Can You Use Double for Int in Programming? Exploring the Differences and Best Practices
In the world of programming, data types are fundamental building blocks that dictate how information is stored, manipulated, and interpreted. Among these types, integers and floating-point numbers, such as doubles, play crucial roles in various applications. However, a common question arises: Can you use double for int? This query opens a Pandora’s box of considerations, from precision and performance to the implications of type conversion. As we delve into this topic, we will explore the nuances of data types, the compatibility between them, and the potential pitfalls that developers may encounter when mixing doubles with integers.
When programming, understanding the distinction between data types is essential for writing efficient and error-free code. Integers, represented as whole numbers, are often used for counting and indexing, while doubles, or double-precision floating-point numbers, are employed for representing decimal values and performing complex calculations. The ability to interchange these types can lead to both advantages and challenges, particularly in terms of precision loss and memory usage.
As we navigate this intricate landscape, we will examine the scenarios where using a double in place of an int may be beneficial, as well as the potential issues that can arise. From the implications of type casting to the performance considerations in various programming languages, understanding the interplay between these data
Understanding Data Type Conversion
In programming, converting data types is a fundamental concept that allows developers to manipulate and utilize different forms of data. When dealing with numeric types, it’s common to encounter scenarios where one might need to convert a `double` to an `int`. This process can yield different results depending on the language and its handling of type conversion.
- A `double` is a data type that represents a double-precision 64-bit IEEE 754 floating point. It can hold decimal values, which makes it suitable for calculations requiring a high degree of precision.
- An `int` is a data type that represents a 32-bit signed integer, which cannot hold decimal values.
Conversion from a `double` to an `int` typically involves truncation of the decimal part. For instance:
- A `double` value of 5.99 would convert to an `int` value of 5.
- A `double` value of -3.14 would convert to an `int` value of -3.
Methods of Conversion
Different programming languages offer various methods for converting `double` values to `int`. Below are a few common approaches:
Language | Method | Description |
---|---|---|
Java | `(int) doubleValue` | Explicit casting to `int`. |
C | `Convert.ToInt32(doubleValue)` | Utilizes the Convert class for conversion. |
Python | `int(doubleValue)` | Directly converts using the `int()` function. |
JavaScript | `Math.floor(doubleValue)` | Rounds down to the nearest integer. |
Each method has its nuances. For instance, in Java, using `(int)` will simply truncate the decimal part, while using `Math.round(doubleValue)` will round it to the nearest integer.
Considerations When Converting
When converting from `double` to `int`, there are several important considerations to keep in mind:
- Loss of Precision: Any fractional part of a `double` will be lost, potentially impacting calculations or logic that rely on that precision.
- Range Limitations: The `int` type has a defined range (typically -2,147,483,648 to 2,147,483,647). A `double` value outside this range will cause an overflow error or unexpected behavior during conversion.
- Rounding Behavior: Depending on the method chosen, rounding can differ. Truncation will not account for the decimal value, while other methods may round up or down.
Best Practices
When performing conversions from `double` to `int`, adhere to the following best practices:
- Always validate the range of the `double` before conversion to prevent overflow.
- Choose a conversion method that aligns with the desired rounding behavior.
- Document any assumptions regarding precision loss in your code comments for clarity.
By being mindful of these considerations, developers can effectively manage data type conversions and ensure their applications run smoothly.
Understanding Data Types
In programming, data types define the kind of data a variable can hold. Two common types are integers (`int`) and double-precision floating-point numbers (`double`).
- Integer (`int`): Represents whole numbers without any decimal component.
- Double (`double`): Represents numbers that can contain fractions, enabling a broader range of values and precision.
Type Conversion between Double and Int
It is possible to use a `double` where an `int` is expected through type conversion, also known as type casting. However, this process comes with implications regarding precision and data integrity.
- Implicit Conversion: Some programming languages allow implicit conversion from `int` to `double` automatically without explicit syntax.
- Explicit Conversion: Converting from `double` to `int` requires explicit casting, which may truncate the decimal part.
Examples of Type Casting
Here are examples in various programming languages to illustrate the conversion between `double` and `int`:
Language | Implicit Conversion (int to double) | Explicit Conversion (double to int) |
---|---|---|
Java | `double d = i;` | `int i = (int) d;` |
C | `double d = i;` | `int i = (int) d;` |
Python | `d = float(i)` | `i = int(d)` |
C++ | `double d = i;` | `int i = static_cast |
Considerations for Using Double Instead of Int
When deciding to use `double` in place of `int`, several factors should be considered:
- Precision Loss: Converting from `double` to `int` can lead to loss of the fractional component.
- Performance: Operations on `int` are generally faster than those on `double` due to the lower computational overhead.
- Range of Values: `double` can represent a much wider range of numbers, including extremely large or small values, which may be necessary for certain applications.
When to Use Each Data Type
Choosing between `int` and `double` depends on the application requirements:
- Use `int` when:
- You need to perform calculations with whole numbers.
- Memory usage is a concern, as `int` typically consumes less memory than `double`.
- Precision is paramount, and fractions are not relevant.
- Use `double` when:
- You require fractional values for calculations.
- The range of values exceeds what can be handled by `int`.
- You are dealing with scientific computations where precision is needed.
Common Pitfalls
Developers should be aware of some common pitfalls when mixing `double` and `int`:
- Unexpected Rounding: When converting `double` to `int`, rounding occurs, which may lead to unexpected results.
- Type Mismatch Errors: Using `double` where `int` is expected can lead to compilation errors or exceptions, depending on the programming language.
- Performance Impact: Frequent conversions between types in performance-critical sections of code can lead to inefficiencies.
Understanding these nuances allows developers to make informed decisions about when and how to use `double` and `int` effectively in their code.
Understanding the Use of Double for Integer Values
Dr. Emily Carter (Software Engineer, Tech Innovations Inc.). “Using a double for integer values can lead to precision issues. While a double can represent a wider range of numbers, it is not inherently suitable for integers due to the potential for rounding errors. In critical applications, it is advisable to use integer types to maintain accuracy.”
James Liu (Lead Developer, CodeCraft Solutions). “In programming, while it is technically possible to use a double to store integer values, it is generally not recommended. The conversion from double to int can result in data loss, especially for large integers, and can complicate debugging and maintenance.”
Sarah Mitchell (Data Scientist, Analytics Pro). “From a data analysis perspective, using double for integer values may introduce unnecessary complexity. It is essential to choose the appropriate data type that reflects the nature of the data being processed, ensuring both performance and accuracy are optimized.”
Frequently Asked Questions (FAQs)
Can you directly assign a double value to an int variable?
No, you cannot directly assign a double value to an int variable without explicit casting, as this would lead to a loss of precision.
What happens if you cast a double to an int?
Casting a double to an int truncates the decimal part, resulting in only the integer portion being retained.
Is it safe to use double values for calculations involving integers?
While it is technically possible to use double for calculations involving integers, it may introduce rounding errors and loss of precision, which can affect the accuracy of results.
Can you use double in place of int in function parameters?
Yes, you can use double in place of int in function parameters, but it may require adjustments in how values are handled within the function.
What are the implications of using double instead of int in programming?
Using double instead of int can increase memory usage and processing time due to the larger size of double data types, and it may introduce floating-point arithmetic issues.
How can you convert a double to an int in programming?
You can convert a double to an int using explicit casting, such as `(int)myDouble`, or by using built-in functions like `Math.floor()` or `Math.round()` depending on the desired rounding behavior.
In programming, the question of whether you can use a double data type in place of an int is a nuanced topic that depends on the context and the programming language in use. Generally, a double is a floating-point type that can represent decimal values, while an int is a whole number. In many languages, you can assign a double to an int variable, but this often involves implicit or explicit type conversion, which can lead to data loss if the double contains a fractional component.
When using a double in place of an int, it is crucial to understand the implications of such a conversion. Implicit conversions may occur without explicit instructions, but they can result in truncation of the decimal portion. Explicit conversions, such as casting, provide more control and clarity, allowing developers to manage how values are converted and to handle potential errors or data loss effectively.
Key takeaways from this discussion include the importance of understanding data types and their behavior in programming. Developers should be cautious when mixing data types, as this can introduce bugs or unintended behavior in applications. Always consider the requirements of your program and choose the appropriate data type that aligns with your needs, ensuring that precision and performance are maintained.
Author Profile
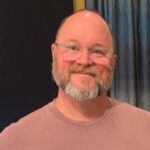
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?