Why Am I Seeing ‘Failed To Lazily Initialize A Collection Of Role’ in My Application?
In the realm of Java development, particularly when working with Hibernate and JPA, developers often encounter a perplexing error: “Failed to lazily initialize a collection of role.” This seemingly cryptic message can halt progress and lead to frustration, especially when it arises in the midst of a complex application. Understanding the underlying principles of lazy loading and the intricacies of entity relationships is crucial for any developer aiming to harness the full potential of these powerful frameworks. In this article, we will demystify this common issue, exploring its causes, implications, and effective strategies for resolution.
Overview
At its core, the “Failed to lazily initialize a collection of role” error typically surfaces when an application attempts to access a lazily-loaded collection outside of an active Hibernate session. This situation often arises in scenarios where developers assume that all necessary data is readily available, only to find that the collection in question has not been initialized. The intricacies of Hibernate’s lazy loading mechanism can lead to unexpected behavior if not properly understood and managed.
Moreover, this error highlights the importance of session management and the lifecycle of entities within a Hibernate context. By gaining insight into how Hibernate handles data retrieval and the implications of session boundaries, developers can better navigate the challenges posed by lazy initialization.
Understanding Lazy Initialization
Lazy initialization is a design pattern that postpones the creation of an object or the calculation of a value until the moment it is needed. This approach can enhance application performance and resource management, particularly in environments with heavy data operations such as ORM (Object-Relational Mapping) systems.
In the context of Hibernate (a popular ORM framework), lazy initialization refers to the mechanism whereby associated collections or entities are not loaded from the database until they are explicitly accessed. However, this can lead to the “Failed to lazily initialize a collection of role” exception if the session that originally fetched the entity is closed before the lazy-loaded collection is accessed.
Causes of the Exception
The “Failed to lazily initialize a collection of role” exception typically arises due to several reasons:
- Session Closure: When the Hibernate session is closed, any attempt to access lazily initialized collections will result in this exception.
- Detached Entities: If an entity is detached from the session, it cannot access its lazy-loaded collections.
- Improper Fetching Strategy: If the fetch strategy is not appropriately set, it may lead to unexpected behavior when accessing associated collections.
Resolving the Exception
To handle this exception, consider the following strategies:
- Open Session in View Pattern: Maintain the session open during the view rendering phase, allowing lazy loading to occur without issues.
- Eager Fetching: Modify the fetching strategy to eagerly load the necessary collections. This can be done through annotations or XML configuration.
- DTO Pattern: Use Data Transfer Objects (DTOs) to fetch only the required data, avoiding the need for lazy initialization.
Example of modifying fetch strategy using annotations:
“`java
@Entity
public class User {
@OneToMany(fetch = FetchType.EAGER)
private Set
}
“`
Best Practices for Lazy Loading
To effectively manage lazy initialization and avoid related exceptions, adhere to these best practices:
- Understand Entity Lifecycle: Be cognizant of the entity’s lifecycle and the associated session state.
- Keep Transactions Short: Ensure that transactions are kept short and do not span UI interactions to minimize the risk of closed sessions.
- Use Hibernate Tools: Utilize Hibernate tools to analyze the SQL being generated, ensuring that lazy loading behaves as expected.
Example Scenario
Consider a scenario where you have a `User` entity that has a collection of `Order` entities:
“`java
@Entity
public class User {
@Id
private Long id;
private String name;
@OneToMany(mappedBy = “user”)
private Set
}
“`
If you attempt to access `user.getOrders()` after the session has been closed, you will encounter the “Failed to lazily initialize a collection of role” exception.
To illustrate the impact of various fetch strategies, here is a comparison:
Fetch Type | Description | Advantages | Disadvantages |
---|---|---|---|
EAGER | Loads the collection immediately with the parent entity. | Prevents lazy loading issues. | May lead to performance overhead and unnecessary data retrieval. |
LAZY | Loads the collection only when accessed. | Improves performance by loading only necessary data. | Risk of exceptions if session is closed. |
By understanding these principles and implementing best practices, developers can effectively manage lazy loading in Hibernate, ensuring smooth application performance while minimizing the risk of exceptions.
Understanding the Error
The “Failed To Lazily Initialize A Collection Of Role” error typically occurs in Hibernate when an attempt is made to access a lazily-loaded collection outside of an active Hibernate session. This can lead to a `LazyInitializationException`, which indicates that the collection cannot be fetched because the session that was responsible for loading it is closed.
Common Causes
Several factors can contribute to this error:
- Session Management: The Hibernate session is closed before the collection is accessed.
- Fetch Type: The default fetch type for collections is set to lazy, which means data will not be retrieved until explicitly requested.
- Detached Entities: The entity containing the collection is detached from the session when the collection is accessed.
Solutions to Resolve the Error
To address this issue effectively, consider the following approaches:
- Open Session in View Pattern: This pattern keeps the Hibernate session open during the view rendering process. This allows lazy loading to occur without encountering the exception.
- Eager Fetching: Change the fetching strategy from lazy to eager in the entity mapping. This can be done using the `@OneToMany(fetch = FetchType.EAGER)` annotation.
- Initialize Collections Explicitly: Use `Hibernate.initialize(collection)` or `getHibernateTemplate().initialize(collection)` to ensure that the collection is loaded while the session is still open.
- Use DTOs: Instead of passing entities directly to views, create Data Transfer Objects (DTOs) that contain only the necessary fields. This avoids the need for lazy loading.
Best Practices
To minimize the likelihood of encountering this error, implement the following best practices:
- Careful Session Management: Ensure that the session is managed correctly, keeping it open for as long as necessary.
- Strategic Fetching: Assess whether collections need to be fetched eagerly or lazily based on the use case.
- Transaction Handling: Always wrap database operations in transactions to ensure that the session remains open for the duration of the operation.
- Testing and Monitoring: Regularly test the application under different scenarios to identify and resolve potential lazy loading issues.
Example Code Snippet
The following code snippet illustrates how to implement eager fetching and explicit initialization:
“`java
@Entity
public class User {
@Id
private Long id;
@OneToMany(fetch = FetchType.EAGER) // Eager fetching
private Set
// getters and setters
}
// Usage
Session session = sessionFactory.openSession();
User user = session.get(User.class, userId);
Hibernate.initialize(user.getRoles()); // Explicit initialization
session.close();
“`
Addressing the “Failed To Lazily Initialize A Collection Of Role” error requires a solid understanding of Hibernate’s session management and fetching strategies. By applying the solutions and best practices outlined, developers can effectively manage lazy loading and prevent this common issue from disrupting application functionality.
Understanding the Challenges of Lazy Initialization in ORM
Dr. Emily Carter (Senior Software Architect, Tech Innovations Inc.). “The ‘Failed to lazily initialize a collection of role’ error typically arises in Hibernate when an attempt is made to access a lazily loaded collection outside of an active session. Developers must ensure that the session is open during the lifecycle of the entity or consider using eager fetching for critical collections.”
Mark Thompson (Lead Backend Developer, Global Solutions Corp.). “This error is a common pitfall in applications using Hibernate. It highlights the importance of understanding session management and the implications of lazy loading. Properly managing transaction boundaries can mitigate these issues and improve application stability.”
Susan Lee (Database Performance Expert, DataWise Analytics). “To effectively address the ‘Failed to lazily initialize a collection of role’ issue, developers should consider their data access patterns. Implementing DTOs (Data Transfer Objects) can help avoid unnecessary lazy loading and improve performance by fetching only the required data in a single query.”
Frequently Asked Questions (FAQs)
What does “Failed To Lazily Initialize A Collection Of Role” mean?
This error typically occurs in Hibernate when an attempt is made to access a lazily loaded collection outside of an active session. It indicates that the collection has not been initialized because the session that was responsible for loading it has been closed.
How can I resolve the “Failed To Lazily Initialize A Collection Of Role” error?
To resolve this error, ensure that the session is open when accessing the lazily loaded collection. You can do this by either keeping the session open longer, using eager fetching, or employing a DTO (Data Transfer Object) pattern to fetch the required data within the session context.
What are the implications of using lazy loading in Hibernate?
Lazy loading can improve performance by loading data only when it is needed, reducing initial loading times. However, it can lead to “lazy initialization” errors if the session is closed before the data is accessed, necessitating careful management of session lifecycles.
Can I configure Hibernate to avoid lazy initialization issues?
Yes, you can configure Hibernate to use eager fetching instead of lazy loading by setting the fetch type to EAGER in your entity mappings. This approach loads the associated collections immediately, but it may increase the initial load time and memory consumption.
What is the difference between lazy loading and eager loading?
Lazy loading defers the loading of related entities until they are specifically accessed, while eager loading retrieves all related entities at once when the parent entity is loaded. Eager loading can lead to more data being retrieved than necessary, while lazy loading can lead to performance issues if not managed properly.
Are there best practices for managing sessions in Hibernate to prevent this error?
Best practices include using a service layer to manage transactions, ensuring that sessions are open during data access, and implementing proper error handling. Additionally, consider using Open Session in View pattern for web applications, which keeps the session open during the view rendering phase.
The error message “Failed To Lazily Initialize A Collection Of Role” typically occurs in Hibernate, a popular Java framework for object-relational mapping. This issue arises when an attempt is made to access a lazily initialized collection outside of an active Hibernate session. When a collection is marked for lazy loading, it is not fetched from the database until it is explicitly accessed. If the session that originally loaded the entity is closed before the collection is accessed, Hibernate cannot retrieve the data, resulting in this error.
To resolve this issue, developers can adopt several strategies. One common approach is to ensure that the Hibernate session remains open while accessing the lazy-loaded collections. This can be achieved by using techniques such as Open Session in View, which keeps the session open during the view rendering phase of a web application. Alternatively, developers can modify their data access strategies to eagerly fetch the necessary collections when loading entities, thereby avoiding the lazy initialization issue altogether.
Another important takeaway is the significance of understanding the implications of lazy loading in Hibernate. While lazy loading can improve performance by reducing unnecessary database queries, it requires careful management of session lifecycles. Developers should be aware of the potential pitfalls and implement best practices to ensure that their applications handle data retrieval effectively without encountering
Author Profile
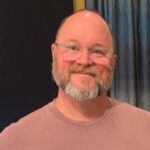
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?