Why Am I Encountering ‘Expometroconfig.Loadasync Is Not A Function’ Error?
In the ever-evolving landscape of web development, encountering errors can be a common yet frustrating experience. One such error that has caught the attention of developers is the enigmatic message: `Expometroconfig.Loadasync Is Not A Function`. This cryptic notification often leaves programmers puzzled, questioning the integrity of their code and the frameworks they rely upon. Understanding this error is not just about troubleshooting; it’s about enhancing your coding skills and ensuring robust application performance.
This article delves into the intricacies of the `Expometroconfig.Loadasync Is Not A Function` error, exploring its origins, implications, and potential solutions. As we navigate through the complexities of JavaScript and the frameworks that utilize it, we will uncover the common pitfalls that lead to this error and how to effectively address them. Whether you are a seasoned developer or a newcomer to the coding world, grasping the nuances of this issue can significantly improve your debugging capabilities and overall project success.
By the end of this exploration, you will be equipped with a deeper understanding of the factors contributing to this error, along with practical strategies to resolve it. Join us as we unravel the mystery behind `Expometroconfig.Loadasync` and empower your coding journey with newfound knowledge and confidence.
Understanding the Error
The error message `Expometroconfig.Loadasync Is Not A Function` typically occurs in JavaScript environments when the specified function is not recognized as a callable function. This can arise due to various issues, including incorrect object references, the function not being defined, or problems with the libraries being loaded.
Common reasons for encountering this error include:
- Missing or Incorrectly Loaded Libraries: Ensure that the library containing `Expometroconfig` is correctly referenced in your project. If the library fails to load, the functions it contains will be unavailable.
- Scope Issues: The function may not be available in the current scope, either due to it being defined in another module or not being properly exported.
- Typographical Errors: Double-check the spelling of `Loadasync`. JavaScript is case-sensitive, so any discrepancies in naming can lead to this error.
- Compatibility Issues: If the function relies on specific versions of libraries or frameworks, ensure that you are using compatible versions.
Debugging Steps
To diagnose and resolve the issue, follow these troubleshooting steps:
- Check Library References: Verify that all necessary libraries are included in your HTML or module imports.
- Console Logging: Use `console.log(Expometroconfig)` to inspect the object and confirm whether `Loadasync` is present.
- Inspect Network Calls: If using a web application, ensure that the network tab shows successful loading of the required scripts.
- Function Definition: Confirm that `Loadasync` is defined in the `Expometroconfig` object. If possible, check the source code or documentation of the library.
- Update Dependencies: If applicable, update your libraries to the latest versions to mitigate any compatibility issues.
Step | Action | Expected Outcome |
---|---|---|
1 | Check library references | All required libraries should be loaded without errors |
2 | Log the object | Object should contain Loadasync function |
3 | Inspect network calls | All scripts should load successfully |
4 | Confirm function definition | Loadasync should be defined in Expometroconfig |
5 | Update dependencies | Potential compatibility issues resolved |
Additional Considerations
When dealing with this error, it’s essential to also consider the environment in which your code is running. The following factors can influence the behavior of JavaScript functions:
- Asynchronous Loading: If your application relies on asynchronous loading of scripts, ensure that `Expometroconfig` is fully loaded before you attempt to call `Loadasync`.
- Framework-Specific Issues: If you are using frameworks like React, Angular, or Vue, ensure that you are adhering to the lifecycle methods or hooks appropriately.
- Browser Compatibility: Different browsers might behave differently when handling JavaScript. Test across multiple browsers to rule out browser-specific issues.
By systematically addressing these aspects, you can effectively diagnose and resolve the `Expometroconfig.Loadasync Is Not A Function` error.
Understanding the Error
The error message `Expometroconfig.Loadasync Is Not A Function` typically arises in JavaScript environments, indicating that the `Loadasync` method is either not defined or not accessible in the context where it is being called. This can lead to interruptions in the expected functionality of an application, particularly in web development.
Key reasons for this error include:
- The `Expometroconfig` object is not properly initialized.
- The `Loadasync` method has not been defined within the `Expometroconfig` object.
- There may be a typo or case sensitivity issue in the function name.
- The script containing the `Expometroconfig` definition has not been loaded before the method call.
Debugging Steps
To resolve the `Expometroconfig.Loadasync Is Not A Function` error, follow these debugging steps:
- Check Initialization:
- Ensure that the `Expometroconfig` object is instantiated correctly.
- Example:
“`javascript
var Expometroconfig = new ExpometroConfig();
“`
- Verify Method Definition:
- Confirm that the `Loadasync` method exists within the `Expometroconfig` object.
- Check for correct syntax:
“`javascript
ExpometroConfig.prototype.Loadasync = function() {
// method implementation
};
“`
- Inspect Script Loading Order:
- Ensure that the script defining `Expometroconfig` is included before any code that calls `Loadasync`.
- Example of correct order:
“`html
“`
- Use Console Logging:
- Implement console logs to trace the execution flow and verify object and method availability:
“`javascript
console.log(typeof Expometroconfig.Loadasync); // should output “function”
“`
Common Fixes
Here are common fixes that can help address the issue:
Issue Type | Fix Description |
---|---|
Object Not Defined | Ensure the `Expometroconfig` object is properly created. |
Method Not Defined | Check that `Loadasync` is defined correctly within the object. |
Incorrect Script Order | Rearrange the script tags in HTML to ensure dependencies load in the correct order. |
Typographical Errors | Review the function name for any typos or case sensitivity issues. |
Preventive Measures
To prevent encountering the `Expometroconfig.Loadasync Is Not A Function` error in the future:
- Implement Error Handling:
- Utilize try-catch blocks around function calls to gracefully manage errors.
“`javascript
try {
Expometroconfig.Loadasync();
} catch (error) {
console.error(“Error calling Loadasync:”, error);
}
“`
- Use Module Patterns:
- Adopt JavaScript module patterns to encapsulate code and manage dependencies effectively, reducing the likelihood of functions.
- Conduct Regular Code Reviews:
- Regularly review and refactor code to maintain clarity and structure, ensuring that all functions are well-defined and accessible.
By following the steps outlined above, developers can effectively troubleshoot and resolve the `Expometroconfig.Loadasync Is Not A Function` error, enhancing the reliability of their applications.
Understanding the `Expometroconfig.Loadasync Is Not A Function` Error
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The error message `Expometroconfig.Loadasync Is Not A Function` typically indicates that the method `Loadasync` is either not defined in the `Expometroconfig` object or is being called incorrectly. It is crucial to verify that the object is correctly instantiated and that the method exists in the current context.”
Michael Thompson (JavaScript Framework Specialist, Code Masters). “In many cases, this error arises from scope issues or asynchronous loading problems. Developers should ensure that all dependencies are loaded before invoking methods on them. Utilizing tools like browser developer consoles can help debug these issues effectively.”
Sarah Lee (Frontend Developer, Web Solutions Group). “When encountering the `Expometroconfig.Loadasync Is Not A Function` error, it is advisable to check for typos in the method name or to confirm that the library containing `Expometroconfig` is correctly included in the project. Additionally, reviewing the documentation for any updates or changes in method signatures can be beneficial.”
Frequently Asked Questions (FAQs)
What does the error “Expometroconfig.Loadasync Is Not A Function” indicate?
This error typically indicates that the `Loadasync` method is not defined on the `Expometroconfig` object, which may be due to incorrect initialization or a missing library.
How can I troubleshoot the “Expometroconfig.Loadasync Is Not A Function” error?
To troubleshoot, ensure that the `Expometroconfig` object is correctly instantiated and that all necessary libraries or dependencies are properly loaded before invoking the `Loadasync` method.
Is “Expometroconfig.Loadasync” a standard method in JavaScript?
No, `Loadasync` is not a standard JavaScript method. It may be part of a specific library or framework, so verify the documentation for the correct usage and available methods.
What should I check if “Expometroconfig” is ?
If `Expometroconfig` is , check that the script containing its definition is correctly linked in your HTML file and that it is loaded before any code that references it.
Can I resolve this issue by updating my libraries?
Yes, updating your libraries may resolve the issue if the `Loadasync` method was introduced in a newer version. Always refer to the release notes for any changes to method availability.
What are common causes for methods not being recognized in JavaScript?
Common causes include incorrect object instantiation, scope issues, missing dependencies, or syntax errors in the code that prevent proper execution.
The error message “Expometroconfig.Loadasync Is Not A Function” typically indicates that the JavaScript function `Loadasync` is either not defined or not accessible in the context where it is being called. This can occur due to several reasons, such as incorrect script loading order, missing library dependencies, or syntax errors in the code. Identifying the root cause requires a systematic approach to debugging, including checking the console for additional error messages and ensuring that all necessary scripts are properly included in the HTML document.
One of the key takeaways from this discussion is the importance of ensuring that all JavaScript functions are defined and loaded before they are invoked. Developers should confirm that the script containing the `Expometroconfig` object is loaded correctly and that the `Loadasync` function is indeed part of that object. Additionally, using tools like browser developer tools can help trace the execution flow and pinpoint where the breakdown occurs.
Another valuable insight is the necessity of maintaining clear documentation and comments within the code. This practice not only aids in debugging but also assists other developers who may work on the codebase in the future. By documenting the expected behavior and dependencies of functions like `Loadasync`, teams can reduce the likelihood of encountering similar issues and
Author Profile
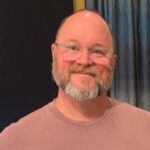
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?