comments: Comment only when necessary to avoid clutter.
- Use Descriptive Comments: If you choose to comment out a block, briefly explain why it is commented out.
Comparison of Commenting Methods
The following table summarizes the advantages and disadvantages of each method for commenting out code blocks in Python:
Method | Advantages | Disadvantages |
---|---|---|
Triple Quotes | Easy to comment large blocks; visually clear | Can be mistaken for a string if not used carefully |
Multiple Single-Line Comments | Simple and straightforward | Can be tedious for long blocks; adds more lines |
By understanding these techniques and best practices, developers can effectively manage code comments in their Python projects, enhancing both collaboration and code quality.
Commenting Out Multiple Lines of Code
In Python, there is no built-in syntax for block comments like in some other programming languages. However, you can effectively comment out multiple lines of code using a couple of methods.
Using the Hash Symbol
The most straightforward method for commenting out multiple lines is by placing a hash symbol (“) at the beginning of each line you want to comment out. This method is simple and clear.
Example:
“`python
This is a comment
This is another comment
print(“This line will not execute.”)
“`
Using Triple Quotes
An alternative approach is to use triple quotes (`”’` or `”””`). While these are primarily intended for multi-line strings and docstrings, they can effectively comment out blocks of code when they are not assigned to a variable.
Example:
“`python
”’
print(“This line will not execute.”)
print(“This line will also not execute.”)
”’
“`
Keep in mind that using triple quotes will create a string object if executed, which can lead to unintended behavior if the code is part of a larger script.
Pros and Cons of Each Method
Method | Pros | Cons |
---|---|---|
Hash Symbol (“) | Clear and explicit, no unintended effects. | Requires adding “ to every line. |
Triple Quotes | Easy to comment/uncomment multiple lines. | Creates a string object, not a comment. |
Best Practices for Commenting Code
Effective commenting practices enhance code readability and maintainability. Here are some best practices to consider:
- Use Comments Sparingly: Only comment on complex logic or sections that may be unclear to others.
- Keep Comments Up to Date: Ensure comments reflect any changes made to the code.
- Explain Why, Not What: Focus on the reasoning behind the code rather than what the code does if the latter is clear from the code itself.
- Avoid Redundant Comments: Comments that simply restate what the code does can clutter your codebase.
By adhering to these practices, you ensure that your comments serve their intended purpose and contribute positively to the overall quality of the code.
Expert Insights on Commenting Out Code in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Commenting out a block of code in Python is essential for debugging and maintaining code clarity. Using the “ symbol for single-line comments is straightforward, but for multi-line comments, developers often use triple quotes. This method allows for quick toggling of code blocks without deleting them, which is invaluable during the development process.”
Michael Chen (Python Developer and Author, Coding Today). “In Python, while there is no built-in syntax for block comments like in some other languages, utilizing triple quotes is a widely accepted practice. This not only serves to comment out code but can also be used for docstrings, enhancing the documentation of functions or classes. Understanding this dual purpose can significantly improve code readability.”
Sarah Johnson (Lead Instructor, Python Programming Bootcamp). “Teaching newcomers to Python, I emphasize the importance of commenting out code effectively. The use of “ for single lines is intuitive, but I encourage students to adopt the triple quotes method for larger sections. This practice not only helps in debugging but also fosters better collaboration among team members by making intentions clear.”
Frequently Asked Questions (FAQs)
How do I comment out a block of code in Python?
To comment out a block of code in Python, you can use triple quotes (either `”’` or `”””`). This method allows you to enclose multiple lines of code, effectively turning them into a multi-line comment.
Can I use the hash symbol () for multi-line comments?
While the hash symbol () is used for single-line comments, you can use it at the beginning of each line to create multi-line comments. However, this is less efficient than using triple quotes for larger blocks of code.
Are there any tools or IDE features that assist with commenting out code?
Many Integrated Development Environments (IDEs) and code editors, such as PyCharm and Visual Studio Code, provide shortcuts to comment or uncomment selected blocks of code quickly. Typically, these shortcuts involve using Ctrl+/ or Command+/ on Mac.
Is there a performance impact when using comments in Python?
Comments in Python do not affect performance during execution as they are ignored by the Python interpreter. However, excessive commenting can make code less readable if not done judiciously.
What is the best practice for commenting code in Python?
Best practices for commenting in Python include writing clear, concise comments that explain the purpose of the code, using comments to clarify complex logic, and avoiding comments that reiterate what the code itself states.
Can I comment out code in Python scripts that are executed in the terminal?
Yes, you can comment out code in Python scripts executed in the terminal using either the hash symbol () for single lines or triple quotes for blocks of code. The comments will be ignored during execution.
In Python, commenting out a block of code is an essential practice for developers, as it allows them to temporarily disable portions of code without deleting them. This can be particularly useful for debugging, testing, or providing explanations within the code. While Python does not have a built-in multi-line comment feature like some other programming languages, developers can achieve similar functionality through the use of triple quotes or by using the hash symbol () for individual lines.
One effective method for commenting out a block of code is to enclose the section within triple quotes (either single or double), which Python interprets as a string literal. This approach is often used for documentation strings (docstrings) but can also serve to comment out code. Alternatively, developers can prepend each line of the code block with the hash symbol, although this method can be more tedious for larger sections of code.
In summary, understanding how to comment out blocks of code in Python is crucial for maintaining clean and manageable code. It enhances collaboration among developers by allowing for clear communication through comments and facilitates easier debugging by enabling the temporary exclusion of code. By mastering these techniques, programmers can improve their coding practices and overall efficiency in software development.
Author Profile
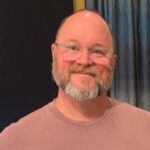
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?