How Can You Git Clone Into an Existing Directory Without Overwriting Files?
In the world of software development, version control systems like Git have become indispensable tools for managing code and collaborating with teams. One of the most common tasks developers encounter is cloning repositories, which allows them to create a local copy of a project. However, there are times when you might want to clone a Git repository into an existing directory rather than creating a new one. This seemingly simple task can raise questions about best practices, potential pitfalls, and the nuances of directory management. In this article, we will explore the intricacies of cloning a Git repository into an existing directory, providing you with the insights you need to navigate this process smoothly.
When you clone a repository, Git typically creates a new directory to house the project files. But what if you already have a directory that you want to populate with the contents of a repository? This scenario often arises when you want to integrate a project into an existing structure or when you’re working on a project that has already been partially set up. Understanding how to do this correctly is essential to avoid overwriting files or ending up with a disorganized workspace.
Throughout this article, we will delve into the techniques and commands necessary to clone a Git repository into an existing directory. We will also discuss the implications of this approach, such as
Understanding the Git Clone Command
The `git clone` command is a fundamental part of using Git, allowing users to create a copy of a remote repository on their local machine. However, when users want to clone a repository into an existing directory that already contains files, it requires a specific approach. It is important to understand how to properly execute this to avoid conflicts or data loss.
When cloning into an existing directory, the following conditions must be met:
- The target directory must be empty, or it must contain only files that are not tracked by Git.
- If the directory contains files that are tracked by Git, the cloning operation will fail.
To clone into an existing directory while ensuring the integrity of your files, follow these guidelines.
Steps to Clone Into an Existing Directory
- Navigate to the Existing Directory: Use the command line to move into the directory where you want to clone the repository.
“`bash
cd path/to/existing/directory
“`
- Initialize Git in the Directory: If the directory is not already a Git repository, initialize it.
“`bash
git init
“`
- Add the Remote Repository: Link the local directory to the remote repository.
“`bash
git remote add origin
“`
- Fetch the Remote Repository: This step retrieves the data from the remote repository without merging it with the current files.
“`bash
git fetch origin
“`
- Checkout the Desired Branch: After fetching, you can check out the branch you want to work with.
“`bash
git checkout -b
“`
This process allows you to clone the repository while maintaining control over your existing files.
Handling Existing Files
If your existing directory contains files, it’s crucial to manage these files before cloning. Here are options you might consider:
- Backup Existing Files: Before proceeding, back up any important files to prevent accidental loss.
- Move Untracked Files: If there are files that do not need to be in the repository, consider moving them to another directory.
The following table summarizes the steps and considerations when cloning into an existing directory:
Step | Action | Considerations |
---|---|---|
1 | Navigate to Directory | Ensure you’re in the correct path |
2 | Initialize Git | Only if the directory is not already a Git repo |
3 | Add Remote Repository | Check the repository URL for accuracy |
4 | Fetch Remote Data | Review fetched data before proceeding |
5 | Checkout Branch | Ensure the branch exists in the remote repo |
By carefully following these steps, users can efficiently clone a Git repository into an existing directory while ensuring that their current files remain unaffected.
Understanding Git Clone Behavior
When using Git to clone a repository, the default behavior is to create a new directory for the cloned repository. However, there are scenarios where you may want to clone a repository into an already existing directory. Understanding how Git handles such situations is crucial for efficient version control management.
- Cloning into a non-empty directory is not directly supported by Git.
- If you attempt to do so, Git will return an error indicating that the directory is not empty.
Preparing an Existing Directory for Git Clone
To successfully clone a repository into an existing directory, follow these preparatory steps:
- Create the Directory: Ensure the directory exists where you want to clone the repository.
“`bash
mkdir existing-directory
cd existing-directory
“`
- Initialize the Directory: If the directory is intended to be a Git repository, initialize it.
“`bash
git init
“`
- Add a Remote: Link the remote repository to your local directory.
“`bash
git remote add origin
“`
- Fetch the Repository: Retrieve the contents from the remote repository without merging.
“`bash
git fetch origin
“`
- Checkout the Desired Branch: Switch to the branch you want to work on.
“`bash
git checkout -b
“`
This sequence allows you to effectively pull the content into an existing directory.
Using Sparse Checkout for Selective Cloning
If the existing directory contains files that may conflict with the repository you are cloning, consider using sparse checkout. This method allows you to selectively check out files from the repository.
- Enable Sparse Checkout:
“`bash
git config core.sparseCheckout true
“`
- Define Sparse Patterns: Specify which files or directories to include by editing the `.git/info/sparse-checkout` file.
“`
/path/to/include/*
“`
- Clone the Repository:
“`bash
git clone –no-checkout
“`
- Checkout the Desired Files:
“`bash
git checkout
“`
This way, only the specified files will be pulled into your existing directory, avoiding conflicts with existing files.
Common Pitfalls and Considerations
When cloning into an existing directory, several considerations and potential pitfalls should be kept in mind:
Issue | Description |
---|---|
Non-empty directory error | Git will refuse to clone into a non-empty directory without initialization. |
Existing files conflict | Files already present may conflict with those being cloned. |
Branch mismatches | Ensure the branch you want to check out exists in the remote repository. |
- Always back up important files before proceeding with cloning into an existing directory.
- Verify the branch names and remote URLs to avoid errors during the fetch and checkout processes.
Following these guidelines will help you effectively clone a Git repository into an existing directory while mitigating common issues.
Expert Insights on Cloning Git Repositories into Existing Directories
Dr. Emily Carter (Senior Software Engineer, Code Innovations Inc.). “Cloning a Git repository into an existing directory is a nuanced process that requires careful consideration of the current directory’s state. It is essential to ensure that the directory is either empty or contains files that will not conflict with the repository’s structure, as this can lead to unexpected behavior or data loss.”
Michael Chen (DevOps Specialist, Agile Solutions Group). “When performing a Git clone into an existing directory, it is crucial to utilize the ‘–no-checkout’ option if you want to avoid overwriting local files. This allows developers to manage their existing files while still integrating the repository’s structure. Proper version control practices are key to maintaining a clean workflow.”
Sarah Thompson (Open Source Contributor and Git Educator). “Many developers overlook the importance of understanding the implications of cloning into an existing directory. It is advisable to first check the status of the current directory and ensure that any untracked files are either committed or stashed. This practice minimizes the risk of merge conflicts and ensures a smoother integration of the cloned repository.”
Frequently Asked Questions (FAQs)
Can I use Git clone to copy a repository into an existing directory?
Yes, you can use Git clone to copy a repository into an existing directory, but the directory must be empty. If the directory contains files, the clone operation will fail.
How do I clone a Git repository into a specific existing directory?
To clone a repository into a specific existing directory, navigate to that directory in your terminal and run the command `git clone
What happens if the existing directory is not empty when I try to clone?
If the existing directory is not empty, Git will return an error message indicating that the destination path already exists and is not an empty directory. You must either choose an empty directory or remove the existing files.
Is it possible to clone a repository and merge it with existing files in a directory?
Cloning a repository directly into a directory with existing files is not supported. However, you can clone the repository into a new directory and then manually merge the contents as needed.
Are there any specific flags or options I can use with Git clone for existing directories?
There are no specific flags for cloning into an existing directory. The standard practice is to ensure the directory is empty or to clone into a new directory and manage the files afterward.
What should I do if I need to keep existing files while cloning a repository?
If you need to keep existing files, clone the repository into a separate directory and then manually copy or merge the necessary files from the cloned repository into your existing directory.
In summary, cloning a Git repository into an existing directory is a task that requires careful consideration of the current contents of that directory. The standard `git clone` command is designed to create a new directory for the repository, but with the right approach, it is possible to clone into an existing folder. This process involves initializing a new Git repository in the target directory and then pulling the desired content from the remote repository.
One of the key insights is that when cloning into an existing directory, users must ensure that the directory is either empty or does not contain files that would conflict with the files being cloned. This is crucial to avoid overwriting or merging issues that could arise from existing files. Additionally, using commands like `git remote add` and `git pull` can facilitate the process of incorporating the repository’s content into the existing structure.
Another important takeaway is the need for proper version control practices. Users should be aware of the implications of merging histories and the potential for conflicts when integrating a remote repository into a local setup. Understanding the foundational commands and their effects on the repository’s state is vital for maintaining a clean and manageable project structure.
Ultimately, cloning into an existing directory can be a powerful technique for developers who
Author Profile
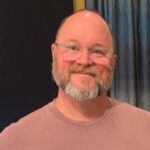
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?