Why Isn’t My Click Event Firing in JavaScript? Troubleshooting Tips and Solutions
### Introduction
In the world of web development, interactivity is king, and click events are the lifeblood of user engagement. Whether you’re building a sleek e-commerce site or a dynamic web application, ensuring that your click events fire correctly is crucial for a seamless user experience. However, many developers encounter the frustrating issue of click events not firing as expected. This problem can lead to lost functionality, user confusion, and ultimately, a negative impact on your site’s performance. In this article, we’ll explore the common pitfalls that can cause click events to fail, empowering you with the knowledge to troubleshoot and resolve these issues effectively.
### Overview
When a click event doesn’t fire in JavaScript, it can stem from a variety of sources, ranging from simple coding errors to more complex issues related to event delegation or browser compatibility. Understanding the underlying mechanics of event handling in JavaScript is essential for diagnosing these problems. Developers often overlook minor details, such as incorrect element selectors or missing event listeners, which can prevent the intended actions from triggering.
Moreover, the dynamic nature of modern web applications introduces additional layers of complexity. Frameworks and libraries can modify the way events are handled, leading to unexpected behavior. By delving into the common reasons behind click event failures, we can equip ourselves with strategies
Common Causes of Click Events Not Firing
Click events may not fire due to several common issues. Understanding these potential pitfalls is crucial for effective debugging. Here are some of the primary reasons:
- Element Overlap: Sometimes, elements can overlap on the page, causing clicks to be registered on the wrong element.
- JavaScript Errors: Any errors in JavaScript execution can prevent subsequent scripts, including click event handlers, from running.
- Event Delegation Issues: If an event is attached to a parent element and not the child, it may not fire if the child element is created after the event handler is defined.
- Preventing Default Behavior: If `event.preventDefault()` or `return ` is used improperly, it may prevent the click event from propagating.
- CSS Display or Visibility: Elements that are not visible or displayed (e.g., `display: none;` or `visibility: hidden;`) will not register click events.
Troubleshooting Strategies
When facing issues with click events not firing, several troubleshooting strategies can be employed:
- Check the Console for Errors: Open the browser’s developer tools and check the console for any JavaScript errors that may be affecting the execution of your scripts.
- Verify Element Visibility: Ensure that the element you are trying to click is visible and not hidden by other elements.
- Inspect Event Listeners: Use the Elements tab in developer tools to inspect if the event listeners are correctly attached to the elements.
- Use Debugging Statements: Insert `console.log()` statements in your event handler to determine if the function is being called.
Event Delegation Example
Event delegation can be a useful technique when working with dynamically generated elements. For instance, if you want to handle click events for a list of items generated at runtime, you can attach the event listener to a parent element.
javascript
document.getElementById(‘parentElement’).addEventListener(‘click’, function(event) {
if (event.target && event.target.matches(‘.childElement’)) {
console.log(‘Child element clicked:’, event.target);
}
});
This approach ensures that even if new `.childElement` items are added later, they will still trigger the click event.
Best Practices for Handling Click Events
To ensure your click events fire reliably, consider the following best practices:
- Use `addEventListener`: This method allows for multiple event listeners on the same element and is preferred over inline event attributes.
- Debounce Clicks: To prevent multiple event firings from rapid clicks, implement a debounce function.
- Avoid Inline JavaScript: Keeping JavaScript separate from HTML improves maintainability and reduces complexity.
- Testing Across Browsers: Always test click events across different browsers to ensure consistent behavior.
Sample Event Listener Table
Here’s a simple comparison of methods for adding click events:
Method | Description | Browser Compatibility |
---|---|---|
addEventListener | Attaches an event handler to the specified element. | Modern browsers (IE9+) |
onclick | Sets a single click event handler for the element. | All browsers |
jQuery .on() | Attaches event handlers to elements, including dynamically created ones. | All browsers (with jQuery) |
Common Causes of Click Events Not Firing
Click events in JavaScript can fail to trigger for several reasons. Understanding these causes can help in diagnosing and resolving issues effectively.
- Event Listener Not Attached: Ensure that the event listener is correctly attached to the intended DOM element. If the element is added dynamically, the listener may not work as expected.
- Element Visibility: If the element is hidden or has CSS properties such as `display: none` or `visibility: hidden`, the click event will not fire.
- JavaScript Errors: Any JavaScript errors occurring before the event listener is invoked can prevent the code from executing properly. Check the console for errors.
- Event Bubbling and Delegation Issues: If you are using event delegation, ensure that the parent element is present in the DOM when the event is triggered.
- Prevent Default Behavior: If the click event is on a link or form element, calling `event.preventDefault()` can stop the default action, but it should not prevent the event from firing unless mismanaged.
Debugging Techniques
When troubleshooting click event issues, consider implementing the following debugging techniques:
- Console Logging: Add `console.log()` statements within the event listener function to verify if the function is being executed.
- Inspect Element: Use developer tools to inspect the element and ensure that it is present in the DOM and visible.
- Check Event Listeners: Utilize `getEventListeners(element)` in the console to confirm if the click event is bound to the correct element.
- Breakpoint Debugging: Set breakpoints in your JavaScript code to step through and observe the flow of execution during the event trigger.
Best Practices for Click Events
To ensure that click events fire reliably, consider the following best practices:
- Use `addEventListener`: Always use `addEventListener` instead of inline event attributes for better separation of HTML and JavaScript.
- Check for Duplicates: Avoid attaching multiple event listeners to the same element unintentionally, which can lead to unexpected behavior.
- Ensure Accessibility: Utilize keyboard event handlers alongside mouse events to ensure that all users can interact with your application effectively.
- Debounce Clicks: Implement debouncing to prevent multiple rapid clicks from triggering the event multiple times unintentionally.
Example Code Snippet
The following example demonstrates proper click event handling:
javascript
document.addEventListener(‘DOMContentLoaded’, () => {
const button = document.getElementById(‘myButton’);
button.addEventListener(‘click’, (event) => {
console.log(‘Button clicked!’, event);
// Additional logic here
});
});
This code waits for the DOM to fully load before attaching a click event listener to a button, ensuring that the element is present and visible when the script runs.
Event Delegation Example
Event delegation can be useful when dealing with dynamically added elements. Here’s an example:
javascript
document.getElementById(‘parentElement’).addEventListener(‘click’, (event) => {
if (event.target && event.target.matches(‘.childButton’)) {
console.log(‘Child button clicked!’, event);
// Additional logic here
}
});
In this case, the click event is attached to a parent element, allowing it to capture clicks on child elements even if they are added after the event listener was set.
While troubleshooting click events in JavaScript, it is crucial to investigate common causes, employ effective debugging strategies, and adhere to best practices for reliable functionality.
Understanding Why Click Events May Not Fire in JavaScript
Jessica Lin (Senior Frontend Developer, Tech Innovations Inc.). “One common reason for click events not firing in JavaScript is improper event binding. Developers often forget to bind the event to the correct element, especially when dynamically generating content. Ensuring that the event listener is attached after the DOM is fully loaded can mitigate this issue.”
Michael Chen (JavaScript Framework Specialist, CodeCraft Academy). “Another frequent cause of click events failing to trigger is the presence of CSS properties like ‘pointer-events: none’ on the target element. This property disables any mouse interactions, including click events. It’s crucial to inspect styles when debugging event issues.”
Sarah Patel (Web Application Architect, Digital Solutions Group). “Event delegation is a powerful technique that can help manage click events more effectively. However, if the parent element does not exist in the DOM at the time of event binding, the click events will not fire. Always ensure that the parent element is present when setting up delegation.”
Frequently Asked Questions (FAQs)
What are common reasons for a click event not firing in JavaScript?
Common reasons include incorrect event listener attachment, elements being dynamically added after the listener is set, event propagation issues, or JavaScript errors in the console preventing execution.
How can I ensure my click event is properly attached to an element?
Use the `addEventListener` method after the DOM has fully loaded. Ensure you are targeting the correct element by checking its existence in the DOM before attaching the listener.
What is event delegation and how can it help with click events?
Event delegation involves attaching a single event listener to a parent element rather than individual child elements. This approach can improve performance and ensure that events are captured for dynamically added elements.
How can I troubleshoot a click event that is not firing?
Check for JavaScript errors in the console, confirm that the event listener is attached correctly, ensure the target element is not covered by another element, and verify that the element is not disabled or removed from the DOM.
Can CSS properties affect whether a click event fires?
Yes, certain CSS properties like `pointer-events: none;` can prevent click events from firing. Ensure that the element is interactive and not styled in a way that disables pointer events.
What tools can I use to debug click events in JavaScript?
Use browser developer tools, such as the Console and Elements tabs, to inspect elements, check event listeners, and monitor JavaScript execution. Tools like breakpoints can also help trace the flow of code execution.
In summary, the issue of a click event not firing in JavaScript can stem from various factors, including incorrect event listener attachment, issues with event propagation, or conflicts with other scripts. Proper debugging techniques, such as using browser developer tools to inspect elements and monitor events, are essential in identifying the root cause of the problem. Additionally, ensuring that the event listener is attached after the DOM is fully loaded can prevent common pitfalls associated with timing issues.
Key takeaways from the discussion include the importance of understanding the event delegation model, which allows for more efficient event handling, especially in dynamic content scenarios. Furthermore, developers should be aware of potential pitfalls, such as using the wrong event type or failing to bind the context correctly, which can lead to unexpected behavior. Testing across different browsers and devices can also help ensure consistent functionality.
Ultimately, resolving click event issues requires a methodical approach to debugging and a solid understanding of JavaScript event handling. By following best practices and leveraging the tools available, developers can effectively troubleshoot and implement robust click event functionality in their applications.
Author Profile
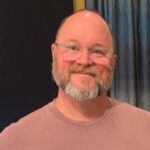
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?