How Does the ‘Do While’ Loop Work in Python 3?
In the world of programming, control flow structures are essential for executing code efficiently and effectively. Among these structures, loops play a pivotal role in automating repetitive tasks, allowing developers to write cleaner and more maintainable code. One such loop, the “Do While” loop, is a powerful construct that ensures a block of code is executed at least once before any condition is evaluated. While Python does not have a built-in “Do While” loop like some other programming languages, understanding its principles can enhance your coding skills and broaden your approach to problem-solving.
In Python, the concept of a “Do While” loop can be simulated using a combination of other control flow statements. This approach not only provides flexibility but also encourages developers to think creatively about how to implement similar functionality. By exploring the mechanics of this loop, you’ll discover how to manage execution flow based on conditions and gain insights into alternative looping techniques available in Python.
As we delve deeper into the nuances of “Do While” loops in Python, you’ll learn how to effectively structure your code to ensure that specific tasks are performed repeatedly until a condition is met. Whether you’re a beginner looking to grasp fundamental concepts or an experienced coder seeking to refine your skills, this exploration will equip you with the tools to harness the power
Understanding the Do While Loop in Python
In Python, there is no built-in `do while` loop construct as found in some other programming languages like C or Java. However, the same functionality can be achieved using a combination of a `while` loop and a `break` statement. The essence of a `do while` loop is that it guarantees the execution of the loop body at least once before evaluating the condition.
To replicate this behavior in Python, one can structure the loop as follows:
“`python
while True:
Code to execute
if not condition:
break
“`
This structure allows the loop to run at least once and then check the condition to determine whether to continue or exit the loop.
Implementation Example
Consider the following example where we want to prompt a user for input until they provide a valid number:
“`python
while True:
user_input = input(“Enter a number (0 to exit): “)
if user_input.isdigit():
number = int(user_input)
print(f”You entered: {number}”)
if number == 0:
break
else:
print(“Invalid input. Please try again.”)
“`
In this example:
- The loop will continue prompting the user until they enter a valid number.
- If the user inputs `0`, the loop will exit, demonstrating the `do while` behavior.
Comparison with Traditional While Loop
The conventional `while` loop in Python checks the condition before executing the loop body, which may lead to situations where the loop body never executes. Here’s a comparison:
Feature | Do While Equivalent | Traditional While Loop |
---|---|---|
Execution Guarantee | Executes at least once | May not execute at all |
Condition Check | After executing the body | Before executing the body |
Advantages of Using Do While Style
- Guaranteed Execution: Ensures that the loop body runs at least once, which is useful for scenarios where initial input or action is necessary.
- Simpler Logic for User Prompts: When validating user input, a `do while` style allows for immediate feedback without needing to check conditions before the first execution.
Common Use Cases
The `do while` loop style can be particularly useful in various scenarios, including:
- User input validation
- Menu-driven applications where an option must be selected at least once
- Situations requiring an initial setup before checking for exit conditions
Implementing a `do while` loop in Python effectively requires an understanding of flow control and the use of loops, ensuring that the code remains clean and efficient while meeting the requirements of the task.
Understanding the Do While Loop in Python 3
Python does not have a built-in `do while` loop construct as found in some other programming languages. However, similar functionality can be achieved using a combination of a `while` loop and a condition check that ensures the loop executes at least once.
Simulating a Do While Loop
To replicate the behavior of a `do while` loop, you can use a `while` loop with an initial execution block that allows the loop body to run at least once before checking the condition. Here’s a basic structure:
“`python
condition = True Initial condition
while True: Infinite loop
Code to execute
print(“This will run at least once.”)
Update the condition
condition = check_condition() Replace with your condition logic
if not condition: Exit if condition is
break
“`
Example of a Simulated Do While Loop
Here is an example that demonstrates how to use this approach:
“`python
count = 0
while True:
count += 1
print(f”Count is: {count}”) This will execute at least once
if count >= 5: Condition to exit
break
“`
In this example, the loop increments the `count` variable and prints its value until it reaches 5.
When to Use a Simulated Do While Loop
The simulated `do while` loop is particularly useful in scenarios where:
- An action must be performed at least once, such as user input validation.
- The termination condition relies on the outcome of the loop’s execution.
- The logic requires a guaranteed initial execution before evaluating the condition.
Comparison with Other Loop Constructs
Understanding the differences between various loop constructs in Python can help determine when to use a simulated `do while` loop. Here’s a comparison:
Loop Type | Execution Guarantee | Condition Check Location |
---|---|---|
`for` loop | None | Before the first iteration |
`while` loop | None | Before each iteration |
Simulated `do while` | At least once | After the first iteration |
Considerations and Best Practices
When implementing a simulated `do while` loop, consider the following best practices:
- Ensure that the loop has a clear exit condition to prevent infinite loops.
- Keep the code within the loop concise to maintain readability.
- Document the purpose of the loop and its exit condition for future reference.
Utilizing the simulated `do while` loop effectively can enhance code clarity and functionality in Python 3, allowing for more controlled execution flows.
Understanding the Do While Loop in Python 3
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “While Python does not have a built-in ‘do while’ loop, developers can mimic this behavior using a combination of a ‘while’ loop and an initial conditional check. This approach allows for executing a block of code at least once before evaluating the condition, which is essential in certain programming scenarios.”
Michael Chen (Lead Software Engineer, CodeCraft Solutions). “The absence of a native ‘do while’ construct in Python 3 often leads to confusion among programmers transitioning from languages that support it. Understanding how to implement similar functionality using ‘while True’ and ‘break’ statements is crucial for writing effective Python code.”
Sarah Thompson (Python Educator, LearnPythonOnline). “For beginners, grasping the concept of a ‘do while’ loop can enhance their problem-solving skills. By using a ‘while’ loop with a post-condition check, learners can appreciate the importance of executing code blocks based on user input or other conditions, reinforcing their understanding of control flow in programming.”
Frequently Asked Questions (FAQs)
What is a “do while” loop in Python 3?
A “do while” loop is a control flow statement that executes a block of code at least once and then repeats the execution as long as a specified condition is true. Python does not have a built-in “do while” loop, but it can be simulated using a `while` loop.
How can I implement a “do while” loop in Python 3?
You can simulate a “do while” loop in Python by using a `while` loop with a condition that is checked after the loop body. For example:
“`python
while True:
Your code here
if not condition:
break
“`
What is the difference between a “do while” loop and a “while” loop in Python 3?
The primary difference is that a “do while” loop guarantees at least one execution of the loop body before checking the condition, while a “while” loop checks the condition before executing the loop body, potentially resulting in zero executions.
Can you provide an example of a “do while” loop simulation in Python 3?
Certainly. Here is an example:
“`python
count = 0
while True:
print(count)
count += 1
if count >= 5:
break
“`
This code prints numbers from 0 to 4, simulating a “do while” loop.
What are the common use cases for a “do while” loop in programming?
Common use cases include scenarios where user input is required, menu-driven programs, and situations where at least one execution of the loop is necessary before evaluating the condition.
Are there any performance considerations when using a simulated “do while” loop in Python 3?
While simulating a “do while” loop is straightforward, it may introduce slight overhead due to the additional condition check at the end. However, this is generally negligible unless used in performance-critical applications.
In Python 3, the traditional “do while” loop structure, commonly found in other programming languages, is not directly available. However, developers can achieve similar functionality using a combination of a “while” loop and a conditional statement. The essence of a “do while” loop is that it guarantees the execution of the loop body at least once before checking the condition, which can be effectively simulated in Python by placing the loop body before the condition check.
To implement a “do while” style loop in Python, one can initialize a variable or set up a loop that continues until a specified condition is met. This approach allows for flexibility and control over the loop’s execution flow. By using a `while True` loop in conjunction with a break statement, developers can replicate the behavior of a “do while” loop, ensuring that the loop executes at least once regardless of the condition.
Key takeaways include the understanding that while Python does not have a built-in “do while” loop, the language’s flexibility allows for alternative methods to achieve the same outcome. This encourages developers to think creatively about control flow and reinforces the importance of understanding the underlying logic of loops in programming. Mastering these techniques can enhance coding efficiency and adaptability in
Author Profile
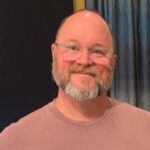
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?