How Can You Convert a Java.Lang.String to a JSON Object?
In the world of software development, the ability to seamlessly manipulate data formats is crucial, especially when it comes to web applications and APIs. One common task that developers frequently encounter is converting a Java `String` into a JSON object. This operation is not merely a technical necessity; it serves as a bridge between different data representations, enabling applications to communicate effectively and efficiently. Whether you’re parsing data from a web service or preparing to send structured information to a client, mastering this conversion can significantly enhance your programming toolkit.
At its core, the conversion of a Java `String` to a JSON object involves understanding the structure of both data types. A `String` in Java can represent a wide array of information, but when it comes to JSON, that information must adhere to a specific format. This transformation is not just about syntax; it also requires developers to grasp the nuances of JSON’s hierarchical structure, including objects, arrays, and key-value pairs. With the right tools and libraries, this process can be streamlined, allowing developers to focus on building robust applications rather than getting bogged down in data formatting issues.
As we delve deeper into this topic, we will explore various methods and libraries available in Java for achieving this conversion. From using built-in functions to leveraging popular libraries like Gson and Jackson
Using JSON Libraries
To convert a `java.lang.String` to a JSON object in Java, utilizing a JSON library is essential. Various libraries facilitate this conversion process, each with its own strengths. The most commonly used libraries include:
- Jackson
- Gson
- org.json
Each of these libraries provides methods to parse a string into a JSON object efficiently.
Jackson Library Example
Jackson is a widely-used library for handling JSON data. To convert a string to a JSON object with Jackson, follow these steps:
- Include the Jackson dependency in your project. If you are using Maven, add the following to your `pom.xml`:
“`xml
“`
- Use the `ObjectMapper` class to perform the conversion. Here’s a code snippet demonstrating this:
“`java
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.JsonNode;
public class JsonExample {
public static void main(String[] args) {
String jsonString = “{\”name\”:\”John\”, \”age\”:30}”;
ObjectMapper objectMapper = new ObjectMapper();
try {
JsonNode jsonNode = objectMapper.readTree(jsonString);
System.out.println(jsonNode);
} catch (Exception e) {
e.printStackTrace();
}
}
}
“`
Gson Library Example
Gson, developed by Google, is another powerful library for JSON parsing. To convert a string to a JSON object using Gson:
- Add the Gson dependency to your project. For Maven, include:
“`xml
“`
- Utilize the `Gson` class as shown below:
“`java
import com.google.gson.JsonObject;
import com.google.gson.JsonParser;
public class GsonExample {
public static void main(String[] args) {
String jsonString = “{\”name\”:\”John\”, \”age\”:30}”;
JsonObject jsonObject = JsonParser.parseString(jsonString).getAsJsonObject();
System.out.println(jsonObject);
}
}
“`
Using org.json Library
The `org.json` library is a simple and straightforward way to handle JSON. To convert a string to a JSON object using this library:
- Include the following dependency:
“`xml
“`
- Convert the string as demonstrated below:
“`java
import org.json.JSONObject;
public class OrgJsonExample {
public static void main(String[] args) {
String jsonString = “{\”name\”:\”John\”, \”age\”:30}”;
JSONObject jsonObject = new JSONObject(jsonString);
System.out.println(jsonObject);
}
}
“`
Comparison of Libraries
The choice of library may depend on various factors such as performance, ease of use, and specific project requirements. Below is a comparison table highlighting key attributes of each library.
Library | Performance | Ease of Use | Features |
---|---|---|---|
Jackson | High | Moderate | Data binding, streaming API |
Gson | Moderate | High | Serialization/deserialization, type safety |
org.json | Low | High | Basic JSON manipulation |
By understanding the capabilities of these libraries, developers can select the most suitable one for converting `java.lang.String` to JSON objects based on their specific needs.
Understanding JSON in Java
In Java, JSON (JavaScript Object Notation) is a lightweight data interchange format that is easy for humans to read and write and easy for machines to parse and generate. The conversion of a `String` to a JSON object typically involves using libraries such as Jackson or Gson, which provide the necessary tools to manipulate JSON data effectively.
Using Jackson to Convert String to JSON Object
Jackson is a popular library for processing JSON data in Java. To convert a `String` to a JSON object using Jackson, follow these steps:
- Add Jackson Dependency: Include the Jackson library in your project. If you are using Maven, add the following dependency to your `pom.xml`:
“`xml
“`
- Convert String to JSON Object: Use the `ObjectMapper` class to convert a string into a JSON object.
“`java
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.JsonNode;
public class JsonExample {
public static void main(String[] args) {
String jsonString = “{\”name\”:\”John\”, \”age\”:30}”;
ObjectMapper objectMapper = new ObjectMapper();
try {
JsonNode jsonNode = objectMapper.readTree(jsonString);
System.out.println(jsonNode);
} catch (Exception e) {
e.printStackTrace();
}
}
}
“`
Using Gson to Convert String to JSON Object
Gson is another widely used library for handling JSON in Java. To convert a `String` to a JSON object using Gson, follow these steps:
- Add Gson Dependency: Include Gson in your project. For Maven, add the following dependency:
“`xml
“`
- Convert String to JSON Object: Use the `Gson` class to convert a string into a JSON object.
“`java
import com.google.gson.JsonElement;
import com.google.gson.JsonParser;
public class JsonExample {
public static void main(String[] args) {
String jsonString = “{\”name\”:\”John\”, \”age\”:30}”;
JsonElement jsonElement = JsonParser.parseString(jsonString);
System.out.println(jsonElement);
}
}
“`
Common Use Cases for String to JSON Conversion
The conversion from a string to a JSON object is common in various scenarios:
- API Responses: Handling responses from web services that return JSON data.
- Data Serialization: Storing or transmitting data in a structured format.
- Configuration Files: Reading configuration settings stored in JSON format.
Handling Errors During Conversion
When converting strings to JSON objects, several issues can arise. It is important to handle exceptions properly:
Error Type | Description | Solution |
---|---|---|
`JsonParseException` | Thrown when the input string is not valid JSON. | Validate the JSON string format. |
`IOException` | Thrown when there is a problem reading the input. | Check for input source issues. |
Implementing proper error handling ensures that your application can gracefully manage unexpected situations during JSON processing.
Expert Insights on Converting Java.Lang.String to JSON Objects
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Converting a Java.Lang.String to a JSON object is a straightforward process when using libraries like Jackson or Gson. These libraries provide robust methods to parse strings into JSON, ensuring that the data structure is correctly interpreted and utilized in Java applications.”
Michael Thompson (Lead Developer, CodeCraft Solutions). “When handling JSON data in Java, it is crucial to ensure that the string being converted is well-formed. Using the appropriate error handling mechanisms can prevent runtime exceptions and ensure a smooth conversion process, which is essential for maintaining application stability.”
Linda Zhang (Java Development Consultant, ByteWise Technologies). “I recommend always validating your JSON strings before conversion. Utilizing tools like JSONLint can help identify any issues in the string format, which can save developers significant time during the debugging process when working with Java applications.”
Frequently Asked Questions (FAQs)
How can I convert a Java.lang.String to a JSON object?
To convert a Java.lang.String to a JSON object, you can use libraries such as Gson or Jackson. For Gson, use `new Gson().fromJson(jsonString, JsonObject.class)`. For Jackson, use `new ObjectMapper().readTree(jsonString)`.
What libraries are commonly used for JSON conversion in Java?
Commonly used libraries for JSON conversion in Java include Gson, Jackson, and org.json. Each library has its own methods for parsing and creating JSON objects.
What is the difference between Gson and Jackson for JSON handling?
Gson is known for its simplicity and ease of use, while Jackson is more feature-rich and offers better performance for large datasets. Jackson also supports more advanced data binding features.
Can I convert a JSON string with nested objects to a Java object?
Yes, both Gson and Jackson can handle nested JSON structures. You can define Java classes that reflect the JSON structure and use the respective library to map the JSON string to these classes.
What exceptions should I handle when converting a string to a JSON object?
When converting a string to a JSON object, handle exceptions such as JsonSyntaxException in Gson and IOException or JsonProcessingException in Jackson to manage parsing errors effectively.
Is it possible to validate a JSON string before conversion?
Yes, it is possible to validate a JSON string before conversion. You can use a JSON schema validator or try parsing the string with a library like Gson or Jackson, which will throw an exception if the string is not valid JSON.
Converting a Java `String` to a JSON object is a common task in Java programming, especially when dealing with web services and APIs that communicate using JSON format. The conversion process typically involves parsing the `String` representation of JSON data into a structured format that can be easily manipulated within a Java application. This is often achieved using libraries such as Jackson or Gson, which provide robust methods for converting between Java objects and JSON.
One of the key insights is the importance of ensuring that the `String` is properly formatted as valid JSON before attempting the conversion. Invalid JSON will lead to parsing errors, which can disrupt the application flow. Therefore, developers should implement error handling mechanisms to gracefully manage any exceptions that arise during the conversion process. Additionally, understanding the structure of the JSON data being processed is crucial for effective data manipulation.
Another takeaway is the efficiency and flexibility offered by modern libraries like Jackson and Gson. These libraries not only facilitate the conversion of `String` to JSON objects but also provide features for serialization and deserialization, making it easier to work with complex data structures. By leveraging these libraries, developers can streamline their code and enhance maintainability, ultimately leading to more robust applications.
Author Profile
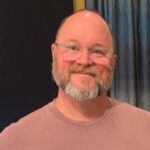
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?