Why Am I Getting the ‘Dataframe Object Is Not Callable’ Error in Python?
In the world of data manipulation and analysis, pandas has emerged as a powerhouse library for Python users. Its ability to handle complex datasets with ease has made it a go-to tool for data scientists and analysts alike. However, as with any powerful tool, it comes with its own set of challenges and pitfalls. One common error that many encounter is the perplexing message: “Dataframe object is not callable.” This seemingly simple mistake can lead to frustration and confusion, especially for those who are just starting their journey into data science.
Understanding the nuances of this error is crucial for anyone working with pandas. It often arises from a misunderstanding of how to properly interact with DataFrame objects, leading to attempts to call them as if they were functions. This article will delve into the reasons behind this error, providing clarity on how to avoid it and troubleshoot effectively. By exploring the underlying concepts of DataFrame manipulation, readers will gain valuable insights that will not only resolve this specific issue but also enhance their overall proficiency with pandas.
As we navigate through the intricacies of this error, we will uncover best practices for working with DataFrames, highlight common coding mistakes, and offer practical tips to ensure smooth sailing in your data analysis endeavors. Whether you are a novice or an experienced programmer,
Understanding the Error
The error message “Dataframe object is not callable” typically arises when you attempt to invoke a pandas DataFrame as if it were a function. This can occur due to a variety of reasons, including variable name conflicts or incorrect syntax. Understanding the underlying causes of this error is crucial for effective debugging.
Common reasons for this error include:
- Variable Shadowing: If you have a variable named `DataFrame` that shadows the pandas DataFrame class, it may lead to confusion.
- Improper Syntax: Attempting to use parentheses instead of brackets when accessing DataFrame methods or attributes.
- Unintentional Reassignment: Assigning a DataFrame to a variable that is later invoked as a function.
Example Scenarios
Consider the following scenarios that illustrate how this error can occur:
- Variable Shadowing:
“`python
import pandas as pd
DataFrame = pd.DataFrame({‘A’: [1, 2], ‘B’: [3, 4]}) Variable shadows the DataFrame class
df = DataFrame() This will raise the error
“`
- Improper Syntax:
“`python
df = pd.DataFrame({‘A’: [1, 2], ‘B’: [3, 4]})
df() Incorrectly trying to call df as a function
“`
- Unintentional Reassignment:
“`python
df = pd.DataFrame({‘A’: [1, 2], ‘B’: [3, 4]})
df = df[‘A’] df is now a Series, not a DataFrame
df() Raises the error since df is not callable
“`
How to Fix the Error
To resolve the “Dataframe object is not callable” error, consider the following strategies:
- Check Variable Names: Ensure that you are not inadvertently using variable names that conflict with pandas’ built-in classes.
- Use Correct Syntax: Always use brackets to access DataFrame attributes and methods.
- Reassign with Caution: Be careful when reassigning variables to ensure that you are not losing the original DataFrame object.
Here’s a quick reference table summarizing these fixes:
Issue | Resolution |
---|---|
Variable Shadowing | Use a different name for your variable. |
Improper Syntax | Use brackets for indexing: df[‘column_name’]. |
Unintentional Reassignment | Avoid overwriting DataFrame with a Series or other objects. |
By following these guidelines, you can effectively troubleshoot and resolve the “Dataframe object is not callable” error, ensuring smoother data manipulation using pandas.
Understanding the Error
The error message “Dataframe object is not callable” typically occurs in Python programming, particularly when using the Pandas library. This error indicates that you are attempting to call a DataFrame object as if it were a function. In Python, parentheses are used to invoke functions, and if a DataFrame is mistakenly treated this way, the interpreter will raise this error.
Common Causes
Several situations can lead to this error:
- Naming Conflicts: If you have a variable name that matches a method name of the DataFrame.
- Misunderstanding Syntax: Using parentheses instead of square brackets when accessing DataFrame elements.
- Accidental Reassignment: Overwriting the DataFrame variable with a non-DataFrame object.
Examples of the Error
Understanding how this error manifests can help in troubleshooting.
- Naming Conflicts:
“`python
import pandas as pd
df = pd.DataFrame({‘A’: [1, 2], ‘B’: [3, 4]})
Incorrectly reassigning df to an integer
df = 10
result = df() Raises TypeError: ‘int’ object is not callable
“`
- Misunderstanding Syntax:
“`python
import pandas as pd
df = pd.DataFrame({‘A’: [1, 2], ‘B’: [3, 4]})
value = df(0, ‘A’) Raises TypeError: ‘DataFrame’ object is not callable
“`
- Accidental Reassignment:
“`python
import pandas as pd
df = pd.DataFrame({‘A’: [1, 2], ‘B’: [3, 4]})
df = df[‘A’] Now df is a Series, not a DataFrame
result = df() Raises TypeError: ‘Series’ object is not callable
“`
How to Fix the Error
Identifying the root cause of the error is essential for rectification. Here are steps to resolve it:
- Check Variable Names: Ensure that you have not inadvertently used a DataFrame method as a variable name. Use descriptive names to avoid conflicts.
- Use Correct Syntax: Access DataFrame elements using square brackets:
“`python
value = df.loc[0, ‘A’] Correct way to access DataFrame elements
“`
- Confirm DataFrame Type: Before calling a method, verify the type of the object:
“`python
print(type(df)) Should return
“`
Best Practices to Avoid the Error
Implementing best practices can significantly reduce the likelihood of encountering this error:
- Descriptive Naming Conventions:
- Use clear and unique names for variables.
- Consistent Data Manipulation:
- Maintain clarity in your data processing pipeline to ensure that DataFrames are not unintentionally reassigned.
- Code Review and Testing:
- Regularly review code to catch potential naming conflicts or incorrect usages early in the development process.
- Utilize IDE Features:
- Use features in Integrated Development Environments (IDEs) that highlight variable types and function definitions to avoid confusion.
By adhering to these guidelines, you can mitigate the risk of running into the “Dataframe object is not callable” error and enhance the robustness of your code.
Understanding the ‘Dataframe Object Is Not Callable’ Error
Dr. Emily Chen (Data Scientist, Tech Innovations Inc.). “The ‘Dataframe object is not callable’ error typically arises when a user mistakenly attempts to call a DataFrame as if it were a function. This often occurs due to naming conflicts where a variable name shadows the DataFrame, leading to confusion in the code execution.”
Michael Thompson (Senior Software Engineer, Data Solutions Corp.). “To resolve this error, developers should ensure that they are not using parentheses when trying to access DataFrame attributes or methods. Instead, they should use square brackets or dot notation to reference columns or perform operations.”
Lisa Patel (Python Instructor, Code Academy). “This error serves as a reminder to maintain clear and distinct variable names. By avoiding the reuse of names that are already associated with DataFrame objects, programmers can minimize the risk of encountering this issue.”
Frequently Asked Questions (FAQs)
What does the error “Dataframe object is not callable” mean?
This error typically occurs when you attempt to call a DataFrame object as if it were a function. In Python, this can happen if you mistakenly use parentheses instead of square brackets to access DataFrame elements or methods.
How can I fix the “Dataframe object is not callable” error?
To resolve this error, ensure that you are using square brackets `[]` to access DataFrame columns or rows. For example, use `df[‘column_name’]` instead of `df(‘column_name’)`.
What are common scenarios that lead to this error?
Common scenarios include mistakenly using parentheses when trying to access a DataFrame column, overwriting the DataFrame variable with a function, or attempting to call a method that does not exist.
Can this error occur with other data structures in Python?
Yes, similar errors can occur with other data structures, such as lists or dictionaries, if you mistakenly use parentheses instead of the appropriate indexing method.
Is there a way to debug this error in my code?
You can debug this error by checking the line of code where the error occurs, ensuring that you are not using parentheses for indexing, and verifying that you have not overwritten your DataFrame with a callable function.
What best practices can help prevent this error?
To prevent this error, adhere to clear naming conventions for DataFrames and functions, avoid using the same name for both, and consistently use square brackets for indexing DataFrames.
The error message “DataFrame object is not callable” typically arises in Python’s Pandas library when a user attempts to call a DataFrame as if it were a function. This situation often occurs due to a naming conflict, where a variable name shadows the DataFrame’s method or attribute. For instance, if a user inadvertently assigns a DataFrame to a variable name that is the same as a method (e.g., `df = df()`), subsequent attempts to call that method will result in this error. Understanding the context in which this error occurs is crucial for effective debugging.
To resolve this issue, users should carefully review their code to identify any variable names that may conflict with DataFrame methods. Renaming such variables to avoid shadowing built-in methods or attributes is a common and effective solution. Additionally, users should ensure that they are using the correct syntax when accessing DataFrame methods, utilizing parentheses only when invoking a method and not when referencing the DataFrame itself.
In summary, the “DataFrame object is not callable” error serves as a reminder of the importance of naming conventions and method usage in programming with Pandas. By adhering to best practices in variable naming and method invocation, users can minimize the occurrence of this error and
Author Profile
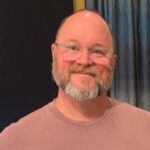
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?