Can You Use a Function Inside a Function in Python? Exploring the Nested Function Concept!
In the world of programming, the ability to create and utilize functions is one of the most powerful tools at your disposal. Functions not only help streamline your code, making it more organized and efficient, but they also allow for the reuse of code snippets across various parts of your application. But what happens when you want to take this concept a step further? Can you use a function inside another function in Python? The answer is a resounding yes, and this practice opens up a realm of possibilities for enhancing your coding capabilities.
When you nest functions in Python, you unlock a variety of programming paradigms, such as closures and higher-order functions. This technique allows for greater flexibility in your code, enabling you to create more complex behaviors while maintaining clarity. For instance, using a function within another function can help encapsulate logic, manage scope, and even facilitate the passing of parameters in a more controlled manner. This not only promotes cleaner code but also enhances the maintainability of your programs.
As you delve deeper into the intricacies of nested functions, you’ll discover how they can be effectively utilized in real-world scenarios. From simplifying repetitive tasks to managing state and data flow, understanding how to leverage functions within functions can significantly elevate your Python programming skills. Get ready to explore the nuances of this powerful
Defining Functions Inside Other Functions
In Python, it is entirely permissible to define a function within another function. This practice is often referred to as a “nested function” or “inner function.” The inner function can access variables from the outer function’s scope, which enables it to operate on data specific to the outer function’s context. This feature can be particularly useful for encapsulating functionality that is only relevant within the outer function.
For instance, consider the following example:
“`python
def outer_function(x):
def inner_function(y):
return y + 1
return inner_function(x) * 2
“`
In this example, `inner_function` is defined within `outer_function`. It takes one argument `y`, increments it by 1, and returns the result. The outer function then calls the inner function and multiplies its result by 2.
Benefits of Using Nested Functions
Nested functions can provide several advantages:
- Encapsulation: Inner functions can help keep the namespace clean by limiting the scope of variables and functions.
- Logical grouping: Related operations can be grouped together, making the code easier to understand and maintain.
- Access to outer variables: Inner functions can access variables defined in their outer function, which can be useful for maintaining state.
Limitations of Nested Functions
While nested functions can be beneficial, there are some limitations to consider:
- Scope: Inner functions cannot be accessed from outside their containing function, which can limit their reusability.
- Memory consumption: If an inner function retains references to variables in the outer function, it can lead to higher memory usage if the outer function is called multiple times.
Here’s a table summarizing the pros and cons of nested functions:
Advantages | Disadvantages |
---|---|
Encapsulation of logic | Limited scope |
Cleaner namespace | Potentially higher memory usage |
Access to outer variables | Reduced reusability |
Using Functions as Return Values
Another powerful feature in Python is the ability to return a function from another function. This creates a factory for functions, allowing you to generate functions tailored to specific needs. Here’s an example:
“`python
def make_multiplier(factor):
def multiply(x):
return x * factor
return multiply
“`
In this case, `make_multiplier` returns a new function `multiply`, which multiplies its input by a specified `factor`. This allows for the creation of multiple multiplier functions:
“`python
double = make_multiplier(2)
triple = make_multiplier(3)
print(double(5)) Outputs: 10
print(triple(5)) Outputs: 15
“`
Utilizing functions inside functions in Python enhances the flexibility and modularity of the code. This approach not only promotes better organization of code but also allows for the creation of functions that are tailored to specific tasks, thereby increasing the maintainability and readability of your codebase.
Using Functions Inside Functions in Python
Defining a function within another function is a common practice in Python, known as a nested function or inner function. This approach can help in organizing code and encapsulating functionality.
Benefits of Nested Functions
- Encapsulation: Inner functions can access variables from the enclosing function, which helps maintain a clean namespace.
- Modularity: Breaking down complex tasks into smaller, manageable inner functions improves readability and maintainability.
- Closure: Nested functions can form closures, retaining access to the outer function’s scope even after the outer function has finished executing.
Syntax and Example
Here is a basic syntax structure for defining a nested function:
“`python
def outer_function(param):
def inner_function(inner_param):
Do something with inner_param
return inner_param * 2
return inner_function(param)
“`
Example Usage
“`python
def calculate_area(shape, dimension):
def area_circle(radius):
return 3.14 * radius * radius
def area_square(side):
return side * side
if shape == ‘circle’:
return area_circle(dimension)
elif shape == ‘square’:
return area_square(dimension)
else:
return “Unknown shape”
Calling the function
print(calculate_area(‘circle’, 5)) Output: 78.5
print(calculate_area(‘square’, 4)) Output: 16
“`
Important Considerations
- Scope: The inner function can access variables from the outer function but not vice versa.
- Performance: Excessive use of nested functions can lead to performance overhead due to increased function calls. Use them judiciously.
- Readability: While nesting can enhance encapsulation, it may also reduce readability if overused. Always strive for a balance.
Use Cases for Nested Functions
Nested functions are particularly useful in scenarios such as:
- Callbacks: When a function requires a callback mechanism, nested functions can serve as local callbacks.
- Decorator Functions: In Python, decorators often utilize nested functions to wrap another function while retaining its identity.
- Stateful Functions: When maintaining state is necessary across multiple invocations of a function.
Best Practices
- Limit the complexity of inner functions to maintain clarity.
- Avoid using nested functions when simpler alternatives exist, such as defining multiple top-level functions.
- Document your code well, especially when using nested functions, to clarify their purpose and usage.
By leveraging nested functions wisely, you can enhance the functionality and organization of your Python code, making it more efficient and manageable.
Understanding Nested Functions in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Corp). “Using a function inside another function, commonly referred to as a nested function, is a powerful feature in Python. It allows for better organization of code and encapsulation of functionality, making it easier to manage complex operations.”
Michael Chen (Python Developer, CodeCraft Solutions). “Nested functions can access variables from the enclosing scope, which is particularly useful for creating closures. This capability enhances code reusability and can lead to more efficient programming patterns.”
Sarah Thompson (Computer Science Professor, University of Technology). “While using functions inside functions can improve code structure, developers should be cautious of potential readability issues. It is essential to maintain clarity, especially in larger projects where nested functions may complicate the flow of logic.”
Frequently Asked Questions (FAQs)
Can you use a function inside another function in Python?
Yes, you can define a function within another function in Python. This is known as a nested function or inner function, and it can access variables from the enclosing function’s scope.
What are the benefits of using nested functions in Python?
Nested functions can help encapsulate functionality, maintain cleaner code, and limit the scope of helper functions to the outer function. This enhances readability and reduces the chance of name clashes.
Can inner functions return values to the outer function?
Yes, inner functions can return values to the outer function. The outer function can then use these returned values for further processing or output.
How do closures relate to nested functions in Python?
Closures occur when a nested function captures the local variables from the enclosing function’s scope. This allows the inner function to remember the environment in which it was created, even after the outer function has finished executing.
Are there any performance implications of using nested functions in Python?
While using nested functions can improve code organization, they may introduce slight performance overhead due to the additional scope management. However, this impact is generally negligible for most applications.
Can you call an inner function from outside its enclosing function?
No, an inner function cannot be called directly from outside its enclosing function. It is only accessible within the scope of the outer function unless returned or exposed in some way.
In Python, it is entirely permissible to use a function inside another function, a practice commonly referred to as “nested functions.” This capability allows for greater modularity and organization within code, enabling developers to encapsulate functionality and maintain cleaner code structures. Nested functions can access variables from their enclosing scope, which can be beneficial for maintaining state or controlling access to certain variables.
One of the key advantages of using functions within functions is the ability to create closures. A closure allows the inner function to remember the environment in which it was created, even after the outer function has finished executing. This feature can be particularly useful for creating decorators or managing state in a controlled manner, enhancing the flexibility of the code.
Moreover, nesting functions can improve code readability and maintainability by grouping related operations together. However, it is essential to use this feature judiciously, as excessive nesting can lead to code that is difficult to understand and debug. Striking a balance between modularity and simplicity is crucial for effective programming in Python.
Author Profile
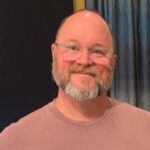
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?