Why Am I Seeing ‘Django.Core.Exceptions.AppRegistryNotReady: Apps Aren’t Loaded Yet’ and How Can I Fix It?
In the dynamic world of web development, Django stands out as a powerful framework that simplifies the creation of robust applications. However, even the most seasoned developers can encounter frustrating errors that disrupt their workflow. One such error, `Django.Core.Exceptions.AppRegistryNotReady: Apps Aren’t Loaded Yet`, can leave you scratching your head and searching for solutions. Understanding this error is crucial for anyone looking to harness the full potential of Django, as it often signals deeper issues within your application’s structure and initialization process.
The `AppRegistryNotReady` exception typically arises when Django’s application registry has not been fully loaded before an attempt is made to access application-specific functionality. This situation can occur during various stages of development, particularly when dealing with models, signals, or database queries that depend on the app’s configuration. Developers may encounter this error during the startup of their application or when running management commands, leading to confusion and delays in project timelines.
To effectively tackle this issue, it’s essential to grasp the underlying principles of Django’s app loading mechanism. By delving into the causes and implications of the `AppRegistryNotReady` exception, developers can not only resolve the immediate problem but also gain insights into best practices for structuring their Django projects. This knowledge will empower them to
Understanding the Error
The error `Django.Core.Exceptions.AppRegistryNotReady: Apps aren’t loaded yet` typically occurs when Django is unable to access its application registry during startup or when an operation that requires the application registry is attempted before it is fully loaded. This situation often arises in the context of using Django’s ORM (Object-Relational Mapping) or during the initialization of models and signals.
Common Causes
Several scenarios can lead to this error:
- Improper Import Timing: If you attempt to import models or access the database before Django has finished initializing its app registry, you will encounter this error. This often happens in the following contexts:
- Importing models at the top level of a module.
- Using Django’s ORM in `settings.py` or during module-level initialization.
- Circular Imports: Circular dependencies between modules can lead to situations where one module tries to load another before Django has had a chance to fully initialize its application registry.
- Running Scripts or Commands: Executing scripts or management commands that access models without the proper setup can trigger this error. This includes standalone scripts or certain custom management commands.
Solutions and Best Practices
To resolve the `AppRegistryNotReady` error, consider the following strategies:
- Use Django’s `apps` Module: If you need to access models in a context that might not be fully loaded, use `django.apps.apps.get_model()` instead of direct imports.
- Delay Imports: Move imports that access models inside functions or methods to ensure they only execute when the Django environment is fully set up. For example:
“`python
Instead of this:
from myapp.models import MyModel
Use this:
def my_function():
from myapp.models import MyModel
Use MyModel here
“`
- Check for Circular Imports: Refactor your code to eliminate circular dependencies. This might involve breaking down large modules or re-structuring the project layout.
Example of Proper Setup
A correctly set up Django application should ensure that all imports and database accesses occur after the app registry is fully initialized. Below is a table highlighting some common patterns and their alternatives.
Problematic Pattern | Recommended Pattern |
---|---|
Global model imports | Function-level imports |
Using `MyModel.objects.all()` in `settings.py` | Deferring to a signal or a management command |
Accessing models in `__init__.py` files | Using Django’s `ready()` method in the app config |
By adhering to these practices, you can prevent the `AppRegistryNotReady` error and ensure that your Django application initializes correctly.
Understanding the Error
The error `Django.Core.Exceptions.AppRegistryNotReady: Apps aren’t loaded yet` occurs when Django attempts to use application models or components before the application registry is fully initialized. This can happen in several scenarios, particularly during the early stages of application startup or when using signals.
Common Causes
Several situations can lead to this error:
- Improper Import Timing: Models or components are imported too early in the application lifecycle.
- Signals Setup: Signals are connected in a way that they reference models before the app registry is ready.
- Circular Imports: Code that causes circular dependencies can lead to premature execution of code that relies on the app registry.
Troubleshooting Steps
To resolve this issue, consider the following steps:
- Delay Imports: Ensure that models and other components are imported only after the Django application is fully loaded.
- Use Django’s `AppConfig.ready()` method to import models or setup signals.
- Check Signal Connections: Review signal connections to ensure they are set up correctly.
- Move signal connections to the `ready()` method of the app’s configuration class.
- Avoid Circular Imports: Refactor your code to eliminate circular dependencies.
- Use local imports within functions instead of global imports at the module level.
Example Fixes
Here are examples of how to implement the suggested troubleshooting steps:
Delaying Imports Using `ready()`:
“`python
apps.py
from django.apps import AppConfig
class MyAppConfig(AppConfig):
name = ‘myapp’
def ready(self):
import myapp.signals Import signals here
“`
Setting Up Signals Correctly:
“`python
signals.py
from django.db.models.signals import post_save
from django.dispatch import receiver
from .models import MyModel
@receiver(post_save, sender=MyModel)
def my_handler(sender, instance, created, **kwargs):
Handler logic here
“`
Prevention Tips
To avoid encountering the `AppRegistryNotReady` error in the future, consider the following practices:
- Use `AppConfig`: Always define your applications using `AppConfig` and set up signals within the `ready()` method.
- Organize Imports: Keep model imports within the relevant methods instead of importing them globally.
- Testing: Regularly test your application to catch import-related issues early in the development cycle.
Reference Links
For further reading and official documentation, consider the following resources:
Topic | Link |
---|---|
Django AppConfig Documentation | [Django Documentation](https://docs.djangoproject.com/en/stable/ref/applications/django.apps.AppConfig) |
Django Signals Documentation | [Django Documentation](https://docs.djangoproject.com/en/stable/topics/signals/) |
Ensuring proper application structure and import timing will significantly reduce the likelihood of encountering this error in Django development.
Understanding the Django AppRegistryNotReady Exception
Dr. Emily Carter (Senior Software Engineer, DjangoCore Solutions). “The ‘AppRegistryNotReady’ exception typically arises when attempting to access models or perform database operations before Django has fully initialized all applications. It is crucial to ensure that any database interactions are deferred until the application is completely loaded.”
Mark Thompson (Lead Developer, WebApp Innovations). “To avoid the ‘Apps Aren’t Loaded Yet’ error, developers should encapsulate their code in functions that are called after the Django application is ready. Utilizing signals or the ready method in AppConfig can help mitigate this issue effectively.”
Lisa Chen (Django Consultant, TechSavvy Solutions). “This exception serves as a reminder of Django’s application lifecycle. Understanding when and how apps are loaded is essential for developers to prevent premature access to models or other app components.”
Frequently Asked Questions (FAQs)
What does the error “Django.Core.Exceptions.Appregistrynotready: Apps Aren’t Loaded Yet” mean?
This error indicates that the Django application is attempting to access models or perform operations before the application registry is fully initialized. This typically occurs during the startup phase of a Django project.
What causes the “Apps Aren’t Loaded Yet” error in Django?
The error is often caused by importing models or executing database queries too early in the application lifecycle, such as in the global scope of a module or before the Django setup has completed.
How can I resolve the “Apps Aren’t Loaded Yet” error?
To resolve this error, ensure that any imports or database interactions involving models occur within functions or methods, rather than at the module level. Additionally, check that your Django settings and application configurations are correctly set up.
Is this error common in Django development?
Yes, this error is relatively common, especially for developers who are new to Django or when making changes to the project structure. It serves as a reminder to respect the application lifecycle.
Can third-party packages cause the “Apps Aren’t Loaded Yet” error?
Yes, third-party packages can also trigger this error if they attempt to access Django models or settings before the application is fully loaded. It is important to review the documentation of any third-party packages for proper usage.
What should I check if I encounter this error during testing?
If you encounter this error during testing, verify that your test cases are properly set up and that any model-related code is executed within the test methods, not at the module level. Additionally, ensure that the test runner is correctly configured.
The error “Django.Core.Exceptions.AppRegistryNotReady: Apps aren’t loaded yet” typically arises in Django applications when there is an attempt to access the application registry before it has been fully initialized. This situation often occurs during the startup phase of a Django project, particularly when trying to import models or access application-specific settings too early in the process. Understanding the timing of when Django applications are loaded is crucial for developers to avoid this common pitfall.
One of the primary causes of this error is the improper placement of import statements or function calls that rely on the app registry. Developers should ensure that such calls are made after the Django setup is complete, which is typically after the `django.setup()` function is called. Additionally, it is essential to review the project structure and the order of imports to prevent premature access to the models or settings that depend on the app registry being fully loaded.
Key takeaways include the importance of adhering to Django’s application lifecycle and understanding the implications of the app registry. Developers should familiarize themselves with Django’s startup process and utilize the appropriate hooks, such as signals or the `ready()` method in application configuration, to safely access models and other app components. By following these best practices, developers can mitigate the risk of
Author Profile
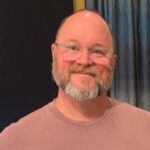
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?