Why Am I Getting the Error: Reference to Non-Static Member Function Must Be Called?
In the world of programming, encountering errors is an inevitable part of the development process. Among the myriad of error messages that can pop up, few are as perplexing as the one that states, “Error: Reference To Non-Static Member Function Must Be Called.” This cryptic message often leaves developers scratching their heads, especially those who are new to object-oriented programming or transitioning to a language that emphasizes the distinction between static and non-static member functions. Understanding this error is crucial for anyone looking to write efficient, error-free code, as it highlights the nuances of class design and function invocation.
At its core, this error arises from a fundamental misunderstanding of how non-static member functions operate within the context of a class. Unlike static functions, which can be called without an instance of the class, non-static member functions require a specific object to invoke them. This distinction is not merely a syntactical detail; it reflects the underlying principles of encapsulation and object-oriented design. When developers attempt to call a non-static function without a corresponding object, they trigger this error, prompting a deeper exploration of their code structure.
As we delve into the intricacies of this error, we will explore its common causes, practical examples, and best practices for avoiding it in the future. By
Error Explanation
When you encounter the error message “Error: Reference To Non-Static Member Function Must Be Called,” it typically indicates that you are trying to call a non-static member function of a class without an instance of that class. Non-static member functions require an object of the class to be invoked, whereas static member functions can be called without creating an instance.
Understanding Member Functions
In object-oriented programming (OOP), member functions can be categorized into two types:
- Static Member Functions: These functions belong to the class rather than any object. They can be called without creating an instance of the class.
- Non-Static Member Functions: These functions operate on instances of the class and have access to instance variables. They must be called on an object of that class.
Example of the Error
Consider the following C++ code snippet:
“`cpp
class Example {
public:
void display() {
std::cout << "Display function called." << std::endl;
}
};
int main() {
Example::display(); // This will cause the error
return 0;
}
```
In this example, `display()` is a non-static member function. Attempting to call it using the class name results in the error because no instance of `Example` is created.
How to Fix the Error
To resolve this error, you need to create an instance of the class and call the non-static member function on that instance. Here’s how you can modify the previous example:
```cpp
class Example {
public:
void display() {
std::cout << "Display function called." << std::endl;
}
};
int main() {
Example ex; // Create an instance of Example
ex.display(); // Correctly calls the display function
return 0;
}
```
Key Points to Remember
- Always ensure you have an instance of the class when calling non-static member functions.
- If a function does not need to access instance variables, consider making it static.
Comparison of Static vs Non-Static Member Functions
Feature | Static Member Function | Non-Static Member Function |
---|---|---|
Access to instance variables | No | Yes |
Called on | Class name | Object instance |
Use case | Utility functions | Instance-specific behavior |
By understanding the distinction between static and non-static member functions, you can avoid common errors in your code and ensure proper usage of class methods.
Understanding the Error
The error message “Reference to non-static member function must be called” typically occurs in C++ when attempting to call a non-static member function without an instance of the class. Non-static member functions require an object of the class to be invoked because they operate on the instance’s data members.
Common Scenarios Leading to the Error
Several situations can trigger this error:
- Calling from a Static Context: When a non-static member function is called from a static member function or a non-member function.
- Incorrect Object Reference: Attempting to call a member function on a pointer or reference that is not properly instantiated.
- Lambda Functions: Using non-static member functions within a lambda that does not capture the object instance.
Examples of the Error
Consider the following examples to illustrate how this error can arise:
“`cpp
class MyClass {
public:
void myFunction() {
// Implementation
}
static void staticFunction() {
myFunction(); // Error: Reference to non-static member function must be called
}
};
“`
In the above code, `myFunction()` cannot be called directly within `staticFunction()` since `myFunction()` is non-static.
Resolving the Error
To fix the error, ensure that non-static member functions are called on an instance of the class. Here are some approaches:
- Use an Instance: Create an object of the class and call the function on that instance.
“`cpp
class MyClass {
public:
void myFunction() {
// Implementation
}
};
void someFunction() {
MyClass obj;
obj.myFunction(); // Correct usage
}
“`
- Pass Instance to Static Function: If you need to call a non-static member function from a static context, pass an instance as a parameter.
“`cpp
class MyClass {
public:
void myFunction() {
// Implementation
}
static void staticFunction(MyClass& obj) {
obj.myFunction(); // Correct usage
}
};
“`
- Capture in Lambda: When using lambdas, ensure you capture the instance of the class.
“`cpp
class MyClass {
public:
void myFunction() {
// Implementation
}
void someMethod() {
auto lambda = [this]() {
myFunction(); // Correct usage
};
lambda();
}
};
“`
Best Practices to Avoid the Error
Implementing the following practices can help prevent this error:
- Understand the Context: Recognize when you are within a static function versus an instance method.
- Use `this` Pointer: When you need to access non-static members within lambdas or static functions, use the `this` pointer to refer to the current instance.
- Code Reviews: Regularly conduct code reviews to catch potential misuse of member functions, ensuring that non-static functions are always called on valid instances.
By understanding the context in which member functions are called and applying best practices, developers can effectively avoid the “Reference to non-static member function must be called” error in C++. Properly structuring class methods and being mindful of static versus non-static context is key to writing robust C++ code.
Understanding the ‘Error: Reference To Non-Static Member Function Must Be Called’
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The error message ‘Reference To Non-Static Member Function Must Be Called’ typically arises when developers attempt to invoke a non-static member function without an instance of the class. This is a common pitfall in object-oriented programming, and understanding the distinction between static and non-static members is crucial for effective coding.”
Mark Thompson (Lead Developer, CodeCraft Solutions). “To resolve this error, developers should ensure they are creating an instance of the class before attempting to call its non-static member functions. It’s essential to grasp the object-oriented principles that dictate how member functions interact with class instances to avoid such issues in the future.”
Linda Zhang (Programming Instructor, Advanced Coding Academy). “This error serves as a reminder of the importance of understanding class design in programming. Non-static member functions are designed to operate on instances of a class, and failing to reference an instance can lead to confusion and bugs. Educating new programmers about these concepts early on can significantly reduce the occurrence of such errors.”
Frequently Asked Questions (FAQs)
What does the error “Reference to non-static member function must be called” mean?
This error indicates that you are attempting to call a non-static member function without an instance of the class. Non-static member functions require an object to be invoked, as they operate on instance data.
How can I fix the “Reference to non-static member function must be called” error?
To resolve this error, ensure that you create an instance of the class before calling the non-static member function. For example, use `ClassName object; object.memberFunction();` instead of calling the function directly.
Can I call a non-static member function from a static context?
No, you cannot call a non-static member function directly from a static context because static methods do not have access to instance-specific data. You must create an instance of the class to access non-static members.
What is the difference between static and non-static member functions?
Static member functions belong to the class itself and can be called without creating an instance. In contrast, non-static member functions belong to instances of the class and can access instance variables and other non-static members.
Are there any scenarios where this error commonly occurs?
This error often arises in callback functions, lambda expressions, or when trying to pass a non-static member function as a pointer or reference without an instance context.
How can I pass a non-static member function as a callback?
To pass a non-static member function as a callback, you can use `std::bind` or lambda functions that capture the instance. For example, `std::bind(&ClassName::memberFunction, this)` or `[this]() { memberFunction(); }` can be used to maintain the correct context.
The error message “Reference To Non-Static Member Function Must Be Called” typically arises in C++ when a non-static member function is invoked without an instance of the class. This situation often occurs when developers mistakenly attempt to call a member function directly using the class name rather than through an object of that class. Understanding the distinction between static and non-static member functions is crucial for effective object-oriented programming in C++.
To resolve this error, one must ensure that non-static member functions are called on an instance of the class. This can be achieved by creating an object of the class and then using that object to invoke the desired member function. Additionally, it is important to recognize scenarios where static member functions can be utilized, as they do not require an instance and can be called directly using the class name.
Key takeaways from this discussion include the importance of object instantiation when working with non-static member functions and the necessity of understanding the differences between static and non-static contexts in C++. By adhering to these principles, developers can avoid common pitfalls associated with this error and write more robust and error-free code.
Author Profile
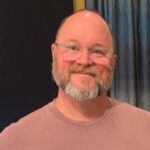
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?