How Can You Effectively Use a Case Statement in a Where Condition?
In the world of SQL and database management, the ability to manipulate and filter data effectively is paramount. One powerful tool at a developer’s disposal is the CASE statement, which allows for conditional logic within queries. While many are familiar with its use in the SELECT clause, its application in the WHERE condition can significantly enhance the flexibility and precision of data retrieval. Imagine being able to apply multiple criteria dynamically, tailoring your queries to meet specific needs without resorting to complex joins or subqueries. This article delves into the intricacies of using the CASE statement within the WHERE condition, illuminating its potential to streamline your SQL queries and improve the efficiency of your data handling.
Understanding how to integrate a CASE statement into a WHERE clause opens up a new realm of possibilities for data filtering. This technique allows developers to implement conditional logic directly in their queries, making it easier to handle various scenarios without cluttering the code with multiple AND/OR conditions. By employing this method, you can create more readable and maintainable SQL statements that adapt to varying requirements, all while ensuring optimal performance.
As we explore this topic further, we will uncover the syntax and practical applications of the CASE statement in the WHERE condition, providing you with the tools to enhance your SQL skill set. Whether you’re a seasoned database administrator or
Using Case Statements in Where Conditions
In SQL, a CASE statement allows for conditional logic to be applied directly within a query. While traditionally used in the SELECT clause to return specific values based on certain conditions, it can also be utilized in the WHERE clause to filter records based on complex conditions. This can be particularly useful when you need to apply different criteria based on varying circumstances.
When implementing a CASE statement in a WHERE condition, it is essential to understand that it evaluates conditions sequentially and returns the first true result. This capability allows for dynamic filtering based on multiple conditions.
Here is the syntax for using a CASE statement in a WHERE clause:
“`sql
SELECT column1, column2
FROM table_name
WHERE column3 =
CASE
WHEN condition1 THEN result1
WHEN condition2 THEN result2
ELSE default_result
END;
“`
This structure enables you to define various conditions and specify what should happen when those conditions are met. However, it’s important to note that the CASE statement must be part of a larger logical condition within the WHERE clause.
Examples of CASE Statements in WHERE Conditions
Consider a database of employees where you want to filter records based on different job titles and their corresponding department codes. A CASE statement can be effectively applied as follows:
“`sql
SELECT employee_id, employee_name
FROM employees
WHERE department_code =
CASE
WHEN job_title = ‘Manager’ THEN ‘MGR’
WHEN job_title = ‘Engineer’ THEN ‘ENG’
ELSE ‘OTH’
END;
“`
In this example, the query selects employees whose department codes are determined by their job titles. Managers are assigned to the ‘MGR’ department, engineers to ‘ENG’, and all others default to ‘OTH’.
Advantages of Using CASE in WHERE Clauses
- Flexibility: Allows for dynamic filtering based on varying conditions without needing multiple OR statements.
- Readability: Improves the clarity of SQL queries by consolidating complex conditions into a single expression.
- Efficiency: Reduces the need for subqueries, potentially improving query performance.
Job Title | Department Code |
---|---|
Manager | MGR |
Engineer | ENG |
Sales Associate | SA |
HR Specialist | HR |
By employing a CASE statement in the WHERE clause, you can create comprehensive filters that adapt to specific business logic, enhancing both the functionality and clarity of your SQL queries. This method is particularly beneficial in complex databases where straightforward filtering may not suffice.
Understanding the CASE Statement
The `CASE` statement in SQL is a powerful tool that allows for conditional logic within your queries. It can be used to return specific values based on different conditions. This is particularly useful in scenarios where you need to evaluate data and provide different outputs based on certain criteria.
Syntax of the CASE Statement
The basic syntax of a `CASE` statement is as follows:
“`sql
CASE
WHEN condition1 THEN result1
WHEN condition2 THEN result2
…
ELSE default_result
END
“`
Types of CASE Statements
- Simple CASE Statement: Compares an expression to a set of simple expressions to determine the result.
- Searched CASE Statement: Evaluates a set of Boolean expressions to determine the result.
Examples
- Simple CASE Example:
“`sql
SELECT employee_name,
CASE department_id
WHEN 1 THEN ‘Sales’
WHEN 2 THEN ‘HR’
ELSE ‘Other’
END AS department_name
FROM employees;
“`
- Searched CASE Example:
“`sql
SELECT employee_name,
CASE
WHEN salary < 30000 THEN 'Low'
WHEN salary BETWEEN 30000 AND 60000 THEN 'Medium'
ELSE 'High'
END AS salary_range
FROM employees;
```
Using CASE in the WHERE Clause
In SQL, you can also incorporate `CASE` statements within the `WHERE` clause to implement complex filtering logic. This approach allows for more dynamic and conditional queries.
Example of CASE in WHERE Clause
Consider the following example where we want to filter results based on different conditions:
“`sql
SELECT employee_name
FROM employees
WHERE department_id =
CASE
WHEN :condition1 THEN 1
WHEN :condition2 THEN 2
ELSE 3
END;
“`
In this case, the `:condition1` and `:condition2` would be placeholders for actual values or parameters that you define when executing the SQL statement.
Use Cases for CASE in WHERE Clause
- Dynamic Filtering: Change the filtering criteria based on user inputs or application logic.
- Complex Logic: Implement complex business rules directly within the query execution.
- Conditional Aggregation: Combine filtering with aggregation functions to create more insightful reports.
Best Practices for Using CASE Statements
To ensure clarity and maintainability in your SQL queries, consider the following best practices:
- Keep It Simple: Avoid overly complex `CASE` statements that may reduce readability.
- Comment Your Logic: Document the purpose of each condition within the `CASE` statement for future reference.
- Test Thoroughly: Validate the results by running test queries to ensure the logic behaves as expected.
- Use Clear Aliases: When returning calculated columns, use clear and descriptive aliases to enhance readability.
Performance Considerations
While `CASE` statements are powerful, they can impact performance. Consider these points:
Factor | Consideration |
---|---|
Complexity of Conditions | More complex conditions may slow down execution. |
Number of Rows Processed | Large datasets can lead to longer query times. |
Index Usage | Ensure that indexes are utilized effectively to maintain performance. |
By understanding how to effectively incorporate `CASE` statements in the `WHERE` clause, you can create more dynamic and flexible SQL queries that meet complex business requirements.
Expert Insights on Using Case Statements in Where Conditions
Dr. Emily Chen (Data Analyst, Tech Insights Journal). “Utilizing a CASE statement within a WHERE condition can significantly enhance query flexibility by allowing for conditional logic directly in the filtering process. This approach not only streamlines complex queries but also improves readability, making it easier for analysts to understand the intent behind data retrieval.”
Michael Thompson (Database Architect, SQL Solutions Inc.). “Incorporating CASE statements in WHERE clauses can be particularly beneficial when dealing with multiple conditions that would otherwise require extensive OR logic. This method reduces the potential for errors and optimizes performance by consolidating logic into a single, coherent expression.”
Sarah Patel (Senior Software Engineer, DataTech Innovations). “While CASE statements can enhance the functionality of WHERE conditions, it is essential to use them judiciously. Overcomplicating queries with nested CASE statements can lead to performance issues and make maintenance challenging. Always consider the trade-offs between complexity and clarity.”
Frequently Asked Questions (FAQs)
What is a case statement in SQL?
A case statement in SQL is a conditional expression that allows you to perform different actions based on specific conditions. It evaluates a series of conditions and returns a value based on the first true condition.
Can a case statement be used in the WHERE clause?
Yes, a case statement can be used in the WHERE clause to conditionally filter records based on multiple criteria. It allows for more complex filtering logic beyond simple comparisons.
How do you structure a case statement in the WHERE condition?
The structure typically involves the CASE keyword followed by WHEN clauses for each condition, and an END statement. For example: `WHERE (CASE WHEN condition1 THEN value1 WHEN condition2 THEN value2 END) = some_value`.
What are the advantages of using a case statement in the WHERE clause?
Using a case statement in the WHERE clause provides flexibility in filtering data based on complex conditions. It enhances readability and allows for dynamic filtering based on varying criteria.
Are there any performance considerations when using a case statement in the WHERE clause?
Yes, using a case statement can impact performance, especially with large datasets. It may lead to increased complexity in query execution plans, so it is advisable to test performance implications in your specific context.
Can you provide an example of a case statement in the WHERE clause?
Certainly. An example would be: `SELECT * FROM employees WHERE (CASE WHEN department = ‘Sales’ THEN salary ELSE bonus END) > 50000;` This filters employees based on their salary or bonus depending on their department.
The use of a CASE statement in the WHERE condition of SQL queries is a powerful technique that enhances the flexibility and functionality of data retrieval. By allowing conditional logic within the WHERE clause, developers can create more dynamic queries that cater to various scenarios without the need for multiple separate queries. This capability is particularly beneficial in complex data environments where conditions may vary based on user input or other factors.
One of the primary advantages of incorporating a CASE statement in the WHERE clause is the ability to streamline queries. Instead of constructing multiple queries to handle different conditions, a single query can be crafted to evaluate various cases, thus improving performance and maintainability. This approach not only reduces the amount of code but also minimizes the risk of errors that can arise from managing multiple query versions.
Additionally, using a CASE statement in the WHERE condition enhances readability and clarity. It allows developers to express complex logic in a more structured manner, making it easier for others to understand the intent behind the query. This clarity is crucial in collaborative environments where multiple team members may interact with the same codebase, ensuring that the logic is transparent and easily interpretable.
leveraging a CASE statement in the WHERE condition is an effective strategy for optimizing SQL queries. It provides
Author Profile
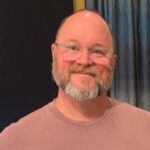
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?