How Can You Effectively Break Out of a While Loop in Programming?
While loops are a fundamental aspect of programming, allowing developers to execute a block of code repeatedly as long as a specified condition remains true. However, there are times when you may need to exit a while loop prematurely—perhaps due to an unexpected condition or a specific user input. Understanding how to break a while loop effectively is essential for writing robust and efficient code. In this article, we’ll explore various techniques and best practices for breaking out of while loops, ensuring your programs can handle dynamic scenarios with ease.
When working with while loops, the ability to break out of the loop at the right moment is crucial for maintaining control over your program’s flow. This can prevent infinite loops, which can lead to unresponsive applications, and allows for more flexible and user-friendly experiences. Whether you’re dealing with user input, error handling, or simply optimizing your code, knowing when and how to implement a break statement can save you time and frustration.
In the following sections, we will delve into the mechanics of breaking while loops, including common scenarios where this might be necessary. We will also discuss the implications of using break statements and alternative approaches that can be employed to manage loop execution effectively. By the end of this article, you’ll have a solid understanding of how to navigate
Using the break Statement
The `break` statement is a powerful tool for terminating a loop prematurely. When executed within a loop, it immediately exits the loop, transferring control to the statement that follows the loop. This is particularly useful when a specific condition is met that warrants stopping the loop’s execution.
Here’s an example of how to use the `break` statement in a while loop:
“`python
count = 0
while True:
if count >= 5:
break
print(count)
count += 1
“`
In this example, the while loop will continue indefinitely until `count` reaches 5, at which point the `break` statement is executed, and the loop exits.
Conditionally Breaking the Loop
To effectively use the `break` statement, it is essential to implement a condition that will dictate when to exit the loop. This can be done by checking a variable or a condition within the loop.
- Define a condition for the loop to run.
- Use the `break` statement when the desired condition is met.
Consider the following scenario:
“`python
number = 0
while number < 10:
number += 1
if number == 5:
break
print(number)
```
In this case, the loop will print numbers from 1 to 4 and stop when `number` equals 5.
Best Practices for Using break
To ensure clarity and maintainability in your code when utilizing the `break` statement, consider the following best practices:
- Use Descriptive Conditions: Make the condition that leads to the `break` clear and descriptive. This enhances code readability.
- Limit Usage: Overusing `break` can make the flow of the program difficult to follow. Use it judiciously.
- Commenting: Provide comments to explain why the loop is being terminated, especially if the condition is complex.
Practice | Description |
---|---|
Descriptive Conditions | Use clear and understandable conditions to enhance readability. |
Limit Usage | Avoid excessive use of break to maintain the structure of the loop. |
Commenting | Add comments for clarity on the termination point of the loop. |
Alternative Methods to Exit a Loop
While the `break` statement is commonly used, there are alternative methods to exit a loop. These include:
- Return Statement: In functions, you can use `return` to exit a loop and the function simultaneously.
- Exception Handling: Raising an exception can also terminate a loop, but this should be done with caution as it may complicate the code flow.
Using these alternatives can be appropriate depending on the context of your code and the specific requirements of your program.
Understanding the While Loop
A while loop is a control flow statement that allows code to be executed repeatedly based on a given Boolean condition. The loop will continue to run until the condition evaluates to .
- Structure of a While Loop:
- Initialization: Set up any necessary variables.
- Condition: The Boolean expression that is evaluated before each iteration.
- Loop Body: The code that executes if the condition is true.
Example:
“`python
while condition:
loop body
“`
Methods to Break a While Loop
There are several techniques to exit a while loop before the condition becomes .
Using the `break` Statement
The `break` statement immediately terminates the loop, regardless of the loop’s condition. This is useful in scenarios where a specific event or condition warrants an early exit.
Example:
“`python
while True:
user_input = input(“Type ‘exit’ to break: “)
if user_input == ‘exit’:
break
“`
Using a Condition Variable
Modify a variable that is part of the loop’s condition so that it eventually evaluates to . This is often used in scenarios where the loop must run until a certain condition is met.
Example:
“`python
count = 0
while count < 10:
print(count)
count += 1
```
Utilizing Exceptions
In some programming languages, throwing an exception can be a method to break out of a loop. This is less common and typically used in complex scenarios.
Example:
“`python
try:
while True:
some code
if some_condition:
raise Exception(“Breaking the loop”)
except Exception as e:
print(e)
“`
Using Flags
Implementing a flag variable can allow you to control the execution of the loop based on external conditions, providing a clear and manageable way to exit.
Example:
“`python
running = True
while running:
some code
if some_condition:
running =
“`
Best Practices When Breaking a While Loop
- Clarity: Ensure that the condition for breaking is easily understandable to avoid confusion.
- Efficiency: Avoid complex conditions that may impact readability and performance.
- Resource Management: Ensure any resources (like file handles or network connections) are properly managed before exiting the loop.
Common Mistakes to Avoid
- Failing to update the loop condition, which can lead to infinite loops.
- Using `break` statements excessively, making code harder to follow.
- Overcomplicating exit conditions, which can cause maintenance challenges.
Mistake | Description | Prevention |
---|---|---|
Infinite Loop | Not updating the condition variable | Ensure variable updates |
Overuse of Break | Making code less readable | Limit `break` usage |
Complex Conditions | Creating hard-to-understand exit criteria | Simplify conditions |
By employing these techniques and adhering to best practices, you can effectively manage and break while loops in your code.
Expert Insights on Breaking While Loops in Programming
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To effectively break a while loop, one must ensure that the loop condition is appropriately defined. It is crucial to include a break statement within the loop that is triggered under specific conditions, allowing for graceful termination of the loop without causing infinite execution.”
Michael Thompson (Lead Developer, CodeCraft Academy). “Utilizing flags or counters within the while loop can provide a clear mechanism for breaking out of the loop. By incrementing a counter or setting a flag based on certain criteria, developers can control the flow of execution and avoid potential performance issues.”
Linda Zhao (Programming Instructor, Future Coders Institute). “It is essential to practice good coding habits when breaking while loops. Implementing error handling and ensuring that the loop’s exit conditions are well-documented will not only improve code readability but also enhance maintainability in collaborative environments.”
Frequently Asked Questions (FAQs)
What is a while loop?
A while loop is a control flow statement that repeatedly executes a block of code as long as a specified condition evaluates to true. It is commonly used for iterations where the number of repetitions is not predetermined.
How can I break out of a while loop in Python?
In Python, you can use the `break` statement to exit a while loop prematurely. When the `break` statement is encountered, the loop terminates immediately, and control passes to the statement following the loop.
Can I use a condition to break a while loop?
Yes, you can use a condition within the loop to determine when to break. By placing an `if` statement inside the loop that checks for a specific condition, you can call the `break` statement when that condition is met.
What happens if I forget to include a break statement?
If you forget to include a break statement and the loop’s condition remains true, the while loop will continue indefinitely, leading to an infinite loop. This can cause your program to become unresponsive or crash.
Are there alternatives to using a while loop for repeated actions?
Yes, alternatives include using `for` loops, which are often more suitable when the number of iterations is known. Additionally, recursion can be used for repeated actions, although it has different implications for memory and performance.
Can I use break in nested while loops?
Yes, you can use the `break` statement in nested while loops. However, it will only exit the innermost loop that contains the `break` statement, allowing the outer loop to continue executing unless a break is also specified there.
In programming, breaking out of a while loop is a fundamental concept that allows developers to control the flow of execution based on specific conditions. A while loop continues to execute as long as its condition evaluates to true. However, there are scenarios where it becomes necessary to exit the loop prematurely. This can be achieved using various methods such as the ‘break’ statement, setting the loop condition to , or using flags to indicate when the loop should terminate.
One of the most common methods to break a while loop is by utilizing the ‘break’ statement. This statement immediately terminates the loop and transfers control to the next statement following the loop. Additionally, modifying the loop’s condition directly or employing a boolean flag can provide more control over the loop’s execution, allowing for a more structured approach to exiting the loop when certain criteria are met.
In summary, understanding how to effectively break a while loop is crucial for writing efficient and manageable code. By employing the appropriate techniques, developers can ensure that their programs run smoothly without getting stuck in infinite loops. This knowledge not only enhances code readability but also improves overall program performance.
Author Profile
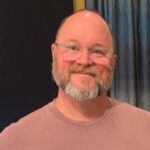
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?