How Can You Resolve the ‘Fatal Error: Allowed Memory Size’ Issue in Your Application?
In the world of web development and server management, encountering a “Fatal Error: Allowed Memory Size” message can be both frustrating and perplexing. This error often serves as a wake-up call, signaling that your application has exceeded the memory limits set by the server, leading to unexpected crashes and downtime. For developers and site administrators, understanding the intricacies of memory allocation is crucial not only for troubleshooting but also for optimizing performance and ensuring a seamless user experience. In this article, we will delve into the causes of this error, explore its implications, and provide practical solutions to help you navigate these challenges effectively.
Overview
The “Fatal Error: Allowed Memory Size” message typically arises in environments where memory usage is tightly regulated, such as PHP applications running on shared hosting platforms. When a script attempts to utilize more memory than what is allocated, the server halts its execution, resulting in a fatal error that can disrupt services and frustrate users. This issue is particularly common in resource-intensive applications, such as content management systems or e-commerce platforms, where the demand for memory can fluctuate significantly based on user interactions and data processing needs.
Understanding the root causes of this error is essential for effective resolution. Factors such as inefficient coding practices, excessive plugin use, or inadequate server
Understanding Memory Limits
Memory limits in PHP determine how much memory a script can consume during its execution. When a script exceeds this limit, it triggers a `Fatal Error: Allowed Memory Size`, leading to a termination of the script. This limit is set in the `php.ini` configuration file, where the `memory_limit` directive defines the maximum amount of memory a script can allocate.
- Common values for `memory_limit` include:
- `128M` (128 Megabytes)
- `256M` (256 Megabytes)
- `512M` (512 Megabytes)
- `-1` (no memory limit)
Adjusting the memory limit may be necessary for resource-intensive applications, such as those that process large datasets or handle complex calculations.
Identifying the Error
When encountering a `Fatal Error: Allowed Memory Size`, the error message typically provides specific details about the memory limit and the amount of memory consumed by the script. For instance, an error message may look like this:
“`
Fatal error: Allowed memory size of 134217728 bytes exhausted (tried to allocate 20480 bytes)
“`
In this example, the allowed memory size is `134217728 bytes` (or `128M`), and the script attempted to allocate an additional `20480 bytes` before failing.
How to Resolve Memory Limit Issues
There are several approaches to resolve this memory limit issue, depending on the environment and access level.
- Increasing the Memory Limit:
- Modify the `php.ini` file:
“`ini
memory_limit = 256M
“`
- Adjust via `.htaccess` file (if using Apache):
“`
php_value memory_limit 256M
“`
- Use `ini_set` function within the script:
“`php
ini_set(‘memory_limit’, ‘256M’);
“`
- Optimizing Code:
- Analyze the script for memory-intensive operations.
- Utilize efficient algorithms and data structures.
- Implement pagination for large data sets.
- Profiling Memory Usage:
- Use tools like Xdebug or Blackfire to profile and analyze memory usage. This helps identify memory leaks or excessive consumption.
Best Practices for Memory Management
To prevent encountering memory limit errors in the future, adopt the following best practices:
- Regularly Monitor Memory Usage: Track memory consumption through logs or monitoring tools to catch issues early.
- Optimize Database Queries: Use efficient queries and avoid loading unnecessary data into memory.
- Leverage Caching: Implement caching strategies to reduce the load on memory, especially for frequently accessed data.
- Limit Object Lifetimes: Dispose of objects that are no longer needed to free up memory resources.
Action | Description |
---|---|
Increase Memory Limit | Adjust settings in php.ini, .htaccess, or via code. |
Optimize Code | Refactor code to be more efficient and reduce memory use. |
Profile Memory Usage | Use tools to analyze and identify memory issues. |
Implement Caching | Store frequently accessed data to minimize memory load. |
Understanding the Error
The error message “Fatal Error: Allowed Memory Size” indicates that a script has attempted to use more memory than is allocated by the PHP configuration. This situation commonly arises in web applications, especially those with resource-intensive tasks such as large database queries or extensive file processing.
When PHP scripts exceed the memory limit, they fail to execute, resulting in a termination of the script. The default memory limit can vary depending on the server configuration, often set at 128MB or 256MB.
Common Causes
Several factors can contribute to encountering the allowed memory size error:
- Inefficient Code: Poorly optimized scripts can consume excessive memory.
- Large Data Processing: Handling large datasets without proper memory management can quickly exhaust available resources.
- Third-Party Plugins or Libraries: Certain plugins can be resource-heavy, especially in content management systems like WordPress.
- Memory Leaks: Bugs in code that fail to release allocated memory can lead to gradual increases in memory usage.
Configuring Memory Limits
To resolve the memory size error, you can adjust the memory limit in your PHP configuration. There are multiple methods to achieve this:
- php.ini File:
Locate the `php.ini` file and modify the `memory_limit` directive. For example:
“`
memory_limit = 256M
“`
- .htaccess File:
If you do not have access to the `php.ini` file, you can set the memory limit in the `.htaccess` file:
“`
php_value memory_limit 256M
“`
- Script-Level Configuration:
You can also set the memory limit within your script using:
“`
ini_set(‘memory_limit’, ‘256M’);
“`
- Web Hosting Control Panels:
Many hosting providers offer a user interface to change PHP settings directly. Consult your hosting documentation for specific instructions.
Monitoring Memory Usage
To effectively manage memory usage, monitoring tools can provide insights into how memory is being utilized by your scripts. Consider the following methods:
Tool/Method | Description |
---|---|
Xdebug | A powerful debugging tool that provides profiling information. |
PHP Memory Usage Functions | Use functions like `memory_get_usage()` to track memory consumption within scripts. |
Performance Monitoring Plugins | For applications like WordPress, plugins such as Query Monitor can help identify memory usage by components. |
Best Practices for Memory Management
To minimize the risk of exceeding memory limits, adhere to these best practices:
- Optimize Code: Regularly review and optimize your code to improve efficiency.
- Limit Data Queries: Fetch only necessary data rather than loading entire datasets.
- Use Pagination: Implement pagination for data-heavy applications to reduce memory footprint.
- Profile Your Applications: Regularly profile your applications to identify memory bottlenecks.
By understanding the implications of memory limits and proactively managing memory usage, you can significantly reduce the likelihood of encountering the “Fatal Error: Allowed Memory Size” issue in your applications.
Expert Insights on Managing Memory Limit Errors in PHP
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The ‘Fatal Error: Allowed Memory Size’ issue typically arises when a script exceeds the memory limit set in the PHP configuration. It is essential to monitor memory usage during development and optimize code to prevent such errors from occurring in production environments.”
Michael Tran (Lead PHP Developer, Web Solutions Group). “Increasing the memory limit in the php.ini file can provide a quick fix for the ‘Allowed Memory Size’ error. However, developers should prioritize identifying memory leaks and optimizing resource-intensive processes to ensure long-term stability and performance of their applications.”
Sarah Johnson (Technical Consultant, CodeCraft Consultancy). “When encountering the ‘Fatal Error: Allowed Memory Size’, it is vital to analyze the stack trace to understand which part of the code is consuming excessive memory. Implementing caching strategies and efficient data handling can significantly mitigate these issues and enhance application efficiency.”
Frequently Asked Questions (FAQs)
What does the error “Fatal Error: Allowed Memory Size” mean?
This error indicates that a PHP script has exceeded the memory limit set in the PHP configuration. It typically occurs when a script tries to allocate more memory than is permitted.
How can I increase the memory limit in PHP?
You can increase the memory limit by modifying the `php.ini` file, adding or updating the line `memory_limit = 256M` (or a higher value as needed). Alternatively, you can also use `ini_set(‘memory_limit’, ‘256M’);` in your script.
What are common causes of exceeding the allowed memory size?
Common causes include inefficient code, memory leaks, large data processing, and using resource-intensive libraries or frameworks that require more memory than allocated.
How can I troubleshoot memory limit issues in my PHP application?
To troubleshoot, review your code for inefficiencies, use profiling tools to identify memory usage, and consider optimizing data handling. Additionally, check for any infinite loops or excessive recursion.
Is it safe to keep increasing the memory limit indefinitely?
No, continuously increasing the memory limit is not a sustainable solution. It is essential to identify and resolve the underlying issues causing high memory usage to ensure optimal performance and stability.
What should I do if increasing the memory limit does not resolve the issue?
If increasing the memory limit does not resolve the issue, consider reviewing and optimizing your code, checking for external factors such as server limitations, or consulting with a developer for a more in-depth analysis.
The “Fatal Error: Allowed Memory Size” issue typically arises in programming environments, particularly in PHP, when a script attempts to use more memory than is allocated by the server’s configuration settings. This error indicates that the memory limit set in the PHP configuration file (php.ini) has been exceeded, which can halt script execution and disrupt application functionality. Understanding the root causes of this error is essential for developers to maintain optimal performance and prevent application crashes.
Common reasons for encountering this error include inefficient code, memory leaks, or handling large datasets without adequate memory management. Developers should regularly review and optimize their code to ensure that memory usage remains within the allocated limits. Additionally, it is advisable to monitor memory consumption during the execution of scripts to identify potential bottlenecks and address them proactively.
To resolve the “Allowed Memory Size” error, developers can either increase the memory limit in the php.ini file or implement more efficient coding practices. Increasing the memory limit can provide a temporary fix, but it is crucial to address the underlying issues to prevent recurrence. Ultimately, a combination of proper configuration and code optimization will lead to more stable and efficient applications.
Author Profile
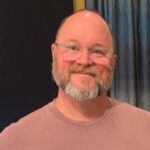
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?