How Can You Format Dates in SQL to DD/MM/YYYY?
When working with databases, the way we handle dates can significantly impact the efficiency and accuracy of our queries. In SQL, the format in which dates are stored and manipulated is crucial for ensuring that data is interpreted correctly. Among the various formats available, the `dd/mm/yyyy` format stands out for its clarity and ease of use, particularly in regions where this convention is prevalent. Understanding how to effectively manage and convert dates in this format can empower developers and data analysts alike to streamline their workflows and enhance data integrity.
In this article, we will delve into the nuances of using the `dd/mm/yyyy` date format in SQL, exploring how to insert, retrieve, and format dates to suit your specific needs. We will discuss the importance of consistency in date formats, particularly when dealing with international datasets, and how SQL functions can help you manipulate dates with precision. Whether you’re a seasoned SQL user or a newcomer eager to learn the ropes, mastering date formats is an essential skill that can elevate your database management capabilities.
As we navigate through the intricacies of date handling in SQL, we will also highlight common pitfalls and best practices to ensure that your date operations are both reliable and efficient. By the end of this exploration, you will have a solid understanding of how to work with dates in the
Date Formatting Functions in SQL
In SQL, handling date formats is essential for ensuring data integrity and accuracy, especially when dealing with internationalization or specific formatting requirements. The `CONVERT` and `FORMAT` functions are commonly used to convert dates into the desired format, such as `DD/MM/YYYY`.
- CONVERT Function: This function is primarily used in SQL Server. It allows you to convert a date value into a specific format. The syntax is as follows:
“`sql
CONVERT(data_type, expression, style)
“`
Here, `style` defines the format of the output. For `DD/MM/YYYY`, the style code is `103`.
- FORMAT Function: This function is available in SQL Server 2012 and later. It provides a more flexible way to format dates. The syntax is:
“`sql
FORMAT(value, format_string)
“`
To format the date as `DD/MM/YYYY`, use:
“`sql
FORMAT(your_date_column, ‘dd/MM/yyyy’)
“`
Example Queries for Date Formatting
To illustrate the use of the `CONVERT` and `FORMAT` functions, consider the following SQL queries:
“`sql
— Using CONVERT to format date
SELECT CONVERT(VARCHAR(10), your_date_column, 103) AS FormattedDate
FROM your_table;
— Using FORMAT to format date
SELECT FORMAT(your_date_column, ‘dd/MM/yyyy’) AS FormattedDate
FROM your_table;
“`
These queries will return the dates from `your_date_column` in the desired `DD/MM/YYYY` format.
Using DATEPART to Extract Components
Sometimes, it may be necessary to extract components of the date for further manipulation or to construct your own formatted strings. The `DATEPART` function can be utilized for this purpose:
“`sql
SELECT
DATEPART(DAY, your_date_column) AS Day,
DATEPART(MONTH, your_date_column) AS Month,
DATEPART(YEAR, your_date_column) AS Year
FROM your_table;
“`
You can then concatenate these components to form a date in the `DD/MM/YYYY` format:
“`sql
SELECT
CAST(DATEPART(DAY, your_date_column) AS VARCHAR(2)) + ‘/’ +
CAST(DATEPART(MONTH, your_date_column) AS VARCHAR(2)) + ‘/’ +
CAST(DATEPART(YEAR, your_date_column) AS VARCHAR(4)) AS FormattedDate
FROM your_table;
“`
Common Issues and Considerations
When working with date formats in SQL, be aware of potential issues:
- Regional Settings: The default date format may vary based on the database server’s locale settings.
- Data Type Mismatches: Ensure that the column data types are appropriate for date operations to prevent conversion errors.
- NULL Values: Handle NULL dates gracefully to avoid runtime errors.
To summarize the key points, here’s a table that outlines the functions and their uses:
Function | Usage | Format Example |
---|---|---|
CONVERT | Convert date to specified format | CONVERT(VARCHAR(10), date_column, 103) |
FORMAT | Flexible date formatting | FORMAT(date_column, ‘dd/MM/yyyy’) |
DATEPART | Extract date components | DATEPART(DAY, date_column) |
Date Format in SQL
SQL databases often use the ISO 8601 format (YYYY-MM-DD) for date representation. However, certain applications or user interfaces may require dates formatted in DD/MM/YYYY. To achieve this format, various SQL functions can be employed depending on the database system in use.
Formatting Dates in Different SQL Databases
The method for formatting dates can vary significantly across SQL databases. Below are examples for several popular systems:
MySQL
In MySQL, the `DATE_FORMAT` function is utilized to format dates. Here is how you can format a date to DD/MM/YYYY:
“`sql
SELECT DATE_FORMAT(NOW(), ‘%d/%m/%Y’) AS formatted_date;
“`
- `%d` – Day of the month, numeric (01 to 31)
- `%m` – Month, numeric (01 to 12)
- `%Y` – Year, numeric, four digits
SQL Server
SQL Server uses the `FORMAT` function or `CONVERT` function to change date formats. Below are examples of both methods:
Using `FORMAT`:
“`sql
SELECT FORMAT(GETDATE(), ‘dd/MM/yyyy’) AS formatted_date;
“`
Using `CONVERT`:
“`sql
SELECT CONVERT(VARCHAR(10), GETDATE(), 103) AS formatted_date;
“`
- `103` – British/French style (DD/MM/YYYY)
PostgreSQL
In PostgreSQL, the `TO_CHAR` function formats dates. Here’s how to format a date:
“`sql
SELECT TO_CHAR(NOW(), ‘DD/MM/YYYY’) AS formatted_date;
“`
- `DD` – Day of the month (01 to 31)
- `MM` – Month (01 to 12)
- `YYYY` – Year (four digits)
Oracle
Oracle uses the `TO_CHAR` function as well. Here’s an example:
“`sql
SELECT TO_CHAR(SYSDATE, ‘DD/MM/YYYY’) AS formatted_date FROM dual;
“`
- `SYSDATE` returns the current date and time.
Inserting Dates in DD/MM/YYYY Format
When inserting dates into SQL databases, the format must typically comply with the database’s expected format. However, if you have a string in DD/MM/YYYY and need to convert it, you can use the following methods:
MySQL
“`sql
INSERT INTO your_table (date_column) VALUES (STR_TO_DATE(’31/12/2023′, ‘%d/%m/%Y’));
“`
SQL Server
“`sql
INSERT INTO your_table (date_column) VALUES (CONVERT(DATE, ’31/12/2023′, 103));
“`
PostgreSQL
“`sql
INSERT INTO your_table (date_column) VALUES (TO_DATE(’31/12/2023′, ‘DD/MM/YYYY’));
“`
Oracle
“`sql
INSERT INTO your_table (date_column) VALUES (TO_DATE(’31/12/2023′, ‘DD/MM/YYYY’));
“`
Utilizing the correct functions for formatting dates is crucial for ensuring data integrity and consistency across applications. Each SQL database has its own syntax and functions that facilitate this process, thereby allowing developers to format and manipulate date values as needed.
Expert Perspectives on Formatting Dates in SQL
Dr. Emily Chen (Database Architect, Tech Innovations Inc.). “Using the `DD/MM/YYYY` format in SQL can be crucial for applications that cater to regions where this format is the standard. It is essential to ensure that the database collation settings support this format to avoid misinterpretation of date values.”
Mark Thompson (Senior SQL Developer, Data Solutions Group). “When working with dates in SQL, it is vital to convert them to the correct format using functions like `CONVERT()` or `FORMAT()`. This ensures consistency across queries and prevents errors during data manipulation.”
Lisa Patel (Data Analyst, Insight Analytics). “Adopting a standardized date format such as `DD/MM/YYYY` in SQL queries can enhance readability and maintainability of the code. It is also advisable to document the expected date formats within the database schema for clarity.”
Frequently Asked Questions (FAQs)
How can I format a date in DD/MM/YYYY format in SQL?
To format a date in DD/MM/YYYY format in SQL, you can use the `FORMAT()` function in SQL Server or the `TO_CHAR()` function in Oracle. For example, in SQL Server: `SELECT FORMAT(GETDATE(), ‘dd/MM/yyyy’)`. In Oracle, use: `SELECT TO_CHAR(SYSDATE, ‘DD/MM/YYYY’)`.
What SQL functions can convert a string to a date in DD/MM/YYYY format?
You can use the `STR_TO_DATE()` function in MySQL, which allows you to specify the format. For example: `SELECT STR_TO_DATE(’31/12/2023′, ‘%d/%m/%Y’)`. In SQL Server, you can use `CONVERT()` with style 103: `SELECT CONVERT(DATE, ’31/12/2023′, 103)`.
Is it possible to store dates in DD/MM/YYYY format in a SQL database?
SQL databases typically store dates in a binary format, not as strings. However, you can format the date as DD/MM/YYYY when retrieving it using formatting functions, but it is advisable to store dates in their native format for accurate date operations.
What is the difference between date formats in SQL Server and MySQL?
SQL Server uses `YYYY-MM-DD` as the default date format, while MySQL supports multiple formats, including `YYYY-MM-DD` and `DD/MM/YYYY` when specified. Always use appropriate functions to convert or format dates as needed in each database system.
Can I use the DD/MM/YYYY format in SQL queries for comparisons?
Yes, you can use the DD/MM/YYYY format in SQL queries for comparisons, but it is recommended to convert the date to the database’s native date format for accurate comparisons. Use functions like `STR_TO_DATE()` in MySQL or `CONVERT()` in SQL Server for this purpose.
How do I handle invalid date formats when inserting data into a SQL table?
To handle invalid date formats, ensure data validation before insertion. Use `TRY_CONVERT()` in SQL Server or `STR_TO_DATE()` in MySQL to catch errors. Implement error handling to manage exceptions during the insertion process.
In SQL, handling dates in the dd/mm/yyyy format requires an understanding of the database system’s date functions and formatting capabilities. Different SQL dialects, such as MySQL, SQL Server, and PostgreSQL, have unique methods for parsing and formatting dates. It is crucial to ensure that the date strings are correctly interpreted by the database to avoid errors in data retrieval and manipulation.
When working with date formats, it is essential to use appropriate functions such as `STR_TO_DATE()` in MySQL or `CONVERT()` in SQL Server to convert strings into date objects. Additionally, formatting output can be achieved with functions like `DATE_FORMAT()` in MySQL or `FORMAT()` in SQL Server. Understanding these functions enables developers to effectively manage and display date information in the desired format.
Moreover, it is important to consider the implications of date formats on internationalization and user experience. Different regions may have varying date representations, and adhering to a standard format can enhance clarity and prevent misinterpretation. Therefore, implementing consistent date handling practices across applications is vital for maintaining data integrity and usability.
Author Profile
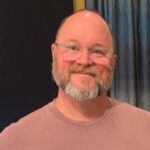
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?