How Can You Easily Check the Package Version in Python?
When working with Python, managing your packages effectively is crucial for ensuring that your projects run smoothly and efficiently. Whether you’re developing a new application or maintaining an existing one, understanding the versions of the packages you depend on can save you from compatibility issues and bugs. But how do you check which versions of packages are installed in your Python environment? This article will guide you through the essential methods and tools available to help you easily check package versions, empowering you to take control of your development environment.
In the world of Python, packages are the building blocks that enable developers to leverage existing code and functionality, making programming more efficient and enjoyable. However, as projects evolve, so do the packages they rely on. Keeping track of the versions of these packages is vital, as different versions can introduce new features, fix bugs, or even lead to breaking changes. Knowing how to check the installed package versions allows you to ensure compatibility and make informed decisions about updates and dependencies.
This article will explore various techniques for checking package versions in Python, from using built-in commands to leveraging popular package management tools. You’ll learn how to quickly retrieve version information, understand the significance of versioning, and discover best practices for maintaining a healthy and up-to-date Python environment. Whether you’re a seasoned developer or just starting out,
Using the Command Line
To check the version of a package installed in your Python environment, you can use the command line interface. This method is straightforward and effective for quickly retrieving the version information of specific packages. Here are the primary commands you can utilize:
- Using pip: The most common tool for managing Python packages is `pip`. You can check the version of a specific package by executing the following command in your terminal or command prompt:
“`
pip show package_name
“`
Replace `package_name` with the actual name of the package. This command will provide detailed information, including the version, location, and dependencies.
- Listing all packages: If you want to see the versions of all installed packages, you can run:
“`
pip list
“`
This command will output a list of all packages along with their versions in a tabular format.
Using Python Code
You can also check the version of a package programmatically within a Python script or an interactive shell. This is particularly useful for confirming package versions when developing applications. Here are some common methods:
- Using `pkg_resources`: The `pkg_resources` module, part of the `setuptools` package, allows you to access package metadata. You can use the following code snippet:
“`python
import pkg_resources
package_name = ‘your_package_name’
version = pkg_resources.get_distribution(package_name).version
print(f”{package_name} version: {version}”)
“`
- Using `importlib.metadata`: For Python 3.8 and later, you can use the `importlib.metadata` module:
“`python
from importlib.metadata import version
package_name = ‘your_package_name’
print(f”{package_name} version: {version(package_name)}”)
“`
Checking Version in a Jupyter Notebook
If you’re working within a Jupyter Notebook, you can easily check the version of a package using the same methods as above, or you can utilize a cell magic command to run shell commands directly:
- Using pip within a cell:
“`python
!pip show package_name
“`
- Using `pkg_resources` or `importlib.metadata` as shown earlier is also applicable within Jupyter cells.
Comparison of Methods
When checking package versions, each method has its own advantages. Below is a comparison table summarizing the approaches:
Method | Pros | Cons |
---|---|---|
Command Line (pip) | Quick and easy; no coding required | Requires terminal access |
pkg_resources | Integrates with Python code; no external tools needed | Requires knowledge of Python programming |
importlib.metadata | Standard library for Python 3.8+; cleaner syntax | Not available in earlier Python versions |
Jupyter Notebook | Interactive and user-friendly; integrates well with data analysis | Requires a running Jupyter environment |
Utilizing these methods will ensure you have the necessary information regarding package versions, aiding in effective package management and development practices.
Using pip to Check Package Version
One of the most straightforward methods to check the installed version of a package in Python is through the `pip` command-line tool. This can be accomplished in several ways:
- Check a specific package version:
Use the following command in your terminal or command prompt:
“`bash
pip show package_name
“`
Replace `package_name` with the name of the package. This command provides detailed information including the version.
- List all installed packages with versions:
To see all installed packages along with their versions, execute:
“`bash
pip list
“`
This will output a list in the format:
“`
Package Version
————— ——-
numpy 1.21.0
requests 2.25.1
“`
Using Python Code to Check Package Version
You can also check the version of a package programmatically using Python code. This is particularly useful for scripts and applications that need to verify package versions.
- Using `pkg_resources`:
The `pkg_resources` module allows you to access package metadata. Here’s how to use it:
“`python
import pkg_resources
version = pkg_resources.get_distribution(“package_name”).version
print(version)
“`
- Using `importlib.metadata`:
In Python 3.8 and later, you can use `importlib.metadata` for a more modern approach:
“`python
from importlib.metadata import version
print(version(“package_name”))
“`
Checking Version with Environment Management Tools
When using environment management tools like `conda`, checking package versions may differ slightly.
- Using conda:
To check the version of a package installed in a conda environment, use:
“`bash
conda list package_name
“`
This displays the version along with other details.
Retrieving Version Information from Within a Package
Many packages have their version information accessible through their own module. This can be particularly useful if you want to confirm the version from within your code.
- Common Pattern:
Many libraries define their version in a `__version__` attribute. Here’s a typical usage:
“`python
import package_name
print(package_name.__version__)
“`
Useful Resources and Tools
For comprehensive package management, consider these tools:
Tool | Description |
---|---|
pip | Python’s package installer and manager. |
conda | An environment manager with package support. |
pipenv | Combines `pip` and `virtualenv` for package management. |
poetry | Dependency management tool for Python. |
Utilizing these methods will ensure that you can effectively manage and verify package versions within your Python environment.
Expert Insights on Checking Package Versions in Python
Dr. Emily Chen (Senior Python Developer, Tech Innovations Inc.). “To check the version of a package in Python, the most straightforward method is to use the command `pip show package_name`. This command provides not only the version but also other metadata about the package, which can be invaluable for debugging and compatibility checks.”
Mark Thompson (Lead Software Engineer, Open Source Solutions). “Utilizing the `pkg_resources` module from the `setuptools` library is another effective way to check package versions programmatically. The command `pkg_resources.get_distribution(‘package_name’).version` allows developers to retrieve the version within their scripts, ensuring that the correct dependencies are loaded during runtime.”
Linda Garcia (Python Instructor, Code Academy). “For those who prefer a more visual approach, using Jupyter Notebook or an interactive Python shell, you can simply import the package and print its `__version__` attribute. This method is particularly useful for quick checks during development and testing phases.”
Frequently Asked Questions (FAQs)
How can I check the version of an installed package in Python?
You can check the version of an installed package by using the command `pip show package_name` in your terminal or command prompt, replacing `package_name` with the actual name of the package.
Is there a way to check package versions directly within a Python script?
Yes, you can check the version of a package within a Python script by importing the package and accessing its `__version__` attribute, for example: `import package_name; print(package_name.__version__)`.
What command can I use to list all installed packages along with their versions?
You can list all installed packages and their versions by executing the command `pip list` in your terminal or command prompt.
Can I check the version of a package using the Python interpreter?
Yes, you can check the version of a package using the Python interpreter by running `import pkg_resources; print(pkg_resources.get_distribution(“package_name”).version)`.
What if the package does not have a `__version__` attribute?
If a package does not have a `__version__` attribute, you can refer to its documentation or use `pip show package_name` to find the version information.
How can I check the version of a package installed in a virtual environment?
To check the version of a package in a virtual environment, activate the virtual environment and then use the command `pip show package_name` or `pip list` as you would in a global environment.
In summary, checking the version of a package in Python is a straightforward process that can be accomplished using several methods. The most common approaches include using the command line interface with pip, importing the package and accessing its `__version__` attribute, or utilizing the `pkg_resources` module from the `setuptools` library. Each of these methods serves to provide developers with the necessary information about the installed packages, ensuring compatibility and aiding in troubleshooting.
Key takeaways from this discussion emphasize the importance of package version management in Python development. Understanding the version of a package can help prevent conflicts that arise from incompatible dependencies. Additionally, being aware of the latest versions can encourage developers to leverage new features and improvements, thereby enhancing the overall functionality of their applications.
Moreover, it is essential for developers to incorporate version checks into their workflows, especially in collaborative environments where multiple contributors might be using different package versions. By adopting best practices for version management, developers can maintain a more stable and reliable codebase, ultimately leading to more efficient project development and deployment.
Author Profile
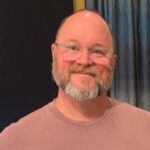
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?