Why Am I Seeing ‘Could Not Autowire. No Beans Of’ Error in My Spring Application?
In the world of Spring Framework, the power of dependency injection is both a boon and a source of frustration for developers. One of the most common hurdles encountered during application development is the dreaded error message: “Could Not Autowire. No Beans Of Type…” This seemingly cryptic notification can halt progress and leave even seasoned developers scratching their heads. Understanding this error is crucial, as it not only impacts the functionality of your application but also illuminates the intricacies of Spring’s bean management system. In this article, we will delve into the causes and solutions for this error, equipping you with the knowledge to navigate and resolve these dependency injection dilemmas with confidence.
As we explore the nuances of the “Could Not Autowire” error, we will first examine the fundamental principles of Spring’s dependency injection and bean lifecycle. By grasping how Spring manages its beans and the context in which they operate, you will gain insight into why this error occurs. We will also highlight common pitfalls that developers encounter when configuring their beans, from misconfigurations to missing annotations, ensuring you are well-prepared to tackle these issues head-on.
Moreover, we will discuss practical strategies and best practices for troubleshooting and resolving autowiring problems. By the end of this article, you
Understanding Autowiring in Spring
Autowiring in Spring is a mechanism that allows Spring to automatically resolve and inject collaborating beans into your application. This is particularly useful for reducing configuration overhead. However, issues can arise, such as the “Could Not Autowire. No Beans Of” error, which indicates that Spring is unable to find a suitable bean to inject.
The autowiring process can be configured in several ways, including:
- By Type: Spring looks for a bean of the specified type.
- By Name: Spring searches for a bean with a matching name.
- Constructor Injection: Spring uses constructor parameters to perform dependency injection.
- Field Injection: Spring directly injects dependencies into fields without needing setters or constructors.
Common Causes of the “Could Not Autowire” Error
Several reasons can lead to the “Could Not Autowire. No Beans Of” error. Understanding these causes is essential for troubleshooting:
- Missing Bean Definition: The specified bean may not be defined in the Spring context.
- Incorrect Configuration: An incorrect configuration or missing annotations can prevent Spring from recognizing the bean.
- Scope Issues: If beans have conflicting scopes (e.g., singleton vs. prototype), it may lead to autowiring failures.
- Circular Dependencies: If two beans depend on each other, Spring may not be able to resolve them.
Resolving the Autowiring Error
To resolve the “Could Not Autowire” error, consider the following steps:
- Check Bean Definitions: Ensure that the bean you are trying to autowire is correctly defined in the Spring context. This can be done via XML configuration or Java annotations.
- Use Annotations: Make use of annotations such as `@Component`, `@Service`, or `@Repository` to define your beans. Ensure that your classes are scanned by Spring by using the `@ComponentScan` annotation.
- Verify Type Compatibility: Confirm that the type of the autowired field matches the type of the bean available in the context.
- Inspect Configuration Files: If using XML configurations, ensure that the bean definitions are correctly specified without typographical errors.
- Review Scopes: Ensure that beans are defined with compatible scopes. If a singleton bean is trying to inject a prototype bean, it may lead to issues.
- Check for Circular Dependencies: Refactor your code to eliminate circular dependencies if they exist.
Example Configuration
The following table outlines a basic configuration for autowiring in Spring:
Bean Class | Annotation | Scope |
---|---|---|
MyService | @Service | Singleton |
MyRepository | @Repository | Singleton |
MyController | @Controller | Singleton |
By following the steps outlined and ensuring proper configurations, you can effectively resolve autowiring issues in your Spring applications.
Understanding the Error Message
The error message “Could Not Autowire. No Beans Of” typically occurs in Spring Framework applications when the dependency injection mechanism cannot find a bean that matches the required type. This can disrupt the functioning of the application and lead to runtime exceptions.
Key points regarding the error include:
- Dependency Injection: Spring uses dependency injection to manage the life cycle of beans and their dependencies. If a required bean is not available in the context, autowiring fails.
- Bean Definitions: Beans must be defined in a configuration class or XML file for Spring to recognize and manage them. Missing or misconfigured beans can lead to this error.
- Component Scanning: If component scanning is not correctly configured, Spring may not detect beans annotated with `@Component`, `@Service`, or other stereotype annotations.
Common Causes
Several common causes lead to the “Could Not Autowire” error:
- Missing Bean Definition: The required bean is not declared in the Spring context.
- Incorrect Qualifiers: Using `@Qualifier` with an incorrect name can prevent the autowiring from succeeding.
- Scope Issues: Mismatched scopes (e.g., singleton vs. prototype) between the bean being injected and the bean requesting it can lead to issues.
- Circular Dependencies: If two beans depend on each other, it can result in an unsolvable dependency graph.
Resolution Steps
To resolve the “Could Not Autowire” issue, follow these steps:
- Check Bean Configuration:
- Ensure the bean is defined in your configuration class or XML file.
- Verify that the bean is annotated with the appropriate Spring annotations.
- Examine Component Scanning:
- Confirm that the package containing the bean is included in the component scan configuration.
- Check if your configuration class is annotated with `@ComponentScan`.
- Use the Correct Qualifier:
- If multiple beans of the same type exist, use `@Qualifier` to specify which bean to inject.
- Ensure that the qualifier name matches the bean name defined.
- Review Constructor and Setter Injection:
- If using constructor injection, ensure all required parameters are available as beans.
- For setter injection, verify that the setter method is properly defined and accessible.
Example of Bean Configuration
Here is an example of how to define beans in a Spring configuration class:
“`java
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class AppConfig {
@Bean
public MyService myService() {
return new MyServiceImpl();
}
@Bean
public MyController myController() {
return new MyController(myService());
}
}
“`
Debugging Tips
When troubleshooting the “Could Not Autowire” issue, consider the following tips:
- Enable Debug Logging: Set the logging level for Spring to DEBUG to see detailed information about bean creation and autowiring.
- Check Application Context: Use the `ApplicationContext` to list all beans and confirm the expected beans are present.
- Review Stack Trace: Analyze the stack trace for more context on where the autowiring failed.
By following these guidelines and understanding the error message, developers can effectively troubleshoot and resolve autowiring issues in Spring applications.
Understanding the “Could Not Autowire. No Beans Of” Error in Spring Framework
Dr. Emily Carter (Senior Software Engineer, Spring Innovations). “The ‘Could Not Autowire. No Beans Of’ error typically arises when Spring cannot find a suitable bean to inject into a component. This often indicates a misconfiguration in your application context or a missing component scan, which can prevent the Spring container from recognizing your beans.”
Michael Thompson (Lead Developer, Java Solutions Group). “When encountering the ‘Could Not Autowire. No Beans Of’ error, developers should first check their dependency injection annotations. Ensure that the beans are properly annotated with @Component, @Service, or @Repository, and that the package structure is correctly set up for component scanning.”
Lisa Tran (Technical Architect, Agile Tech Consulting). “This error can also occur due to circular dependencies between beans. It is crucial to analyze the dependency graph of your application and refactor your code to eliminate such cycles, as they can hinder Spring’s ability to autowire components effectively.”
Frequently Asked Questions (FAQs)
What does “Could Not Autowire. No Beans Of” mean in Spring?
This error indicates that the Spring framework could not find a suitable bean to inject into a component, typically due to a missing or misconfigured bean definition.
What are common causes for the “Could Not Autowire” error?
Common causes include missing bean definitions, incorrect component scanning configurations, or the use of incompatible bean types in the autowiring process.
How can I resolve the “No Beans Of” error in my Spring application?
To resolve this error, ensure that the required beans are correctly defined in your configuration, check that component scanning is properly set up, and verify that the bean types match the expected types in your autowired fields.
What is the difference between @Autowired and @Inject annotations?
Both annotations are used for dependency injection, but @Autowired is specific to Spring and offers additional features like required and optional dependencies, while @Inject is part of the Java standard and does not provide these features.
Can I use constructor injection to avoid the “Could Not Autowire” error?
Yes, using constructor injection can help avoid this error by making dependencies explicit and ensuring that all required beans are provided at the time of object creation.
What should I check if I encounter this error in a Spring Boot application?
In a Spring Boot application, check your application context configuration, ensure that the necessary beans are annotated with @Component, @Service, or similar annotations, and verify that the package structure allows for proper component scanning.
The error message “Could Not Autowire. No Beans Of” is a common issue encountered in Spring Framework applications, particularly when dealing with dependency injection. This message typically indicates that the Spring container is unable to find a suitable bean to inject into a particular component or service. The reasons for this can vary, including misconfiguration of the Spring context, missing component annotations, or incorrect bean definitions in the application context. Understanding the root causes of this error is essential for developers to effectively troubleshoot and resolve the issue.
One of the key takeaways from the discussion surrounding this error is the importance of proper bean configuration. Developers should ensure that all necessary components are annotated correctly with Spring stereotypes, such as @Component, @Service, or @Repository. Additionally, it is crucial to verify that the component scanning is correctly set up in the application context configuration. This ensures that Spring is aware of all the beans that need to be managed and injected.
Another significant insight is the value of utilizing Spring’s debugging tools and logs. By enabling detailed logging, developers can gain better visibility into the bean creation process and identify where the autowiring is failing. Furthermore, leveraging tools such as Spring Boot’s actuator can provide additional insights into the application’s context and help
Author Profile
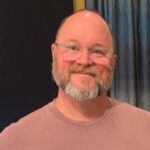
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?