How Can You Add a String to a List in Python?
In the world of programming, lists are one of the most versatile and widely used data structures, particularly in Python. They allow you to store multiple items in a single variable, making it easy to manage collections of data. Whether you’re building a simple application or tackling complex data manipulation tasks, knowing how to efficiently add strings—or any other elements—to a list is an essential skill for any Python developer. In this article, we will explore the various methods for adding strings to lists in Python, empowering you to enhance your coding toolkit and streamline your projects.
When working with lists in Python, the ability to dynamically add elements is crucial. This flexibility allows you to modify your data structures on the fly, accommodating changes in your program’s requirements. From appending a single string to inserting multiple entries at once, Python offers several built-in methods to handle these tasks seamlessly. Understanding these techniques not only improves your coding efficiency but also enhances the readability and maintainability of your code.
As we delve deeper into the topic, we will cover the most common approaches to adding strings to lists, including the use of methods like `append()`, `extend()`, and `insert()`. Each of these methods serves a unique purpose and can be applied in different scenarios, providing you with the tools
Using the append() Method
The most straightforward way to add a string to a list in Python is by using the `append()` method. This method allows you to add a single element to the end of the list. The syntax is simple and intuitive:
“`python
my_list = [‘apple’, ‘banana’, ‘cherry’]
my_list.append(‘orange’)
“`
After executing the above code, `my_list` will become `[‘apple’, ‘banana’, ‘cherry’, ‘orange’]`. The `append()` method modifies the list in place and returns `None`.
Utilizing the insert() Method
If you need to add a string at a specific position within the list, the `insert()` method is the ideal choice. This method takes two arguments: the index at which to insert the new element and the element itself. For instance:
“`python
my_list = [‘apple’, ‘banana’, ‘cherry’]
my_list.insert(1, ‘orange’)
“`
In this example, `my_list` will now be `[‘apple’, ‘orange’, ‘banana’, ‘cherry’]`, as the string “orange” is inserted at index 1.
Combining Lists with the extend() Method
When you want to add multiple strings to a list, the `extend()` method is useful. It allows you to concatenate another iterable (like a list of strings) to your existing list. Here’s how it works:
“`python
my_list = [‘apple’, ‘banana’]
my_list.extend([‘cherry’, ‘orange’])
“`
After this operation, `my_list` will be `[‘apple’, ‘banana’, ‘cherry’, ‘orange’]`. The `extend()` method also modifies the list in place and is efficient for adding multiple elements.
Using the + Operator
Another way to combine lists is through the use of the `+` operator. This approach creates a new list that consists of the elements of both lists. Here’s an example:
“`python
my_list = [‘apple’, ‘banana’]
new_list = my_list + [‘cherry’, ‘orange’]
“`
In this case, `new_list` will be `[‘apple’, ‘banana’, ‘cherry’, ‘orange’]`, while `my_list` remains unchanged.
Table of Methods for Adding Strings to a List
Method | Description | Example |
---|---|---|
append() | Adds a single element to the end of the list. | my_list.append(‘orange’) |
insert() | Inserts an element at a specified index. | my_list.insert(1, ‘orange’) |
extend() | Appends multiple elements from another iterable. | my_list.extend([‘cherry’, ‘orange’]) |
+ | Concatenates two lists, creating a new one. | new_list = my_list + [‘cherry’, ‘orange’] |
Adding strings to a list in Python can be accomplished in several ways, depending on your specific needs. The methods discussed above provide flexibility for both single and multiple additions, ensuring that you can manipulate lists effectively in your programming tasks.
Methods to Add a String to a List in Python
In Python, there are multiple methods to add a string to a list, each suited to different scenarios. Below are the most common techniques.
Using the `append()` Method
The `append()` method adds a single element to the end of a list. This is the most straightforward way to add a string.
“`python
my_list = [‘apple’, ‘banana’]
my_list.append(‘cherry’)
print(my_list) Output: [‘apple’, ‘banana’, ‘cherry’]
“`
Using the `extend()` Method
The `extend()` method can be used to add multiple elements to a list. When adding a string, it treats each character in the string as a separate element.
“`python
my_list = [‘apple’, ‘banana’]
my_list.extend(‘cherry’)
print(my_list) Output: [‘apple’, ‘banana’, ‘c’, ‘h’, ‘e’, ‘r’, ‘r’, ‘y’]
“`
To add a string as a single element, it’s better to use `append()` or wrap the string in a list:
“`python
my_list.extend([‘cherry’])
print(my_list) Output: [‘apple’, ‘banana’, ‘cherry’]
“`
Using the `insert()` Method
The `insert()` method allows you to add a string at a specific position in the list. The first argument is the index where the string should be added, and the second argument is the string itself.
“`python
my_list = [‘apple’, ‘banana’]
my_list.insert(1, ‘cherry’)
print(my_list) Output: [‘apple’, ‘cherry’, ‘banana’]
“`
Using List Concatenation
You can also concatenate lists to create a new list that includes the original elements and the new string. This method is not in-place and results in a new list.
“`python
my_list = [‘apple’, ‘banana’]
my_list = my_list + [‘cherry’]
print(my_list) Output: [‘apple’, ‘banana’, ‘cherry’]
“`
Using List Comprehension
Another method is to use list comprehension to create a new list that includes the original elements along with the new string.
“`python
my_list = [‘apple’, ‘banana’]
my_list = [item for item in my_list] + [‘cherry’]
print(my_list) Output: [‘apple’, ‘banana’, ‘cherry’]
“`
Comparison of Methods
Method | In-place Modification | Adds Multiple Elements | Specific Position |
---|---|---|---|
`append()` | Yes | No | No |
`extend()` | Yes | Yes | No |
`insert()` | Yes | No | Yes |
Concatenation | No | Yes | No |
List Comprehension | No | Yes | No |
Each method has its advantages depending on the requirements of your specific use case. Choose the one that best fits your needs for adding strings to a list in Python.
Expert Insights on Adding Strings to Lists in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “In Python, the most straightforward way to add a string to a list is by using the `append()` method. This method allows you to add a single element to the end of the list, which is particularly useful when dynamically building a collection of strings.”
Michael Tran (Python Developer, CodeMaster Solutions). “Another effective approach is to use the `insert()` method, which enables you to specify the index at which you want to add the string. This can be beneficial when the order of elements in your list is crucial.”
Lisa Chen (Data Scientist, Analytics Hub). “For scenarios where you need to add multiple strings at once, consider using the `extend()` method. This method efficiently appends elements from an iterable, allowing you to expand your list without the need for multiple calls to `append()`.”
Frequently Asked Questions (FAQs)
How do I add a string to a list in Python?
You can add a string to a list in Python using the `append()` method. For example, if you have a list named `my_list`, you can add a string `my_string` by executing `my_list.append(my_string)`.
Can I add multiple strings to a list at once?
Yes, you can add multiple strings to a list using the `extend()` method. For instance, if you have a list `my_list` and a list of strings `my_strings`, you can execute `my_list.extend(my_strings)` to add all strings from `my_strings` to `my_list`.
What happens if I use the `insert()` method to add a string?
The `insert()` method allows you to add a string at a specific index in the list. For example, `my_list.insert(0, my_string)` will add `my_string` at the beginning of `my_list`, shifting other elements to the right.
Is there a difference between `append()` and `extend()`?
Yes, `append()` adds a single element (including a string) to the end of the list, while `extend()` adds multiple elements from an iterable (like a list of strings) to the end of the list.
Can I add a string to a list using the `+` operator?
You can use the `+` operator to concatenate lists. For example, if you have a list `my_list` and a string `my_string`, you can create a new list with `my_list + [my_string]`, but this does not modify `my_list` in place.
What is the best practice for adding items to a list in Python?
The best practice is to use `append()` for adding single items and `extend()` for adding multiple items. This approach ensures clarity and maintains the intended structure of the list.
In Python, adding a string to a list can be accomplished using several methods, each serving different use cases. The most common approach is to use the `append()` method, which adds the string as a single element at the end of the list. Alternatively, the `insert()` method allows for more flexibility by enabling the addition of the string at a specified index within the list. Furthermore, the `extend()` method can be utilized to add multiple strings or elements from another iterable, effectively merging them into the existing list.
Understanding these methods is crucial for effective list manipulation in Python. The choice of method depends on the desired outcome—whether one needs to maintain the structure of the list, insert elements at specific positions, or combine multiple strings into a single list. Each method provides a straightforward way to enhance the functionality of lists, which are fundamental data structures in Python programming.
In summary, mastering the techniques for adding strings to lists not only improves code efficiency but also enhances overall programming skills in Python. By selecting the appropriate method based on the context, developers can ensure their code remains clean, readable, and efficient. This knowledge is essential for anyone looking to work effectively with lists in Python.
Author Profile
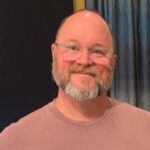
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?