How Do I Pass an Array as a Query Parameter in My API Requests?
In the world of web development, the ability to effectively communicate between the client and server is paramount. One common challenge developers face is how to pass complex data structures, such as arrays, through URL query parameters. Whether you’re building a dynamic web application or an API, understanding the nuances of how to encode and transmit arrays as query parameters can significantly enhance the functionality and user experience of your application. This article will guide you through the intricacies of passing arrays as query parameters, ensuring that your data is transmitted accurately and efficiently.
When it comes to passing arrays as query parameters, the approach can vary depending on the programming language or framework you are using. Different technologies have their own conventions and methods for encoding arrays, which can lead to confusion if not handled properly. By grasping the fundamental principles behind query parameter encoding, you can ensure that your application communicates seamlessly with the server, regardless of the complexity of the data being sent.
Additionally, understanding how to structure your query parameters can help you avoid common pitfalls, such as data loss or misinterpretation on the server side. As we delve deeper into this topic, we will explore various techniques and best practices for passing arrays as query parameters, equipping you with the knowledge to tackle this essential aspect of web development with confidence. Whether you’re a
Passing Arrays in URL Query Parameters
When working with web applications, passing arrays as query parameters can be essential for effectively transmitting multiple values. Different programming languages and frameworks handle this task in various ways, and understanding these differences can help ensure proper data handling.
Common Formats for Passing Arrays
Arrays can be represented in URL query parameters using several common formats. The choice of format may depend on the server-side technology being used. Here are the most prevalent formats:
- Comma-Separated Values:
- Example: `?items=value1,value2,value3`
- Repeated Parameters:
- Example: `?items=value1&items=value2&items=value3`
- Index-Based Parameters:
- Example: `?items[0]=value1&items[1]=value2&items[2]=value3`
Each of these formats has implications for how the server-side code parses the incoming data.
Format | Example | Parsing Method |
---|---|---|
Comma-Separated Values | ?items=value1,value2,value3 | Split string on commas |
Repeated Parameters | ?items=value1&items=value2&items=value3 | Collect values into an array |
Index-Based Parameters | ?items[0]=value1&items[1]=value2&items[2]=value3 | Map values based on index |
Implementing Array Parameters in Different Languages
The implementation of array parameters can vary significantly between different programming languages. Below are examples for JavaScript (Node.js) and PHP.
JavaScript (Node.js)
In Node.js, when using the Express framework, the query parameters can be accessed directly through the `req.query` object. Here’s an example of handling repeated parameters:
“`javascript
app.get(‘/items’, (req, res) => {
const items = req.query.items; // Will be an array if repeated parameters are used
res.send(items);
});
“`
For a comma-separated format, you would handle it as follows:
“`javascript
app.get(‘/items’, (req, res) => {
const items = req.query.items.split(‘,’); // Split the string into an array
res.send(items);
});
“`
PHP
In PHP, query parameters are automatically parsed into the `$_GET` superglobal. For repeated parameters, PHP will automatically convert them into an array:
“`php
$items = $_GET[‘items’]; // Automatically an array if repeated
“`
For comma-separated values, you would parse the string:
“`php
$items = explode(‘,’, $_GET[‘items’]); // Convert string to array
“`
Best Practices
When passing arrays as query parameters, consider the following best practices:
- Keep it Simple: Use the simplest format that meets your needs.
- Document Your API: Clearly document how arrays should be passed in your API.
- Limit Size: Be mindful of URL length limits; most browsers have a limit of around 2000 characters.
- Encode Values: Always URL-encode parameter values to avoid issues with special characters.
By adhering to these practices, you can ensure better interoperability and user experience in your web applications.
Passing Arrays in Query Parameters
To pass an array as a query parameter in a URL, it is essential to follow a specific format that is widely recognized by web servers and APIs. The general approach depends on the programming language or framework being used, but the underlying principles remain consistent across various technologies.
Common Formats for Query Parameters
When passing arrays as query parameters, several formats can be utilized. Here are the most common methods:
- Repeated Key Method: Each value in the array is represented by the same key.
- Example: `?item=value1&item=value2&item=value3`
- Indexed Key Method: Each value is indexed in the key.
- Example: `?item[0]=value1&item[1]=value2&item[2]=value3`
- Comma-Separated Values: All values are concatenated in a single string, typically separated by commas.
- Example: `?item=value1,value2,value3`
Implementing in Various Languages
Different programming languages and libraries have unique methods for constructing query strings with arrays. Below is a table showcasing how to implement each method in popular languages:
Language | Method | Example Code |
---|---|---|
JavaScript | Repeated Key Method |
`const params = new URLSearchParams();` `array.forEach(item => params.append(‘item’, item));` |
Python (requests) | Repeated Key Method |
`params = {‘item’: array}` `response = requests.get(url, params=params)` |
PHP | Indexed Key Method | `http_build_query([‘item’ => $array])` |
Java (Spring) | Indexed Key Method |
`@RequestParam List |
Encoding and Decoding
When passing arrays as query parameters, ensure that the data is correctly encoded to handle special characters. Most programming languages provide functions or libraries to encode URLs properly.
- URL Encoding: Use functions like `encodeURIComponent` in JavaScript or `urllib.parse.quote` in Python to encode your parameters.
- Decoding: On the server-side, decode the parameters using appropriate methods to retrieve the original array structure.
Best Practices
When constructing query parameters for arrays, consider the following best practices:
- Limit Array Size: Keep the number of items in an array reasonable to avoid exceeding URL length limits.
- Use Clear Naming Conventions: Ensure that parameter names are descriptive to enhance readability and maintainability.
- Consider Security: Validate and sanitize all incoming query parameters to prevent injection attacks and ensure data integrity.
By adhering to these methods and practices, you can effectively pass arrays as query parameters in a variety of programming environments, facilitating seamless data transmission between clients and servers.
Expert Insights on Passing Arrays as Query Parameters
Dr. Emily Chen (Senior Software Engineer, Tech Innovations Inc.). “When passing an array as a query parameter, it is essential to serialize the array correctly. Common methods include using comma-separated values or repeating the parameter name for each array element, ensuring that the server can interpret the data accurately.”
Michael Thompson (Web Development Consultant, CodeCraft Solutions). “Utilizing libraries such as Axios or jQuery can simplify the process of passing arrays in query parameters. These tools often handle serialization automatically, allowing developers to focus on other aspects of their applications.”
Jessica Patel (API Integration Specialist, DataConnect Corp.). “It is crucial to consider the limitations of URL length when passing large arrays. In such cases, it may be more efficient to send the data in the request body instead of as query parameters, especially when working with RESTful APIs.”
Frequently Asked Questions (FAQs)
How do I pass an array as a query parameter in a URL?
You can pass an array as a query parameter by repeating the parameter name for each value. For example, `?items=value1&items=value2&items=value3`.
What is the format for passing an array in a GET request?
In a GET request, the array can be represented as `?array[]=value1&array[]=value2&array[]=value3` or `?array=value1,value2,value3`, depending on the server’s expected format.
Are there any limitations to passing arrays in query parameters?
Yes, URL length limitations may restrict the amount of data you can send. Additionally, some servers may have restrictions on the number of parameters.
How do I handle array parameters in a server-side application?
Most server-side frameworks automatically parse repeated query parameters into an array. For example, in PHP, you can access them via `$_GET[‘items’]`.
Can I pass complex objects as query parameters?
While you can encode complex objects as JSON strings, it is generally not recommended due to URL length and readability issues. Instead, consider using POST requests for complex data.
What are the best practices for passing arrays in query parameters?
Use clear and concise parameter names, avoid excessive nesting, and ensure the URL remains readable. Additionally, consider using URL encoding for special characters.
Passing an array as a query parameter is a common requirement in web development, particularly when dealing with APIs or web applications that need to send multiple values for a single parameter. The general approach involves encoding the array elements in a way that they can be easily parsed on the server side. This is typically done by using a specific format, such as repeating the parameter name for each value or using a comma-separated list. Understanding the appropriate encoding method is essential for ensuring that the data is transmitted correctly and can be interpreted accurately by the receiving system.
When constructing query parameters for arrays, developers must consider the conventions of the web framework or programming language they are using. For example, in PHP, you can pass an array using the syntax `param[]=value1¶m[]=value2`, while in JavaScript, you might use `param=value1,value2`. Additionally, many frameworks provide built-in methods to handle this encoding, which can simplify the process and reduce the likelihood of errors. It is also important to be aware of URL length limitations, as excessively long query strings can lead to issues in some environments.
In summary, effectively passing an array as a query parameter requires a clear understanding of the encoding techniques and the specific requirements of the technology
Author Profile
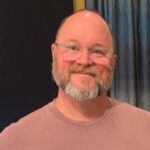
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?