Why Am I Encountering ‘Cannot Import Name ‘Default_Ciphers’ From ‘Urllib3.Util.Ssl_’ Error?
In the ever-evolving landscape of software development, even small changes in libraries can lead to significant headaches for developers. One such issue that has recently surfaced is the error message: “Cannot Import Name ‘Default_Ciphers’ From ‘Urllib3.Util.Ssl_'” This seemingly innocuous problem can disrupt workflows and lead to frustrating debugging sessions, especially for those relying on the popular `urllib3` library for HTTP requests in Python. As developers strive to build secure and efficient applications, understanding the underlying causes of this import error becomes crucial for maintaining code integrity and functionality.
The `Default_Ciphers` import error is emblematic of broader challenges faced when integrating third-party libraries into projects. It often arises from version discrepancies, deprecated features, or changes in library structure, which can leave developers scrambling to find solutions. This article delves into the implications of this error, exploring how it affects not only individual projects but also the broader ecosystem of Python development. By examining the roots of the issue and potential workarounds, we aim to equip developers with the knowledge they need to navigate these common pitfalls.
As we unpack the intricacies of the `Cannot Import Name ‘Default_Ciphers’` error, we’ll also highlight best practices for managing dependencies and ensuring compatibility within your projects
Understanding the Import Error
The error message “Cannot Import Name ‘Default_Ciphers’ From ‘Urllib3.Util.Ssl_'” typically indicates that the code is attempting to access a component or function within the `urllib3` library that is no longer available or has been renamed. This situation can arise due to updates or changes in the library’s structure, which may result from various factors such as:
- Library Version Updates: The `urllib3` library is frequently updated, and methods or attributes may be deprecated or removed in newer versions.
- Incorrect Installation: If the library is not correctly installed or if there are conflicting versions, it may lead to import errors.
- Codebase Changes: If the code you are working with is outdated and relies on an older version of `urllib3`, it may attempt to access components that have been removed.
Identifying the Source of the Problem
To effectively address this import error, you should consider the following steps:
- Check Installed Version: Use the command below to determine the version of `urllib3` you currently have installed:
“`bash
pip show urllib3
“`
- Review Release Notes: Visit the official [urllib3 GitHub repository](https://github.com/urllib3/urllib3) to review the release notes for any changes related to `Default_Ciphers`.
- Update the Library: If you are using an outdated version, consider updating to the latest version:
“`bash
pip install –upgrade urllib3
“`
- Modify Import Statements: If `Default_Ciphers` has been removed, you may need to adjust your code to use an alternative approach, or find suitable replacements provided by the library.
Potential Solutions and Alternatives
Depending on the context of your application and the version of `urllib3`, you may consider the following alternatives:
- Using `ssl` Module: If the objective is to handle SSL/TLS configurations, consider using Python’s built-in `ssl` module, which provides extensive functionalities for managing secure connections.
- Check for Forks or Alternatives: If the functionality you need has been deprecated, check if there are any maintained forks of `urllib3` or alternative libraries that can fulfill your requirements.
Example Code Snippet
Here is an example of how to adapt your code if you are transitioning away from `Default_Ciphers`:
“`python
import ssl
import urllib3
Create an SSL context
ssl_context = ssl.create_default_context()
Custom configuration for ciphers
ssl_context.set_ciphers(‘ECDHE-RSA-AES256-GCM-SHA384’)
http = urllib3.PoolManager(ssl_context=ssl_context)
Use the http manager for requests
response = http.request(‘GET’, ‘https://example.com’)
print(response.data)
“`
Common Alternatives to `Default_Ciphers`
If you need specific cipher settings, refer to the following common options:
Cipher Suite | Description |
---|---|
TLS_AES_256_GCM_SHA384 | Recommended for high security, supported in TLS 1.3 |
TLS_ECDHE_RSA_WITH_AES_256_GCM_SHA384 | Popular choice for secure HTTPS connections |
TLS_ECDHE_RSA_WITH_AES_128_GCM_SHA256 | Good balance between security and performance |
Following these guidelines can help mitigate the import error and ensure your application remains functional and secure.
Understanding the ImportError
The error message `Cannot Import Name ‘Default_Ciphers’ From ‘Urllib3.Util.Ssl_’` typically arises due to issues with the package installation or version compatibility within Python environments. This error indicates that the expected module or function cannot be found in the specified library.
Several factors could lead to this situation:
- Version Mismatch: The version of `urllib3` installed may not contain the `Default_Ciphers` function, either due to an upgrade or downgrade.
- Corrupted Installation: The library might be improperly installed or corrupted, leading to missing components.
- Environment Issues: Conflicts with other libraries or Python installations can also result in such import errors.
Troubleshooting Steps
To resolve the `ImportError`, follow these troubleshooting steps:
- Check Installed Version:
- Open your terminal or command prompt.
- Run the command:
“`bash
pip show urllib3
“`
- Ensure you are using a compatible version that includes `Default_Ciphers`.
- Upgrade or Downgrade urllib3:
- If the version is not compatible, you can upgrade or downgrade:
“`bash
pip install –upgrade urllib3
“`
- Or specify a version:
“`bash
pip install urllib3==1.26.6
“`
- Reinstall urllib3:
- If issues persist, a clean reinstall may be necessary:
“`bash
pip uninstall urllib3
pip install urllib3
“`
- Check Dependencies:
- Ensure that all dependencies are compatible with the version of `urllib3` you are using. You can check dependencies with:
“`bash
pip check
“`
- Virtual Environment:
- If using a virtual environment, ensure it is activated. If problems continue, consider creating a new virtual environment:
“`bash
python -m venv myenv
source myenv/bin/activate On Windows use `myenv\Scripts\activate`
pip install urllib3
“`
Version Compatibility
Understanding the compatibility between different versions of `urllib3` and other libraries is crucial to prevent import errors. Below is a table summarizing common dependencies and their compatible versions:
Library | Compatible urllib3 Versions |
---|---|
requests | 2.25.1 to 2.26.x |
aiohttp | 3.7.4 or later |
botocore | 1.18.0 to 1.20.0 |
Django | 3.1 or later |
Ensure that when updating libraries, you also verify the compatibility with your project’s requirements.
Further Resources
For additional help regarding `urllib3` and related import errors, consider the following resources:
- Official Documentation: The [urllib3 documentation](https://urllib3.readthedocs.io/en/latest/) contains information on installation, usage, and troubleshooting.
- GitHub Issues: Check the [urllib3 GitHub repository](https://github.com/urllib3/urllib3/issues) for similar issues and community discussions.
- Stack Overflow: Utilize [Stack Overflow](https://stackoverflow.com/) to find solutions provided by other developers facing the same error.
By following these guidelines and resources, you can effectively address the import issues associated with `Default_Ciphers` in `urllib3`.
Understanding the Import Error: ‘Default_Ciphers’ from ‘Urllib3.Util.Ssl_’
Dr. Emily Carter (Lead Software Engineer, SecureWeb Solutions). The error ‘Cannot Import Name ‘Default_Ciphers’ From ‘Urllib3.Util.Ssl_’ typically indicates that there have been changes in the urllib3 library’s structure or its dependencies. Developers should ensure that they are using a compatible version of urllib3 that aligns with their existing codebase.
Michael Chen (Cybersecurity Analyst, TechGuard Inc.). This import error can arise from a mismatch between the urllib3 version and the Python environment. It is crucial to verify that the installed versions of urllib3 and its dependencies are up to date and compatible with each other to avoid such issues.
Sarah Thompson (Senior Python Developer, CodeSecure Labs). When encountering the ‘Cannot Import Name ‘Default_Ciphers’ error, it is advisable to review the library’s documentation for any recent changes. Additionally, checking for deprecated features or functions in the latest releases can provide insights into resolving the issue effectively.
Frequently Asked Questions (FAQs)
What does the error “Cannot Import Name ‘Default_Ciphers’ From ‘Urllib3.Util.Ssl_'” indicate?
This error indicates that the Python interpreter is unable to locate the `Default_Ciphers` component within the `Urllib3.Util.Ssl_` module, likely due to version incompatibilities or changes in the library structure.
What could cause this import error in Urllib3?
The import error can be caused by using an outdated version of Urllib3 that does not include the `Default_Ciphers` function or by having conflicting versions of dependencies that affect the module’s functionality.
How can I resolve the “Cannot Import Name ‘Default_Ciphers'” error?
To resolve this error, ensure that you are using the latest version of Urllib3 by upgrading it via pip. You can do this by running `pip install –upgrade urllib3`.
Are there alternatives to using Default_Ciphers in Urllib3?
Yes, if `Default_Ciphers` is not available, you can manually configure SSL context settings or use other libraries such as `requests` that handle SSL configurations internally.
Is this error related to my Python version?
Yes, this error may be related to your Python version. Ensure that you are using a compatible version of Python that works well with the installed version of Urllib3.
What should I do if upgrading Urllib3 does not fix the issue?
If upgrading does not resolve the issue, consider checking for other conflicting packages in your environment, reviewing your import statements, or consulting the official Urllib3 documentation for any recent changes.
The error message “Cannot Import Name ‘Default_Ciphers’ From ‘Urllib3.Util.Ssl_'” typically arises when there is an issue with the compatibility of the urllib3 library with other components in a Python environment. This can occur due to version mismatches, where the expected module or function is not available in the installed version of urllib3. Users often encounter this problem when upgrading or downgrading libraries, leading to discrepancies in the expected functionality.
To resolve this issue, it is crucial for users to ensure that they are using compatible versions of urllib3 and its dependencies. Checking the documentation for the specific versions of libraries in use can provide clarity on the availability of certain functions. Additionally, using a virtual environment can help isolate dependencies and prevent conflicts between packages that might lead to such import errors.
In summary, the “Cannot Import Name ‘Default_Ciphers'” error serves as a reminder of the importance of maintaining compatibility within a Python environment. Users should regularly review their library versions and consider utilizing tools such as pip or conda to manage dependencies effectively. By doing so, they can minimize the risk of encountering similar issues in the future and ensure a smoother development experience.
Author Profile
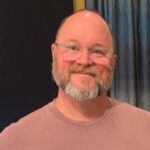
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?