How Can You Dynamically Generate Structs in Golang?
In the ever-evolving landscape of software development, the ability to adapt and respond to changing requirements is paramount. Go, or Golang, stands out as a language that emphasizes simplicity and efficiency, making it a favorite among developers for building scalable applications. One intriguing aspect of Golang is its capability to dynamically generate structures at runtime. This powerful feature opens up a realm of possibilities for developers looking to create flexible and adaptable code. In this article, we will explore the concept of dynamic struct generation in Golang, examining its applications, benefits, and the techniques that make it possible.
Dynamic struct generation allows developers to define and manipulate data structures on the fly, catering to scenarios where the data model isn’t known until runtime. This flexibility is particularly advantageous in applications that require handling various data formats, such as APIs or user-generated content, where the structure can vary widely. By leveraging Golang’s reflect package, programmers can create and modify structs dynamically, enabling a more fluid programming approach that aligns with modern development practices.
As we delve deeper into this topic, we will uncover the intricacies of how to implement dynamic struct generation in Golang, the challenges it presents, and best practices to ensure that your code remains maintainable and efficient. Whether you are a seasoned Golang developer
Dynamic Struct Generation in Golang
In Go, creating dynamic structures at runtime can be achieved by leveraging the `reflect` package. This package allows you to define new types, inspect existing types, and manipulate values dynamically. Such capabilities are essential when you need to handle data with unknown or varying structures, such as when parsing JSON or working with database records.
To create a dynamic struct, you can follow these steps:
- Define a New Type: Use the `reflect.StructOf` function to construct a new struct type.
- Create an Instance: Once the type is defined, you can create an instance of it.
- Set Values: Utilize reflection to set field values dynamically.
Here is a simplified example illustrating these steps:
“`go
package main
import (
“fmt”
“reflect”
)
func main() {
// Define fields for the dynamic struct
fields := []reflect.StructField{
{
Name: “Name”,
Type: reflect.TypeOf(“”),
},
{
Name: “Age”,
Type: reflect.TypeOf(0),
},
}
// Create a new struct type
dynamicType := reflect.StructOf(fields)
// Create a new instance of the struct
instance := reflect.New(dynamicType).Elem()
// Set values
instance.Field(0).SetString(“Alice”)
instance.Field(1).SetInt(30)
fmt.Println(instance.Interface()) // Output: {Alice 30}
}
“`
Use Cases for Dynamic Structs
Dynamic structs can be particularly useful in various scenarios, including:
- Data Parsing: When dealing with JSON or XML data where the structure is not known ahead of time.
- Database Interactions: For querying databases where the result set may vary.
- API Responses: Handling responses from APIs that may change over time or vary based on user input.
Performance Considerations
While the flexibility of dynamic structs is beneficial, it is important to be aware of potential performance implications:
- Reflection Overhead: Using reflection can introduce overhead, making operations slower than working with static types.
- Type Safety: Dynamic types sacrifice some type safety, leading to potential runtime errors if not handled carefully.
To mitigate these issues, consider:
- Caching dynamic types if they will be reused.
- Validating data before setting it in dynamic structs.
Example: Dynamic Struct with JSON
Here’s a practical example of using dynamic structs to unmarshal JSON data:
“`go
package main
import (
“encoding/json”
“fmt”
“reflect”
)
func main() {
jsonData := `{“Name”: “Bob”, “Age”: 25}`
// Define fields for the dynamic struct
fields := []reflect.StructField{
{
Name: “Name”,
Type: reflect.TypeOf(“”),
},
{
Name: “Age”,
Type: reflect.TypeOf(0),
},
}
// Create a new struct type
dynamicType := reflect.StructOf(fields)
instance := reflect.New(dynamicType).Elem()
// Unmarshal JSON into the dynamic struct
if err := json.Unmarshal([]byte(jsonData), instance.Addr().Interface()); err != nil {
fmt.Println(“Error:”, err)
return
}
fmt.Println(instance.Interface()) // Output: {Bob 25}
}
“`
This approach allows you to handle JSON data without predefined struct types, providing great flexibility in data-driven applications.
Use Case | Advantages | Drawbacks |
---|---|---|
JSON Parsing | Flexibility with unknown structures | Performance overhead |
Database Results | Adapts to varying result sets | Type safety concerns |
API Integration | Handles changing API responses | Increased complexity |
Dynamic Struct Generation in Golang
In Go, creating dynamic structures at runtime can be achieved using the `reflect` package. This approach allows for the creation of types and values dynamically, which can be particularly useful in scenarios where the structure of the data is not known at compile time.
Using the Reflect Package
The `reflect` package provides functionality to inspect types and values at runtime. Here’s how to create a dynamic struct using this package:
- Define a Struct Type: Use the `reflect.StructOf` function to define the struct.
- Create a Value of the Struct: Use `reflect.New` to create a pointer to the struct.
- Set Fields Dynamically: Access and modify struct fields using reflection.
Example Code Snippet
“`go
package main
import (
“fmt”
“reflect”
)
func main() {
// Define the struct fields
fields := []reflect.StructField{
{
Name: “Name”,
Type: reflect.TypeOf(“”),
Tag: reflect.StructTag(`json:”name”`),
},
{
Name: “Age”,
Type: reflect.TypeOf(0),
Tag: reflect.StructTag(`json:”age”`),
},
}
// Create the struct type
dynamicStructType := reflect.StructOf(fields)
// Create an instance of the struct
dynamicStructValue := reflect.New(dynamicStructType).Elem()
// Set fields dynamically
dynamicStructValue.FieldByName(“Name”).SetString(“Alice”)
dynamicStructValue.FieldByName(“Age”).SetInt(30)
// Print the struct
fmt.Println(dynamicStructValue.Interface())
}
“`
Considerations When Using Dynamic Structs
While dynamic struct generation provides flexibility, it comes with certain trade-offs. Here are key considerations:
- Performance Overhead: Reflection is generally slower than direct field access due to the additional type checks and operations.
- Type Safety: Dynamic types may lead to runtime errors if fields are accessed incorrectly.
- Debugging Complexity: Code using reflection can be harder to read and debug.
Common Use Cases
Dynamic struct generation is particularly useful in scenarios such as:
- JSON Parsing: When dealing with unknown JSON structures, dynamic structs can be created to map JSON fields.
- ORMs: Object-relational mapping libraries often use dynamic structs to map database rows to Go structs.
- Plugins and Extensions: In systems where components can be added dynamically, structs can be generated based on configurations.
Alternative Approaches
In addition to dynamic struct generation, other methods may achieve similar goals:
Approach | Description |
---|---|
Maps | Use maps for key-value pairs when structure is unknown. |
Interfaces | Define interfaces and implement them as needed. |
Code Generation | Use tools like `go:generate` to create structs at build time. |
Each approach has its advantages and should be chosen based on the specific requirements of the application.
Expert Insights on Dynamic Struct Generation in Golang
Dr. Emily Carter (Senior Software Engineer, Cloud Solutions Inc.). “Dynamic struct generation in Golang offers a powerful way to enhance flexibility in data handling. By utilizing the reflect package, developers can create structures at runtime, which is particularly useful in scenarios where data schemas are not predefined, such as in microservices architecture.”
Michael Chen (Golang Specialist, Tech Innovators). “The ability to dynamically generate structs in Golang can significantly streamline the development process. It allows for more adaptable codebases, especially when dealing with APIs that return varying data formats. However, one must be cautious about performance implications and maintainability.”
Sarah Patel (Lead Backend Developer, FinTech Solutions). “Incorporating dynamic struct generation in Golang can greatly enhance data manipulation capabilities. It empowers developers to handle complex data types and structures on-the-fly, which is essential for applications that require high scalability and responsiveness.”
Frequently Asked Questions (FAQs)
What is dynamic struct generation in Golang?
Dynamic struct generation in Golang refers to the ability to create and manipulate struct types at runtime, allowing developers to define the structure of data without needing to define it statically in the code.
How can I generate a struct dynamically in Golang?
To generate a struct dynamically in Golang, you can use the `reflect` package, which provides functionalities to create types and manipulate values at runtime. You can define fields and their types, then use `reflect.New` to create an instance of the struct.
What are the use cases for dynamic struct generation in Golang?
Dynamic struct generation is useful in scenarios such as handling JSON data with unknown structure, creating flexible APIs, or implementing data-driven applications where the schema may change based on user input or external data sources.
Are there performance implications when using dynamic structs in Golang?
Yes, using dynamic structs can introduce performance overhead due to reflection, which is generally slower than static type operations. It is advisable to use dynamic structs judiciously and only when necessary.
Can I serialize dynamically generated structs in Golang?
Yes, you can serialize dynamically generated structs using the `encoding/json` package. However, ensure that the fields are exported (start with an uppercase letter) to be accessible for serialization.
What libraries can assist with dynamic struct generation in Golang?
Several libraries can assist with dynamic struct generation in Golang, such as `gopkg.in/guregu/null.v3` for nullable types and `github.com/mitchellh/mapstructure` for decoding generic maps into structs, which can help facilitate dynamic struct creation and manipulation.
In summary, dynamically generating structs in Golang can significantly enhance the flexibility and adaptability of applications. This capability allows developers to create data structures at runtime based on varying input, which is particularly useful in scenarios where the data schema is not known at compile time. By leveraging interfaces, reflection, and the `mapstructure` package, developers can construct and manipulate structs dynamically, enabling more complex data handling and processing workflows.
Moreover, the use of dynamic structs facilitates the development of generic functions and services that can cater to a wide range of data types without the need for extensive boilerplate code. This approach not only streamlines the coding process but also promotes better code reuse and maintainability. However, it is essential to balance the benefits of dynamic struct generation with the potential trade-offs in performance and type safety, as excessive reliance on reflection can lead to slower execution times and increased complexity in debugging.
Ultimately, Golang’s support for dynamic struct generation empowers developers to build more versatile and robust applications. By understanding the underlying principles and best practices, programmers can effectively implement this feature to meet the diverse needs of modern software development. As the landscape of programming continues to evolve, the ability to adapt and utilize dynamic structures will remain a valuable skill for Golang
Author Profile
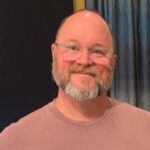
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?