How Can You Resolve an Unclosed String Literal Error in Your Code?
### Introduction
In the world of programming, few things are as frustrating as encountering an unclosed string literal error. This seemingly innocuous mistake can halt your code execution and leave you scratching your head, wondering where it all went wrong. Whether you’re a seasoned developer or a novice coder, understanding how to effectively resolve this issue is crucial for maintaining the flow of your work and ensuring your projects run smoothly. In this article, we’ll delve into the intricacies of string literals, explore common pitfalls that lead to this error, and equip you with the knowledge to tackle it head-on.
String literals are a fundamental aspect of programming languages, allowing developers to represent text within their code. However, when these strings are not properly closed with matching quotation marks, they can cause syntax errors that disrupt the entire program. This article will guide you through the typical scenarios that lead to unclosed string literals, shedding light on the importance of syntax accuracy and the role it plays in code functionality.
By understanding the nuances of string handling and the common mistakes that can occur, you’ll be better prepared to identify and fix these errors swiftly. With practical tips and insights, we aim to empower you to write cleaner, more efficient code while avoiding the pitfalls that can lead to frustrating debugging sessions. Get ready to enhance your
Understanding Unclosed String Literals
An unclosed string literal error occurs when a string in your code is not properly terminated. This can lead to syntax errors that prevent your program from running successfully. String literals are sequences of characters enclosed within quotation marks. If these quotes are mismatched or missing, the interpreter cannot determine where the string begins and ends.
Common causes of unclosed string literal errors include:
- Missing the closing quotation mark
- Using different types of quotation marks (e.g., starting with a single quote and ending with a double quote)
- Commenting out parts of the code that inadvertently include string literals
- Incorrectly escaping characters within the string
Identifying the Error
To effectively address an unclosed string literal error, you must first identify its location. Most programming environments provide error messages that indicate the line number where the issue occurs. Pay close attention to the following:
- Review the line number mentioned in the error message.
- Check the preceding lines for any missing or mismatched quotes.
- Inspect the entire block of code to ensure all strings are properly closed.
Steps to Fix Unclosed String Literals
When you encounter an unclosed string literal error, follow these steps to resolve it:
- Locate the error: Use the error message to find the exact line.
- Check quotation marks: Ensure that every opening quote has a corresponding closing quote.
- Correct mismatched quotes: If you used different types of quotes, align them properly.
- Escape characters: If your string contains special characters, ensure they are correctly escaped.
- Run the code: After making corrections, run the code again to verify that the error is resolved.
Common Issues | Resolution |
---|---|
Missing closing quote | Add the missing quote at the end of the string. |
Mismatched quotes | Change the opening or closing quote to match. |
Improper escape of characters | Use the correct escape sequence (e.g., `\”` for double quotes). |
Comments interfering with strings | Ensure comments do not inadvertently include string literals. |
Preventive Measures
To minimize the occurrence of unclosed string literal errors in your code, consider the following best practices:
- Use a code editor with syntax highlighting: This helps visualize strings and identify mismatches.
- Adopt consistent quoting conventions: Stick to either single or double quotes throughout your code.
- Regularly run your code: Frequent testing can help catch errors early.
- Utilize linters: Tools like ESLint can automatically detect unclosed string literals before runtime.
By implementing these strategies, you can effectively reduce the likelihood of encountering unclosed string literal errors and enhance the overall quality of your code.
Understanding Unclosed String Literal Errors
An unclosed string literal error occurs when a string in your code is initiated but not properly terminated. This can lead to syntax errors, causing the code to fail during compilation or execution. Understanding the causes of this error is crucial for effective debugging.
Common causes include:
- Missing closing quotes.
- Using mismatched quotes (e.g., starting with a single quote and ending with a double quote).
- Special characters in strings that are not properly escaped.
Identifying the Error
To effectively address an unclosed string literal error, first identify where it has occurred. Most programming languages provide error messages indicating the line number and the nature of the error.
Steps to locate the error:
- Read the error message: It often specifies the line number and the type of error.
- Examine the code: Look closely at the indicated line and the lines preceding it for any string literals that do not have matching opening and closing quotes.
- Check for special characters: Ensure that any special characters within strings are properly escaped.
Common Programming Languages and Their Solutions
Different programming languages may handle string literals uniquely. Below is a concise table summarizing how to fix unclosed string literal errors in some popular languages:
Language | Fixing Method |
---|---|
Python | Ensure all strings have matching quotes. Use triple quotes for multi-line strings. |
JavaScript | Verify that all quotes match and escape special characters with a backslash. |
Java | Check for unclosed double or single quotes in string literals and ensure proper escape of special characters. |
C# | Look for mismatched quotes or improper concatenation of strings. |
Ruby | Ensure strings are properly enclosed and escaped as needed. |
Best Practices for Avoiding Unclosed String Literals
To minimize the chances of encountering unclosed string literal errors, consider the following best practices:
- Consistent Quoting: Choose single or double quotes and stick to them within a project.
- Use an IDE or Text Editor: Many Integrated Development Environments (IDEs) and text editors highlight string literals and mismatched quotes.
- Linting Tools: Utilize linting tools that analyze your code for potential issues, including unclosed string literals.
- Code Reviews: Implement a code review process where peers can catch errors before code merges.
Example Scenarios
Consider the following examples that illustrate unclosed string literal errors and their corrections:
Example 1: Python
python
# Error
message = “Hello, World # Missing closing quote
# Correction
message = “Hello, World”
Example 2: JavaScript
javascript
// Error
let greeting = ‘Hello, World; // Missing closing quote
// Correction
let greeting = ‘Hello, World’;
Example 3: Java
java
// Error
String str = “Hello, World; // Missing closing quote
// Correction
String str = “Hello, World”;
By following these guidelines and understanding the mechanics behind unclosed string literals, you can effectively troubleshoot and resolve these errors in your code.
Expert Insights on Resolving Unclosed String Literal Errors
Dr. Emily Carter (Senior Software Engineer, CodeGuard Solutions). “Unclosed string literal errors are often the result of overlooked syntax, particularly in languages that require strict adherence to quotation marks. A thorough review of the code, focusing on string declarations, can often reveal the missing closing quote.”
Mark Thompson (Lead Developer, Tech Innovations Inc.). “When encountering an unclosed string literal error, it is crucial to utilize your IDE’s syntax highlighting features. These tools can help identify mismatched quotes quickly, allowing for a more efficient debugging process.”
Linda Chen (Programming Language Expert, DevInsights Journal). “To prevent unclosed string literal errors, developers should adopt a consistent coding style that includes regular code reviews and pair programming. These practices foster better attention to detail and can significantly reduce syntax-related issues.”
Frequently Asked Questions (FAQs)
What is an unclosed string literal error?
An unclosed string literal error occurs when a string in programming code is not properly terminated with a closing quotation mark. This can lead to syntax errors and prevent the code from executing.
How can I identify an unclosed string literal in my code?
To identify an unclosed string literal, check for strings that start with a quotation mark but do not have a corresponding closing quotation mark. Most integrated development environments (IDEs) will highlight the error or provide error messages indicating the line number.
What are common causes of unclosed string literal errors?
Common causes include forgetting to close a string, using mismatched quotation marks, or accidentally commenting out part of a string. Additionally, copying and pasting code can sometimes lead to this error if the quotation marks are omitted.
How do I fix an unclosed string literal error?
To fix an unclosed string literal error, locate the string in question and ensure it has both an opening and a closing quotation mark. Verify that the quotation marks match in type (single or double) and are correctly placed.
Can unclosed string literals affect program performance?
Yes, unclosed string literals can significantly affect program performance by causing syntax errors that prevent the code from compiling or running. This can lead to increased debugging time and hinder development progress.
Are there tools to help detect unclosed string literal errors?
Yes, many code editors and IDEs feature built-in linting tools that automatically detect syntax errors, including unclosed string literals. Additionally, static code analysis tools can be used to identify such issues before runtime.
In programming, an unclosed string literal error occurs when a string is not properly enclosed within quotation marks. This can lead to syntax errors, preventing the code from executing correctly. To resolve this issue, developers must carefully review their code to identify any strings that lack closing quotation marks. It is essential to ensure that every opening quotation mark has a corresponding closing mark, as this maintains the integrity of the code and enables the interpreter or compiler to parse it correctly.
Additionally, developers should utilize code editors or integrated development environments (IDEs) that provide syntax highlighting and error detection features. These tools can help identify unclosed string literals and other syntax errors quickly. Furthermore, implementing best practices such as consistent formatting and code reviews can significantly reduce the occurrence of such errors in the future.
addressing unclosed string literal errors is crucial for maintaining code functionality and readability. By being vigilant about string closures and leveraging available tools, programmers can enhance their coding efficiency and minimize debugging time. Ultimately, fostering a disciplined coding approach will contribute to more robust and error-free software development.
Author Profile
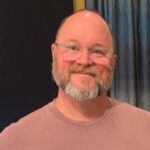
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?