How Can You Add a Title to a Histogram in Python?
Creating visually appealing and informative data visualizations is a crucial aspect of data analysis, and histograms are among the most effective tools for representing the distribution of numerical data. A well-crafted histogram not only highlights the underlying patterns in your dataset but also conveys your insights clearly to your audience. However, one often overlooked element in creating impactful visualizations is the title. A descriptive title can provide context, guide interpretation, and enhance the overall presentation of your histogram. In this article, we will explore how to add a title to a histogram in Python, ensuring your visualizations are both informative and engaging.
When working with Python, particularly with libraries like Matplotlib and Seaborn, adding a title to your histogram is a straightforward yet essential step. A title serves as a succinct summary of what the viewer can expect from the visual representation, setting the stage for understanding the data being presented. Whether you are illustrating the distribution of test scores, sales figures, or any other dataset, a clear title can significantly enhance the viewer’s comprehension and interest.
In the following sections, we will delve into the various methods for adding titles to histograms in Python, showcasing best practices and tips to ensure your titles are not only descriptive but also visually appealing. From simple commands to more advanced formatting options, you will
Using Matplotlib to Add a Title
To add a title to a histogram in Python, the most common library used is Matplotlib. This powerful library allows for extensive customization of plots, including titles. Here’s how to do it:
- Import Matplotlib: Begin by importing the necessary library.
- Create your histogram: Use the `hist()` function to generate the histogram.
- Add the title: Utilize the `title()` function to set the title of the histogram.
Here’s a simple example demonstrating these steps:
“`python
import matplotlib.pyplot as plt
import numpy as np
Sample data
data = np.random.randn(1000)
Create histogram
plt.hist(data, bins=30, alpha=0.5)
Add title
plt.title(‘Histogram of Random Data’)
Show plot
plt.show()
“`
In this example, `plt.title(‘Histogram of Random Data’)` effectively adds the title above the histogram.
Customizing the Title
Matplotlib allows for further customization of the title, including font size, color, and style. The `title()` function accepts several parameters to enhance the appearance of the title.
Key parameters include:
- fontsize: To specify the size of the font.
- color: To change the color of the title text.
- fontweight: To set the weight of the font (e.g., bold).
- loc: To determine the alignment of the title (e.g., ‘left’, ‘center’, ‘right’).
Here’s an example of a customized title:
“`python
plt.title(‘Histogram of Random Data’, fontsize=14, color=’blue’, fontweight=’bold’, loc=’center’)
“`
Adding Subtitles and Multiple Titles
If you wish to add a subtitle or multiple titles, you can use the `suptitle()` function, which places a title above the main title in a subplot. Here’s an example:
“`python
plt.suptitle(‘Main Title: Data Analysis’, fontsize=16)
plt.title(‘Histogram of Random Data’, fontsize=14)
“`
This approach provides a clear structure to your visualization, ensuring that the viewer can easily understand the context.
Example with Table
To illustrate how different parameters affect the appearance of the title, consider the following table:
Parameter | Description | Example Value |
---|---|---|
fontsize | Size of the title font | 14 |
color | Color of the title text | ‘blue’ |
fontweight | Weight of the font | ‘bold’ |
loc | Alignment of the title | ‘center’ |
This table summarizes key parameters that help customize the title of your histogram effectively. By leveraging these options, you can enhance the visual appeal and clarity of your data presentation.
Adding a Title to a Histogram
To add a title to a histogram in Python, you typically use the Matplotlib library, which provides a straightforward approach to customizing plots. Here’s how you can achieve this effectively.
Using Matplotlib to Create a Histogram
First, ensure you have the Matplotlib library installed. You can install it using pip if it is not already available in your environment:
“`bash
pip install matplotlib
“`
Once you have Matplotlib installed, you can create a histogram and add a title using the following steps:
- Import the necessary libraries:
“`python
import matplotlib.pyplot as plt
import numpy as np
“`
- Generate some data:
For demonstration, you can create a sample dataset:
“`python
data = np.random.randn(1000) Generates 1000 random numbers
“`
- Create the histogram:
Use the `plt.hist()` function to create the histogram:
“`python
plt.hist(data, bins=30, color=’blue’, alpha=0.7)
“`
- Add a title:
Use the `plt.title()` method to set the title of the histogram:
“`python
plt.title(‘Distribution of Random Numbers’)
“`
- Show the plot:
Finally, display the histogram with the title:
“`python
plt.show()
“`
Example Code
Here is the complete code for creating a histogram with a title:
“`python
import matplotlib.pyplot as plt
import numpy as np
Generate random data
data = np.random.randn(1000)
Create histogram
plt.hist(data, bins=30, color=’blue’, alpha=0.7)
Add title
plt.title(‘Distribution of Random Numbers’)
Show plot
plt.show()
“`
Customizing the Title
Matplotlib allows you to customize the title in various ways. You can modify:
- Font size: Change the size of the title text using the `fontsize` parameter.
- Font weight: Make the title bold by setting `fontweight` to ‘bold’.
- Color: Change the color of the title text using the `color` parameter.
Here’s an example of how to implement these customizations:
“`python
plt.title(‘Distribution of Random Numbers’, fontsize=16, fontweight=’bold’, color=’darkred’)
“`
Additional Customizations
You can also customize other aspects of the histogram, including:
- Axis labels: Use `plt.xlabel()` and `plt.ylabel()` to add labels to the x-axis and y-axis, respectively.
- Grid: Add a grid for better readability using `plt.grid()`.
Example of including these customizations:
“`python
plt.xlabel(‘Value’)
plt.ylabel(‘Frequency’)
plt.grid(True)
“`
Incorporating these customizations, the full code can look like this:
“`python
import matplotlib.pyplot as plt
import numpy as np
data = np.random.randn(1000)
plt.hist(data, bins=30, color=’blue’, alpha=0.7)
plt.title(‘Distribution of Random Numbers’, fontsize=16, fontweight=’bold’, color=’darkred’)
plt.xlabel(‘Value’)
plt.ylabel(‘Frequency’)
plt.grid(True)
plt.show()
“`
With these instructions, you can effectively add titles and customize your histograms in Python using Matplotlib.
Expert Insights on Adding Titles to Histograms in Python
Dr. Emily Carter (Data Scientist, Analytics Innovations). “Adding a title to a histogram in Python is essential for clarity. It provides context to the viewer, helping them understand the data being presented. Utilizing libraries like Matplotlib, one can easily implement titles using the `plt.title()` function, enhancing the overall interpretability of the visualization.”
Mark Chen (Senior Data Visualization Specialist, Visualize It Inc.). “Incorporating a title into your histogram not only informs the audience about the data set but also sets the tone for the analysis. I recommend using descriptive titles that reflect the data’s story. This can be achieved seamlessly in Python with the `title` method from the Matplotlib library, which allows customization in font size and style.”
Linda Garcia (Python Programming Instructor, Code Academy). “When teaching students how to create histograms in Python, I emphasize the importance of titles. A well-crafted title can guide the audience’s understanding and engagement. The `plt.title()` function in Matplotlib is straightforward and should be a fundamental part of any histogram creation process, ensuring that the visual communicates effectively.”
Frequently Asked Questions (FAQs)
How do I add a title to a histogram in Python?
To add a title to a histogram in Python, use the `title()` function from the Matplotlib library after plotting the histogram. For example:
“`python
import matplotlib.pyplot as plt
plt.hist(data)
plt.title(‘Your Title Here’)
plt.show()
“`
Can I customize the title font size and style in a histogram?
Yes, you can customize the title font size and style by passing additional parameters to the `title()` function. For instance:
“`python
plt.title(‘Your Title Here’, fontsize=14, fontweight=’bold’)
“`
Is it possible to add a subtitle to a histogram in Python?
While Matplotlib does not have a direct `subtitle()` function, you can simulate a subtitle by adding another text element using `text()` or `suptitle()` for a more structured layout. Example:
“`python
plt.suptitle(‘Main Title’, fontsize=16)
plt.title(‘Subtitle Here’, fontsize=12)
“`
Can I change the position of the title in a histogram?
Yes, you can change the position of the title by using the `loc` parameter in the `title()` function. For example:
“`python
plt.title(‘Your Title Here’, loc=’right’)
“`
What libraries are commonly used to create histograms in Python?
The most commonly used libraries for creating histograms in Python are Matplotlib and Seaborn. Both libraries provide extensive functionality for customizing plots, including adding titles.
How can I save a histogram with a title to a file in Python?
To save a histogram with a title to a file, use the `savefig()` function after plotting. For example:
“`python
plt.hist(data)
plt.title(‘Your Title Here’)
plt.savefig(‘histogram.png’)
“`
In summary, adding a title to a histogram in Python is a straightforward process that enhances the clarity and interpretability of the visualized data. Utilizing libraries such as Matplotlib, which is widely used for plotting in Python, allows users to easily incorporate titles into their histograms. The primary function for this purpose is `plt.title()`, which can be called after creating the histogram to set an informative title that reflects the data being represented.
Moreover, it is important to consider the context of the data when crafting a title. A well-chosen title not only provides insight into what the histogram represents but also engages the audience, making the visualization more effective. Users can customize the title further by adjusting parameters such as font size, style, and color, thereby enhancing the overall presentation of the histogram.
mastering the addition of titles to histograms in Python is an essential skill for data visualization. By leveraging the capabilities of libraries like Matplotlib, users can create more informative and visually appealing representations of their data, ultimately leading to better analysis and communication of insights derived from the data.
Author Profile
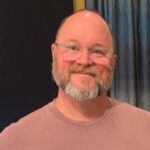
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?