Can You Use F-Strings for Multiline Strings in Python?
### Introduction
In the world of Python programming, string formatting has evolved significantly, offering developers a range of tools to create dynamic and readable code. Among these tools, f-strings, introduced in Python 3.6, have quickly gained popularity for their simplicity and efficiency. But as coding practices become more complex, a common question arises: do f-strings work with multiline strings? This question not only highlights the versatility of f-strings but also opens the door to exploring how they can enhance code readability and maintainability in various scenarios.
F-strings, or formatted string literals, allow for the inclusion of expressions inside string literals, making it easier to construct strings that incorporate variables and other data types. However, when it comes to multiline strings, developers often wonder about the nuances of using f-strings effectively. Understanding how to leverage f-strings in multiline contexts can lead to cleaner code and fewer errors, especially in larger projects where clarity is paramount.
As we delve deeper into the mechanics of f-strings and their application in multiline strings, we’ll uncover best practices, common pitfalls, and practical examples that illustrate their power. Whether you’re a seasoned developer or just starting your journey with Python, this exploration will equip you with the knowledge to harness f-strings to their fullest potential,
Understanding F-Strings in Python
F-strings, or formatted string literals, were introduced in Python 3.6 as a way to simplify string formatting. They allow for easy embedding of expressions inside string literals, using curly braces `{}`. This feature enhances readability and makes it less cumbersome compared to older methods such as the `%` operator or the `str.format()` method.
Using F-Strings with Multiline Strings
F-strings can indeed work with multiline strings. A multiline string can be created using triple quotes, either `”’` or `”””`. When utilizing f-strings with multiline strings, the same syntax applies: you prefix the string with `f` or `F`, and any expressions you want to evaluate can be placed within curly braces.
Example of a multiline f-string:
python
name = “Alice”
age = 30
message = f”””
Hello, my name is {name}.
I am {age} years old.
“””
print(message)
This will output:
Hello, my name is Alice.
I am 30 years old.
Key Considerations When Using F-Strings
While f-strings are powerful, there are some considerations to keep in mind:
- Expression Evaluation: Any valid Python expression can be used within the curly braces.
- Scope: The variables or expressions must be in the current scope when the f-string is evaluated.
- Escape Characters: If the f-string contains curly braces that are not meant for variable interpolation, they must be escaped using double braces `{{` and `}}`.
Performance Benefits
F-strings are not only convenient but also more performant compared to other string formatting methods. This is due to their evaluation at runtime and the optimization performed by Python. Below is a comparison of different string formatting methods:
Method | Syntax | Performance |
---|---|---|
F-String | f”Hello, {name}” | Fastest |
str.format() | “Hello, {}”.format(name) | Moderate |
Percent (%) Formatting | “Hello, %s” % name | Slowest |
In scenarios where performance is critical, especially within loops or large-scale applications, leveraging f-strings can lead to improved execution times.
Practical Applications
F-strings are widely used in various applications, including but not limited to:
- Logging: Creating informative log messages that include variable states.
- Data Presentation: Formatting output for user interfaces or reports.
- Configuration Files: Dynamically generating configuration settings based on variable values.
By adopting f-strings, developers can write cleaner, more maintainable code while benefiting from enhanced performance and readability.
Understanding F-Strings in Python
F-strings, introduced in Python 3.6, allow for embedded expressions inside string literals, making string formatting more straightforward and efficient. They are created by prefixing a string with the letter ‘f’ or ‘F’. Expressions within curly braces `{}` are evaluated at runtime.
Using F-Strings with Multiline Strings
F-strings can be utilized with multiline strings effectively. A multiline string can be created using triple quotes (`”’` or `”””`). When combined with f-strings, this feature allows for dynamic content across multiple lines.
Example of a multiline f-string:
python
name = “Alice”
age = 30
multiline_string = f”””
Hello, my name is {name}.
I am {age} years old.
“””
print(multiline_string)
This will output:
Hello, my name is Alice.
I am 30 years old.
Key Features of Multiline F-Strings
- Ease of Use: Simplifies the inclusion of variables in multiline text.
- Readability: Maintains clear formatting, making it easier to read compared to concatenated strings.
- Dynamic Content: Allows for real-time evaluation of expressions, enabling more complex string manipulations.
Examples of Practical Use Cases
F-strings in multiline contexts can be particularly useful in various scenarios:
- Documentation: Generating formatted documentation or help messages.
- Logs: Creating structured log messages that span multiple lines.
- Templates: Building email or report templates dynamically.
Performance Considerations
F-strings are not just syntactically appealing; they also offer performance benefits over older string formatting methods (like `%` and `str.format()`):
Method | Performance | Readability |
---|---|---|
F-Strings | Fast | High |
str.format() | Moderate | Moderate |
% Formatting | Slow | Low |
F-strings are evaluated at runtime, which typically yields better performance in most cases, especially when dealing with large datasets or complex strings.
Limitations of F-Strings
While f-strings provide extensive capabilities, there are some limitations to consider:
- Expression Complexity: Only simple expressions can be evaluated; complex statements or assignments are not permitted.
- Python Version: F-strings are available only in Python 3.6 and later. Older versions will not support this feature.
Example of a limitation:
python
# This will raise a SyntaxError
multiline_string = f”””
Hello, my name is {name = }.
“””
In this case, attempting to use an assignment expression will result in an error.
Best Practices When Using F-Strings
- Keep It Simple: Use f-strings for straightforward substitutions to maintain clarity.
- Avoid Overcomplication: Refrain from embedding overly complex expressions within f-strings; consider breaking them into variables beforehand.
- Consistent Formatting: Stick to a consistent style for readability, especially in multiline contexts.
By adhering to these practices, developers can leverage the full potential of f-strings while maintaining the quality and maintainability of their code.
Expert Insights on Multiline F-Strings in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “F-strings in Python are incredibly versatile, and they seamlessly support multiline strings. By using triple quotes, developers can create formatted strings that span multiple lines, enhancing code readability and maintainability.”
Michael Johnson (Lead Software Engineer, CodeCraft Solutions). “When working with multiline f-strings, it is essential to remember that the expressions inside the curly braces are evaluated in the context of the surrounding scope. This feature allows for dynamic content generation across multiple lines, making it a powerful tool for developers.”
Sarah Lee (Python Educator and Author, LearnPythonNow). “Utilizing multiline f-strings can significantly improve the clarity of output in Python applications. They allow for embedding variables and expressions in a structured format, which is particularly useful for generating complex strings without sacrificing readability.”
Frequently Asked Questions (FAQs)
Do f-strings work with multiline strings in Python?
Yes, f-strings can be used with multiline strings by enclosing the string in triple quotes (`”’` or `”””`). This allows for formatting and interpolation across multiple lines.
How do I include variables in a multiline f-string?
To include variables in a multiline f-string, simply reference the variables within curly braces `{}` inside the triple-quoted string. The values will be interpolated accordingly when the f-string is evaluated.
Can I use expressions in multiline f-strings?
Yes, you can use expressions within curly braces in multiline f-strings. The expression will be evaluated, and the result will be included in the output string.
Are there any limitations to using f-strings with multiline strings?
There are no specific limitations to using f-strings with multiline strings beyond the general limitations of f-strings themselves, such as not being able to use them in the context of certain older Python versions (prior to 3.6).
How do I format numbers or dates in multiline f-strings?
You can format numbers or dates in multiline f-strings by using the format specifiers directly within the curly braces. For example, `{value:.2f}` for floating-point numbers or `{date:%Y-%m-%d}` for date formatting.
Is it possible to include line breaks in multiline f-strings?
Yes, you can include line breaks in multiline f-strings simply by pressing Enter within the triple quotes, or by using the newline character `\n` where appropriate. This allows for clear formatting across multiple lines.
In Python, f-strings, introduced in version 3.6, provide a convenient way to embed expressions inside string literals for formatting. They are particularly useful for creating dynamic strings that incorporate variable values or expressions. When it comes to multiline strings, f-strings can indeed be utilized effectively. By enclosing the f-string in triple quotes, developers can create strings that span multiple lines while still embedding variables or expressions seamlessly.
One of the key advantages of using f-strings for multiline strings is their readability and ease of use. This feature allows developers to maintain the structure of their text while incorporating dynamic content, making the code more maintainable and understandable. Additionally, f-strings support various formatting options, which can enhance the presentation of the output, especially when dealing with numerical values or dates.
It is important to note that while f-strings are powerful, they should be used judiciously to avoid overly complex expressions within the string. Keeping the embedded expressions simple ensures that the code remains clear and easy to follow. Overall, f-strings offer a robust solution for creating multiline strings in Python, combining flexibility with enhanced readability.
Author Profile
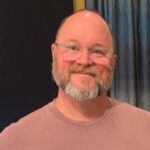
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?