Why Am I Getting Multiple Values for Argument Errors in My Code?
In the world of programming, encountering errors is an inevitable part of the development process. Among these, the phrase “Got multiple values for argument” often pops up, leaving many developers puzzled and frustrated. This common error message can be a significant roadblock, especially for those who are new to coding or working with complex functions. Understanding the underlying causes of this error is crucial for troubleshooting and refining your code, enabling you to enhance your programming skills and streamline your workflow.
This article delves into the intricacies of the “Got multiple values for argument” error, shedding light on its origins and implications. We’ll explore how this error arises in various programming languages, particularly in Python, and the common scenarios that lead to its occurrence. By dissecting the nuances of function arguments and the importance of proper parameter management, we aim to equip you with the knowledge necessary to avoid this pitfall in your coding journey.
As we navigate through the potential causes and solutions, you’ll gain insights into best practices for defining and calling functions effectively. Whether you’re debugging a simple script or tackling a more complex application, understanding this error will empower you to write cleaner, more efficient code. Join us as we unravel the mystery behind “Got multiple values for argument” and turn a frustrating experience into an
Understanding the Error
The “Got Multiple Values For Argument” error occurs in Python when a function receives more arguments than it is designed to handle. This situation typically arises due to a conflict between positional and keyword arguments. Understanding this error is crucial for debugging and writing more robust code.
When a function is defined, it can accept parameters in two ways:
- Positional Arguments: These are the most common type and are assigned based on their position in the function call.
- Keyword Arguments: These are specified by name and can be provided in any order.
The error manifests when a function call inadvertently passes the same argument multiple times, either by position and keyword or by naming the same keyword argument more than once.
Common Scenarios Leading to the Error
Several scenarios can lead to this error. Here are some typical examples:
- Passing the Same Argument Twice: When a function is called with a positional argument that is also provided as a keyword argument.
“`python
def example_function(param1, param2):
return param1 + param2
Error: Got multiple values for argument ‘param1’
example_function(5, param1=10)
“`
- Incorrect Function Definition: A function may be defined with a certain number of parameters, and the call may mistakenly include additional arguments.
“`python
def add_numbers(a, b):
return a + b
Error: Got multiple values for argument ‘b’
add_numbers(1, 2, b=3)
“`
- Using Unpacking with Conflicting Keys: When unpacking dictionaries into function calls, if the keys conflict with existing positional arguments.
“`python
def greet(name, greeting):
return f”{greeting}, {name}!”
data = {‘name’: ‘Alice’, ‘greeting’: ‘Hello’, ‘greeting’: ‘Hi’}
Error: Got multiple values for argument ‘greeting’
greet(**data)
“`
Best Practices to Avoid the Error
To mitigate the chances of encountering this error, consider the following best practices:
- Be Clear About Function Signatures: Clearly define the parameters of your functions to avoid confusion.
- Use Keyword Arguments Judiciously: When calling functions, especially with many parameters, prefer using keyword arguments for clarity.
- Check for Duplicates in Unpacking: When unpacking dictionaries, ensure that keys do not overlap with the positional arguments already passed.
Here’s a simple table summarizing the differences between positional and keyword arguments:
Argument Type | Usage | Order Sensitivity |
---|---|---|
Positional | Passed by their position in the function call | Order matters |
Keyword | Passed using the parameter name | Order does not matter |
By adhering to these practices, developers can significantly reduce the likelihood of encountering the “Got Multiple Values For Argument” error and enhance the maintainability of their code.
Understanding the Error
The error message “Got multiple values for argument” typically arises in Python when a function receives more positional or keyword arguments than it expects. This can lead to confusion, especially in complex function calls or when using variable-length arguments.
Key reasons for this error include:
- Duplicate Keyword Arguments: When you pass the same keyword argument multiple times.
- Positional and Keyword Conflict: A positional argument is passed that conflicts with a keyword argument.
- Unpacking Errors: Using the `*` operator incorrectly while unpacking arguments from a dictionary.
Common Scenarios
- Duplicate Keyword Arguments:
“`python
def func(arg1, arg2):
pass
func(arg1=1, arg2=2, arg2=3) Raises TypeError
“`
- Conflicting Argument Types:
“`python
def func(arg1, arg2):
pass
func(1, arg2=2) Works fine
func(1, 2, arg2=3) Raises TypeError
“`
- Using Unpacking Incorrectly:
“`python
def func(arg1, arg2):
pass
my_args = {‘arg1’: 1, ‘arg2’: 2, ‘arg2’: 3}
func(**my_args) Raises TypeError
“`
Debugging Steps
To resolve this error effectively, follow these debugging steps:
- Review Function Definition: Check the function signature to confirm the expected parameters.
- Inspect Argument Passing: Look for instances where arguments are passed multiple times or in conflicting formats.
- Use Print Statements: Add print statements or use debugging tools to trace the values being passed to the function.
- Check Variable Unpacking: When unpacking dictionaries or lists, ensure that no keys or indices are duplicated.
Best Practices to Avoid Errors
Implement the following best practices to prevent encountering this error in your code:
- Use Clear Naming Conventions: Ensure that parameter names are distinct and meaningful.
- Limit Keyword Arguments: When possible, reduce the number of keyword arguments to minimize confusion.
- Adopt Consistent Argument Structures: Stick to a consistent pattern of positional and keyword arguments across functions.
- Leverage Type Hints: Utilize Python’s type hints to clarify expected argument types and improve code readability.
Examples of Correct Usage
Here are examples demonstrating correct usage to avoid the “Got multiple values for argument” error:
“`python
def func(arg1, arg2, arg3=0):
return arg1 + arg2 + arg3
Correct calls
result1 = func(1, 2)
result2 = func(1, 2, arg3=3)
result3 = func(arg1=1, arg2=2, arg3=3)
“`
Tools and Resources
Utilize the following tools and resources for better understanding and debugging:
Tool/Resource | Description |
---|---|
Python Documentation | Official documentation provides comprehensive details on functions and argument handling. |
Linters (e.g., Pylint) | Static code analysis tools that can help catch errors before runtime. |
Debugging Tools (e.g., pdb) | Built-in debugger for step-by-step execution and inspection of variables. |
By adhering to these guidelines, developers can significantly reduce the incidence of the “Got multiple values for argument” error in their Python projects.
Understanding the “Got Multiple Values For Argument” Error in Programming
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The ‘Got Multiple Values For Argument’ error typically arises in Python when a function receives more arguments than it expects. This often occurs due to incorrect parameter passing, such as using both positional and keyword arguments simultaneously. Developers should ensure that their function calls align with the defined parameters to avoid this issue.”
James Liu (Lead Developer, CodeCraft Solutions). “In many programming languages, including Python, this error serves as a reminder to maintain clarity in function signatures. It is crucial to adhere to the expected input types and formats. Using default values for parameters can also help mitigate this error by providing flexibility in argument passing.”
Sarah Thompson (Technical Trainer, DevMaster Academy). “Understanding the context of this error is vital for new programmers. It underscores the importance of reading function documentation carefully. When you encounter ‘Got Multiple Values For Argument’, take a moment to review your function calls and ensure that you are not inadvertently providing conflicting arguments.”
Frequently Asked Questions (FAQs)
What does the error “Got Multiple Values For Argument” mean?
This error indicates that a function or method has received more than one value for a single argument, which is not allowed. This typically occurs when positional and keyword arguments are mixed incorrectly.
How can I resolve the “Got Multiple Values For Argument” error?
To resolve this error, review the function call to ensure that each argument is provided only once. Check for any positional arguments that may conflict with keyword arguments.
What are common scenarios that trigger this error?
Common scenarios include passing the same argument both positionally and as a keyword, or when a function is called with an incorrect number of arguments that overlap in naming.
Can this error occur in any programming language?
Yes, while the phrasing may differ, many programming languages that support functions or methods can produce a similar error when conflicting arguments are provided.
Is there a way to prevent this error in my code?
To prevent this error, use clear and consistent argument naming conventions, and prefer using keyword arguments when the function accepts both positional and keyword arguments.
What tools can help identify this error in my code?
Most integrated development environments (IDEs) and code editors offer linting tools that can help identify potential argument conflicts before runtime, allowing for early error detection.
The phrase “Got Multiple Values For Argument” often arises in programming and software development contexts, particularly when dealing with functions or methods that expect a single value for a parameter but receive more than one. This situation can lead to errors or unexpected behavior in code execution. Understanding the underlying causes of this issue is crucial for developers to troubleshoot effectively and ensure that their code functions as intended.
One common scenario that leads to this error is the misuse of data structures, such as lists or arrays, when passing arguments to functions. Developers may inadvertently pass an entire collection instead of a single item, resulting in the function receiving multiple values. Additionally, this issue can occur when default parameters are set in a way that conflicts with the intended input, causing ambiguity in how arguments are interpreted. Addressing these concerns requires careful attention to the function signatures and the data being passed.
To mitigate the occurrence of “Got Multiple Values For Argument” errors, developers should adopt best practices such as validating input types and ensuring that function calls align with their expected parameters. Implementing thorough testing and debugging strategies can also help identify and resolve these issues before they manifest in production environments. By fostering a clear understanding of function parameters and argument handling, developers can enhance the reliability and maintainability of
Author Profile
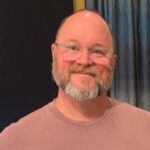
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?