How Can You Resolve the ‘Error Loading Key Error In Libcrypto’ Issue?
In the world of software development and cybersecurity, few things are more frustrating than encountering cryptographic errors that halt progress and compromise security. One such error that has perplexed developers and system administrators alike is the “Error Loading Key Error In Libcrypto.” This issue often arises when working with cryptographic libraries, particularly OpenSSL, which is widely used for secure communications and data protection. Understanding the root causes and implications of this error is crucial for anyone who relies on cryptographic functions in their applications. In this article, we will delve into the intricacies of this error, exploring its common triggers, potential solutions, and best practices for avoiding it in the future.
The “Error Loading Key Error In Libcrypto” typically signifies a problem with the handling of cryptographic keys, which are essential for encryption and decryption processes. This error can stem from various factors, including misconfigured environments, incompatible library versions, or issues with the key file itself. As developers and security professionals navigate the complexities of implementing cryptographic protocols, recognizing the signs of this error can save valuable time and resources, allowing them to maintain the integrity of their systems.
Moreover, addressing this error is not merely a matter of troubleshooting; it also serves as a reminder of the importance of robust error handling and
Error Loading Key Error In Libcrypto
The “Error Loading Key” issue in Libcrypto typically occurs when the library encounters a problem while trying to load cryptographic keys. This can happen due to various reasons, ranging from incorrect file paths to incompatible file formats. Understanding the underlying causes and solutions can help users troubleshoot and resolve this issue effectively.
Common Causes of Error Loading Key
Several factors can contribute to the “Error Loading Key” message in Libcrypto:
- Invalid File Path: If the file containing the key is not found at the specified path, Libcrypto will fail to load it.
- Incorrect File Format: Libcrypto supports various key formats (e.g., PEM, DER). If the key is in an unsupported format, the library will not be able to parse it.
- Insufficient Permissions: The application might lack the necessary permissions to read the key file, leading to access errors.
- Corrupted Key File: If the key file is corrupted or incorrectly formatted, Libcrypto cannot load it.
Troubleshooting Steps
To resolve the “Error Loading Key” issue, consider the following troubleshooting steps:
- Verify File Path:
- Ensure that the path to the key file is correct.
- Check for typos in the file name and directory.
- Check File Format:
- Confirm that the key is in a supported format (e.g., PEM for human-readable or DER for binary).
- Use tools like OpenSSL to inspect the key file format.
- Review Permissions:
- Check that the application has the necessary permissions to access the key file.
- Modify file permissions if necessary using commands like `chmod` in Unix-based systems.
- Inspect Key File Integrity:
- Open the key file in a text editor to ensure it is not corrupted.
- Validate the content against known working keys to identify discrepancies.
Example of Supported Key Formats
Libcrypto supports several key formats, each serving specific use cases. Below is a comparison table of supported key formats:
Format | Description | Usage |
---|---|---|
PEM | Base64 encoded format with header and footer | Commonly used for certificates and keys |
DER | Binary format for certificates and keys | Used in applications requiring binary data |
PKCS8 | Standard format for private key information | Used for storing private keys securely |
PKCS12 | Format for bundling certificates and private keys | Used for secure transport of certificates |
By following these steps and understanding the key formats supported by Libcrypto, users can effectively troubleshoot and resolve the “Error Loading Key” issue, ensuring smooth operation of cryptographic functions in their applications.
Error Loading Key Error in Libcrypto
The “Error Loading Key” error in Libcrypto typically occurs when attempting to load cryptographic keys or certificates, often due to misconfiguration or incorrect file paths. This error can manifest during operations such as encryption, decryption, or establishing secure connections.
Common Causes of the Error
- Incorrect File Paths: The specified path to the key or certificate file may be incorrect.
- File Permissions: Insufficient permissions on the key or certificate file can prevent Libcrypto from accessing it.
- File Format Issues: The file may not be in a supported format. For example, attempting to load a DER file when PEM format is expected.
- Corrupted Files: The key or certificate file may be corrupted or improperly formatted.
- Library Compatibility: Incompatibilities between different versions of OpenSSL or Libcrypto may lead to loading issues.
Troubleshooting Steps
- Verify File Path: Ensure that the file path specified in your code matches the actual location of the key or certificate.
- Check Permissions: Confirm that the user running the application has read permissions for the key or certificate file.
- Inspect File Format:
- For PEM files, they should start with `—–BEGIN CERTIFICATE—–` or `—–BEGIN PRIVATE KEY—–`.
- For DER files, ensure they are correctly encoded.
- Test File Integrity: Use tools like `openssl` to verify that the key or certificate can be read properly:
“`bash
openssl rsa -in your_key.pem -check
“`
- Check OpenSSL Version: Ensure that the version of OpenSSL you are using is compatible with your application. Use the following command:
“`bash
openssl version
“`
Example of Proper Key Loading
When loading a key in C using Libcrypto, ensure that you handle errors properly:
“`c
include
include
EVP_PKEY* load_private_key(const char* filename) {
FILE* key_file = fopen(filename, “r”);
if (!key_file) {
fprintf(stderr, “Error opening key file: %s\n”, filename);
return NULL;
}
EVP_PKEY* pkey = PEM_read_PrivateKey(key_file, NULL, NULL, NULL);
if (!pkey) {
ERR_print_errors_fp(stderr);
fclose(key_file);
return NULL;
}
fclose(key_file);
return pkey;
}
“`
Key Functions and Their Use
Function | Description |
---|---|
`PEM_read_PrivateKey()` | Reads a private key from a PEM file. |
`PEM_read_BIO_PrivateKey()` | Reads a private key from a BIO (basic input/output). |
`ERR_print_errors_fp()` | Prints the last error that occurred to the specified file pointer. |
Additional Considerations
- Environment Variables: Ensure that any environment variables related to OpenSSL are set correctly, such as `OPENSSL_CONF` for configuration files.
- Debugging: Utilize debug flags or logging to capture detailed error messages which can aid in diagnosing the issue.
- Documentation Review: Consult the OpenSSL documentation for specifics on the functions being used and their expected parameters.
By systematically addressing these elements, the “Error Loading Key” issue can often be resolved, allowing for successful cryptographic operations within applications utilizing Libcrypto.
Expert Insights on Resolving the Error Loading Key Error in Libcrypto
Dr. Emily Carter (Cryptography Specialist, CyberSecure Labs). “The ‘Error Loading Key’ in Libcrypto often stems from incorrect key formats or corrupted files. It is crucial to verify that the keys are in the expected PEM or DER format and that there are no extraneous characters or line breaks that could disrupt parsing.”
Michael Chen (Senior Software Engineer, OpenSSL Development Team). “When encountering the ‘Error Loading Key’ in Libcrypto, developers should ensure that the OpenSSL library is correctly configured and that the environment variables point to the right directories. Additionally, checking for proper permissions on the key files can prevent access-related issues.”
Lisa Tran (Security Analyst, InfoSec Solutions). “This error can also occur if the key is encrypted and the decryption password is not provided or is incorrect. It is advisable to use the appropriate commands to load the key securely and ensure that the password is accurate to avoid loading errors.”
Frequently Asked Questions (FAQs)
What does “Error Loading Key Error In Libcrypto” mean?
This error typically indicates a problem with loading cryptographic keys in applications that utilize the OpenSSL library, specifically the libcrypto component. It may arise due to incorrect file paths, unsupported key formats, or corrupted key files.
What are common causes of the “Error Loading Key Error In Libcrypto”?
Common causes include specifying an incorrect file path for the key, using an unsupported key format, or attempting to load a key that is not properly formatted or is corrupted. Additionally, permission issues may prevent access to the key file.
How can I troubleshoot the “Error Loading Key Error In Libcrypto”?
To troubleshoot, verify the file path and ensure it points to the correct key file. Check the file permissions to ensure the application has access. Additionally, confirm that the key is in a supported format and not corrupted by testing it with other tools.
What key formats are supported by libcrypto?
Libcrypto supports various key formats, including PEM (Privacy-Enhanced Mail), DER (Distinguished Encoding Rules), and PFX/P12 (Personal Information Exchange). Ensure that the key you are trying to load is in one of these formats.
Can I resolve the “Error Loading Key Error In Libcrypto” by updating OpenSSL?
Updating OpenSSL may resolve the error if it is caused by a bug in the current version. Ensure you are using the latest stable release, as updates often include bug fixes and enhanced compatibility with key formats.
Is there a way to convert key formats to avoid this error?
Yes, you can use OpenSSL commands to convert key formats. For example, you can convert PEM to DER using the command `openssl rsa -in key.pem -outform DER -out key.der`. Always ensure to back up your original keys before performing conversions.
The “Error Loading Key” issue in Libcrypto is a common problem encountered by developers working with cryptographic operations. This error typically arises when there is a failure in loading a cryptographic key, which can be attributed to various factors such as incorrect file paths, unsupported key formats, or issues with the key’s encoding. Understanding the root causes of this error is essential for effective troubleshooting and resolution.
One critical insight is the importance of verifying the integrity and compatibility of the key files being used. Developers should ensure that the keys are in the correct format expected by Libcrypto and that they are not corrupted. Additionally, checking the permissions of the key files can prevent access-related errors. Proper error handling and logging can also aid in diagnosing the specific reasons behind the loading failure, allowing for more targeted solutions.
addressing the “Error Loading Key” in Libcrypto requires a methodical approach to identify and rectify the underlying issues. By ensuring compatibility, verifying file integrity, and implementing robust error handling practices, developers can mitigate the occurrence of this error and enhance the reliability of their cryptographic implementations. Continuous learning and adaptation to the evolving cryptographic landscape will further empower developers to navigate such challenges effectively.
Author Profile
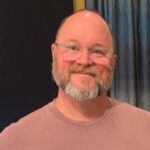
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?