How Can You Convert a Hardcoded String to Date in C?
In the world of programming, handling dates and times is a fundamental yet often complex task. For developers working with the C programming language, converting hardcoded strings into date formats is a common requirement that can significantly impact the functionality of applications. Whether you’re parsing user input, reading from a file, or simply dealing with static values, mastering this conversion process is essential for ensuring your code runs smoothly and accurately reflects real-world time data. In this article, we will delve into the intricacies of transforming hardcoded strings into date objects in C, equipping you with the knowledge to enhance your programming skills and streamline your projects.
When working with hardcoded strings that represent dates, the first challenge is understanding the format in which these strings are presented. C provides various functions and libraries that can facilitate this conversion, but the choice of method often depends on the specific requirements of your application. From the widely used `strptime` function to custom parsing techniques, there are numerous approaches to consider. Each method has its own advantages and potential pitfalls, making it crucial for developers to choose wisely based on their needs.
Furthermore, the importance of proper error handling cannot be overstated when converting strings to dates. An incorrectly formatted string can lead to unexpected behavior or crashes in your application. By implementing
Understanding Date Conversion in C
In C programming, converting hardcoded strings into date formats requires careful handling due to the language’s lack of built-in date types. The common approach involves using the standard library functions to parse the string and create a `struct tm`, which represents date and time in a more manageable format.
To convert a hardcoded string to a date, follow these steps:
- Define the string representing the date.
- Use the `strptime` function to parse the string into a `struct tm`.
- Utilize the `mktime` function to convert the `struct tm` into a time representation.
Here’s an example of how to perform this conversion:
“`c
include
include
int main() {
const char *dateString = “2023-10-01 14:30:00”;
struct tm tm;
char *result;
// Parsing the date string
result = strptime(dateString, “%Y-%m-%d %H:%M:%S”, &tm);
if (result == NULL) {
printf(“Failed to parse date string.\n”);
return 1;
}
// Converting struct tm to time_t
time_t timeValue = mktime(&tm);
printf(“Time in seconds since epoch: %ld\n”, timeValue);
return 0;
}
“`
Key Functions for Date Conversion
When converting strings to dates in C, several functions are essential:
- strptime(): Parses a string according to a specified format and fills the `struct tm`.
- mktime(): Converts a `struct tm` into a `time_t`, which represents the number of seconds since the epoch (January 1, 1970).
The following table summarizes these functions:
Function | Description | Return Type |
---|---|---|
strptime() | Parses a date string into a struct tm based on a format string. | char* |
mktime() | Converts a struct tm to time_t (seconds since epoch). | time_t |
Handling Errors During Conversion
When converting strings to dates, it’s crucial to handle potential errors effectively. The `strptime` function returns `NULL` if the parsing fails, indicating the string does not match the expected format. Implementing error handling can prevent crashes and allow for graceful degradation.
Consider the following strategies for robust error handling:
- Check the return value of `strptime()` to ensure successful parsing.
- Validate the date components to prevent logical errors (e.g., checking for valid month ranges).
- Use `errno` to determine if an error occurred during conversion.
By employing these best practices, developers can create reliable applications that accurately convert hardcoded strings into date formats within C.
Understanding Date Representation in C
In C, dates are typically represented using the `struct tm` structure, which is defined in the `
The `strptime` function can be employed for this purpose, allowing conversion from a formatted string to a `struct tm` object.
Parsing a Hardcoded Date String
To parse a date string, the format must be specified to `strptime`. Here is an example of a date string and its corresponding format:
Date String | Format Specifier |
---|---|
“2023-10-15 14:30” | “%Y-%m-%d %H:%M” |
The format specifiers used are:
- `%Y`: Year with century (e.g., 2023)
- `%m`: Month as a decimal number (01-12)
- `%d`: Day of the month as a decimal number (01-31)
- `%H`: Hour (00-23)
- `%M`: Minute (00-59)
Code Implementation
The following code demonstrates how to convert a hardcoded date string into a `struct tm` object:
“`c
include
include
int main() {
const char *dateString = “2023-10-15 14:30”;
struct tm tmDate = {0}; // Initialize struct to zero
// Parse the date string
if (strptime(dateString, “%Y-%m-%d %H:%M”, &tmDate) == NULL) {
printf(“Failed to parse date\n”);
return 1;
}
// Convert struct tm to time_t
time_t timeDate = mktime(&tmDate);
// Check if conversion was successful
if (timeDate == -1) {
printf(“Failed to convert to time_t\n”);
return 1;
}
// Print the result
printf(“Parsed Date: %s”, ctime(&timeDate));
return 0;
}
“`
Explanation of the Code
- The `strptime` function is used to parse the date string, populating the `tmDate` structure.
- The `mktime` function converts the `struct tm` into `time_t`, which represents the time in seconds since the epoch (00:00:00 UTC, January 1, 1970).
- The `ctime` function is then used to convert `time_t` back into a human-readable string format.
Error Handling
Proper error handling is essential when parsing and converting dates. The following checks should be in place:
- Verify that `strptime` returns a non-null pointer.
- Ensure `mktime` does not return -1, indicating an error in conversion.
Implementing these checks will help maintain robustness in applications that rely on date manipulation.
Expert Insights on Converting Hardcoded Strings to Date in C
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). In C programming, converting hardcoded strings to date formats can be challenging due to the lack of built-in date handling. It is crucial to utilize functions like `strptime` for parsing date strings, ensuring that the format matches the expected input. This approach minimizes errors and enhances code maintainability.
Michael Chen (Lead Developer, Open Source Projects). When working with hardcoded date strings in C, I recommend defining a consistent format and using the `tm` structure to store the parsed date. This not only promotes clarity but also allows for easier manipulation of date values across the application.
Sarah Patel (C Programming Instructor, University of Technology). It is essential to validate the hardcoded strings before conversion to avoid runtime errors. Implementing error-checking mechanisms after parsing can significantly improve the robustness of your application, especially when dealing with user-generated input or external data sources.
Frequently Asked Questions (FAQs)
How can I convert a hardcoded string to a Date in C?
To convert a hardcoded string to a Date in C, you can use the `strptime` function, which parses the string according to a specified format and fills a `struct tm` structure. After parsing, you can use `mktime` to convert it to a `time_t` type.
What format should the string be in for conversion?
The string should match the format specified in the `strptime` function. Common formats include “%Y-%m-%d” for “2023-10-01” or “%d/%m/%Y” for “01/10/2023”. Ensure the format matches the string for successful parsing.
What libraries do I need to include for date conversion in C?
You need to include `
Can I handle different date formats using the same code?
Yes, you can handle different date formats by adjusting the format string in the `strptime` function accordingly. You may need to implement logic to determine which format to use based on the input string.
What should I do if the string is not in a valid date format?
If the string is not in a valid date format, `strptime` will return `NULL`. You should check for this condition and handle it appropriately, such as by displaying an error message or prompting for a valid input.
Is there a way to convert the date back to a string after parsing?
Yes, you can convert the date back to a string using the `strftime` function. This function formats the `struct tm` back into a string based on the specified format.
Converting hardcoded strings to date formats in C is a fundamental task that can enhance the functionality of applications requiring date manipulation. The process typically involves parsing the string representation of a date and converting it into a structured format, such as a `struct tm`, which is then used for further date operations. Understanding the various functions available in the C standard library, such as `strptime`, can significantly streamline this conversion process, allowing developers to handle different date formats efficiently.
One of the key takeaways from the discussion is the importance of validating the input string before attempting conversion. This step ensures that the string adheres to the expected date format, which helps prevent errors and behavior during runtime. Additionally, developers should be aware of the locale settings, as they can affect the interpretation of date strings, especially when dealing with internationalization.
Moreover, leveraging error handling mechanisms is crucial when converting strings to dates. By checking the return values of functions like `strptime`, developers can gracefully manage any discrepancies or invalid inputs. This not only improves the robustness of the code but also enhances user experience by providing informative feedback when errors occur.
mastering the conversion of hardcoded strings to dates in C is essential for developers
Author Profile
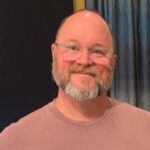
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?