How Can You Access Session Variables in JavaScript?
In the dynamic world of web development, managing user sessions effectively is crucial for creating seamless and personalized experiences. As users navigate through your application, maintaining their state and preferences can significantly enhance usability and engagement. One of the key components in this process is the use of session variables, which allow developers to store and retrieve information about a user’s session. In this article, we will explore how to access session variables in JavaScript, empowering you to harness the full potential of your web applications.
Session variables act as temporary storage for user data, enabling developers to keep track of user interactions without requiring constant server communication. By leveraging these variables, you can create a more interactive and responsive user experience, such as remembering user preferences or maintaining login states. In JavaScript, accessing session variables is straightforward, but understanding the underlying principles and best practices is essential for effective implementation.
As we delve deeper into the topic, we will discuss various methods for accessing session variables, including the use of the `sessionStorage` API and server-side techniques. Whether you are building a simple web application or a complex platform, mastering session variable management will equip you with the tools necessary to enhance user experience and streamline application performance. Get ready to unlock the secrets of session variables in JavaScript and take your web
Understanding Session Variables
Session variables are a method of storing data that is specific to a user’s session on a web application. These variables exist on the server side and can be accessed by the client through various means, primarily using JavaScript in conjunction with server-side languages.
In JavaScript, accessing session variables generally involves making requests to the server, as JavaScript on its own cannot directly access server-side session data. This can be achieved through AJAX calls or by embedding the session data within the HTML sent to the client.
Accessing Session Variables via AJAX
To access session variables using AJAX, you will typically follow these steps:
- Make an AJAX call to a server-side script (such as PHP, Node.js, or Python) that retrieves the session variable.
- The server-side script should return the session variable in a format that JavaScript can easily process, such as JSON.
- Use JavaScript to handle the response and utilize the session variable as needed.
Here’s an example using jQuery to fetch a session variable:
“`javascript
$.ajax({
url: ‘getSessionVariable.php’, // Server-side script
type: ‘GET’,
dataType: ‘json’,
success: function(data) {
console.log(data.sessionVariable); // Use the session variable
},
error: function(xhr, status, error) {
console.error(‘Error fetching session variable:’, error);
}
});
“`
Embedding Session Variables in HTML
Another method to access session variables is to embed them directly within the HTML that is generated by the server. This approach can be efficient for small data sets.
For example, in PHP you can do the following:
“`php
“`
This script tag will make the session variable available to the JavaScript running on the client side.
Common Use Cases for Session Variables
Session variables are often employed in web applications for various purposes, including:
- User Authentication: Storing user credentials or tokens to maintain logged-in status.
- Shopping Carts: Keeping track of items added to a cart during a shopping session.
- User Preferences: Retaining user-selected settings or configurations across pages.
Example Table of Session Variables
The following table illustrates potential session variables and their purposes:
Session Variable Name | Description |
---|---|
user_id | Stores the unique identifier for the logged-in user. |
cart_items | Holds the list of items in the user’s shopping cart. |
preferences | Contains user-defined preferences for the application. |
By utilizing these methods, developers can effectively manage and access session variables in JavaScript, enhancing the user experience by providing tailored content and functionality.
Understanding Session Variables
Session variables are used to store data for a user’s session. They persist while the browser is open and are typically used to maintain user state across multiple pages in a web application. In JavaScript, accessing session variables typically involves the use of the `sessionStorage` object.
Using sessionStorage
The `sessionStorage` object allows you to store data in the browser for the duration of the page session. Data stored in `sessionStorage` is accessible only within the same tab and is cleared when the tab is closed.
- Storing a Value: Use the `setItem` method to store data.
“`javascript
sessionStorage.setItem(‘key’, ‘value’);
“`
- Retrieving a Value: Use the `getItem` method to retrieve data.
“`javascript
const value = sessionStorage.getItem(‘key’);
“`
- Removing a Value: Use the `removeItem` method to delete specific data.
“`javascript
sessionStorage.removeItem(‘key’);
“`
- Clearing All Data: Use the `clear` method to remove all session variables.
“`javascript
sessionStorage.clear();
“`
Example of Accessing Session Variables
Here’s an example that demonstrates how to set, retrieve, and remove session variables in JavaScript:
“`javascript
// Store session variable
sessionStorage.setItem(‘username’, ‘JohnDoe’);
// Retrieve session variable
const username = sessionStorage.getItem(‘username’);
console.log(username); // Outputs: JohnDoe
// Remove session variable
sessionStorage.removeItem(‘username’);
“`
Considerations When Using Session Variables
When utilizing session variables, consider the following:
Aspect | Description |
---|---|
Scope | Data is limited to the current tab or window. |
Lifespan | Data persists until the tab is closed, unlike cookies which can have longer lifespans. |
Size Limit | Typically, browsers limit storage to about 5MB per origin. |
Data Type | All data stored as strings; objects must be serialized (e.g., JSON). |
Best Practices
To effectively manage session variables, adhere to these best practices:
- Use Descriptive Keys: Ensure that keys are intuitive and descriptive to avoid confusion.
- Limit Data Storage: Store only necessary data to optimize performance and avoid exceeding storage limits.
- Handle Data Serialization: For complex data types, use `JSON.stringify()` to store and `JSON.parse()` to retrieve.
- Security Considerations: Avoid storing sensitive information like passwords in session storage.
Common Use Cases
Session variables are commonly used in the following scenarios:
- User authentication states (e.g., storing user IDs or tokens).
- Maintaining user preferences across different pages.
- Tracking temporary data during a multi-step process (e.g., in forms or wizards).
By leveraging session variables effectively, developers can enhance user experience and application performance.
Expert Insights on Accessing Session Variables in JavaScript
Dr. Emily Carter (Web Development Specialist, Tech Innovations Inc.). “Accessing session variables in JavaScript is crucial for maintaining user state across multiple pages. Utilizing the `sessionStorage` API allows developers to store data for the duration of the page session, and it can be accessed easily through methods like `sessionStorage.getItem()`.”
Michael Chen (Senior Software Engineer, CodeCraft Solutions). “When dealing with session variables, it is essential to understand the difference between `sessionStorage` and `localStorage`. While both are part of the Web Storage API, `sessionStorage` is designed for temporary data that lasts only for the session, making it ideal for sensitive information that should not persist beyond the tab’s lifetime.”
Sarah Thompson (Lead Frontend Developer, Digital Dynamics). “To effectively manage session variables in JavaScript, developers should implement proper error handling when accessing sessionStorage. This ensures that if a variable is not found, the application can gracefully handle the situation without crashing, thereby enhancing user experience.”
Frequently Asked Questions (FAQs)
What are session variables in JavaScript?
Session variables are temporary data storage mechanisms that persist for the duration of a user’s session on a web application. They are often used to store user-specific information such as login status or preferences.
How can I set session variables in JavaScript?
In JavaScript, session variables can be set using the `sessionStorage` object. You can use the `setItem(key, value)` method to store data, where `key` is the name of the variable and `value` is the data you want to store.
How do I retrieve session variables in JavaScript?
To retrieve session variables, use the `sessionStorage.getItem(key)` method, where `key` is the name of the session variable you want to access. This method returns the value associated with the specified key.
Can session variables be accessed across different tabs in the same browser?
Yes, session variables stored in `sessionStorage` are accessible across different tabs within the same browser window as long as they are part of the same session. However, they are not shared across different browser windows or incognito modes.
How do I remove session variables in JavaScript?
To remove a specific session variable, use the `sessionStorage.removeItem(key)` method. To clear all session variables, use the `sessionStorage.clear()` method, which removes all key/value pairs stored in session storage.
Are session variables secure for storing sensitive information?
Session variables are not inherently secure. Sensitive information should be encrypted before being stored in session variables, and developers should implement additional security measures to protect user data.
Accessing session variables in JavaScript is a crucial aspect of web development, particularly when managing user sessions and maintaining state across different pages. Session variables are typically stored on the server side, and JavaScript can interact with these variables through various methods, such as AJAX calls or by using frameworks that facilitate server communication. Understanding how to properly access and manipulate these variables is essential for creating dynamic and responsive web applications.
One of the key takeaways is the importance of using session storage in JavaScript for client-side management of temporary data. The Web Storage API, particularly the sessionStorage object, allows developers to store data for the duration of the page session. This is particularly useful for retaining user preferences or form data without requiring server-side storage. However, it is important to note that sessionStorage is limited to the specific tab or window and does not persist across different sessions.
Additionally, leveraging AJAX to fetch session variables from the server can enhance user experience by allowing real-time updates without requiring a page refresh. This method enables developers to maintain a seamless interaction with the server, ensuring that session variables are accessed and updated as needed. Overall, understanding both client-side and server-side techniques for handling session variables is vital for effective web development.
Author Profile
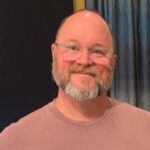
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?