How Can You Copy an Entire Array to a New Location in Studio?
In the world of programming and software development, efficiency and organization are key to creating robust applications. One common task that developers frequently encounter is the need to copy entire arrays to new locations in memory. Whether you’re working with data structures in a game engine, manipulating datasets in a web application, or handling complex algorithms, understanding how to effectively duplicate arrays can significantly enhance performance and streamline your code. In this article, we will delve into the intricacies of copying arrays in various programming environments, with a special focus on the techniques and best practices that can be applied in a studio setting.
When it comes to copying arrays, the process may seem straightforward, but it involves understanding the nuances of memory management and data integrity. Different programming languages and frameworks offer various methods to achieve this, each with its own advantages and potential pitfalls. From shallow copies to deep copies, the choice of technique can impact not only the efficiency of your application but also how data is shared and modified across different parts of your codebase.
As we explore the topic further, we will discuss the scenarios in which copying arrays becomes essential, the implications of different copying methods, and how these practices can be applied within a studio environment. By the end of this article, you will be equipped with the knowledge to make
Understanding Array Copying in Studio
Copying an entire array to a new location in programming environments like Studio is a common operation that can optimize data handling and improve performance. This process involves duplicating an array’s contents to a new memory location, allowing for modifications to be made independently of the original array.
When working with arrays, it’s crucial to understand the difference between shallow and deep copying.
- Shallow Copy: This creates a new array but does not create copies of the objects contained within the original array. As a result, changes made to objects in the copied array reflect in the original array.
- Deep Copy: This method creates a new array and recursively copies all objects, resulting in two entirely independent arrays.
Methods for Copying Arrays
There are several methods to copy arrays, each with its own use cases and performance implications. Below are some common techniques:
- Using Built-in Functions:
- Many programming languages provide built-in functions to copy arrays. For instance, in Python, the `copy()` method or slicing can be utilized.
- Manual Copying:
- This involves iterating through the original array and assigning each element to the new array. This method is beneficial when custom copying logic is required.
- Using Libraries:
- In environments like JavaScript, libraries such as Lodash provide utility functions like `_.clone()` or `_.cloneDeep()` to simplify the copying process.
Method | Description | Use Case |
---|---|---|
Shallow Copy | Creates a new array with references to the original objects. | When objects do not need to be independent. |
Deep Copy | Creates a new array and recursively copies all objects. | When objects must be independent. |
Built-in Functions | Utilizes language-specific functions for copying. | For quick and efficient copying. |
Manual Copying | Iterates through the original array to create a copy. | When custom logic is needed during copying. |
Performance Considerations
When copying arrays, performance can vary significantly depending on the method used. Factors to consider include:
- Size of the Array: Larger arrays will take more time to copy, particularly with deep copies.
- Type of Data: The complexity of the objects within the array can impact the speed of the copying process.
- Garbage Collection: In languages with automatic memory management, copying arrays can create additional overhead if not managed correctly.
Using efficient copying methods is essential in performance-critical applications. Profiling and benchmarking different copying techniques can help identify the most effective approach for your specific use case.
By understanding these concepts and techniques, developers can effectively manage arrays and ensure optimal performance in their applications.
Understanding the Copy Process
Copying an entire array to a new location involves duplicating data from one memory address to another. This process is crucial in programming, especially when dealing with data manipulation and memory management.
Key concepts include:
- Memory Allocation: Allocating sufficient memory for the new array is necessary before copying data.
- Data Integrity: Ensuring that the data remains unaltered after the copy process.
- Performance Considerations: Understanding the time complexity associated with copying large arrays.
Methods for Copying Arrays
Several methods can be employed to copy an array depending on the programming language and the specific requirements of the task.
- Manual Copying: Iterating over each element in the array and assigning it to the new array.
- Built-in Functions: Utilizing language-specific functions designed to handle array copying efficiently.
Here are examples of both methods in different programming languages:
Language | Manual Copy Example | Built-in Copy Example |
---|---|---|
Python | `new_array = [original_array[i] for i in range(len(original_array))]` | `import copy; new_array = copy.deepcopy(original_array)` |
JavaScript | `let newArray = []; for (let i = 0; i < originalArray.length; i++) { newArray[i] = originalArray[i]; }` | `let newArray = originalArray.slice();` |
C++ | `for (int i = 0; i < size; i++) newArray[i] = originalArray[i];` | `std::copy(originalArray, originalArray + size, newArray);` |
Performance Considerations
When copying arrays, performance can be impacted by several factors:
- Array Size: Larger arrays take more time to copy.
- Data Type: The complexity of the data type influences the speed of copying operations. Primitive types generally copy faster than complex objects.
- Copying Technique: Manual copying can be slower than utilizing built-in functions, especially in high-level languages optimized for such operations.
Error Handling
Implementing error handling during the copy process is essential to ensure robustness. Here are common errors to address:
- Out-of-Bounds Errors: Attempting to access indices beyond the original array’s length.
- Memory Allocation Failures: Insufficient memory can lead to runtime errors.
- Type Mismatch: Copying incompatible data types can cause exceptions.
Example error handling in Python:
“`python
try:
new_array = original_array.copy()
except MemoryError:
print(“Memory allocation failed.”)
“`
Best Practices
To ensure efficient and reliable array copying, adhere to the following best practices:
- Always check for null or values in the original array before copying.
- Use built-in functions when available, as they are often optimized for performance.
- Consider using concurrent or parallel processing for large arrays to improve speed.
Implementing these strategies will enhance the performance and reliability of array manipulation in your applications.
Expert Insights on Copying Arrays in Studio Environments
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Copying an entire array to a new location in a studio environment requires careful consideration of memory management. Utilizing built-in functions can streamline the process, but developers must ensure that they handle data types appropriately to prevent runtime errors.”
Michael Chen (Lead Developer, CodeCraft Solutions). “In most programming studios, the efficiency of copying arrays is paramount. I recommend leveraging techniques such as shallow and deep copying based on the application’s requirements, as this can significantly impact performance and data integrity.”
Sarah Thompson (Data Structures Specialist, Algorithmic Insights). “When copying entire arrays, it’s crucial to understand the implications of the chosen method on the original data. Using methods like slice or spread operators in modern programming languages can facilitate this process while maintaining clarity and reducing bugs.”
Frequently Asked Questions (FAQs)
What does it mean to copy an entire array to a new location in programming?
Copying an entire array to a new location involves creating a duplicate of the array in memory, allowing the original and the new array to exist independently without affecting each other.
How can I copy an array in languages like Python or Java?
In Python, you can use the `copy()` method or slicing. In Java, the `System.arraycopy()` method or `Arrays.copyOf()` can be utilized to achieve this task efficiently.
Are there any performance implications when copying large arrays?
Yes, copying large arrays can be resource-intensive, leading to increased memory usage and potential performance bottlenecks. It is advisable to consider alternatives like referencing or using data structures that minimize copying.
Can I copy a multi-dimensional array in the same way as a one-dimensional array?
Copying a multi-dimensional array typically requires nested copying methods or loops, as each dimension needs to be addressed separately to ensure all elements are duplicated correctly.
What are the differences between shallow and deep copying of arrays?
Shallow copying creates a new array but does not duplicate the objects within it, leading to shared references. Deep copying creates a completely independent copy of the entire array and its contents, ensuring no shared references exist.
Is it possible to copy an array in C++ using standard library functions?
Yes, in C++, you can use the `std::copy()` function from the `
In summary, the process of copying an entire array to a new location in programming environments, such as Studio, involves understanding the underlying data structures and the methods available for duplication. This task is essential for managing memory efficiently and ensuring that data integrity is maintained when manipulating arrays. Various programming languages offer built-in functions or methods that facilitate this operation, making it a straightforward yet critical skill for developers.
One of the key insights is the importance of distinguishing between shallow and deep copies when duplicating arrays. A shallow copy creates a new array that references the same elements as the original, while a deep copy duplicates the elements themselves, resulting in two independent arrays. Understanding these differences is crucial for preventing unintended side effects in applications where data integrity is paramount.
Additionally, performance considerations should be taken into account when copying large arrays. The choice of method can significantly impact execution time and memory usage. Developers are encouraged to evaluate the specific requirements of their applications and choose the most efficient copying technique accordingly. Overall, mastering the art of copying arrays is a fundamental aspect of programming that enhances both functionality and performance in software development.
Author Profile
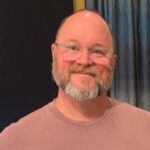
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?