Why Am I Getting the Error ‘Could Not Open JPA EntityManager for Transaction’?
In the world of Java Persistence API (JPA), managing transactions effectively is crucial for maintaining data integrity and ensuring smooth application performance. However, developers often encounter the frustrating error message: “Could Not Open JPA EntityManager For Transaction.” This issue can arise from various underlying causes, ranging from configuration mishaps to resource limitations. Understanding the intricacies of this error is essential for troubleshooting and optimizing your JPA usage, ultimately leading to more robust applications. In this article, we will delve into the common reasons behind this error and explore practical solutions to help you navigate the complexities of JPA transactions with confidence.
Overview
The “Could Not Open JPA EntityManager For Transaction” error typically signals a problem with the EntityManager’s ability to establish a connection to the underlying database. This can stem from a variety of issues, including incorrect database configurations, connection pool exhaustion, or even mismanagement of the EntityManager lifecycle. As applications scale and demand increases, these challenges can become more pronounced, making it essential for developers to understand the root causes and implement effective strategies for resolution.
Additionally, the implications of this error extend beyond mere inconvenience; they can lead to significant downtime and hinder the overall user experience. By proactively addressing the factors that contribute to this error, developers can
Understanding the Root Causes
When encountering the error message “Could Not Open Jpa Entitymanager For Transaction,” it is essential to delve into the various underlying causes that may lead to this issue. The persistence context, which is managed by the JPA EntityManager, can face several obstacles that prevent it from opening successfully for transaction management.
Key factors contributing to this error include:
- Misconfiguration of DataSource: Ensure that the DataSource properties in your configuration file are correct. This includes the database URL, username, and password.
- EntityManagerFactory Issues: An improperly configured or uninitialized EntityManagerFactory can prevent the creation of an EntityManager.
- Transaction Management Problems: Issues in transaction boundaries or incorrect handling of transactions can lead to failure in opening the EntityManager.
- Database Connectivity: Network issues or database server downtime can hinder connectivity, leading to this error.
- Resource Exhaustion: The database might be running out of connections or resources, causing the failure to open a new EntityManager.
Common Solutions
To address the “Could Not Open Jpa Entitymanager For Transaction” error, several solutions can be implemented. Here are some effective approaches:
- Check Database Connection: Validate the connectivity to your database using tools like ping or telnet to ensure the database server is reachable.
- Review Configuration Files: Double-check your persistence.xml or application.properties files for any misconfigurations related to your DataSource or JPA settings.
- Update Dependencies: Ensure that you are using compatible versions of JPA, Hibernate, and your database driver. Outdated dependencies can lead to compatibility issues.
- Monitor Resource Usage: Use monitoring tools to track database connections and resource utilization. Adjust connection pool settings if necessary.
- Enable Logging: Increase the logging level for JPA and Hibernate to gather more details about the error. This can provide insights into the root cause.
Configuration Example
Here is an example configuration for a JPA DataSource in a `persistence.xml` file:
“`xml
Diagnostic Table
The following table summarizes common symptoms, causes, and potential fixes for the error:
Symptom | Possible Cause | Solution |
---|---|---|
EntityManagerFactory not found | Misconfiguration in persistence.xml | Check persistence.xml for correctness |
Transaction rollback | Transaction management issue | Review transaction annotations and boundaries |
Database connection timeout | Network issues or resource limits | Check network connectivity and increase connection pool size |
No suitable driver found | Missing or incorrect JDBC driver | Ensure the correct JDBC driver is included |
By systematically addressing each of these areas, it is possible to resolve the “Could Not Open Jpa Entitymanager For Transaction” error and restore normal operation to your application.
Common Causes of the Error
The “Could Not Open Jpa Entitymanager For Transaction” error can stem from several underlying issues. Understanding these causes is crucial for troubleshooting effectively. Here are some common factors that may lead to this error:
- Database Connection Issues:
- Incorrect database URL or credentials.
- Database server not running or unreachable.
- Configuration Errors:
- Misconfigured `persistence.xml` settings.
- Missing or incorrect JPA provider configuration.
- Transaction Management Problems:
- Improperly managed transactions leading to resource lock.
- Attempting to open a transaction when the EntityManager is closed.
- Resource Limitations:
- Exceeded connection pool size.
- Insufficient database resources (CPU, memory).
Troubleshooting Steps
To resolve the “Could Not Open Jpa Entitymanager For Transaction” error, follow these troubleshooting steps:
- Verify Database Connectivity:
- Check if the database server is running.
- Test the connection using a database client with the same credentials.
- Inspect Configuration Files:
- Review `persistence.xml` for accuracy in database connection details.
- Ensure the JPA provider is properly specified.
- Examine Transaction Management:
- Confirm that transactions are being started and committed appropriately.
- Ensure that the EntityManager is open when attempting to initiate a transaction.
- Monitor Resource Usage:
- Check the connection pool settings to ensure it can handle the load.
- Analyze database performance metrics for potential bottlenecks.
Configuration Example
A correctly configured `persistence.xml` is essential for JPA to function correctly. Below is an example configuration:
“`xml
Ensure that each property is correctly set according to your database configuration.
Best Practices
To avoid encountering the “Could Not Open Jpa Entitymanager For Transaction” error, consider the following best practices:
- Use Connection Pooling: Implement a connection pool to manage database connections efficiently.
- Regularly Update Dependencies: Keep your JPA provider and database drivers updated to the latest stable versions.
- Implement Error Handling: Use try-catch blocks around transaction management code to catch and log exceptions effectively.
- Monitor Application Performance: Use monitoring tools to track database performance and identify potential issues before they escalate.
By adhering to these practices, you can reduce the likelihood of encountering this error in your applications.
Expert Insights on Jpa EntityManager Transaction Issues
Dr. Emily Carter (Lead Software Architect, Tech Innovations Inc.). “The error ‘Could Not Open Jpa EntityManager For Transaction’ typically indicates a misconfiguration in the persistence context or an issue with the underlying database connection. It is crucial to ensure that the data source is correctly defined in your application properties and that the database is accessible.”
Michael Thompson (Senior Java Developer, CodeCraft Solutions). “In my experience, this issue often arises from transaction management problems. Ensure that your transaction boundaries are correctly defined and that the EntityManager is being properly initialized before attempting to access it. Additionally, check for any exceptions that may be thrown during the transaction lifecycle.”
Laura Chen (Database Administrator, Cloud Data Systems). “When encountering the ‘Could Not Open Jpa EntityManager For Transaction’ error, I recommend examining the connection pool settings. Insufficient connections in the pool can lead to this error. Monitoring the database logs can also provide insights into any connectivity issues that may be affecting the EntityManager.”
Frequently Asked Questions (FAQs)
What does the error “Could Not Open Jpa Entitymanager For Transaction” indicate?
This error typically indicates that there is an issue with the configuration or initialization of the JPA EntityManager, preventing it from establishing a connection to the database for transaction management.
What are common causes of this error?
Common causes include incorrect database connection settings, missing or misconfigured persistence.xml, or issues with the underlying data source, such as network problems or database server downtime.
How can I troubleshoot the “Could Not Open Jpa Entitymanager For Transaction” error?
To troubleshoot, check the database connection parameters in your configuration, ensure that the persistence.xml is properly defined, and verify that the database server is running and accessible.
Are there specific logs I should check when encountering this error?
Yes, check the application server logs for any stack traces or error messages related to JPA or database connectivity. Additionally, reviewing the logs of the database server can provide insights into connection attempts and failures.
Can this error be caused by transaction management issues?
Yes, improper transaction management, such as trying to manage transactions outside of a valid context or using an incorrect transaction type, can lead to this error when the EntityManager cannot be opened.
What steps can I take to resolve this issue in a production environment?
In a production environment, ensure that your database connection pool is correctly configured, validate the persistence context settings, and consider implementing robust error handling and logging to capture detailed information about the error for further analysis.
The error message “Could Not Open Jpa EntityManager For Transaction” typically indicates an issue with the Java Persistence API (JPA) configuration or the underlying database connection. This error can arise from various factors, including incorrect database connection settings, issues with the JPA provider, or problems with the transaction management setup. Understanding the root cause of this error is crucial for effective troubleshooting and resolution.
One of the primary reasons for this error is misconfigured database connection properties in the persistence.xml file or equivalent configuration settings. Ensuring that the database URL, username, and password are correctly specified is essential. Additionally, verifying that the database server is running and accessible can help eliminate connectivity issues. Another common cause can be related to the JPA provider itself, such as Hibernate or EclipseLink, which may require specific settings or dependencies to function correctly.
Moreover, transaction management plays a significant role in the functioning of JPA. If the transaction manager is not properly configured or if there are conflicting transaction settings, it can lead to the inability to open the EntityManager. Developers should ensure that they are using the appropriate transaction management strategy, whether it be container-managed or application-managed transactions, and that all related configurations are in sync.
In conclusion
Author Profile
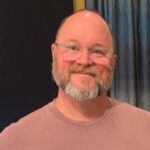
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?