Why Am I Getting an ‘Extraneous Input’ Error: What Does ‘Expecting Id’ Mean?
In the realm of programming and software development, encountering errors can be both a frustrating and enlightening experience. One such error that often perplexes developers is the cryptic message: “Extraneous Input ‘[‘ Expecting Id.” This seemingly simple notification can signal a range of issues, from syntax errors to misconfigured parameters. Understanding the nuances behind this error is essential for anyone looking to refine their coding skills and enhance their troubleshooting abilities. As we delve into the intricacies of this message, we will uncover the common pitfalls that lead to its emergence and explore effective strategies for resolution.
When faced with the “Extraneous Input ‘[‘ Expecting Id” error, developers are often left grappling with its implications. This issue typically arises in contexts where the programming language or framework expects a specific identifier but encounters an unexpected character instead. Such errors can stem from a variety of sources, including misplaced brackets, incorrect variable names, or even typographical mistakes. Recognizing the underlying causes is crucial for swiftly diagnosing and correcting the problem, ultimately leading to more robust code.
Moreover, the significance of this error extends beyond mere annoyance; it serves as a reminder of the importance of precision in coding. By examining the circumstances under which this error occurs, developers can cultivate a deeper understanding of syntax rules and best
Understanding the Error Message
The error message “Extraneous Input ‘[‘ Expecting Id” typically occurs in programming environments, particularly those involving parsing or compilation processes. It signifies that the parser encountered an unexpected character—specifically, a square bracket—when it was anticipating a valid identifier. Identifiers are names used to identify variables, functions, classes, or other entities in programming languages.
Common scenarios where this error may arise include:
- Syntax Errors: When the code structure does not conform to the expected syntax rules of the programming language.
- Typographical Mistakes: Misspellings or misplaced characters can lead to this confusion for the parser.
- Improper Use of Delimiters: Utilizing square brackets incorrectly, especially in languages that reserve them for specific functions like array declarations or indexing.
Troubleshooting Steps
To resolve the “Extraneous Input ‘[‘ Expecting Id” error, follow these troubleshooting steps:
- Examine the Code Around the Error: Locate the line number indicated by the error message. Check for any misplaced brackets or identifiers.
- Check for Typos: Ensure that all identifiers are spelled correctly and that there are no accidental extra characters.
- Verify Syntax Rules: Consult the language documentation to ensure that the code adheres to the required syntax.
- Reformat the Code: Sometimes, simply restructuring the code can help the parser interpret it correctly.
- Use an IDE or Linter: Integrated Development Environments (IDEs) or linting tools can highlight syntax errors, making it easier to identify and fix the issue.
Example Scenario
Consider the following example in a programming language:
“`python
def example_function():
values = [1, 2, 3
return values
“`
In this case, the error arises because of a missing closing bracket for the list declaration. The corrected version should be:
“`python
def example_function():
values = [1, 2, 3]
return values
“`
Common Causes of the Error
Understanding the common causes of this error can help developers avoid it in the future. Here are some frequent issues:
- Incorrect Array or List Initialization: Forgetting to close brackets properly.
- Using Reserved Characters: Misusing characters that have specific meanings in the programming language.
- Improper Nesting: Nesting brackets or parentheses incorrectly can lead to confusion in the parser.
Comparison of Syntax Elements
A table comparing the syntax elements that can lead to this error is provided below:
Element | Correct Usage | Common Mistake |
---|---|---|
Array Declaration | array = [1, 2, 3] | array = [1, 2, 3 |
Function Call | functionName(arg1, arg2) | functionName(arg1, [arg2] |
Conditional Statement | if (condition) { doSomething(); } | if [condition) { doSomething(); } |
By addressing these common pitfalls, developers can reduce the likelihood of encountering the “Extraneous Input ‘[‘ Expecting Id” error and enhance their coding efficiency.
Understanding the Error Message
The error message `Extraneous Input ‘[‘ Expecting Id` typically indicates a syntax error in code, often occurring in languages such as SQL or programming frameworks that utilize specific syntax rules. This error generally points to an unexpected character, in this case, an opening square bracket `[`, that disrupts the expected structure of the code.
Common Causes
Several factors can lead to this error:
- Improper Syntax: Using brackets without proper context. For instance, in SQL, brackets are often used for object identifiers but must be correctly placed.
- Incorrectly Formatted Queries: Missing required elements such as keywords or identifiers that the parser expects.
- Typographical Errors: Simple mistakes like an extra character or misplaced punctuation can trigger this error.
- Version Mismatches: Different versions of a database or programming language may have varying syntax rules.
Troubleshooting Steps
To resolve the `Extraneous Input ‘[‘ Expecting Id` error, follow these steps:
- Check Syntax:
- Review the line indicated by the error message.
- Ensure that all brackets are correctly used and paired.
- Validate Query Structure:
- Ensure that the SQL query or code adheres to the correct format.
- Look for missing keywords or identifiers.
- Use a Linter or Validator:
- Implement tools that highlight syntax errors.
- Linters can provide instant feedback on code quality.
- Review Documentation:
- Consult the documentation for the specific language or framework to confirm the correct syntax.
- Look for examples that illustrate proper usage of brackets.
- Test Incrementally:
- If possible, break the code into smaller parts and test incrementally.
- This can help isolate the problematic area.
Example Scenarios
Scenario | Description | Solution |
---|---|---|
SQL Query with Brackets | `SELECT * FROM [TableName] WHERE [ColumnName] = ‘Value’` | Ensure `TableName` and `ColumnName` are valid identifiers. |
Programming Language Error | `array = [1, 2, 3]` without proper context in a function call | Check if the array is being declared correctly. |
Incorrect Function Call | `function([parameter])` where brackets are not expected | Remove brackets or use parentheses if required. |
Preventive Measures
To avoid encountering this error in the future, consider the following practices:
- Code Reviews: Regularly conduct peer reviews to catch syntax issues early.
- Consistent Formatting: Maintain consistent code formatting to improve readability and reduce errors.
- Continuous Learning: Stay updated with the latest syntax changes and best practices in the programming language or framework being used.
- Error Handling: Implement robust error handling to provide more informative messages for debugging.
By understanding the context and structure required by the language or framework in use, many syntax errors, including `Extraneous Input ‘[‘ Expecting Id`, can be effectively managed and resolved.
Understanding the Error: ‘Extraneous Input ‘[‘ Expecting Id’
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The error message ‘Extraneous Input ‘[‘ Expecting Id’ typically arises in programming languages when the parser encounters an unexpected character or symbol. It indicates that the syntax is incorrect, and developers should review their code for misplaced brackets or misformatted expressions.”
Mark Thompson (Database Administrator, Data Solutions Group). “In database queries, this error can occur when using SQL syntax improperly. It is crucial to ensure that all identifiers are correctly formatted and that there are no additional characters that could confuse the parser, such as an unescaped bracket.”
Lisa Chen (Lead Developer, CodeCraft Technologies). “When faced with the ‘Extraneous Input ‘[‘ Expecting Id’ error, I advise developers to utilize debugging tools that can help trace the source of the syntax error. Often, the issue lies in a previous line of code that affects how the parser reads subsequent lines.”
Frequently Asked Questions (FAQs)
What does the error message ‘Extraneous Input ‘[‘ Expecting Id’ mean?
This error typically indicates that there is an unexpected character, specifically a bracket ‘[‘ in the code or query where an identifier (Id) was anticipated. This often occurs in programming or database query contexts.
What are common causes of the ‘Extraneous Input’ error?
Common causes include syntax errors, misplaced or unmatched brackets, or incorrectly formatted queries. It can also arise from using reserved keywords or incorrect data types in the code.
How can I troubleshoot the ‘Extraneous Input’ error?
To troubleshoot, review the code or query for syntax errors, ensure all brackets are correctly matched, and verify that identifiers are properly formatted. Debugging tools or syntax checkers can also help identify issues.
Is this error specific to any programming language or database?
While the error message may vary slightly, the concept of extraneous input is common across many programming languages and database systems, including SQL and various scripting languages.
Can this error affect the performance of my application?
Yes, if not addressed, this error can prevent code execution, leading to application crashes or failures in data retrieval, ultimately impacting overall performance and user experience.
What steps should I take if I cannot resolve the error?
If resolution proves difficult, consider seeking assistance from online forums, consulting documentation for the specific language or database, or reaching out to a professional developer for guidance.
The error message “Extraneous Input ‘[‘ Expecting Id” typically arises in programming contexts, particularly when dealing with syntax errors in code. This message indicates that the parser encountered an unexpected character, in this case, the square bracket ‘[‘, when it was anticipating an identifier. Identifiers are crucial in programming as they represent variable names, function names, and other entities within the code. Understanding this error is essential for debugging and ensuring that code runs smoothly.
One of the main points to consider is the importance of syntax in programming languages. Each language has specific rules regarding how code should be structured. When these rules are not followed, errors like the one mentioned can occur, leading to confusion and potential delays in development. Developers must be diligent in adhering to the syntax rules of the language they are using to minimize such errors.
Additionally, this error highlights the necessity for thorough code review and testing practices. By implementing robust testing frameworks and conducting regular code reviews, developers can catch such syntax errors early in the development process. This proactive approach not only enhances code quality but also improves overall productivity by reducing the time spent on debugging.
the “Extraneous Input ‘[‘ Expecting Id” error serves as a reminder of the critical
Author Profile
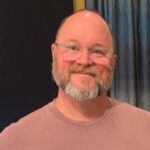
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?