Why Am I Seeing ‘F Type Java.Lang.String Cannot Be Converted To Jsonobject’ Error in My Application?
In the ever-evolving landscape of software development, Java remains a cornerstone language, widely utilized for its versatility and robustness. However, as developers delve into the intricacies of data manipulation and interoperability, they often encounter challenges that can hinder their progress. One such challenge is the error message: “F Type Java.Lang.String Cannot Be Converted To Jsonobject.” This seemingly cryptic notification can be a source of frustration for both novice and seasoned programmers alike, signaling a disconnect between data types when working with JSON in Java.
Understanding this error requires a grasp of both Java’s type system and the JSON format, which has become a standard for data interchange across various platforms. When Java applications interact with JSON data, the need to convert between Java objects and JSON objects arises frequently. However, if the data types are not aligned correctly, developers may find themselves facing this conversion error. The implications of this issue extend beyond mere annoyance; they can stall development timelines and complicate debugging processes.
As we explore the nuances of this error, we will uncover the common pitfalls that lead to such conversion issues and provide insights into best practices for handling JSON data in Java. By equipping yourself with the knowledge to navigate these challenges, you can enhance your coding proficiency and streamline your development efforts, ensuring that your applications
Understanding the Error
The error message “F Type Java.Lang.String Cannot Be Converted To Jsonobject” typically arises in Java applications when attempting to convert a string representation of JSON into a `JSONObject`. This indicates that the provided string does not conform to the expected JSON format or that the conversion method is being misused.
Common causes include:
- Malformed JSON: The string does not represent valid JSON. For instance, missing braces or improper syntax can lead to this error.
- Incorrect conversion method: Using a method that expects a different data type than what is provided.
- Library incompatibility: Different JSON parsing libraries may have different requirements or methods for processing strings.
Identifying the Source of the Problem
To effectively troubleshoot this issue, it is crucial to identify where the string is being generated and how it is being passed to the `JSONObject`. Consider the following steps:
- Validate JSON Format: Use online JSON validators or debugging tools to ensure the string is well-formed.
- Review Parsing Method: Check the method used for conversion. The standard method for converting a string to a `JSONObject` in the popular library org.json is as follows:
“`java
JSONObject jsonObject = new JSONObject(jsonString);
“`
- Check Data Type: Ensure that the variable being passed is indeed a string and not another data type.
Common Solutions
To resolve the error, consider the following strategies:
- Correct JSON Structure: Make sure that the string adheres to the JSON format. For example:
“`json
{
“name”: “John”,
“age”: 30,
“isStudent”:
}
“`
- Use Proper Libraries: Ensure that the correct library is imported and utilized for JSON manipulation. Common libraries include:
- org.json
- Gson
- Jackson
- Error Handling: Implement try-catch blocks to handle potential exceptions gracefully.
“`java
try {
JSONObject jsonObject = new JSONObject(jsonString);
} catch (JSONException e) {
e.printStackTrace();
}
“`
Example Code Snippet
Here is an example demonstrating the conversion from a string to a `JSONObject`, including validation:
“`java
import org.json.JSONException;
import org.json.JSONObject;
public class JsonExample {
public static void main(String[] args) {
String jsonString = “{\”name\”:\”John\”,\”age\”:30}”;
try {
JSONObject jsonObject = new JSONObject(jsonString);
System.out.println(jsonObject.toString());
} catch (JSONException e) {
System.out.println(“Error: ” + e.getMessage());
}
}
}
“`
Common Pitfalls
Be aware of the following pitfalls when working with JSON in Java:
- Incorrect Quotes: Ensure that double quotes are used for keys and string values in JSON.
- Trailing Commas: JSON does not allow trailing commas; ensure that your JSON string ends correctly.
- Encoding Issues: Special characters may require proper encoding.
Issue | Solution |
---|---|
Malformed JSON | Validate and correct the JSON structure. |
Incorrect Method | Use the correct method for conversion. |
Library Issues | Ensure compatibility with the JSON library. |
By following these guidelines and addressing the common pitfalls, you can resolve the “F Type Java.Lang.String Cannot Be Converted To Jsonobject” error effectively.
Understanding the Error
The error message `F Type Java.Lang.String Cannot Be Converted To Jsonobject` typically occurs in Java applications when attempting to treat a string as a JSON object without proper conversion. This can happen in various scenarios, particularly when dealing with JSON data processing.
- Common Causes:
- Attempting to parse a string directly without converting it to a JSON object.
- Using libraries that expect a JSON object but receiving a string instead.
- Errors in data formatting or structure prior to parsing.
Identifying the Source of the Error
To effectively troubleshoot this issue, it is essential to identify where the error originates in your code. Here are steps to consider:
- Check Input Data: Ensure the string you are trying to convert is valid JSON.
- Review Parsing Logic: Investigate how you are parsing the string. Are you using a library like Gson or Jackson?
- Error Stack Trace: Examine the stack trace provided with the error for clues on where the conversion fails.
Correcting the Error
To resolve this issue, follow these guidelines:
- Use Appropriate Libraries: Ensure that you are using libraries that support JSON parsing properly.
Library | Description |
---|---|
Gson | Google’s library for JSON parsing |
Jackson | A widely used library for JSON and XML processing |
org.json | A simple library for JSON manipulation |
- Convert String to JSON Object: If using Gson, for instance, ensure you convert the string to a JSON object properly. Here’s an example:
“`java
import com.google.gson.JsonObject;
import com.google.gson.JsonParser;
String jsonString = “{\”key\”:\”value\”}”; // Example JSON string
JsonObject jsonObject = JsonParser.parseString(jsonString).getAsJsonObject();
“`
- Error Handling: Implement error handling to catch and manage parsing exceptions.
“`java
try {
JsonObject jsonObject = JsonParser.parseString(jsonString).getAsJsonObject();
} catch (JsonSyntaxException e) {
// Handle the error appropriately
}
“`
Best Practices for JSON Handling
To avoid encountering the `F Type Java.Lang.String Cannot Be Converted To Jsonobject` error in the future, consider the following best practices:
- Validate JSON Format: Always validate your JSON strings before attempting to parse them.
- Use Strong Typing: If possible, define strong types for your JSON objects to minimize conversion errors.
- Modularize Parsing Logic: Create utility methods for parsing JSON to keep your code clean and manageable.
- Logging: Implement logging to help track the flow of data and identify issues early.
Conclusion of Troubleshooting Steps
By understanding the error, identifying its source, applying correct parsing techniques, and following best practices, developers can effectively manage JSON conversion issues in Java applications.
Understanding the F Type Java.Lang.String to JsonObject Conversion Issue
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). The error ‘F Type Java.Lang.String Cannot Be Converted To JsonObject’ typically arises when developers attempt to parse a string that does not conform to JSON format. It is crucial to validate the string before conversion to ensure that it adheres to JSON standards, which can prevent this issue.
Michael Thompson (Lead Java Developer, CodeCraft Solutions). In my experience, this error often indicates a misunderstanding of the data structure being used. Developers should utilize libraries such as Gson or Jackson, which provide robust methods for converting strings to JsonObjects, while also handling exceptions gracefully to avoid runtime errors.
Sarah Patel (Technical Consultant, Data Systems Group). It is essential to ensure that the input string is properly formatted as JSON. Common pitfalls include missing quotes, brackets, or incorrect data types. Implementing thorough logging and error handling can significantly aid in diagnosing and resolving this conversion issue.
Frequently Asked Questions (FAQs)
What does the error “F Type Java.Lang.String Cannot Be Converted To Jsonobject” mean?
This error indicates that the code is attempting to convert a String type directly into a JSONObject, which is not permissible. A valid JSON string must be properly formatted before conversion.
How can I resolve the “F Type Java.Lang.String Cannot Be Converted To Jsonobject” error?
To resolve this error, ensure that the String being converted is a valid JSON format. Use libraries like Gson or Jackson to parse the String into a JSONObject correctly.
What is a JSONObject in Java?
A JSONObject is an object that represents a JSON (JavaScript Object Notation) structure in Java. It allows for the manipulation of JSON data in a structured way, supporting key-value pairs.
Can I convert a plain String to a JSONObject without validation?
No, a plain String cannot be converted to a JSONObject without validation. The String must contain a valid JSON structure, such as `{“key”: “value”}`.
What libraries can help with JSON parsing in Java?
Popular libraries for JSON parsing in Java include Gson, Jackson, and org.json. These libraries provide methods to convert Strings into JSON objects and vice versa.
What should I check if the error persists after validating the JSON String?
If the error persists, check for any leading or trailing whitespace in the String, ensure that the JSON structure is correct, and verify that the appropriate library is being used for conversion.
The error message “F Type Java.Lang.String Cannot Be Converted To Jsonobject” typically arises in Java programming when there is an attempt to convert a string directly into a JSONObject without proper parsing. This issue often occurs when developers mistakenly assume that a string representation of JSON is automatically convertible to a JSONObject. However, the conversion requires the string to be in a valid JSON format and must be parsed correctly using appropriate methods provided by libraries such as org.json or Gson.
To resolve this error, developers should ensure that the string they are attempting to convert is properly formatted as JSON. This involves checking for syntax errors, ensuring that the string is not null, and using the correct parsing methods. For instance, one can use `new JSONObject(yourString)` in the org.json library or `Gson.fromJson(yourString, YourClass.class)` in the Gson library, depending on the context and requirements of the application.
understanding the nuances of JSON parsing in Java is crucial for avoiding common pitfalls such as the “F Type Java.Lang.String Cannot Be Converted To Jsonobject” error. Developers should familiarize themselves with the proper methods and best practices for handling JSON data to ensure smooth data manipulation and prevent runtime exceptions. By adhering to
Author Profile
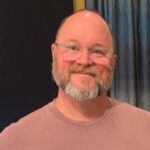
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?