How Can You Bold Text in Python? A Quick Guide
In the world of programming, clarity and emphasis are key components of effective communication. Whether you’re crafting a user interface, generating reports, or simply outputting text to the console, the ability to make certain words or phrases stand out can significantly enhance the readability and impact of your work. If you’ve ever wondered how to bold text in Python, you’re not alone. This seemingly simple task can vary based on the context in which you’re working—be it a terminal, a web application, or a graphical user interface. In this article, we will explore the various methods to achieve bold text in Python, equipping you with the tools to elevate your coding projects.
When it comes to bolding text in Python, the approach you take largely depends on the environment you’re operating in. For instance, if you’re printing to the console, you might utilize ANSI escape codes to change the text style. On the other hand, if you’re developing a web application using frameworks like Flask or Django, you might leverage HTML and CSS to achieve that bold effect. Each method has its own nuances and applications, which we will delve into, ensuring you have a comprehensive understanding of how to implement bold text across different platforms.
Moreover, as Python continues to evolve, so do the libraries and frameworks that enhance
Using ANSI Escape Codes
To create bold text in Python when outputting to the terminal, you can use ANSI escape codes. These codes are sequences of characters that control formatting on the terminal. The escape code for bold text is `\033[1m` and for resetting the formatting is `\033[0m`. Here is an example:
“`python
print(“\033[1mThis text is bold\033[0m and this is normal.”)
“`
This method works in many terminal emulators that support ANSI codes, allowing you to emphasize important messages in your output.
Using Libraries for Rich Text Formatting
If you require more complex formatting, using libraries such as `rich` or `colorama` can enhance your ability to produce bold text and other styles easily.
- Rich: This library allows you to render bold text and much more in a user-friendly way. To use it, first install the library using pip:
“`bash
pip install rich
“`
Then, you can use the following code to display bold text:
“`python
from rich.console import Console
console = Console()
console.print(“This is bold text”, style=”bold”)
“`
- Colorama: This library can also be used for colored output, including bold text. Install it via pip:
“`bash
pip install colorama
“`
Example usage:
“`python
from colorama import init, Style
init()
print(Style.BRIGHT + “This is bold text” + Style.RESET_ALL)
“`
Formatting Strings in Jupyter Notebooks
In Jupyter notebooks, bold text can be created using Markdown. You can use double asterisks or double underscores to make text bold. For example:
“`markdown
This text is bold
“`
or
“`markdown
__This text is bold__
“`
When you run the cell, it will display the text in bold format.
Comparison of Methods for Bold Text
The table below summarizes the different methods available for making text bold in Python, including their use cases and environments:
Method | Library/Tool | Environment | Example Code |
---|---|---|---|
ANSI Escape Codes | None | Terminal | print(“\033[1mBold\033[0m”) |
Rich | rich | Terminal, Scripts | console.print(“Bold”, style=”bold”) |
Colorama | colorama | Terminal, Scripts | print(Style.BRIGHT + “Bold” + Style.RESET_ALL) |
Markdown | Jupyter | Jupyter Notebooks | **Bold** |
These methods provide flexibility depending on the environment in which you are working, allowing you to emphasize text effectively in Python applications.
Bold Text in Console Output
In Python, there are various ways to display bold text in the console. The most common methods involve using ANSI escape codes or third-party libraries. Below are detailed approaches:
Using ANSI Escape Codes
ANSI escape codes can be utilized to format console output, including making text bold. Below is an example of how to implement this:
“`python
ANSI escape code for bold text
BOLD = ‘\033[1m’
RESET = ‘\033[0m’
print(f”{BOLD}This text is bold!{RESET}”)
“`
- BOLD: `\033[1m` is the escape code for bold text.
- RESET: `\033[0m` resets the text formatting back to normal.
Using the Colorama Library
The Colorama library simplifies working with console text formatting across different operating systems. To use Colorama for bold text, follow these steps:
- Install Colorama if it isn’t already:
“`bash
pip install colorama
“`
- Example code to display bold text:
“`python
from colorama import init, Style
init(autoreset=True) Automatically resets styles after each print
print(f”{Style.BRIGHT}This text is bold!{Style.NORMAL}”)
“`
Key Functions
Function | Description |
---|---|
`init()` | Initializes Colorama. |
`Style.BRIGHT` | Applies bold formatting to text. |
`Style.NORMAL` | Resets text formatting to default. |
Bold Text in GUI Applications
When working with graphical user interfaces (GUIs), the method for displaying bold text varies by library. Here are examples for two popular libraries: Tkinter and PyQt.
Using Tkinter
In Tkinter, you can use the `font` module to create bold text:
“`python
import tkinter as tk
from tkinter import font
root = tk.Tk()
bold_font = font.Font(weight=”bold”)
label = tk.Label(root, text=”This is bold text”, font=bold_font)
label.pack()
root.mainloop()
“`
Using PyQt5
For PyQt5 applications, you can set text properties using `QFont`:
“`python
from PyQt5.QtWidgets import QApplication, QLabel
from PyQt5.QtGui import QFont
app = QApplication([])
label = QLabel(“This is bold text”)
font = QFont()
font.setBold(True)
label.setFont(font)
label.show()
app.exec_()
“`
Comparison of GUI Libraries
Library | Method for Bold Text |
---|---|
Tkinter | Use `font.Font(weight=”bold”)` |
PyQt5 | Use `QFont.setBold(True)` |
Bold Text in Web Applications with Flask
When developing web applications using Flask, you can render bold text using HTML tags directly in your templates:
“`html
This is bold text
“`
In your Flask route, render the template as follows:
“`python
from flask import Flask, render_template
app = Flask(__name__)
@app.route(‘/’)
def home():
return render_template(‘index.html’)
“`
This approach leverages HTML’s inherent capability to display bold text using the `` or `` tags.
Expert Insights on Formatting Text in Python
Dr. Emily Carter (Senior Software Engineer, CodeCraft Solutions). “To bold text in Python, particularly when working with libraries like Rich or using Jupyter Notebooks, it is essential to understand the formatting capabilities of these environments. For instance, using Markdown syntax or the Rich library’s built-in methods can significantly enhance the readability of your output.”
Michael Chen (Python Developer, Tech Innovations Inc.). “When it comes to terminal applications, leveraging ANSI escape codes is a powerful way to achieve bold text. However, for GUI applications, utilizing frameworks such as Tkinter or PyQt can provide more user-friendly options for text styling.”
Lisa Thompson (Data Scientist, Analytics Pro). “In data visualization libraries like Matplotlib, bolding text can be accomplished through font weight parameters in the text functions. This is crucial for emphasizing key points in graphs and charts, ensuring that the information is conveyed effectively.”
Frequently Asked Questions (FAQs)
How can I bold text in a Python console application?
To bold text in a Python console application, you can use ANSI escape codes. For example, `print(“\033[1mThis text is bold\033[0m”)` will display the text in bold in terminals that support ANSI codes.
Is there a way to bold text in a Python GUI application?
Yes, in GUI frameworks like Tkinter, you can use the `font` module to set the font weight to bold. For instance, `font.Font(weight=”bold”)` can be applied to a label or text widget to make the text bold.
Can I bold text in Jupyter Notebooks?
In Jupyter Notebooks, you can use Markdown to bold text. Simply wrap the text with double asterisks or double underscores, like `This text is bold` or `__This text is bold__`, and it will render as bold when the cell is executed.
How do I bold text in a Python web application using Flask?
In a Flask web application, you can use HTML tags to bold text. For example, you can return a string like `”This text is bold“` in your route, and it will render as bold in the browser.
Are there libraries that help with text formatting in Python?
Yes, libraries such as `rich` and `colorama` can help format text, including making it bold. For example, using `from rich.console import Console; console = Console(); console.print(“This text is bold”, style=”bold”)` will display bold text in the console.
Can I bold text in Python when generating PDF files?
Yes, when generating PDF files using libraries like `ReportLab` or `FPDF`, you can specify the font style as bold. For instance, in ReportLab, you can use `canvas.setFont(“Helvetica-Bold”, 12)` to set the font to bold before writing text.
In Python, bolding text can be achieved through various methods, depending on the context in which the text is being displayed. For console applications, ANSI escape codes can be utilized to format text output, allowing users to create bold text in terminal environments. In graphical user interfaces, libraries such as Tkinter and PyQt provide options to set font styles, including bold, for text elements. Additionally, when generating HTML content, the use of HTML tags like `` or `` can effectively render text in bold when viewed in a web browser.
It is essential to choose the appropriate method for bolding text based on the specific application and its requirements. For instance, while ANSI codes are suitable for command-line interfaces, GUI applications may benefit more from the font styling capabilities offered by dedicated libraries. Furthermore, understanding the context in which the text will be displayed ensures that the bold formatting enhances readability and user experience.
In summary, bolding text in Python is a straightforward process that can be accomplished through various techniques. By leveraging the right tools and libraries, developers can effectively emphasize important information in their applications. As Python continues to evolve, staying informed about the latest libraries and methods will further enhance the ability to format text dynamically and effectively.
Author Profile
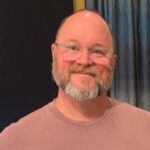
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?