Why Did I Encounter ‘Failed To Execute ‘Postmessage’ On ‘Worker’ Error?
In the ever-evolving landscape of web development, the seamless interaction between different components of an application is crucial for delivering a smooth user experience. However, developers often encounter a range of challenges that can disrupt this harmony, one of which is the notorious error: “Failed to execute ‘PostMessage’ on ‘Worker’.” This error can be a frustrating roadblock, particularly when it comes to using web workers for background processing. Understanding the nuances of this issue is essential for developers striving to build efficient, responsive applications.
The “Failed to execute ‘PostMessage’ on ‘Worker'” error typically arises when there are communication issues between the main thread and web workers. Web workers allow scripts to run in the background, enabling developers to perform complex tasks without freezing the user interface. However, when the messaging system that facilitates this interaction encounters problems, it can lead to unexpected behavior and application crashes. This article delves into the common causes of this error, offering insights into how to diagnose and resolve it effectively.
As we explore the intricacies of this error, we will also highlight best practices for using web workers and ensuring smooth communication between threads. By understanding the underlying mechanisms and potential pitfalls, developers can enhance their applications’ performance and reliability. Whether you’re a seasoned developer or just
Understanding the Error
The error message “Failed To Execute ‘Postmessage’ On ‘Worker'” typically arises in web development, particularly when working with Web Workers in JavaScript. This error indicates that there was an attempt to use the `postMessage` method on a worker, but the message could not be sent due to various reasons.
Common causes of this error include:
- Invalid Message Format: The message being sent to the worker must be serializable. If non-serializable objects, such as functions or DOM elements, are sent, this error may occur.
- Worker Termination: If the worker has been terminated or is in the process of being terminated, any attempts to send messages will result in this error.
- Cross-Origin Issues: Sending messages between workers and their parent contexts can lead to issues if they are not properly handled, particularly with regards to cross-origin resource sharing (CORS).
Debugging Strategies
To effectively address the “Failed To Execute ‘Postmessage’ On ‘Worker'” error, developers can utilize several debugging strategies:
- Check Message Format: Ensure that the data being sent is a serializable object. Use primitive data types or structured objects that can be easily serialized.
- Verify Worker State: Before sending a message, check whether the worker is still active. This can be done by implementing checks in the main thread.
- Handle Errors Gracefully: Implement try-catch blocks around your postMessage calls to capture and handle any errors gracefully.
Example of Proper Usage
Here’s a simple example illustrating the correct way to use `postMessage` with a Web Worker:
“`javascript
// Main thread
const worker = new Worker(‘worker.js’);
worker.onmessage = function(event) {
console.log(‘Message received from worker:’, event.data);
};
try {
const message = { type: ‘start’, data: ‘some data’ };
worker.postMessage(message);
} catch (error) {
console.error(‘Error sending message:’, error);
}
“`
“`javascript
// worker.js
onmessage = function(event) {
const receivedData = event.data;
// Process received data and send a message back
postMessage(‘Processed: ‘ + receivedData.data);
};
“`
Best Practices
To minimize the occurrence of this error, adhere to the following best practices:
- Use Simple Data Types: When possible, limit the data sent to simple types like strings, numbers, or arrays.
- Implement Worker Lifecycle Management: Properly manage the lifecycle of your workers, ensuring they are alive when messages are sent.
- Test Across Browsers: Different browsers may handle Web Workers and messages differently, so thorough testing is essential.
Cause | Solution |
---|---|
Invalid Message Format | Ensure only serializable data types are sent. |
Worker Termination | Check worker state before sending messages. |
Cross-Origin Issues | Set up appropriate CORS headers. |
Understanding the Error
The error message `Failed To Execute ‘PostMessage’ On ‘Worker’` typically arises in web development when attempting to send messages to a Web Worker that is no longer available or has not been properly instantiated. This can occur due to several reasons:
- Worker Termination: The worker might have been terminated before the message was sent.
- Incorrect Worker Context: The message may be sent from an incorrect context that does not have access to the worker.
- Cross-Origin Restrictions: Sending messages across different origins may trigger security restrictions, leading to the failure.
Common Causes
Identifying the root cause of this error is crucial for effective troubleshooting. Here are the most common causes:
- Worker Lifecycle Management:
- Workers can be terminated automatically when they are no longer needed. Ensure that the worker is alive at the time of the message.
- Initialization Issues:
- The worker must be properly initialized before sending messages. Check for any asynchronous loading issues that may prevent the worker from being ready.
- Message Format:
- The message being sent must be serializable. Circular references or unsupported data types can lead to errors.
- Scope and Context:
- Verify that the postMessage call is made from the correct scope. Messages sent from an incorrect context will not reach the intended worker.
Troubleshooting Steps
To address the `Failed To Execute ‘Postmessage’ On ‘Worker’` error, follow these troubleshooting steps:
- Check Worker Status:
- Confirm that the worker is running by implementing logging in both the main thread and worker script.
- Verify Initialization:
- Ensure that the worker is fully initialized and ready to receive messages before any postMessage calls.
- Inspect Message Data:
- Utilize debugging tools to inspect the data being passed to postMessage. Ensure it is serializable.
- Cross-Origin Policies:
- If applicable, check the same-origin policy and ensure that both the main thread and worker are from the same origin.
- Error Handling:
- Implement error handling in the worker to catch and log any errors that may occur when processing messages.
Best Practices
Adopting best practices can prevent this error from occurring:
- Lifecycle Management:
- Use appropriate lifecycle management techniques to control when workers are created and terminated.
- Use Promises:
- Wrap the postMessage calls in promises to handle asynchronous behavior and improve error handling.
- Centralized Communication:
- Create a centralized communication module to manage messages between the main thread and workers, reducing the risk of miscommunication.
- Debugging Tools:
- Leverage browser developer tools to monitor worker activity and inspect messages being sent and received.
Best Practice | Description |
---|---|
Lifecycle Management | Control worker creation and termination effectively. |
Use Promises | Manage asynchronous postMessage calls with error handling. |
Centralized Communication | Implement a module for consistent message handling. |
Debugging Tools | Utilize tools to monitor worker communications and diagnose issues. |
Adhering to the outlined strategies and understanding the potential causes can significantly mitigate the occurrence of the `Failed To Execute ‘Postmessage’ On ‘Worker’` error, thereby enhancing the robustness of web applications utilizing Web Workers.
Understanding the ‘Postmessage’ Error in Web Workers
Dr. Emily Carter (Web Development Specialist, Tech Innovations Inc.). “The ‘Failed To Execute ‘Postmessage’ On ‘Worker” error typically arises when there is an attempt to send a message to a worker that is either not properly instantiated or has already terminated. Ensuring that the worker is active and correctly set up is crucial for seamless communication.”
Michael Chen (Lead Software Engineer, Cloud Solutions Corp.). “This error can also occur due to serialization issues with the data being sent. If the message contains non-serializable objects, the worker will reject the message. Developers must ensure that only serializable data types are used when communicating with web workers.”
Sarah Thompson (Senior Frontend Developer, Digital Dynamics). “Debugging this error often involves checking the lifecycle of the web worker. If a worker is terminated prematurely or if there’s an error in the worker’s script, it can lead to this message failure. Proper error handling and logging within the worker can greatly aid in identifying the root cause.”
Frequently Asked Questions (FAQs)
What does the error ‘Failed To Execute ‘Postmessage’ On ‘Worker” mean?
This error indicates that there was an attempt to use the `postMessage` method on a web worker, but the message could not be sent due to various reasons, such as invalid data types or the worker being terminated.
What are common causes of the ‘Failed To Execute ‘Postmessage’ On ‘Worker” error?
Common causes include attempting to send non-serializable objects, sending messages after the worker has been terminated, or exceeding the message size limits imposed by the browser.
How can I resolve the ‘Failed To Execute ‘Postmessage’ On ‘Worker” error?
To resolve this error, ensure that the data being sent is serializable, avoid sending large objects, and check that the worker is still active before sending messages.
Can this error occur in all browsers?
Yes, this error can occur in all modern browsers that support web workers. However, the specifics of the error handling may vary slightly between different browser implementations.
Is there a way to debug the ‘Failed To Execute ‘Postmessage’ On ‘Worker” error?
Debugging can be done by using browser developer tools to inspect the messages being sent and received, checking the worker’s lifecycle, and ensuring that the data types are appropriate for serialization.
Does this error affect the performance of my web application?
While this error itself does not directly impact performance, frequent occurrences may indicate underlying issues in your application’s architecture, potentially leading to performance degradation if not addressed.
The error message “Failed to execute ‘PostMessage’ on ‘Worker'” typically arises in web development when there is an issue with communication between the main thread and a web worker. This problem can occur due to various reasons, including attempting to send messages to a worker that has already terminated, or when the data being sent is not serializable. Understanding the underlying causes of this error is crucial for developers seeking to implement effective multi-threading in JavaScript applications.
One key takeaway is the importance of ensuring that the web worker is in a valid state before attempting to communicate with it. Developers should implement checks to confirm that the worker is active and ready to receive messages. Additionally, it is essential to ensure that the data being sent is compatible with the structured clone algorithm, which is used by the postMessage method to serialize the data.
Another valuable insight is the significance of proper error handling when working with web workers. By incorporating robust error handling mechanisms, developers can gracefully manage situations where communication fails, thereby improving the overall user experience. This includes using try-catch blocks and monitoring the worker’s lifecycle to prevent attempts to post messages to a non-existent worker.
In summary, addressing the “Failed to execute ‘PostMessage’
Author Profile
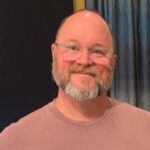
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?