Why Am I Seeing the Error: Ng0205 – Injector Has Already Been Destroyed?
In the dynamic world of Angular development, encountering errors is an inevitable part of the journey. One such perplexing issue that can leave developers scratching their heads is the notorious `Error: Ng0205: Injector Has Already Been Destroyed.` This error typically emerges during the lifecycle of an Angular application, often signaling deeper issues related to dependency injection and component management. As applications grow in complexity, understanding the nuances behind this error becomes crucial for maintaining smooth performance and enhancing user experience. In this article, we will unravel the intricacies of this error, explore its common causes, and provide practical strategies for troubleshooting and resolution.
Overview
The `Ng0205` error serves as a reminder of the importance of Angular’s dependency injection system, which is designed to manage the lifecycle of services and components efficiently. When this error occurs, it often indicates that an attempt is being made to access a service or component that has already been destroyed, leading to potential memory leaks or unexpected behavior in the application. Understanding the context in which this error arises is essential for developers aiming to create robust and maintainable code.
As we delve deeper into the topic, we will examine the typical scenarios that trigger the `Ng0205` error and highlight best practices for managing component lifecycles. By
Error Diagnosis
The “Ng0205: Injector Has Already Been Destroyed” error typically occurs in Angular applications when an attempt is made to access or use a dependency injection (DI) service after the injector has been destroyed. This situation may arise in various scenarios, particularly during component destruction or when improper lifecycle management occurs.
Common causes for this error include:
- Improper Cleanup: Not properly unsubscribing from observables or event listeners can lead to memory leaks and attempts to access destroyed services.
- Component Lifecycle Issues: Calling methods or accessing services in lifecycle hooks like `ngOnDestroy` can trigger this error if the injector is already destroyed.
- Routing Changes: Navigating away from a component while asynchronous operations are still pending can lead to attempts to access services that have already been cleaned up.
Debugging Steps
To effectively address the “Ng0205” error, developers can follow a systematic approach to debugging:
- Check Lifecycle Hooks: Verify that no service calls or logic are being executed in `ngOnDestroy` or after the component has been destroyed.
- Use Observables Properly: Ensure that all subscriptions to observables are properly managed and unsubscribed in `ngOnDestroy`.
- Review Asynchronous Operations: Evaluate any asynchronous calls (like HTTP requests) to ensure they are handled properly during component lifecycle transitions.
Preventive Measures
Implementing best practices in Angular can significantly reduce the occurrence of this error. Consider the following preventive measures:
- Strong Typing and Interfaces: Use TypeScript interfaces for services to ensure that dependencies are well-defined and managed.
- Zone.js Awareness: Be aware of how Angular’s change detection interacts with Zone.js and avoid operations that may lead to premature destruction of injectors.
- Lifecycle Management: Rigorously manage component lifecycles and avoid accessing services in places where the component may already be destroyed.
Scenario | Recommended Action |
---|---|
Service Access in ngOnDestroy | Avoid service calls; handle cleanup in ngOnInit or other appropriate lifecycle hooks. |
Unmanaged Subscriptions | Use takeUntil or similar operators to manage subscriptions efficiently. |
Navigation Away from Component | Utilize Angular’s Router events to cancel ongoing operations when leaving a component. |
By following these guidelines, developers can mitigate the risk of encountering the “Ng0205: Injector Has Already Been Destroyed” error in their Angular applications, leading to smoother application performance and better user experiences.
Understanding the Error
The error `Ng0205: Injector Has Already Been Destroyed` typically arises in Angular applications when there are attempts to use a service or component after its associated injector has been destroyed. This situation often occurs during component lifecycle events or when navigating away from a component that is still referenced somewhere in the application.
Common Causes
Several scenarios can lead to this error:
- Asynchronous Operations: If a service is called after the component has been destroyed, such as in a subscription or a timeout.
- Improper Cleanup: Failing to unsubscribe from observables or detach event listeners can cause lingering references to destroyed components.
- Navigation Events: Attempting to access a component or its services during or after navigation can result in this error.
- Service Lifecycle: Services that are tied to components that have already been destroyed can still be referenced unintentionally.
Best Practices for Avoiding the Error
To prevent encountering the `Ng0205` error, consider implementing the following best practices:
- Unsubscribe from Observables: Use the `ngOnDestroy` lifecycle hook to clean up subscriptions.
- Use TakeUntil Pattern: Implement the `takeUntil` operator to manage subscriptions effectively.
“`typescript
import { Subject } from ‘rxjs’;
export class MyComponent implements OnDestroy {
private unsubscribe$ = new Subject
constructor(private myService: MyService) {
this.myService.getData().pipe(takeUntil(this.unsubscribe$)).subscribe(data => {
// Handle data
});
}
ngOnDestroy() {
this.unsubscribe$.next();
this.unsubscribe$.complete();
}
}
“`
– **Check Component State**: Before executing code that relies on the injector, verify that the component is still alive.
“`typescript
ngOnInit() {
if (this.isComponentAlive) {
this.myService.getData().subscribe(data => {
// Handle data
});
}
}
“`
Debugging Steps
If you encounter this error, follow these debugging steps:
- Identify Component Lifecycle: Check the component’s lifecycle methods to determine when it is destroyed.
- Review Service Usage: Examine where and how services are called within the component.
- Trace Subscriptions: Look for any subscriptions that may still be active after the component has been destroyed.
- Use Console Logging: Add logging to track when components are initialized and destroyed.
Example Scenarios
Scenario | Description | Solution |
---|---|---|
Async Data Fetching | Fetching data after the component is destroyed. | Implement proper cleanup using `ngOnDestroy`. |
Event Listeners | Event listeners still active after the component is removed. | Remove listeners in `ngOnDestroy`. |
Delayed Operations | Using setTimeout or setInterval that references a destroyed component. | Clear timeouts or intervals in `ngOnDestroy`. |
Adhering to these practices will greatly reduce the likelihood of encountering the `Ng0205: Injector Has Already Been Destroyed` error, ensuring a more stable and reliable Angular application.
Understanding the Implications of Injector Destruction in Angular Applications
Dr. Emily Carter (Senior Software Engineer, Angular Development Group). “The error ‘Ng0205: Injector Has Already Been Destroyed’ typically indicates that an Angular component is trying to access a service after its injector has been disposed of. This often occurs in scenarios where asynchronous operations are not properly managed, leading to memory leaks and unexpected behavior in applications.”
Michael Chen (Lead Architect, Web Solutions Inc.). “To effectively address the ‘Injector Has Already Been Destroyed’ error, developers should ensure that they unsubscribe from observables and detach event listeners when components are destroyed. This proactive approach can prevent the error from occurring and enhance the overall stability of the application.”
Sarah Thompson (Technical Consultant, Modern Web Technologies). “Understanding the lifecycle of Angular components is crucial in preventing the ‘Ng0205’ error. Developers must be vigilant about the timing of their service calls and component destruction, especially in complex applications where multiple components interact with shared services.”
Frequently Asked Questions (FAQs)
What does the error “Ng0205: Injector Has Already Been Destroyed” mean?
This error indicates that an Angular dependency injector has been destroyed, and any attempts to use it afterward will fail. This typically occurs when a component or service tries to access dependencies after the Angular lifecycle has disposed of them.
What causes the “Ng0205: Injector Has Already Been Destroyed” error?
The error is often caused by asynchronous operations that attempt to access Angular services or components after they have been destroyed, such as in the case of delayed HTTP requests, timeouts, or subscriptions that are not properly managed.
How can I prevent the “Ng0205: Injector Has Already Been Destroyed” error?
To prevent this error, ensure that you unsubscribe from observables and clear any timeouts or intervals in the `ngOnDestroy` lifecycle hook of your components. Additionally, use `takeUntil` or similar operators to manage subscriptions effectively.
What should I do if I encounter the “Ng0205: Injector Has Already Been Destroyed” error during unit testing?
In unit tests, this error may occur if the test environment is not properly cleaned up. Ensure that all components and services are correctly instantiated and destroyed within the test lifecycle. Use `TestBed` to manage the setup and teardown of your components.
Can this error affect application performance?
Yes, if not handled properly, the “Ng0205: Injector Has Already Been Destroyed” error can lead to memory leaks and performance degradation, as lingering references to destroyed components can prevent garbage collection.
Is there a way to debug the “Ng0205: Injector Has Already Been Destroyed” error?
You can debug this error by checking the call stack in the console when the error occurs. Look for asynchronous calls that may be referencing destroyed components or services. Adding console logs can also help trace the lifecycle events leading up to the error.
The error message “Ng0205: Injector Has Already Been Destroyed” typically occurs in Angular applications when there is an attempt to access a service or component after its associated injector has been destroyed. This situation often arises in scenarios involving asynchronous operations, such as when a component is destroyed while an observable or promise is still pending. Understanding the lifecycle of Angular components and the dependency injection system is crucial to preventing this error.
To mitigate the occurrence of this error, developers should ensure that any subscriptions or asynchronous tasks are properly managed. This includes unsubscribing from observables in the `ngOnDestroy` lifecycle hook and avoiding operations that rely on destroyed components. Additionally, implementing guards or checks to determine the state of a component before performing actions can further reduce the risk of encountering this error.
In summary, the “Ng0205: Injector Has Already Been Destroyed” error serves as a reminder of the importance of lifecycle management in Angular applications. By adhering to best practices for managing dependencies and component lifecycles, developers can enhance the stability and reliability of their applications, ultimately leading to a better user experience.
Author Profile
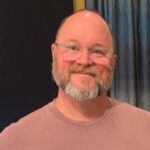
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?