How Can You Retrieve Product Attributes in Catalog-Add-To-Cart.js in Magento 2?
In the ever-evolving world of e-commerce, Magento 2 stands out as a powerful platform that empowers businesses to create highly customizable online stores. One of the critical aspects of enhancing user experience and driving sales is the effective management of product attributes. Among the various components that contribute to this functionality, the `catalog-add-to-cart.js` file plays a pivotal role. This JavaScript file is not just a backend utility; it serves as a bridge between the user interface and the product data, ensuring that customers have all the necessary information at their fingertips when making purchasing decisions.
Understanding how to get product attributes within the `catalog-add-to-cart.js` file is essential for developers looking to optimize the shopping experience. This file is responsible for handling the complexities of adding products to the cart, including the dynamic retrieval of attributes that can influence a customer’s choice. By tapping into the right attributes, businesses can tailor their offerings, showcase essential product details, and ultimately enhance customer satisfaction.
As we delve deeper into this topic, we will explore the intricacies of accessing product attributes in Magento 2, the significance of the `catalog-add-to-cart.js` file, and how these elements work together to create a seamless shopping experience. Whether you’re a seasoned developer or a newcomer to Magento, understanding this
Understanding Product Attributes in Magento 2
In Magento 2, product attributes are essential for defining the characteristics of products. These attributes can influence the shopping experience, helping customers make informed decisions. When integrating product attributes into the `Add-To-Cart.js` file, it is crucial to understand how to retrieve and utilize these attributes effectively.
Product attributes can be classified into several types, including:
- Text Fields: Simple text-based attributes, such as product names or descriptions.
- Dropdowns: Attributes that allow selection from predefined options, such as color or size.
- Swatches: Visual representations of attributes, commonly used for colors or patterns.
- Multiselect: Attributes allowing multiple selections, such as tags or features.
These attributes are defined in the Magento admin panel and can be accessed programmatically in JavaScript files, such as `Add-To-Cart.js`.
Accessing Product Attributes in Add-To-Cart.js
To access product attributes within the `Add-To-Cart.js`, you typically utilize the product object available in the JavaScript context. The product object contains various properties, including the attributes defined in the Magento backend.
Here’s how to extract product attributes:
- **Load the Product Data**: Ensure that the product data is loaded correctly before attempting to access its attributes. This is typically done through AJAX calls or data preloaded in the page context.
- **Retrieve Attributes**: Use the product object to access specific attributes. Here’s an example of how to retrieve a product attribute:
“`javascript
var productData = /* Load your product data */;
var productAttribute = productData.custom_attributes.find(attr => attr.attribute_code === ‘your_attribute_code’);
console.log(productAttribute.value);
“`
- Handle Different Attribute Types: Depending on the attribute type, you may need to format the display of the attribute differently. For instance, swatches may require additional markup to show colors visually.
Example of Attribute Retrieval
Here’s a practical example showcasing how to retrieve different product attributes in `Add-To-Cart.js`:
“`javascript
require([‘jquery’, ‘mage/storage’], function($, storage) {
var productSku = ‘your-product-sku’;
storage.get(‘V1/products/’ + productSku).done(function(productData) {
var attributes = productData.custom_attributes;
attributes.forEach(function(attribute) {
console.log(attribute.attribute_code + ‘: ‘ + attribute.value);
});
});
});
“`
In this example, we fetch product data using the product SKU and log all custom attributes to the console.
Table of Common Product Attributes
Attribute Code | Attribute Type | Description |
---|---|---|
color | Dropdown | The color of the product. |
size | Dropdown | The size options available for the product. |
material | Text | Material the product is made from. |
features | Multiselect | List of features available for the product. |
Understanding how to effectively access and manipulate product attributes in Magento 2’s `Add-To-Cart.js` is vital for enhancing the user experience, ensuring that customers have all the information they need to make a purchase.
Understanding the Catalog Add to Cart Functionality
The `catalog-add-to-cart.js` file in Magento 2 plays a critical role in managing the add-to-cart functionality for products in the catalog. This JavaScript module is responsible for handling user interactions when adding products to the cart, including managing product attributes. Understanding how this file operates can help developers customize and enhance the e-commerce experience.
Accessing Product Attributes
To effectively retrieve product attributes when a user adds an item to the cart, developers must interact with the Magento 2 API. The following methods are typically employed:
- Using AJAX Calls: The JavaScript file makes asynchronous requests to the server to fetch product details, including attributes.
- Data Binding: Magento 2 uses Knockout.js for data binding, allowing the frontend to dynamically update based on user input.
Key Functions in `catalog-add-to-cart.js`
The `catalog-add-to-cart.js` file contains several key functions that facilitate product attribute retrieval:
- `addToCart` Method: This method is triggered when a user clicks the add-to-cart button. It gathers the necessary product data, including selected attributes.
- `getProductData` Method: This function fetches the current product’s data, including attributes like size, color, and custom options.
Example of Retrieving Product Attributes
The following example illustrates how to retrieve product attributes within the `addToCart` method:
“`javascript
define([
‘jquery’,
‘Magento_Catalog/js/model/product/add-to-cart’
], function ($, addToCart) {
‘use strict’;
return function (productId, selectedOptions) {
const productData = {
product: productId,
options: selectedOptions
};
addToCart(productData).done(function (response) {
// Handle successful add to cart
console.log(‘Product added:’, response);
}).fail(function (error) {
// Handle error
console.error(‘Error adding product:’, error);
});
};
});
“`
In this example, `selectedOptions` contains the product attributes that the user has chosen.
Handling Custom Options and Required Attributes
When dealing with custom options and required attributes, specific considerations must be accounted for:
- Validation: Ensure that all required attributes are selected before allowing the product to be added to the cart.
- User Feedback: Provide immediate feedback to users if they attempt to add a product without selecting necessary options.
Integrating with Magento 2 Backend
To ensure that the JavaScript functions correctly communicate with the backend, developers must:
- Configure Web API: Set up proper API endpoints to handle requests from the frontend.
- Handle Responses: Ensure that responses from the backend are appropriately managed, updating the UI as needed based on success or failure.
Customizing Product Attribute Retrieval
Developers can customize how product attributes are retrieved and displayed by:
- Extending JavaScript Components: Modify or extend existing components to add new features or alter the behavior of the default add-to-cart functionality.
- Using Plugins: Create plugins for the product repository to manipulate data before it is returned to the frontend.
Best Practices for Enhancing User Experience
To create a seamless add-to-cart experience, consider the following best practices:
- Asynchronous Loading: Use AJAX to load product attributes dynamically without page refresh.
- Clear Messaging: Ensure users receive clear messaging regarding required attributes.
- Testing Across Devices: Verify that the add-to-cart functionality works consistently across different devices and browsers.
By understanding the intricacies of `catalog-add-to-cart.js` and how to interact with product attributes, developers can create a more efficient and user-friendly shopping experience in Magento 2.
Expert Insights on Accessing Product Attributes in Magento 2’s Catalog Add-To-Cart.js
Jessica Lin (E-commerce Solutions Architect, TechCommerce Consulting). “Understanding how to retrieve product attributes in the Catalog Add-To-Cart.js file is crucial for enhancing user experience. Properly leveraging these attributes allows developers to create dynamic and personalized shopping experiences that can significantly boost conversion rates.”
Mark Thompson (Senior Magento Developer, Digital Innovations Inc.). “When working with Catalog Add-To-Cart.js in Magento 2, it is essential to ensure that the product attributes are correctly defined in the backend. This ensures that the front-end JavaScript can access the necessary data, which is vital for functionalities like custom pricing and product options.”
Linda Garcia (Magento Certified Trainer, E-commerce Academy). “Developers must pay close attention to how product attributes are structured in Magento 2. Utilizing the appropriate methods to fetch these attributes in the Add-To-Cart.js file can streamline the checkout process and enhance the overall functionality of the e-commerce site.”
Frequently Asked Questions (FAQs)
What is the purpose of the `Add-To-Cart.js` file in Magento 2?
The `Add-To-Cart.js` file in Magento 2 is responsible for handling the add-to-cart functionality on the frontend. It manages user interactions, updates the cart, and communicates with the backend to ensure products are added correctly.
How can I retrieve product attributes using `Add-To-Cart.js`?
To retrieve product attributes in `Add-To-Cart.js`, you can access the product data object that is typically passed to the script. Use the appropriate attribute keys to extract the desired values from the product object.
Can I customize the attributes fetched in `Add-To-Cart.js`?
Yes, you can customize the attributes by modifying the JavaScript file or by extending the functionality through a custom module. Ensure that you include the necessary attributes in the product data when rendering the product on the frontend.
What are common issues when getting product attributes in `Add-To-Cart.js`?
Common issues include missing attributes in the product data, incorrect attribute keys used in the script, or JavaScript errors that prevent the execution of the add-to-cart functionality. Debugging the console can help identify these issues.
Is it necessary to use `Add-To-Cart.js` for custom themes in Magento 2?
While it is not strictly necessary, using `Add-To-Cart.js` is recommended for maintaining consistency with Magento’s core functionality. Custom themes can override or extend this file to ensure proper integration with the cart system.
How can I test changes made to `Add-To-Cart.js`?
To test changes made to `Add-To-Cart.js`, clear the cache and refresh the page. Use browser developer tools to monitor network requests and console logs to verify that the add-to-cart functionality behaves as expected after modifications.
In Magento 2, the process of retrieving product attributes in the catalog’s Add-To-Cart JavaScript file is crucial for enhancing the user experience and ensuring that the shopping cart reflects the correct product information. The Add-To-Cart functionality is a pivotal part of the e-commerce workflow, and accessing product attributes allows developers to customize the shopping experience by displaying relevant information, such as size, color, and availability, directly within the catalog interface.
To effectively get product attributes in the catalog’s Add-To-Cart.js file, developers can utilize Magento’s built-in JavaScript components and APIs. This involves understanding how to interact with the product data structure and leveraging the Magento 2 framework’s capabilities to fetch and manipulate product attributes dynamically. By doing so, developers can create a more engaging and informative shopping experience, which can lead to increased customer satisfaction and potentially higher conversion rates.
In summary, mastering the retrieval of product attributes within the Add-To-Cart.js file is essential for Magento 2 developers. It not only enhances the functionality of the catalog but also contributes to a seamless user journey. By focusing on best practices and utilizing Magento’s robust architecture, developers can ensure that they are providing customers with the most relevant and timely product information during their
Author Profile
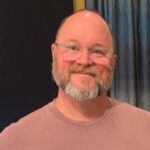
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?