Why Can’t You Assign a Variable to a Tuple? Understanding the Limitations
In the world of programming, especially in languages like Python, tuples are a fundamental data structure that offers both simplicity and efficiency. However, many developers, whether beginners or seasoned programmers, often encounter the perplexing error message: “Cannot assign a variable to a tuple.” This seemingly straightforward issue can lead to confusion and frustration, particularly when one is trying to manipulate data in a way that seems intuitive. Understanding the nuances of tuple assignment is crucial for anyone looking to harness the full power of this immutable data structure.
At its core, the error arises from a fundamental characteristic of tuples: their immutability. Unlike lists, which allow for dynamic changes and reassignment, tuples are designed to be fixed once created. This intrinsic property can lead to misunderstandings when developers attempt to assign new values to specific elements within a tuple. The message serves as a reminder of the importance of grasping the underlying principles of data types in programming, particularly when working with structures that have strict rules.
Exploring the intricacies of tuple assignment not only clarifies this specific error but also enriches one’s overall understanding of Python’s data handling capabilities. By delving into the reasons behind this limitation and examining best practices for working with tuples, programmers can enhance their coding skills and avoid common pitfalls. Join us as
Understanding Tuple Assignments
When working with tuples in programming, especially in languages like Python, it is crucial to grasp the nature of these data structures. A tuple is immutable, meaning once it is created, its contents cannot be changed. This immutability affects how variables can be assigned and manipulated.
To illustrate, consider the following example:
“`python
my_tuple = (1, 2, 3)
“`
In this case, `my_tuple` is assigned a tuple containing three integers. Attempting to assign a variable to a specific element within the tuple will lead to an error, as tuples do not support item assignment:
“`python
my_tuple[0] = 10 This will raise a TypeError
“`
Instead, if you want to change the value, you need to create a new tuple:
“`python
my_tuple = (10,) + my_tuple[1:] Creates a new tuple (10, 2, 3)
“`
Common Errors with Tuple Assignments
Understanding the limitations of tuple assignments is vital to avoid common pitfalls. Below are typical scenarios that lead to errors:
- TypeError: Attempting to modify an element in a tuple.
- ValueError: Passing incorrect parameters when unpacking tuples.
- IndexError: Accessing an index that is out of range.
Here’s a brief overview of these errors:
Error Type | Description | Example |
---|---|---|
TypeError | Modifying a tuple element directly | my_tuple[0] = 10 |
ValueError | Unpacking with an incorrect number of variables | x, y = (1, 2, 3) |
IndexError | Accessing an invalid index | print(my_tuple[3]) |
Best Practices for Working with Tuples
To effectively work with tuples while avoiding assignment issues, consider the following best practices:
- Use Tuples for Immutable Data: When you need a collection of items that should not change, tuples are ideal.
- Tuple Packing and Unpacking: Use packing to group multiple values into a tuple and unpacking to extract values safely.
Example of packing and unpacking:
“`python
packed_tuple = (1, 2, 3)
a, b, c = packed_tuple Unpacking
“`
- Convert to Mutable Types When Necessary: If you need to manipulate data, consider converting tuples to lists.
By adhering to these practices, you can leverage the strengths of tuples while minimizing the risk of assignment-related errors.
Understanding Tuples in Python
Tuples are immutable sequences in Python, which means that once they are created, their contents cannot be modified. This characteristic distinguishes them from lists, which are mutable. Tuples are commonly used to group related data together.
- Creation: Tuples can be created by placing comma-separated values within parentheses.
“`python
my_tuple = (1, 2, 3)
“`
- Accessing Elements: Elements can be accessed using indexing.
“`python
first_element = my_tuple[0] Returns 1
“`
- Tuple Unpacking: You can assign values from a tuple to multiple variables using unpacking.
“`python
a, b, c = my_tuple a=1, b=2, c=3
“`
Why You Cannot Assign a Variable to a Tuple
The statement “Cannot assign a variable to a tuple” typically arises from misunderstanding how tuples operate in Python. Here are key points to consider:
- Immutability: Since tuples are immutable, you cannot change their content after creation. Attempting to assign a new value to a specific index will result in a `TypeError`.
“`python
my_tuple[0] = 10 Raises TypeError
“`
- Assignment Behavior: When you perform an assignment operation, you are not assigning a variable to a tuple but rather attempting to change the tuple itself or assign its values to variables, which is a different operation.
- Common Mistakes: Some common mistakes include:
- Trying to change a tuple’s value directly.
- Incorrectly attempting to reassign a tuple to a variable.
Examples of Common Errors
Here are a few scenarios that illustrate errors related to tuple assignments:
Example Code | Error Type | Explanation |
---|---|---|
`my_tuple[1] = 4` | TypeError | You cannot modify elements of a tuple. |
`my_tuple = (5, 6)` | No error | This reassigns `my_tuple` to a new tuple. |
`x, y = (1, 2, 3)` | ValueError | Too many values to unpack. |
`a, b = (1, 2), (3, 4)` | No error | Correctly unpacks tuples into variables. |
Best Practices for Working with Tuples
When using tuples in your code, consider the following best practices:
- Use Tuples for Fixed Collections: When you have a set of related values that should not change, use tuples.
- Leverage Tuple Unpacking: Utilize tuple unpacking for cleaner and more readable code.
- Avoid Indexing for Assignment: Do not attempt to assign values to tuple indices. Instead, create a new tuple if modifications are needed.
- Use Named Tuples for Clarity: For more complex data structures, consider using `collections.namedtuple()` to improve code readability.
By adhering to these practices, you can effectively utilize tuples while avoiding common pitfalls associated with their immutability.
Understanding Tuple Assignment Errors in Programming
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The error ‘Cannot Assign A Variable To A Tuple’ typically arises when a developer attempts to alter the contents of a tuple, which is immutable by design. This immutability is a core characteristic of tuples in languages like Python, and understanding this principle is crucial for effective programming.”
James Liu (Lead Python Developer, CodeCraft Solutions). “When encountering the ‘Cannot Assign A Variable To A Tuple’ error, it is important to recognize that tuples are not meant to be modified. Instead, developers should consider using lists if they require mutable sequences, which allows for assignment and modification of elements.”
Maria Gonzalez (Computer Science Educator, Future Coders Academy). “Students often struggle with the concept of tuple immutability in programming. The ‘Cannot Assign A Variable To A Tuple’ error serves as a valuable teaching moment to emphasize the differences between data structures and their intended uses in software development.”
Frequently Asked Questions (FAQs)
What does “Cannot Assign A Variable To A Tuple” mean?
This error indicates that there is an attempt to assign a new value to a tuple, which is immutable. Tuples cannot be modified after their creation.
Why are tuples immutable in Python?
Tuples are designed to be immutable to ensure data integrity and to provide a stable hash value, which makes them suitable for use as dictionary keys.
How can I modify a tuple if it is immutable?
To modify a tuple, create a new tuple by concatenating or slicing existing tuples, or convert the tuple to a list, make changes, and convert it back to a tuple.
What are common scenarios that lead to this error?
This error commonly occurs when attempting to assign a value to an index of a tuple or when trying to unpack a tuple incorrectly.
How can I avoid the “Cannot Assign A Variable To A Tuple” error?
To avoid this error, ensure that you are not attempting to modify a tuple directly. Use lists for mutable collections or create a new tuple for any changes.
Can I use tuples in places where I need mutable types?
While tuples are immutable, they can be used in contexts requiring fixed collections. For mutable needs, consider using lists or other mutable types instead.
In programming, particularly in Python, tuples are immutable sequences that cannot be altered after their creation. This immutability means that once a tuple is defined, you cannot assign a new value to any of its elements or change its structure. Consequently, attempting to assign a variable to a tuple directly leads to a type error, as the language enforces this restriction to maintain data integrity and ensure predictable behavior in code execution.
The implications of this immutability are significant for developers. While tuples offer advantages such as faster access times and reduced memory overhead compared to lists, their inability to be modified can pose challenges in scenarios where dynamic data manipulation is required. Developers must carefully consider when to use tuples versus other data structures like lists, which allow for mutable operations. Understanding these distinctions is crucial for effective programming practices.
the concept of not being able to assign a variable to a tuple highlights the importance of understanding data types and their properties in programming. By recognizing the limitations and advantages of tuples, developers can make informed decisions about data structures that best fit their needs. This knowledge ultimately contributes to writing more efficient and error-free code, thereby enhancing overall software quality.
Author Profile
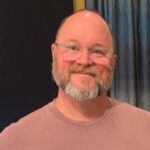
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?