Why Am I Facing the ‘Cannot Resolve Scoped Service From Root Provider’ Error in My Application?
In the world of modern software development, the intricacies of dependency injection can often lead to perplexing challenges. One such challenge that developers frequently encounter is the error message: “Cannot Resolve Scoped Service From Root Provider.” This seemingly cryptic message can halt progress and leave even seasoned programmers scratching their heads. Understanding the underlying principles of dependency injection, particularly in frameworks like ASP.NET Core, is essential for navigating these pitfalls and ensuring robust application architecture.
This article delves into the nuances of scoped services and their lifecycle, shedding light on why this error occurs and how to effectively troubleshoot it. We will explore the fundamental concepts of service lifetimes, including transient, scoped, and singleton services, and how they interact within the dependency injection container. By grasping these concepts, developers can better manage their application’s dependencies and avoid common misconfigurations that lead to runtime errors.
Furthermore, we will discuss best practices for structuring services and the importance of context when resolving dependencies. Whether you are a novice developer or an experienced architect, this exploration will equip you with the insights needed to overcome the “Cannot Resolve Scoped Service From Root Provider” error and enhance the overall stability and maintainability of your applications. Join us as we unravel the complexities of dependency injection and empower you to build more
Understanding Scoped Services
Scoped services in dependency injection (DI) are designed to exist for a single request or session. This means that they are instantiated once per request and can be shared within the same request but are not shared between different requests. This behavior is essential for maintaining the integrity of user-specific data or operations, as it prevents the leakage of data across different requests.
When using scoped services, it’s important to register them correctly in the DI container. Typically, this is done in the `Startup.cs` file within the `ConfigureServices` method. Here’s an example of how to register a scoped service:
“`csharp
public void ConfigureServices(IServiceCollection services)
{
services.AddScoped
}
“`
This registration ensures that `MyService` is instantiated once per request. However, issues can arise if these scoped services are requested from a root provider, leading to the error `Cannot Resolve Scoped Service From Root Provider`.
Common Causes of the Error
The error `Cannot Resolve Scoped Service From Root Provider` occurs when a scoped service is requested from a context that does not have an active scope, such as:
- Singleton Services: If a singleton service tries to resolve a scoped service, it will throw this error because singletons are created once for the application lifetime, while scoped services are created per request.
- Background Tasks: Tasks that run outside of the request scope, such as hosted services or background tasks, can also lead to this error when they attempt to access scoped services.
- Static Methods or Classes: Any attempt to resolve a scoped service from a static context will result in this error since static members do not have access to the DI scope.
Solutions to Resolve the Error
To address the `Cannot Resolve Scoped Service From Root Provider` error, several strategies can be employed:
- Use a Service Scope: When working with background tasks, explicitly create a service scope within the method that runs the task:
“`csharp
public class MyBackgroundService : BackgroundService
{
private readonly IServiceProvider _serviceProvider;
public MyBackgroundService(IServiceProvider serviceProvider)
{
_serviceProvider = serviceProvider;
}
protected override async Task ExecuteAsync(CancellationToken stoppingToken)
{
using (var scope = _serviceProvider.CreateScope())
{
var myService = scope.ServiceProvider.GetRequiredService
// Use myService here
}
}
}
“`
- Avoid Singleton Dependencies: If possible, refactor your singleton services to avoid direct dependencies on scoped services. Instead, consider using factory patterns or passing scoped services as parameters.
- Refactor Static Methods: If you have static methods that need to use scoped services, refactor them to accept service instances as parameters instead of resolving them directly.
Impact of Scoped Service Misuse
Improper handling of scoped services can lead to various issues, including:
Issue | Description |
---|---|
Data Leakage | User-specific data can leak across requests. |
Memory Leaks | Scoped services may not be disposed of correctly. |
Performance Overhead | Creating unnecessary instances can affect performance. |
Ensuring that scoped services are used correctly is vital for the reliability and performance of your applications. By adhering to the principles of dependency injection and understanding the lifecycle of services, developers can avoid common pitfalls and create robust applications.
Understanding Scoped Services
Scoped services in dependency injection are instantiated once per request. This means they are typically created when a web request is made and disposed of when the request is completed. Common scenarios for using scoped services include:
- Web applications: Where each HTTP request is treated as a separate scope.
- Database contexts: Such as Entity Framework, which should be scoped to the lifetime of a request to ensure proper tracking and disposal of resources.
Using scoped services allows for better management of resources, particularly when handling operations that require a fresh instance per request while maintaining state within that request.
Common Causes of the Error
The error message “Cannot resolve scoped service from root provider” typically arises from attempting to resolve a scoped service from a singleton or root context. This can happen due to several reasons, including:
- Service Lifetime Mismatch: Attempting to inject a scoped service into a singleton service.
- Incorrect Dependency Injection Setup: Misconfiguring the service lifetimes during registration.
- Manual Service Resolution: Using service providers directly in a singleton or static context.
Best Practices for Resolving the Error
To avoid encountering the “Cannot resolve scoped service from root provider” error, follow these best practices:
- Service Lifetime Alignment: Ensure that your services are aligned in terms of their lifetimes. Avoid injecting scoped services into singleton services.
- Use Factory Methods: If you need to access a scoped service from a singleton, consider using a factory method to resolve the scoped service within the appropriate context.
- Use Middleware: If you need to access scoped services in a middleware, ensure they are resolved within the request pipeline.
Example Implementation
Here is an example demonstrating how to properly configure and use scoped services:
“`csharp
public void ConfigureServices(IServiceCollection services)
{
services.AddScoped
services.AddSingleton
}
“`
In this scenario, if `MySingletonService` requires `IMyScopedService`, consider the following approach:
“`csharp
public class MySingletonService : IMySingletonService
{
private readonly IServiceProvider _serviceProvider;
public MySingletonService(IServiceProvider serviceProvider)
{
_serviceProvider = serviceProvider;
}
public void SomeMethod()
{
using (var scope = _serviceProvider.CreateScope())
{
var scopedService = scope.ServiceProvider.GetRequiredService
// Use scopedService here
}
}
}
“`
Debugging Techniques
When troubleshooting the “Cannot resolve scoped service from root provider” error, consider these techniques:
- Check Service Registrations: Review your `ConfigureServices` method to ensure all services are registered with the correct lifetimes.
- Review Service Dependencies: Trace the dependencies of your services to ensure they align properly with their expected lifetimes.
- Use Logging: Implement logging to capture the service resolution process, helping to identify where the mismatch occurs.
By following these guidelines, you can effectively manage the lifetimes of your services and avoid common pitfalls associated with dependency injection in .NET applications.
Understanding the Challenges of Scoped Services in Dependency Injection
Dr. Emily Carter (Software Architect, Tech Innovations Inc.). “The error ‘Cannot Resolve Scoped Service From Root Provider’ typically arises when attempting to inject a scoped service into a singleton. This is a fundamental issue in dependency injection lifetimes, as scoped services are designed to be created per request, while singletons persist for the entire application lifecycle.”
Michael Chen (Lead Developer, CodeCraft Solutions). “To resolve this issue, developers should consider redesigning their service lifetimes. One effective strategy is to ensure that the service being injected is either a singleton or to use factory patterns that allow for scoped services to be created within the appropriate context.”
Sarah Thompson (Senior Software Engineer, DevOps Dynamics). “Often, the ‘Cannot Resolve Scoped Service From Root Provider’ error indicates a deeper architectural flaw. It is crucial to evaluate the service registration in your application’s startup configuration and ensure that the dependencies align with the intended lifetimes to avoid such conflicts.”
Frequently Asked Questions (FAQs)
What does “Cannot Resolve Scoped Service From Root Provider” mean?
This error indicates that an attempt was made to resolve a service that is registered with a scoped lifetime from a singleton or root service provider. Scoped services are designed to be created once per request, whereas root providers do not have a request context.
How can I fix the “Cannot Resolve Scoped Service From Root Provider” error?
To resolve this error, ensure that scoped services are only requested within the context of a scope, such as within a web request or a specific operation that creates a scope. Alternatively, consider changing the lifetime of the service to singleton if it does not require scoped behavior.
What are the different service lifetimes in dependency injection?
In dependency injection, there are three main service lifetimes: Singleton (one instance for the entire application), Scoped (one instance per request), and Transient (a new instance each time it is requested). Understanding these lifetimes is crucial for proper service resolution.
Can I use a scoped service in a singleton service?
Directly using a scoped service within a singleton service is not recommended, as it leads to the “Cannot Resolve Scoped Service From Root Provider” error. If necessary, create a scope within the singleton to resolve the scoped service correctly.
What is the recommended approach for using scoped services in ASP.NET Core?
In ASP.NET Core, the recommended approach is to inject scoped services into controllers or other services that are themselves scoped. Avoid injecting scoped services into singleton services or into the application’s root service provider.
Are there any patterns to avoid this error in ASP.NET Core applications?
Yes, common patterns include using factories or service locators to create scopes when needed, or restructuring your services to ensure that scoped services are only used within the appropriate context. This helps maintain the integrity of service lifetimes and avoids resolution errors.
The issue of “Cannot Resolve Scoped Service From Root Provider” typically arises in dependency injection scenarios within applications that utilize frameworks like ASP.NET Core. This error indicates that a service registered with a scoped lifetime is being requested from a root provider, which is not permissible. Scoped services are designed to be instantiated once per request, ensuring that they maintain state throughout the lifecycle of that request. However, when they are accessed from a singleton or root context, the framework cannot provide the necessary instance, leading to this error.
Understanding the lifecycle of services—transient, scoped, and singleton—is crucial for effective dependency injection management. Developers must ensure that services are resolved within the appropriate context. For example, if a scoped service is needed, it should be requested within a controller or a service that is also scoped to the request, rather than from a singleton service that persists beyond the request’s lifespan. This adherence to the lifecycle rules is essential for maintaining application stability and avoiding runtime errors.
Key takeaways from this discussion include the importance of correctly configuring service lifetimes and being mindful of how services are resolved within the application. Developers should familiarize themselves with the dependency injection principles and best practices to avoid common pitfalls. Additionally, leveraging tools such as middleware or factory patterns
Author Profile
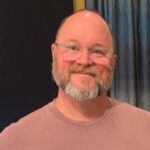
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?