How Do You Declare a Constant in Java?
In the world of programming, constants play a crucial role in maintaining the integrity and readability of code. Java, a language renowned for its robustness and versatility, offers developers the ability to declare constants that can enhance both performance and clarity in their applications. Whether you’re a seasoned programmer or just starting your journey in coding, understanding how to effectively declare and utilize constants in Java is essential for writing clean, maintainable code. This article will guide you through the nuances of constants in Java, shedding light on their significance and the best practices for implementation.
Declaring a constant in Java is a straightforward process, yet it carries profound implications for how your code behaves. Constants are immutable values that, once assigned, cannot be altered throughout the program’s execution. This immutability not only helps prevent accidental changes to critical values but also makes your code easier to understand and debug. By using constants, developers can avoid the pitfalls of magic numbers and hard-coded values, leading to a more organized and professional coding style.
In Java, constants are typically declared using the `final` keyword, which signifies that the variable’s value cannot be modified after its initial assignment. This feature is particularly useful in scenarios where certain values, such as mathematical constants or configuration settings, should remain unchanged. As we delve deeper into the
Declaring Constants in Java
In Java, constants are declared using the `final` keyword, which indicates that the variable’s value cannot be changed once it has been assigned. This is particularly useful for values that should remain unchanged throughout the execution of a program, such as mathematical constants, configuration values, or fixed settings.
To declare a constant, you typically follow this syntax:
“`java
final dataType CONSTANT_NAME = value;
“`
Example of Constant Declaration
Here’s a simple example of declaring a constant in Java:
“`java
final int MAX_USERS = 100;
final String COMPANY_NAME = “Tech Solutions”;
“`
In this example:
- `MAX_USERS` is a constant of type `int`, representing the maximum number of users allowed.
- `COMPANY_NAME` is a constant of type `String`, which holds the name of the company.
Naming Conventions
When declaring constants in Java, it is customary to use uppercase letters with underscores separating words. This convention helps distinguish constants from regular variables.
Benefits of Using Constants
Using constants provides several advantages:
- Readability: Constants make the code more readable and self-documenting.
- Maintainability: If a constant value needs to change, you only need to update it in one place.
- Error Prevention: Constants help prevent accidental modifications of fixed values.
Constant Types
Constants can be declared for various data types, including:
- Primitive types (e.g., `int`, `double`, `char`)
- Reference types (e.g., `String`, arrays, objects)
Table of Constant Declaration Examples
Constant Name | Data Type | Value |
---|---|---|
PI | double | 3.14159 |
GRAVITY | float | 9.81f |
MAX_CONNECTIONS | int | 50 |
DEFAULT_LANGUAGE | String | “English” |
Best Practices
- Use the `final` keyword whenever you intend to define a constant.
- Group related constants in a dedicated class or interface, which can also be marked as `final`.
- Consider using enumerations (`enum`) for a fixed set of constants that are related, providing type safety and readability.
By adhering to these practices, developers can create robust and maintainable Java applications.
Understanding Constants in Java
In Java, constants are variables whose values cannot be changed once assigned. They are declared using the `final` keyword, which ensures that the variable’s value is immutable after its initial assignment. This feature is crucial for maintaining the integrity of data throughout the program.
Declaring a Constant
To declare a constant in Java, follow these steps:
- Use the `final` keyword.
- Specify the data type of the constant.
- Provide a name for the constant (it is convention to use uppercase letters).
- Assign a value to the constant.
The syntax for declaring a constant is as follows:
“`java
final dataType CONSTANT_NAME = value;
“`
Example of Constant Declaration
“`java
final int MAX_USERS = 100;
final double PI = 3.14159;
final String APPLICATION_NAME = “MyApp”;
“`
In this example:
- `MAX_USERS` represents the maximum number of users allowed.
- `PI` holds the mathematical constant π.
- `APPLICATION_NAME` stores the name of the application.
Benefits of Using Constants
Using constants in Java offers several advantages:
- Improved Readability: Constants provide clear meaning to values, making the code easier to understand.
- Maintainability: Changing the value in one place updates it throughout the program, reducing errors.
- Performance: The compiler can optimize code better when it knows that certain values are constants.
Best Practices for Constants
When working with constants in Java, consider the following best practices:
- Use uppercase letters with underscores for constant names (e.g., `MAX_LENGTH`).
- Group related constants in an interface or a class.
- Use `static final` for constants that belong to a class.
Example of Grouping Constants
“`java
public class Constants {
public static final int MAX_LENGTH = 255;
public static final String DEFAULT_USERNAME = “Guest”;
public static final double TAX_RATE = 0.07;
}
“`
This approach encapsulates constants logically, enhancing organization and maintainability.
Constant Scope
The scope of a constant depends on where it is declared:
- Class-level constants: Declared within a class and accessible throughout the class.
- Method-level constants: Declared within a method and accessible only within that method.
Immutable Collections as Constants
Java also allows the creation of immutable collections, which can serve as constants. The `Collections.unmodifiableList()` method can be used to create a read-only list.
“`java
final List
“`
This ensures that the `NAMES` list cannot be modified after its creation.
Conclusion on Constants
Using constants effectively in Java enhances code quality, readability, and maintainability. By following established conventions and best practices, developers can ensure their applications are robust and easier to manage.
Expert Insights on Declaring Constants in Java
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Declaring constants in Java is essential for maintaining code readability and preventing accidental modifications. By using the `final` keyword, developers can ensure that certain values remain unchanged throughout the execution of the program, which is crucial for avoiding bugs.”
Michael Chen (Java Developer Advocate, CodeCraft Academy). “The use of constants in Java not only enhances performance by reducing the overhead of repeated calculations but also serves as a form of documentation. When a constant is declared, it provides clarity on the intended use of the value within the code, making it easier for others to understand.”
Sarah Patel (Lead Java Instructor, Advanced Programming School). “Constants are a fundamental part of Java programming. By declaring constants using the `static final` modifiers, developers can create class-level constants that are accessible without needing to instantiate the class, thereby promoting efficient memory usage and improving application performance.”
Frequently Asked Questions (FAQs)
What is a constant in Java?
A constant in Java is a variable whose value cannot be changed once it is assigned. Constants are declared using the `final` keyword.
How do you declare a constant in Java?
To declare a constant in Java, use the `final` keyword followed by the data type, the variable name, and assign it a value. For example: `final int MAX_VALUE = 100;`.
Can a constant be modified after declaration?
No, once a constant is declared with the `final` keyword, its value cannot be modified. Attempting to change it will result in a compilation error.
What is the convention for naming constants in Java?
Constants are typically named using uppercase letters with underscores separating words. For example: `final int MAX_CONNECTIONS = 10;`.
Can constants be declared in an interface in Java?
Yes, constants can be declared in an interface. By default, all variables in an interface are `public`, `static`, and `final`, making them constants.
Is it possible to declare a constant for a reference type in Java?
Yes, you can declare a constant for a reference type. However, while the reference itself cannot be changed, the object it points to can still be modified unless the object’s fields are also declared as `final`.
In Java, declaring a constant is primarily accomplished using the `final` keyword. This keyword indicates that a variable’s value cannot be changed once it has been assigned. By convention, constants are typically named in uppercase letters with underscores separating words, which enhances code readability and conveys the immutability of the variable. For example, a constant for the value of Pi might be declared as `final double PI = 3.14159;`.
Constants in Java can be of any data type, including primitive types like `int`, `double`, and `char`, as well as reference types such as strings and objects. Utilizing constants effectively can lead to cleaner and more maintainable code, as they prevent the use of “magic numbers” or hard-coded values throughout the codebase. This practice not only reduces the likelihood of errors but also makes the code easier to understand and modify in the future.
In summary, the use of the `final` keyword in Java is essential for declaring constants, ensuring that certain values remain unchanged throughout the execution of a program. This approach promotes better programming practices by enhancing clarity and reducing potential bugs. Developers are encouraged to adopt this practice to improve the overall quality of their code.
Author Profile
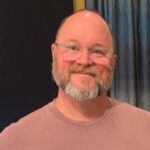
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?