How Can You Effectively Clean a Dataset in Python?
In the world of data science, the quality of your dataset can make or break your analysis. Imagine pouring hours into building complex models, only to realize that your results are skewed by missing values, outliers, or irrelevant features. This is where the art of dataset cleaning comes into play. If you’re looking to elevate your data analysis game, mastering the techniques of cleaning your dataset in Python is not just beneficial—it’s essential.
Cleaning a dataset is a fundamental step in the data preparation process that involves identifying and rectifying inaccuracies or inconsistencies within your data. This process can include tasks such as handling missing values, removing duplicates, and converting data types to ensure that your dataset is not only accurate but also ready for insightful analysis. Python, with its rich ecosystem of libraries like Pandas and NumPy, offers powerful tools that streamline this process, making it easier for data professionals to transform raw data into a polished product.
As we delve deeper into the intricacies of dataset cleaning in Python, you’ll discover a variety of techniques and best practices that can help you tackle common data issues effectively. Whether you are a seasoned data analyst or just starting your journey, understanding how to clean your dataset will empower you to derive meaningful insights and make informed decisions based on your data. Get ready
Identifying Missing Values
Identifying missing values in a dataset is crucial as they can lead to biased results in analyses. In Python, the `pandas` library provides tools to easily detect these missing entries. The `isnull()` method can be utilized to check for null values in a DataFrame.
Example:
“`python
import pandas as pd
Load dataset
df = pd.read_csv(‘data.csv’)
Identify missing values
missing_values = df.isnull().sum()
print(missing_values)
“`
This code snippet will display the count of missing values for each column in the DataFrame. You can visualize missing data using a heatmap with the `seaborn` library, which provides an intuitive understanding of the extent of missing values.
Handling Missing Values
Once missing values have been identified, it’s essential to determine how to address them. Common strategies include:
- Removing rows or columns: This is suitable when a small portion of the data is missing.
- Imputing values: Replace missing values with a statistic like the mean, median, or mode.
- Using algorithms: Some machine learning algorithms can handle missing values internally.
Example of imputation using `SimpleImputer` from the `sklearn` library:
“`python
from sklearn.impute import SimpleImputer
Create an imputer object with a mean filling strategy
imputer = SimpleImputer(strategy=’mean’)
Fit and transform the data
df[[‘column_name’]] = imputer.fit_transform(df[[‘column_name’]])
“`
Removing Duplicates
Duplicate entries can skew the results of data analysis. The `drop_duplicates()` method in `pandas` is effective for this purpose. It removes duplicate rows based on specified columns.
Example:
“`python
Remove duplicates
df_cleaned = df.drop_duplicates()
“`
You can also specify which columns to consider when identifying duplicates:
“`python
Remove duplicates based on specific columns
df_cleaned = df.drop_duplicates(subset=[‘column1’, ‘column2’])
“`
Standardizing Data Formats
Consistency in data formats is vital for accurate analysis. This may involve converting data types, normalizing text (e.g., lowercasing), or formatting dates. The following are common standardization techniques:
- String manipulation: Use `.str` methods to clean text data.
- Date conversion: Convert strings to `datetime` objects using `pd.to_datetime()`.
Example:
“`python
Convert column to lowercase
df[‘text_column’] = df[‘text_column’].str.lower()
Convert a string column to datetime
df[‘date_column’] = pd.to_datetime(df[‘date_column’])
“`
Outlier Detection and Treatment
Outliers can significantly affect statistical analyses and machine learning models. Techniques for detecting outliers include:
- Statistical methods: Using z-scores or IQR (Interquartile Range).
- Visualization: Box plots can visually indicate outliers.
Once identified, outliers can be treated by:
- Removing them: If they are deemed erroneous.
- Transforming data: Using log transformation or winsorization.
Example of IQR method:
“`python
Calculate Q1 and Q3
Q1 = df[‘numerical_column’].quantile(0.25)
Q3 = df[‘numerical_column’].quantile(0.75)
IQR = Q3 – Q1
Identify outliers
outliers = df[(df[‘numerical_column’] < (Q1 - 1.5 * IQR)) | (df['numerical_column'] > (Q3 + 1.5 * IQR))]
“`
Table of Common Data Cleaning Techniques
Technique | Description | Example Code |
---|---|---|
Identifying Missing Values | Check for null entries in the dataset. | df.isnull().sum() |
Imputation | Fill missing values with a statistic. | SimpleImputer(strategy='mean') |
Removing Duplicates | Eliminate duplicate rows. | df.drop_duplicates() |
Standardizing Formats | Ensure consistent data types and formats. | pd.to_datetime() |
Outlier Treatment | Detect and manage outliers. | df[(df['numerical_column'] < (Q1 - 1.5 * IQR)) | (df['numerical_column'] > (Q3 + 1.5 * IQR))] |
Identifying Missing Values
Identifying missing values is a crucial step in cleaning datasets. In Python, libraries such as Pandas provide efficient methods to locate and handle these gaps.
- Use the `.isnull()` method to identify missing values.
- Combine with `.sum()` to count missing values per column:
“`python
import pandas as pd
data = pd.read_csv(‘data.csv’)
missing_values = data.isnull().sum()
print(missing_values)
“`
This will output the count of missing values for each column, enabling you to determine the extent of the issue.
Handling Missing Values
Once missing values are identified, they can be handled in various ways:
- Remove Rows/Columns: If a significant portion of data is missing, consider dropping those rows or columns:
“`python
Drop rows with missing values
data_cleaned = data.dropna()
Drop columns with missing values
data_cleaned = data.dropna(axis=1)
“`
- Imputation: For numerical data, replace missing values with the mean, median, or mode:
“`python
Fill missing numerical values with the mean
data[‘column_name’].fillna(data[‘column_name’].mean(), inplace=True)
“`
- Forward/Backward Fill: For time series data, forward or backward fill can be used to propagate the next or previous value:
“`python
data.fillna(method=’ffill’, inplace=True) Forward fill
data.fillna(method=’bfill’, inplace=True) Backward fill
“`
Removing Duplicates
Duplicates can skew analysis and must be addressed. The Pandas library provides a straightforward method to identify and remove duplicate entries.
- Use the `.duplicated()` method to find duplicates:
“`python
duplicates = data.duplicated()
print(data[duplicates])
“`
- To remove duplicates, utilize the `.drop_duplicates()` method:
“`python
data_cleaned = data.drop_duplicates()
“`
This operation retains the first occurrence of each duplicate and discards the rest.
Standardizing Data Formats
Standardizing formats is essential for consistency. For example, dates and strings should follow a uniform format.
- Date Formatting: Convert date strings to a `datetime` format:
“`python
data[‘date_column’] = pd.to_datetime(data[‘date_column’])
“`
- String Standardization: Ensure string data is consistent in case and whitespace:
“`python
data[‘string_column’] = data[‘string_column’].str.strip().str.lower()
“`
Outlier Detection and Treatment
Outliers can disproportionately affect statistical analyses. Identifying and handling them is critical for accurate results.
- Use statistical methods such as the Z-score or IQR (Interquartile Range) to detect outliers:
“`python
from scipy import stats
z_scores = stats.zscore(data[‘numeric_column’])
outliers = data[(z_scores < -3) | (z_scores > 3)]
“`
- Outliers can be treated by removing them or capping them within a specified range:
“`python
data = data[(data[‘numeric_column’] >= lower_bound) & (data[‘numeric_column’] <= upper_bound)]
```
Normalization and Scaling
Normalizing and scaling features ensure that they contribute equally to distance metrics used in algorithms.
- Min-Max Scaling: Scale features to a range of [0, 1]:
“`python
from sklearn.preprocessing import MinMaxScaler
scaler = MinMaxScaler()
data_scaled = scaler.fit_transform(data[[‘feature1’, ‘feature2’]])
“`
- Standardization: Center the distribution of the data to have a mean of 0 and standard deviation of 1:
“`python
from sklearn.preprocessing import StandardScaler
scaler = StandardScaler()
data_standardized = scaler.fit_transform(data[[‘feature1’, ‘feature2’]])
“`
By following these practices, datasets can be effectively cleaned and prepared for analysis, ensuring reliable and valid results.
Expert Insights on Cleaning Datasets in Python
Dr. Emily Carter (Data Scientist, Tech Innovations Inc.). “Cleaning datasets in Python requires a systematic approach, starting with identifying missing values and outliers. Utilizing libraries such as Pandas and NumPy can significantly streamline the process, allowing for efficient data manipulation and preparation.”
Michael Chen (Machine Learning Engineer, AI Solutions Group). “In my experience, the key to effective dataset cleaning is automation. Implementing functions to handle repetitive tasks, such as removing duplicates or normalizing data formats, can save time and reduce human error during the data preprocessing stage.”
Sarah Patel (Data Analyst, Insight Analytics). “Understanding the context of your data is crucial when cleaning datasets. It is essential to apply domain knowledge to determine which cleaning methods are appropriate, ensuring that the integrity of the data is maintained throughout the process.”
Frequently Asked Questions (FAQs)
How do I handle missing values in a dataset using Python?
To handle missing values in Python, you can use the `pandas` library. Methods include dropping missing values with `dropna()`, filling them with a specific value using `fillna()`, or using interpolation methods.
What libraries are commonly used for data cleaning in Python?
Common libraries for data cleaning in Python include `pandas` for data manipulation, `numpy` for numerical operations, and `scikit-learn` for preprocessing data. Additionally, `regex` can be useful for text cleaning.
How can I remove duplicates from a dataset in Python?
You can remove duplicates in a dataset using the `drop_duplicates()` method in the `pandas` library. This method allows you to specify which columns to consider for identifying duplicates.
What techniques can I use to standardize text data in Python?
To standardize text data, you can convert text to lowercase, remove punctuation, and trim whitespace using string methods in Python. The `str.replace()` and `str.strip()` functions in `pandas` can facilitate this process.
How do I convert data types in a pandas DataFrame?
You can convert data types in a pandas DataFrame using the `astype()` method. This method allows you to specify the desired data type for one or more columns.
What is the role of regular expressions in data cleaning?
Regular expressions (regex) play a crucial role in data cleaning by allowing you to identify and manipulate text patterns. They can be used for tasks such as removing unwanted characters, validating formats, and extracting specific information from strings.
In summary, cleaning a dataset in Python is a crucial step in the data analysis process that ensures the integrity and reliability of the results. The cleaning process typically involves several key tasks, including handling missing values, removing duplicates, correcting inconsistent data entries, and standardizing data formats. Utilizing libraries such as Pandas and NumPy can significantly streamline these tasks, allowing for efficient manipulation and transformation of data.
Moreover, understanding the nature of the dataset and the specific issues it may present is essential for effective cleaning. Techniques such as data imputation for missing values, outlier detection, and normalization of data can enhance the quality of the dataset. Additionally, employing visualization tools can help identify patterns and anomalies that may require attention during the cleaning process.
Ultimately, a well-cleaned dataset not only improves the accuracy of analyses but also facilitates better decision-making based on the insights derived from the data. By following best practices and leveraging Python’s powerful libraries, data professionals can ensure that their datasets are robust and ready for further exploration and modeling.
Author Profile
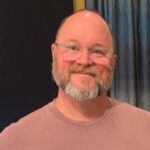
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?