How Can You Effectively Comment a Block of Code in Python?
How To Comment Block Of Code In Python
In the world of programming, clarity and organization are paramount. As developers, we often find ourselves wrestling with complex code, and it’s in these moments that the power of comments becomes evident. Comments serve as the unsung heroes of code, providing context, explanations, and guidance not just for others who may read our work, but also for our future selves. In Python, a language celebrated for its readability and simplicity, knowing how to effectively comment a block of code is an essential skill that enhances both collaboration and maintainability.
Commenting in Python isn’t just a matter of adding a few notes here and there; it’s about creating a narrative that guides the reader through your logic and intentions. Whether you’re working on a small script or a large project, understanding how to comment effectively can make a significant difference in the way your code is perceived and understood. By learning the conventions and best practices for commenting blocks of code, you can foster a more productive coding environment and ensure that your code remains accessible and comprehensible.
In this article, we will delve into the various methods of commenting in Python, focusing specifically on how to create block comments that can encapsulate larger sections of code. We’ll explore the nuances of multi-line comments
Multi-line Comments in Python
In Python, there is no built-in syntax specifically for multi-line comments like other programming languages. However, there are effective techniques that can simulate this functionality. The most common method is to use triple quotes, which are primarily designed for multi-line strings but are frequently utilized for comments.
To create a multi-line comment, you can use either triple single quotes (`”’`) or triple double quotes (`”””`). Here is an example of how to implement this:
“`python
”’
This is a multi-line comment.
It can span multiple lines,
allowing for detailed explanations.
”’
“`
“`python
“””
This is another way to create
a multi-line comment in Python.
“””
“`
While the Python interpreter does not treat these triple quotes as comments, they are ignored when not assigned to a variable or used in expressions, effectively serving the purpose of commenting out blocks of code.
Commenting Out Code Blocks
Sometimes, you may want to temporarily disable a block of code during development or debugging. You can achieve this by using triple quotes around the code block, or you can prepend each line with a hash (“).
Using triple quotes:
“`python
”’
print(“This line will not execute.”)
print(“Neither will this one.”)
”’
“`
Using hash for each line:
“`python
print(“This line will not execute.”)
print(“Neither will this one.”)
“`
The choice of method depends on the context and your personal or team coding style preferences.
Best Practices for Code Comments
Effective commenting enhances the readability and maintainability of your code. Here are some best practices to consider:
- Be Clear and Concise: Write comments that are easy to understand. Avoid overly complex language or jargon.
- Explain the Why: Focus on explaining why a particular approach was taken rather than just describing what the code does.
- Keep Comments Updated: Ensure comments are revised when the code changes to prevent misinformation.
- Use Comments Sparingly: Avoid excessive commenting; aim to write self-explanatory code.
Example Table of Commenting Techniques
Method | Description | Use Case |
---|---|---|
Single-line Comment | Using a hash (“) to comment a single line. | Quick notes or explanations for specific lines of code. |
Multi-line Comment | Using triple quotes to comment multiple lines. | Detailed explanations or temporarily disabling blocks of code. |
Docstrings | Using triple quotes at the beginning of a function or class to describe its purpose. | Documentation for functions and classes. |
By adhering to these practices and techniques, you can effectively manage comments in your Python code, leading to better clarity and teamwork in collaborative environments.
Commenting in Python
In Python, commenting is essential for improving code readability and maintainability. Comments can be added to explain the purpose of a block of code, clarify complex logic, or provide insights into the functionality of the program. Python supports two types of comments: single-line comments and multi-line comments.
Single-Line Comments
Single-line comments are created using the hash symbol (“). Any text following the “ on that line is considered a comment and is ignored by the Python interpreter.
Example:
“`python
This is a single-line comment
print(“Hello, World!”) This prints a greeting
“`
Multi-Line Comments
For longer comments that span multiple lines, Python does not have a specific multi-line comment syntax like some other programming languages. However, you can achieve this by using triple quotes (`”’` or `”””`). While these are technically string literals, they are often used as multi-line comments when not assigned to a variable.
Example:
“`python
”’
This is a multi-line comment.
It can span multiple lines.
”’
print(“Hello, World!”)
“`
Alternatively, you can use multiple single-line comments for clarity.
Example:
“`python
This is a multi-line comment
It uses multiple single-line comments
print(“Hello, World!”)
“`
Best Practices for Commenting Code
To ensure that comments effectively enhance code quality, consider the following best practices:
- Be Clear and Concise: Write comments that are easy to understand and to the point.
- Avoid Redundancy: Do not state the obvious. If a line of code is self-explanatory, it may not need a comment.
- Update Comments: Whenever you modify code, update the corresponding comments to reflect those changes.
- Use Comments for Complex Logic: When the logic is complicated, provide comments to elucidate how it works.
- Document Functions and Classes: Use docstrings (triple quotes placed right under function or class definitions) to describe their purpose, parameters, and return values.
Using Docstrings
Docstrings are a type of comment used to document modules, classes, methods, and functions. They are accessible via the help function and provide an easy way to understand what a particular block of code is intended to do.
Example:
“`python
def add(a, b):
“””
Add two numbers and return the result.
Parameters:
a (int or float): The first number.
b (int or float): The second number.
Returns:
int or float: The sum of a and b.
“””
return a + b
“`
Summary Table of Commenting Techniques
Technique | Syntax | Use Case |
---|---|---|
Single-Line Comment | `comment` | Brief explanations or notes |
Multi-Line Comment | `”’ comment ”’` or `””” comment “””` | Longer explanations or descriptions |
Docstring | `””” docstring “””` | Documenting functions, classes, and modules |
Following these guidelines will enhance the readability of your Python code and make it easier for others (or yourself) to understand and maintain in the future.
Expert Insights on Commenting Code Blocks in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Commenting blocks of code in Python is essential for maintaining readability and facilitating collaboration among developers. Using triple quotes for multi-line comments is a straightforward approach that enhances clarity in complex code sections.”
Michael Johnson (Lead Python Developer, CodeCraft Solutions). “In Python, while there is no official syntax for block comments, leveraging the triple quotes method is widely accepted. This practice not only documents the code effectively but also aids in debugging by allowing developers to temporarily disable sections of code without deleting them.”
Sarah Kim (Technical Writer, Python Programming Journal). “Effective commenting is a hallmark of professional coding. When commenting blocks of code in Python, it is crucial to ensure that the comments are concise yet descriptive. This not only helps in understanding the logic behind the code but also serves as a guide for future modifications.”
Frequently Asked Questions (FAQs)
How do I comment a block of code in Python?
You can comment a block of code in Python by using the “ symbol at the beginning of each line. Alternatively, you can use triple quotes (`”’` or `”””`) to create a multi-line string, which can serve as a comment if not assigned to a variable.
Is there a shortcut to comment multiple lines in Python?
Most code editors and IDEs provide a shortcut to comment multiple lines. For example, in Visual Studio Code, you can select the lines and press `Ctrl + /` (Windows) or `Cmd + /` (Mac) to toggle comments.
Can I use multi-line strings as comments in Python?
Yes, multi-line strings can be used as comments in Python. However, they are technically string literals and not true comments. They will not execute if not assigned to a variable, but they can still be included in the code for documentation purposes.
What happens if I use a comment inside a function?
Comments inside a function will not affect the execution of the code. They serve only as notes for developers and will be ignored by the Python interpreter.
Are there any best practices for commenting code in Python?
Best practices include writing clear and concise comments, avoiding obvious comments, and using comments to explain complex logic or decisions. Additionally, maintain consistency in comment style throughout the codebase.
Can I disable comments in Python?
No, comments cannot be disabled in Python as they are ignored by the interpreter. However, you can remove or modify comments as needed to keep the code clear and maintainable.
In Python, commenting a block of code is essential for enhancing code readability and maintainability. Unlike some programming languages that offer multi-line comment syntax, Python primarily uses the hash symbol () for single-line comments. However, for multi-line comments, developers often utilize triple quotes (”’ or “””) to create block comments. This approach allows for the inclusion of extensive explanations or notes without affecting the execution of the code.
It is important to note that while triple quotes are technically used for multi-line strings, they serve the dual purpose of commenting out code. This flexibility can be advantageous when temporarily disabling sections of code during debugging or development. Nevertheless, developers should be mindful of the context in which they use triple quotes, ensuring that they do not inadvertently introduce string literals into their code.
In summary, effectively commenting code in Python is a best practice that aids in collaboration and future code maintenance. By employing the appropriate commenting techniques, such as using the hash symbol for single lines and triple quotes for blocks, developers can create clearer and more understandable codebases. Ultimately, a well-commented code not only benefits the original author but also assists others who may work with the code in the future.
Author Profile
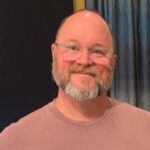
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?